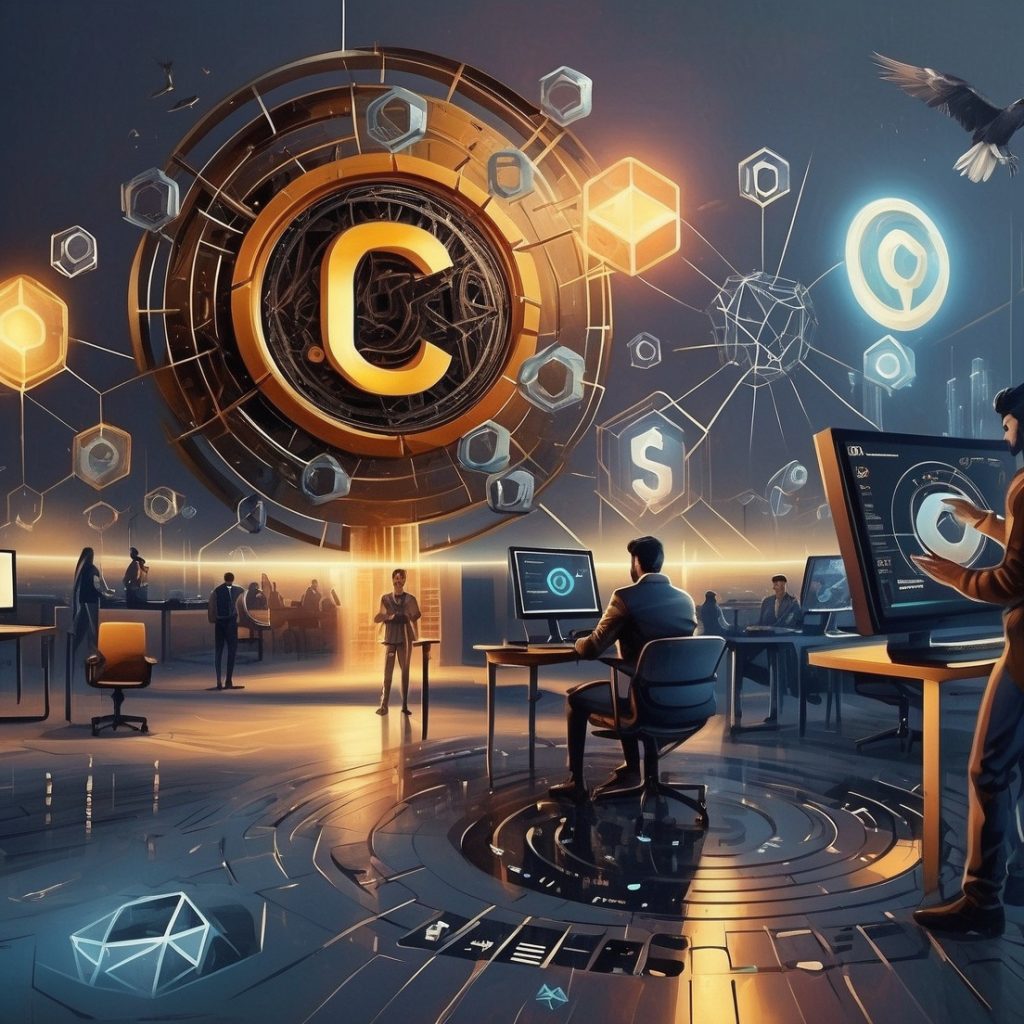
Minting Magic: Unveiling the Secrets of Token Creation on Cronos
Creating and managing tokens has become a central aspect of blockchain technology, driving innovations in decentralized finance (DeFi), gaming, supply chain, and more. Cronos, as a blockchain platform, offers unique capabilities for token creation. In this blog, we will delve into the secrets of token creation on Cronos, exploring its ecosystem, providing detailed guides, and presenting sample codes to illustrate the process.
Understanding Cronos: A Brief Overview
What is Cronos?
Cronos is a blockchain network designed to facilitate the seamless interaction between decentralized applications (dApps) and users. Leveraging the capabilities of both Ethereum and Cosmos, Cronos aims to provide a scalable and interoperable environment for developers. Its compatibility with Ethereum’s EVM (Ethereum Virtual Machine) allows developers to deploy and manage Ethereum-based dApps and tokens on Cronos with ease.
Why Choose Cronos for Token Creation?
Choosing Cronos for token creation comes with several advantages:
- Scalability: Cronos can handle a high volume of transactions, making it suitable for applications requiring quick and efficient processing.
- Interoperability: Built on Cosmos SDK and Tendermint, Cronos enables interoperability between different blockchains.
- Low Fees: Transaction costs on Cronos are significantly lower compared to Ethereum, making it economically viable for various use cases.
- Developer-Friendly: With support for Ethereum-based tools and languages, developers can easily transition their projects to Cronos.
Setting Up Your Environment
Prerequisites
Before diving into token creation on Cronos, ensure you have the following prerequisites in place:
- Node.js: Install Node.js from here.
- NPM: Node Package Manager comes with Node.js.
- Metamask: A crypto wallet that supports Cronos.
- Cronos Testnet Faucet: Obtain test CRO tokens from the Cronos faucet.
Installing Dependencies
To start with token creation, you need to set up your development environment. Follow these steps:
- Install Truffle Suite: Truffle is a development environment, testing framework, and asset pipeline for Ethereum, aiming to make life as a developer easier.
npm install -g truffle
- Set Up a New Truffle Project: Create a new directory for your project and initialize it with Truffle.
mkdir crono-token
cd crono-token
truffle init
- Install OpenZeppelin Contracts: OpenZeppelin provides secure and community-reviewed smart contracts for Ethereum.
npm install @openzeppelin/contracts
Writing Your First Token Contract
Creating the Token Contract
In this section, we will create a basic ERC-20 token contract using OpenZeppelin. ERC-20 is a standard for fungible tokens on the Ethereum blockchain.
- Create a New Contract File: Navigate to the
contracts
directory and create a new file namedMyToken.sol
.
// contracts/MyToken.sol
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
}
In this code:
- We import the ERC-20 contract from OpenZeppelin.
- We define a constructor that mints an initial supply of tokens to the contract creator.
- Compile the Contract: Compile the contract using Truffle.
truffle compile
Deploying the Token Contract
Deployment Script
To deploy the contract to the Cronos testnet, you need a deployment script.
- Create a Deployment Script: In the
migrations
directory, create a new file named2_deploy_contracts.js
.
// migrations/2_deploy_contracts.js
const MyToken = artifacts.require("MyToken");
module.exports = function (deployer) {
const initialSupply = web3.utils.toWei('1000', 'ether'); // Adjust the initial supply as needed
deployer.deploy(MyToken, initialSupply);
};
- Configure Truffle for Cronos: Update the
truffle-config.js
file to include the Cronos testnet configuration.
// truffle-config.js
const HDWalletProvider = require('@truffle/hdwallet-provider');
module.exports = {
networks: {
cronosTestnet: {
provider: () => new HDWalletProvider('YOUR_METAMASK_MNEMONIC', 'https://cronos-testnet.crypto.org:8545'),
network_id: 338,
confirmations: 2,
timeoutBlocks: 200,
skipDryRun: true
}
},
compilers: {
solc: {
version: "0.8.0"
}
}
};
Replace 'YOUR_METAMASK_MNEMONIC'
with your Metamask wallet mnemonic.
- Deploy the Contract: Deploy the contract to the Cronos testnet.
truffle migrate --network cronosTestnet
Interacting with the Deployed Contract
Verifying Deployment
Once the contract is deployed, you can verify it on the Cronos explorer.
- Get Contract Address: The contract address will be displayed in the Truffle migration output. You can also find it in the
build/contracts
directory under theMyToken.json
file. - Add Token to Metamask: Open Metamask, go to the “Assets” tab, and click “Add Token.” Enter the contract address to add your newly created token to your wallet.
Writing a Simple Script to Interact with the Contract
- Install Web3.js: Web3.js is a JavaScript library for interacting with the Ethereum blockchain.
npm install web3
- Create a Script to Check the Balance: Create a new file named
check_balance.js
.
// check_balance.js
const Web3 = require('web3');
const MyToken = require('./build/contracts/MyToken.json');
const web3 = new Web3('https://cronos-testnet.crypto.org:8545');
const contractAddress = 'YOUR_CONTRACT_ADDRESS';
const accountAddress = 'YOUR_ACCOUNT_ADDRESS';
const contract = new web3.eth.Contract(MyToken.abi, contractAddress);
async function checkBalance() {
const balance = await contract.methods.balanceOf(accountAddress).call();
console.log(`Balance: ${web3.utils.fromWei(balance, 'ether')} MTK`);
}
checkBalance();
Replace YOUR_CONTRACT_ADDRESS
and YOUR_ACCOUNT_ADDRESS
with the appropriate values.
- Run the Script: Execute the script to check the balance.
node check_balance.js
Advanced Token Features
Minting and Burning Tokens
Minting and burning tokens are essential features for managing token supply.
- Extend the Token Contract: Update the
MyToken.sol
contract to include minting and burning functionalities.
// contracts/MyToken.sol
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyToken is ERC20, Ownable {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
function mint(address to, uint256 amount) public onlyOwner {
_mint(to, amount);
}
function burn(uint256 amount) public {
_burn(msg.sender, amount);
}
}
- Deploy the Updated Contract: Recompile and deploy the updated contract using Truffle.
truffle compile
truffle migrate --network cronosTestnet
Interacting with Minting and Burning Functions
- Create a Script to Mint Tokens: Create a new file named
mint_tokens.js
.
// mint_tokens.js
const Web3 = require('web3');
const MyToken = require('./build/contracts/MyToken.json');
const HDWalletProvider = require('@truffle/hdwallet-provider');
const provider = new HDWalletProvider('YOUR_METAMASK_MNEMONIC', 'https://cronos-testnet.crypto.org:8545');
const web3 = new Web3(provider);
const contractAddress = 'YOUR_CONTRACT_ADDRESS';
const contract = new web3.eth.Contract(MyToken.abi, contractAddress);
async function mintTokens() {
const accounts = await web3.eth.getAccounts();
const amount = web3.utils.toWei('100', 'ether');
await contract.methods.mint(accounts[0], amount).send({ from: accounts[0] });
console.log(`Minted 100 MTK to ${accounts[0]}`);
}
mintTokens();
- Create a Script to Burn Tokens: Create a new file named
burn_tokens.js
.
// burn_tokens.js const Web3 = require('web3');
const MyToken = require('./build/contracts/MyToken.json');
const HDWalletProvider = require
const HDWalletProvider = require('@truffle/hdwallet-provider');
const provider = new HDWalletProvider('YOUR_METAMASK_MNEMONIC', 'https://cronos-testnet.crypto.org:8545');
const web3 = new Web3(provider);
const contractAddress = 'YOUR_CONTRACT_ADDRESS';
const contract = new web3.eth.Contract(MyToken.abi, contractAddress);
async function burnTokens() {
const accounts = await web3.eth.getAccounts();
const amount = web3.utils.toWei('50', 'ether');
await contract.methods.burn(amount).send({ from: accounts[0] });
console.log(`Burned 50 MTK from ${accounts[0]}`);
}
burnTokens();
- Run the Scripts: Execute the scripts to mint and burn tokens.
node mint_tokens.js
node burn_tokens.js
Enhancing Token Functionality with Advanced Features
Implementing Pausable Token
Pausable tokens allow the contract owner to pause and unpause all token transfers. This can be useful in emergency scenarios.
- Update the Token Contract: Modify the
MyToken.sol
contract to include pausable functionality.
// contracts/MyToken.sol
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/security/Pausable.sol";
contract MyToken is ERC20, Ownable, Pausable {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
function mint(address to, uint256 amount) public onlyOwner {
_mint(to, amount);
}
function burn(uint256 amount) public {
_burn(msg.sender, amount);
}
function pause() public onlyOwner {
_pause();
}
function unpause() public onlyOwner {
_unpause();
}
function _beforeTokenTransfer(address from, address to, uint256 amount)
internal
whenNotPaused
override
{
super._beforeTokenTransfer(from, to, amount);
}
}
- Deploy the Updated Contract: Recompile and deploy the updated contract using Truffle.
truffle compile
truffle migrate --network cronosTestnet
Interacting with Pausable Token Functions
- Create a Script to Pause and Unpause the Contract: Create a new file named
pause_contract.js
.
// pause_contract.js
const Web3 = require('web3');
const MyToken = require('./build/contracts/MyToken.json');
const HDWalletProvider = require('@truffle/hdwallet-provider');
const provider = new HDWalletProvider('YOUR_METAMASK_MNEMONIC', 'https://cronos-testnet.crypto.org:8545');
const web3 = new Web3(provider);
const contractAddress = 'YOUR_CONTRACT_ADDRESS';
const contract = new web3.eth.Contract(MyToken.abi, contractAddress);
async function pauseContract() {
const accounts = await web3.eth.getAccounts();
await contract.methods.pause().send({ from: accounts[0] });
console.log('Contract paused');
}
async function unpauseContract() {
const accounts = await web3.eth.getAccounts();
await contract.methods.unpause().send({ from: accounts[0] });
console.log('Contract unpaused');
}
// Call the desired function here
// pauseContract();
// unpauseContract();
- Run the Script: Execute the script to pause and unpause the contract.
node pause_contract.js
Adding Custom Token Features
Introducing Governance Tokens
Governance tokens allow token holders to participate in the decision-making process of a project. These tokens typically grant voting rights on proposals.
- Update the Token Contract: Extend the
MyToken.sol
contract to include governance functionality.
// contracts/MyToken.sol
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyToken is ERC20, Ownable {
mapping(address => uint256) private _votes;
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
function mint(address to, uint256 amount) public onlyOwner {
_mint(to, amount);
}
function burn(uint256 amount) public {
_burn(msg.sender, amount);
}
function delegateVotes(address delegatee, uint256 amount) public {
require(balanceOf(msg.sender) >= amount, "Insufficient balance to delegate");
_votes[delegatee] += amount;
}
function votesOf(address account) public view returns (uint256) {
return _votes[account];
}
}
- Deploy the Updated Contract: Recompile and deploy the updated contract using Truffle.
truffle compile
truffle migrate --network cronosTestnet
Interacting with Governance Functions
- Create a Script to Delegate Votes: Create a new file named
delegate_votes.js
.
// delegate_votes.js
const Web3 = require('web3');
const MyToken = require('./build/contracts/MyToken.json');
const HDWalletProvider = require('@truffle/hdwallet-provider');
const provider = new HDWalletProvider('YOUR_METAMASK_MNEMONIC', 'https://cronos-testnet.crypto.org:8545');
const web3 = new Web3(provider);
const contractAddress = 'YOUR_CONTRACT_ADDRESS';
const contract = new web3.eth.Contract(MyToken.abi, contractAddress);
async function delegateVotes() {
const accounts = await web3.eth.getAccounts();
const delegatee = 'DELEGATEE_ADDRESS';
const amount = web3.utils.toWei('10', 'ether');
await contract.methods.delegateVotes(delegatee, amount).send({ from: accounts[0] });
console.log(`Delegated 10 MTK votes to ${delegatee}`);
}
delegateVotes();
Replace DELEGATEE_ADDRESS
with the address of the delegatee.
- Run the Script: Execute the script to delegate votes.
node delegate_votes.js
Conclusion
Creating tokens on Cronos is a powerful way to leverage blockchain technology for various applications. By understanding the fundamentals and following the steps outlined in this guide, you can create, deploy, and manage your own tokens with advanced features like minting, burning, pausing, and governance.
Cronos offers a robust and scalable platform for token creation, making it an ideal choice for developers looking to innovate in the blockchain space. With lower transaction costs and high interoperability, Cronos stands out as a practical solution for both new and experienced blockchain developers.
Whether you’re building a new decentralized application or enhancing an existing one, the flexibility and capabilities of Cronos will empower you to achieve your project goals effectively. By mastering the secrets of token creation on Cronos, you open up a world of possibilities for decentralized finance, gaming, supply chains, and more.