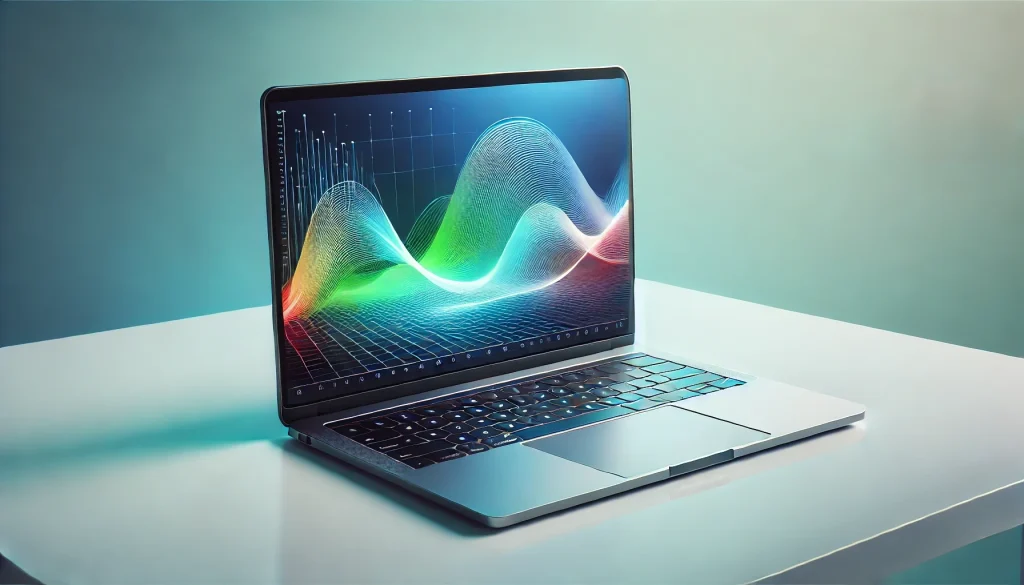
Matplotlib Masterclass: Create Stunning Visualizations for Your AI Projects
Welcome to our Matplotlib Masterclass, where you’ll learn how to create stunning visualizations for your AI projects. Whether you’re a college student diving into data science or a young professional working on cutting-edge AI applications, mastering Matplotlib can significantly enhance your data presentation skills. In this comprehensive guide, we’ll walk you through the essentials, provide plenty of sample codes, and ensure you can create visualizations that not only look great but also convey your insights effectively.
Why Matplotlib?
Powerful and Versatile: Matplotlib is one of the most popular Python libraries for data visualization. Its versatility allows you to create a wide range of static, animated, and interactive plots.
Highly Customizable: With Matplotlib, you can fine-tune every aspect of your plot to match your specific needs and preferences. This level of customization is essential when presenting AI project results to different audiences.
Wide Range of Plot Types: From basic line and bar charts to complex 3D plots and heatmaps, Matplotlib covers a wide range of visualization needs, making it a go-to tool for AI researchers and data scientists.
Getting Started with Matplotlib
Before diving into the advanced features, let’s ensure you have Matplotlib installed and understand the basics.
Installation
To install Matplotlib, you can use pip:
pip install matplotlib
Once installed, you can start creating your first plot.
Your First Plot
Let’s create a simple line plot to get a feel for Matplotlib.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Creating the plot
plt.plot(x, y)
# Adding a title
plt.title('Simple Line Plot')
# Adding labels
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Displaying the plot
plt.show()
This script creates a basic line plot with labeled axes and a title. It’s a great starting point for more complex visualizations.
Customizing Your Plots
To create truly stunning visualizations, customization is key. Matplotlib allows you to customize almost every aspect of your plots.
Titles and Labels
Adding informative titles and labels is crucial for making your plots understandable.
# Adding title and labels
plt.title('Sample Plot Title', fontsize=14, fontweight='bold')
plt.xlabel('X Axis Label', fontsize=12)
plt.ylabel('Y Axis Label', fontsize=12)
Legends
Legends help distinguish different datasets in your plot.
# Sample data
x1 = [1, 2, 3, 4, 5]
y1 = [2, 3, 5, 7, 11]
x2 = [1, 2, 3, 4, 5]
y2 = [1, 4, 6, 8, 10]
# Creating the plot
plt.plot(x1, y1, label='Dataset 1')
plt.plot(x2, y2, label='Dataset 2')
# Adding a legend
plt.legend()
Colors and Styles
Using different colors and line styles can make your plots more engaging.
# Creating the plot with custom styles
plt.plot(x1, y1, color='blue', linestyle='--', marker='o', label='Dataset 1')
plt.plot(x2, y2, color='red', linestyle='-', marker='x', label='Dataset 2')
Grid and Background
Adding a grid and customizing the background can enhance readability.
# Adding grid
plt.grid(True)
# Customizing background color
plt.gca().set_facecolor('lightgrey')
Advanced Plotting Techniques
Now that you understand the basics, let’s explore some advanced plotting techniques that will make your visualizations stand out.
Subplots
Creating multiple plots in a single figure can help compare different datasets effectively.
# Creating subplots
fig, axs = plt.subplots(2, 2)
# First subplot
axs[0, 0].plot(x1, y1, 'tab:blue')
axs[0, 0].set_title('Plot 1')
# Second subplot
axs[0, 1].plot(x2, y2, 'tab:orange')
axs[0, 1].set_title('Plot 2')
# Third subplot
axs[1, 0].plot(x1, y2, 'tab:green')
axs[1, 0].set_title('Plot 3')
# Fourth subplot
axs[1, 1].plot(x2, y1, 'tab:red')
axs[1, 1].set_title('Plot 4')
# Display the plots
plt.tight_layout()
plt.show()
Bar Charts
Bar charts are excellent for comparing categorical data.
# Sample data
categories = ['A', 'B', 'C', 'D']
values = [10, 15, 7, 10]
# Creating the bar chart
plt.bar(categories, values, color='skyblue')
# Adding title and labels
plt.title('Bar Chart Example')
plt.xlabel('Categories')
plt.ylabel('Values')
# Displaying the plot
plt.show()
Histograms
Histograms are useful for showing the distribution of a dataset.
# Sample data
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5]
# Creating the histogram
plt.hist(data, bins=5, color='purple', edgecolor='black')
# Adding title and labels
plt.title('Histogram Example')
plt.xlabel('Value')
plt.ylabel('Frequency')
# Displaying the plot
plt.show()
Scatter Plots
Scatter plots are great for visualizing relationships between two variables.
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Creating the scatter plot
plt.scatter(x, y, color='green', marker='x')
# Adding title and labels
plt.title('Scatter Plot Example')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Displaying the plot
plt.show()
Pie Charts
Pie charts can be effective for showing proportions.
# Sample data
labels = ['A', 'B', 'C', 'D']
sizes = [15, 30, 45, 10]
# Creating the pie chart
plt.pie(sizes, labels=labels, autopct='%1.1f%%', startangle=140)
# Adding title
plt.title('Pie Chart Example')
# Displaying the plot
plt.show()
Creating Interactive Visualizations
While static plots are useful, interactive visualizations can provide a more engaging experience. Matplotlib can be combined with other libraries to create interactive plots.
Using mplcursors for Interactivity
mplcursors is a library that allows you to add interactive cursors to your Matplotlib plots.
import matplotlib.pyplot as plt
import mplcursors
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Creating the plot
fig, ax = plt.subplots()
ax.plot(x, y, 'o-')
# Adding interactive cursor
mplcursors.cursor(hover=True)
# Displaying the plot
plt.show()
Interactive Widgets with ipywidgets
ipywidgets can be used to create interactive widgets in Jupyter Notebooks.
import matplotlib.pyplot as plt
import numpy as np
import ipywidgets as widgets
from ipywidgets import interact
# Sample data
x = np.linspace(0, 2 * np.pi, 100)
# Interactive plot function
def plot_function(freq):
y = np.sin(freq * x)
plt.plot(x, y)
plt.title(f'Sine Wave with Frequency {freq}')
plt.show()
# Creating interactive widget
interact(plot_function, freq=widgets.FloatSlider(value=1, min=0.1, max=5, step=0.1))
Incorporating Matplotlib into AI Projects
Visualizations play a crucial role in AI projects, from data exploration to presenting final results. Here are some examples of how you can use Matplotlib in your AI projects.
Data Exploration and Analysis
During the data exploration phase, visualizations can help you understand the distribution and relationships within your data.
import pandas as pd
# Sample data
data = {
'Feature1': [1, 2, 3, 4, 5],
'Feature2': [2, 3, 5, 7, 11],
'Target': [0, 1, 0, 1, 0]
}
df = pd.DataFrame(data)
# Creating scatter plot to explore relationships
plt.scatter(df['Feature1'], df['Feature2'], c=df['Target'], cmap='viridis')
plt.title('Feature1 vs Feature2')
plt.xlabel('Feature1')
plt.ylabel('Feature2')
plt.colorbar(label='Target')
plt.show()
Model Performance Evaluation
Visualizing model performance can provide insights into how well your AI models are performing.
from sklearn.metrics import confusion_matrix
import seaborn as sns
# Sample confusion matrix data
y_true = [0, 1, 0, 1, 0, 1, 0, 0, 1, 1]
y_pred = [0, 1, 0, 0, 0, 1, 0, 1, 1, 1]
# Creating the confusion matrix
cm = confusion_matrix(y_true, y_pred)
# Visualizing the confusion matrix
plt.figure(figsize=(8, 6))
sns.heatmap(cm, annot=True, fmt='d', cmap='Blues')
plt.title('Confusion Matrix')
plt.xlabel('Predicted Label')
plt.ylabel('True Label')
plt.show()
Training Progress Visualization
Visualizing the training process helps in understanding how your model is learning over time.
# Sample data for training loss and accuracy
epochs = range(1, 11)
loss = [0.8, 0.6, 0.5, 0.4, 0.35, 0.3, 0.25, 0.2, 0.15, 0.1]
accuracy = [0.5, 0.6, 0.65, 0.7, 0.75, 0.78, 0.82, 0.85, 0.88, 0.9]
# Creating the plot
plt.figure(figsize=(12, 5))
# Plotting loss
plt.subplot(1, 2, 1)
plt.plot(epochs, loss, 'o-r')
plt.title('Training Loss')
plt.xlabel('Epochs')
plt.ylabel('Loss')
# Plotting accuracy
plt.subplot(1, 2, 2)
plt.plot(epochs, accuracy, 'o-b')
plt.title('Training Accuracy')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
# Displaying the plots
plt.tight_layout()
plt.show()
Enhancing Your Visualizations
Adding the final touches can turn a good plot into a great one. Here are some tips for enhancing your visualizations:
Annotations
Annotations can highlight important parts of your plot.
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Creating the plot
plt.plot(x, y, 'o-')
# Adding annotation
plt.annotate('Peak', xy=(4, 7), xytext=(3, 6),
arrowprops=dict(facecolor='black', shrink=0.05))
# Adding title and labels
plt.title('Annotated Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Displaying the plot
plt.show()
3D Plots
3D plots can be particularly useful for visualizing complex datasets.
from mpl_toolkits.mplot3d import Axes3D
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
z = [1, 4, 9, 16, 25]
# Creating the 3D plot
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(x, y, z, c='r', marker='o')
# Adding title and labels
ax.set_title('3D Scatter Plot')
ax.set_xlabel('X Axis')
ax.set_ylabel('Y Axis')
ax.set_zlabel('Z Axis')
# Displaying the plot
plt.show()
Heatmaps
Heatmaps are ideal for visualizing matrices and showing data density.
import numpy as np
# Sample data
data = np.random.rand(10, 10)
# Creating the heatmap
plt.imshow(data, cmap='hot', interpolation='nearest')
# Adding title and color bar
plt.title('Heatmap Example')
plt.colorbar()
# Displaying the plot
plt.show()
Combining Plots
Combining different types of plots can provide a comprehensive view of your data.
# Sample data
x = np.linspace(0, 2 * np.pi, 100)
y1 = np.sin(x)
y2 = np.cos(x)
# Creating the plot
fig, ax1 = plt.subplots()
# Plotting the first dataset
ax1.plot(x, y1, 'b-')
ax1.set_xlabel('X Axis')
ax1.set_ylabel('Sin', color='b')
# Creating a second y-axis
ax2 = ax1.twinx()
ax2.plot(x, y2, 'r-')
ax2.set_ylabel('Cos', color='r')
# Adding title
plt.title('Combined Plot Example')
# Displaying the plot
plt.show()
Conclusion
Mastering Matplotlib is a valuable skill for anyone involved in AI and data science. This powerful library allows you to create a wide range of visualizations, from basic plots to complex interactive graphics. By customizing your plots, you can effectively communicate your data insights and make your AI projects stand out.
We’ve covered the basics, explored advanced plotting techniques, and shown how to enhance your visualizations with annotations, 3D plots, and more. With the sample codes provided, you should be well-equipped to start creating stunning visualizations for your AI projects.
Additional Resources
To further deepen your understanding of Matplotlib and data visualization, consider exploring the following resources:
Disclaimer
This blog aims to provide accurate and helpful information about Matplotlib and its uses in AI projects. However, if you find any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.