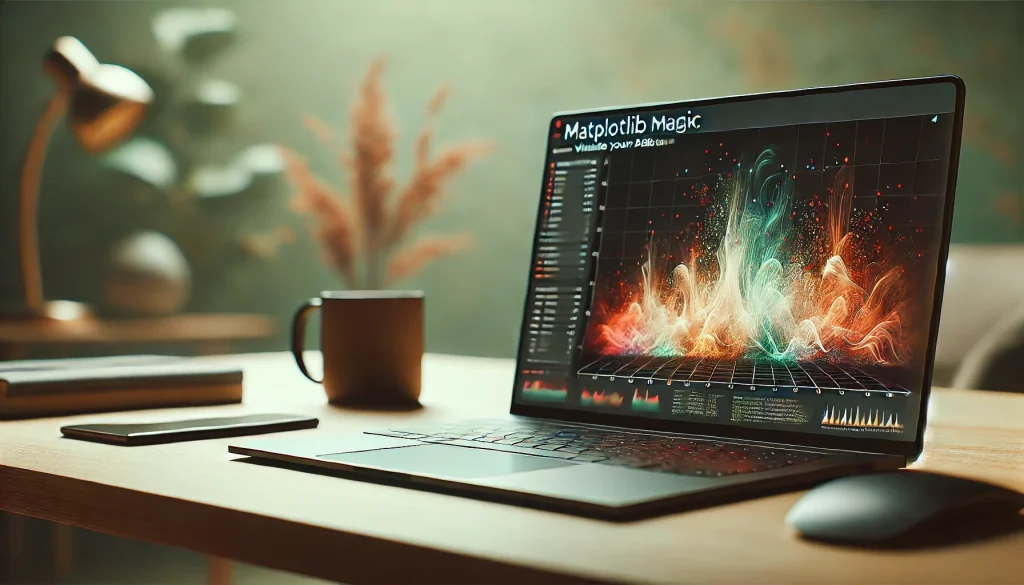
Matplotlib Magic: Visualize Your AI Data Like a Pro
Hello, data enthusiasts! If you’ve stumbled upon this blog, chances are you’re looking to elevate your data visualization skills. Whether you’re a college student, a budding data scientist, or a young professional, you’ve come to the right place. Today, we’re diving deep into the world of Matplotlib, a powerful library in Python that’s essential for visualizing AI data like a pro.
Matplotlib is your gateway to transforming complex data into insightful visuals. Let’s embark on this journey together, uncovering the magic behind Matplotlib and how you can leverage it to present your AI data compellingly.
Why Matplotlib?
Before we dive into the nitty-gritty, let’s address the elephant in the room: Why Matplotlib? There are numerous data visualization tools out there, so why should you choose this one?
Matplotlib is incredibly versatile and widely used in the data science community. It integrates seamlessly with other Python libraries like NumPy and pandas, making it the go-to choice for many data scientists. With Matplotlib, you can create a wide range of static, animated, and interactive plots, ensuring your data tells the right story.
Getting Started with Matplotlib
Installation
First things first, you need to have Matplotlib installed. If you haven’t installed it yet, you can do so using pip:
pip install matplotlib
Once you have Matplotlib installed, you’re ready to start visualizing your data.
Basic Plot
Let’s start with a simple plot. The code snippet below demonstrates how to create a basic line plot.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a line plot
plt.plot(x, y)
# Add title and labels
plt.title("Basic Line Plot")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# Show plot
plt.show()
In this snippet, we import pyplot
from Matplotlib, define our data points, and use the plot()
function to create a line plot. We also add a title and labels to our axes to make the plot more informative.
Customizing Your Plots
One of the best things about Matplotlib is its flexibility. You can customize almost every aspect of your plots to make them as informative and aesthetically pleasing as possible.
Changing Line Styles and Colors
You can change the style and color of your lines to make your plots more visually appealing. Here’s how:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a line plot with customized style and color
plt.plot(x, y, linestyle='--', color='r', marker='o')
# Add title and labels
plt.title("Customized Line Plot")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# Show plot
plt.show()
In this example, we use the linestyle
, color
, and marker
parameters to customize the appearance of our plot. The result is a dashed red line with circular markers at each data point.
Adding Legends
Legends are crucial for making your plots understandable, especially when dealing with multiple data series. Here’s how to add a legend to your plot:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y1 = [2, 3, 5, 7, 11]
y2 = [1, 4, 6, 8, 10]
# Create line plots
plt.plot(x, y1, label='Series 1')
plt.plot(x, y2, label='Series 2')
# Add title, labels, and legend
plt.title("Plot with Legend")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.legend()
# Show plot
plt.show()
Here, we add a label
to each data series and use the legend()
function to display the legend on the plot.
Advanced Plotting Techniques
Now that we’ve covered the basics, let’s move on to some advanced plotting techniques that can help you create more complex and informative visualizations.
Subplots
Sometimes, you need to display multiple plots in a single figure. This is where subplots come in handy. Here’s how to create subplots in Matplotlib:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y1 = [2, 3, 5, 7, 11]
y2 = [1, 4, 6, 8, 10]
# Create subplots
fig, (ax1, ax2) = plt.subplots(1, 2)
# Plot data on each subplot
ax1.plot(x, y1)
ax1.set_title("Subplot 1")
ax2.plot(x, y2)
ax2.set_title("Subplot 2")
# Show plot
plt.show()
In this example, we use the subplots()
function to create a figure with two subplots arranged in a single row.
Histograms
Histograms are useful for visualizing the distribution of a dataset. Here’s how to create a histogram in Matplotlib:
import matplotlib.pyplot as plt
import numpy as np
# Generate random data
data = np.random.randn(1000)
# Create a histogram
plt.hist(data, bins=30, alpha=0.5, color='b')
# Add title and labels
plt.title("Histogram")
plt.xlabel("Value")
plt.ylabel("Frequency")
# Show plot
plt.show()
In this example, we use the hist()
function to create a histogram with 30 bins and a semi-transparent blue color.
Visualizing AI Data
When it comes to AI and machine learning, visualizing data effectively can help you gain insights and communicate your findings more effectively. Let’s explore some common types of visualizations used in AI.
Scatter Plots
Scatter plots are great for visualizing the relationship between two variables. Here’s an example:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a scatter plot
plt.scatter(x, y, color='g', marker='x')
# Add title and labels
plt.title("Scatter Plot")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# Show plot
plt.show()
In this example, we use the scatter()
function to create a scatter plot with green ‘x’ markers.
Confusion Matrix
A confusion matrix is a useful tool for evaluating the performance of a classification model. Here’s how to visualize a confusion matrix using Matplotlib:
import matplotlib.pyplot as plt
import numpy as np
# Sample confusion matrix data
confusion_matrix = np.array([[50, 10], [5, 35]])
# Create a heatmap
plt.imshow(confusion_matrix, cmap='Blues', interpolation='nearest')
# Add title and labels
plt.title("Confusion Matrix")
plt.xlabel("Predicted Label")
plt.ylabel("True Label")
# Add color bar
plt.colorbar()
# Show plot
plt.show()
In this example, we use the imshow()
function to create a heatmap of the confusion matrix, with a color bar indicating the scale.
Tips and Tricks for Professional-Looking Plots
To wrap up our journey, here are some tips and tricks to make your plots look more professional and polished.
Consistent Styling
Consistency is key to making your plots look professional. Use consistent colors, fonts, and styles across all your plots.
Annotations
Annotations can help you highlight important points in your plot. Here’s an example:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a line plot
plt.plot(x, y)
# Add annotation
plt.annotate('Peak', xy=(4, 7), xytext=(3, 8),
arrowprops=dict(facecolor='black', shrink=0.05))
# Add title and labels
plt.title("Plot with Annotation")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# Show plot
plt.show()
In this example, we use the annotate()
function to add an annotation with an arrow pointing to the peak of the line plot.
Saving Your Plots
You can save your plots as image files using the savefig()
function. Here’s how:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a line plot
plt.plot(x, y)
# Save plot
plt.savefig('line_plot.png')
# Show plot
plt.show()
In this example, we save the plot as a PNG file before displaying it.
Conclusion
And there you have it! A comprehensive guide to using Matplotlib for visualizing your AI data like a pro. By now, you should have a solid understanding of how to create, customize, and enhance your plots using Matplotlib. Remember, practice makes perfect, so keep experimenting with different styles and techniques to find what works best for your data.
Whether you’re presenting your findings to a team or exploring data on your own, Matplotlib is a powerful tool in your data visualization arsenal. Keep exploring its features, and you’ll soon master the art of turning raw data into insightful, compelling visuals.
Happy plotting!
Disclaimer: This blog post is intended for educational purposes. While we strive for accuracy, errors may occur. Please report any inaccuracies so we can correct them promptly.