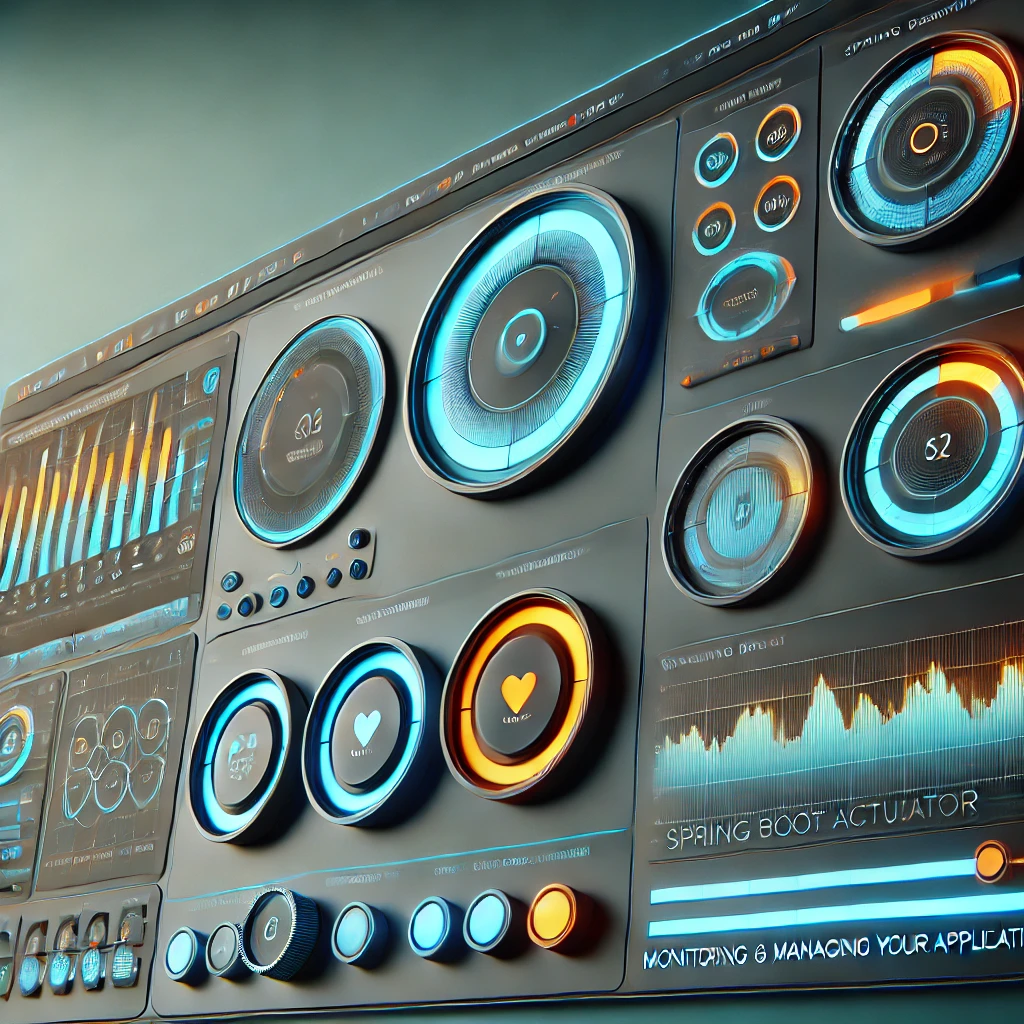
Monitoring and Managing Your Application using Spring Boot Actuator
In the ever-evolving landscape of software development, monitoring and managing applications have become crucial aspects of maintaining robust and efficient systems. As applications grow in complexity, the need for real-time insights into their health, performance, and operational status becomes paramount. This is where Spring Boot Actuator steps in, offering a powerful set of tools and features that empower developers and operations teams to gain valuable insights into their Spring Boot applications.
Spring Boot Actuator is an essential sub-project of the Spring Boot framework, designed to enhance the monitoring and management capabilities of your applications. It provides a wealth of production-ready features that can be easily integrated into your Spring Boot projects, allowing you to monitor, gather metrics, understand traffic patterns, and even interact with your application’s state during runtime.
In this comprehensive guide, we’ll delve deep into the world of Spring Boot Actuator, exploring its core concepts, key features, and practical applications. We’ll walk you through the process of setting up Actuator in your Spring Boot project, configuring its endpoints, and leveraging its capabilities to gain actionable insights into your application’s behavior. Whether you’re a seasoned Spring developer or just starting your journey with Spring Boot, this blog post will equip you with the knowledge and tools to effectively monitor and manage your applications using Spring Boot Actuator.
Understanding Spring Boot Actuator
Spring Boot Actuator is a powerful extension of the Spring Boot framework that brings production-ready features to your application. It provides a set of pre-built endpoints and tools that allow you to monitor and interact with your application in real-time. These endpoints expose operational information about your running application, such as health status, metrics, environment properties, and more.
Key Concepts of Spring Boot Actuator:
- Endpoints: Actuator endpoints are the core of its functionality. Each endpoint exposes specific information or allows certain actions to be performed on your application.
- Health Indicators: These are components that provide information about the health of your application and its dependencies.
- Metrics: Actuator collects various metrics about your application’s performance and resource usage.
- Auditing: It provides mechanisms to track and log important events within your application.
- HTTP Tracing: This feature allows you to trace HTTP requests and responses.
Spring Boot Actuator is designed with security in mind, allowing you to control access to sensitive information and operations. It’s highly configurable, enabling you to customize which endpoints are exposed and how they behave. This flexibility makes it suitable for a wide range of applications, from simple microservices to complex enterprise systems.
By leveraging Spring Boot Actuator, you can gain valuable insights into your application’s behavior, performance, and health, all without writing extensive custom code. This not only saves development time but also ensures that you’re using battle-tested, production-ready monitoring and management features.
Setting Up Spring Boot Actuator
Integrating Spring Boot Actuator into your project is a straightforward process. Let’s walk through the steps to set up Actuator in a Spring Boot application.
1. Add Actuator Dependency
To begin, you need to add the Actuator dependency to your project. If you’re using Maven, add the following to your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
For Gradle users, add this to your build.gradle
file:
implementation 'org.springframework.boot:spring-boot-starter-actuator'
2. Configure Actuator Endpoints
By default, most Actuator endpoints are disabled for security reasons. You can enable them in your application.properties
or application.yml
file. Here’s an example configuration that enables all endpoints:
management.endpoints.web.exposure.include=*
However, it’s generally recommended to only expose the endpoints you need. For example, to enable only the health and info endpoints:
management.endpoints.web.exposure.include=health,info
3. Customize Endpoint Paths
You can customize the base path for Actuator endpoints. By default, they’re accessible under /actuator
. To change this, add the following to your properties file:
management.endpoints.web.base-path=/management
4. Configure Security (Optional but Recommended)
If your application uses Spring Security, you’ll want to configure access control for Actuator endpoints. Here’s a basic example of how to secure Actuator endpoints using Spring Security:
@Configuration
public class ActuatorSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requestMatcher(EndpointRequest.toAnyEndpoint())
.authorizeRequests()
.anyRequest().hasRole("ACTUATOR")
.and()
.httpBasic();
}
}
This configuration requires users to have the “ACTUATOR” role to access any Actuator endpoint.
5. Verify Setup
Once you’ve completed these steps, start your Spring Boot application. You should be able to access Actuator endpoints at http://localhost:8080/actuator
(or your custom base path if you’ve changed it).
By following these steps, you’ll have Spring Boot Actuator set up and ready to use in your application. The next sections will dive deeper into specific features and how to leverage them effectively.
Exploring Actuator Endpoints
Spring Boot Actuator provides a wide range of endpoints that expose different aspects of your application. Let’s explore some of the most commonly used endpoints and their purposes.
1. Health Endpoint (/actuator/health)
The health endpoint provides basic information about your application’s health. It can be extended to include custom health indicators for various components of your system.
GET /actuator/health
Sample response:
{
"status": "UP",
"components": {
"diskSpace": {
"status": "UP",
"details": {
"total": 499963174912,
"free": 91300651008,
"threshold": 10485760
}
},
"db": {
"status": "UP",
"details": {
"database": "H2",
"validationQuery": "isValid()"
}
}
}
}
2. Info Endpoint (/actuator/info)
This endpoint displays arbitrary application information. You can customize this in your application.properties
file:
info.app.name=My Spring Application
info.app.description=A sample Spring Boot Actuator application
info.app.version=1.0.0
3. Metrics Endpoint (/actuator/metrics)
The metrics endpoint exposes various metrics collected by your application. You can access specific metrics by appending their names to the URL.
GET /actuator/metrics
GET /actuator/metrics/http.server.requests
4. Environment Endpoint (/actuator/env)
This endpoint exposes the current environment properties. It includes information about the system environment, application properties, and more.
5. Loggers Endpoint (/actuator/loggers)
The loggers endpoint allows you to view and modify the logging levels of your application at runtime.
6. Threaddump Endpoint (/actuator/threaddump)
This endpoint provides a thread dump of the application, which can be useful for diagnosing concurrency issues.
7. Heapdump Endpoint (/actuator/heapdump)
The heapdump endpoint generates a heap dump of the JVM, which can be analyzed to diagnose memory-related issues.
Here’s a table summarizing some key Actuator endpoints:
Endpoint | Description |
---|---|
/health | Application health information |
/info | Arbitrary application information |
/metrics | Application metrics |
/env | Environment properties |
/loggers | Application logging configuration |
/threaddump | Performs a thread dump |
/heapdump | Generates a heap dump |
/mappings | Displays all @RequestMapping paths |
/configprops | Displays @ConfigurationProperties |
To effectively use these endpoints, you can create custom dashboards or integrate with monitoring tools that can consume and visualize this data. In the next sections, we’ll explore how to customize and extend these endpoints to better suit your application’s needs.
Customizing Health Indicators
The health endpoint is one of the most crucial features of Spring Boot Actuator, providing a quick overview of your application’s status. While the default health check is useful, you often need to include custom health checks for various components of your system. Spring Boot makes it easy to create and include custom health indicators.
Creating a Custom Health Indicator
To create a custom health indicator, you need to implement the HealthIndicator
interface. Here’s an example of a custom health indicator that checks the status of an external API:
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthIndicator;
import org.springframework.stereotype.Component;
import org.springframework.web.client.RestTemplate;
@Component
public class ExternalApiHealthIndicator implements HealthIndicator {
private final RestTemplate restTemplate = new RestTemplate();
@Override
public Health health() {
try {
// Perform API call
restTemplate.getForObject("https://api.example.com/health", String.class);
return Health.up().withDetail("externalApi", "API is reachable").build();
} catch (Exception e) {
return Health.down().withDetail("externalApi", "API is unreachable").withException(e).build();
}
}
}
This health indicator attempts to make a request to an external API. If successful, it reports the health as UP; otherwise, it reports it as DOWN.
Aggregating Health Indicators
Spring Boot automatically aggregates all health indicators to provide an overall health status. The aggregation strategy can be configured in your application.properties
file:
management.health.status.order=DOWN,OUT_OF_SERVICE,UP,UNKNOWN
This configuration determines the order of precedence for health statuses. In this example, if any component is DOWN, the overall status will be DOWN.
Customizing Health Response
You can customize the information shown in the health endpoint response. For example, to show more details when the application is down:
management.endpoint.health.show-details=always
management.endpoint.health.show-components=always
With these settings, the health endpoint will always show detailed information about each component’s health.
Creating Composite Health Indicators
For complex systems, you might want to create composite health indicators that group related checks. Here’s an example:
import org.springframework.boot.actuate.health.CompositeHealthContributor;
import org.springframework.boot.actuate.health.HealthContributor;
import org.springframework.boot.actuate.health.NamedContributor;
import org.springframework.stereotype.Component;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
@Component
public class DatabaseHealthIndicator implements CompositeHealthContributor {
private Map<String, HealthContributor> contributors = new LinkedHashMap<>();
public DatabaseHealthIndicator(
PrimaryDatabaseHealthIndicator primaryDb,
SecondaryDatabaseHealthIndicator secondaryDb) {
contributors.put("primary", primaryDb);
contributors.put("secondary", secondaryDb);
}
@Override
public HealthContributor getContributor(String name) {
return contributors.get(name);
}
@Override
public Iterator<NamedContributor<HealthContributor>> iterator() {
return contributors.entrySet().stream()
.map((entry) -> NamedContributor.of(entry.getKey(), entry.getValue()))
.iterator();
}
}
This composite health indicator groups checks for primary and secondary databases. Each database would have its own health indicator implementing the HealthIndicator
interface.
By customizing health indicators, you can provide more meaningful and specific health information about your application and its dependencies. This can be invaluable for monitoring and quickly identifying issues in production environments.
Leveraging Metrics with Micrometer
Spring Boot Actuator uses Micrometer, a vendor-neutral application metrics facade, to capture and expose metrics. Micrometer provides a simple facade over the instrumentation clients for the most popular monitoring systems, allowing you to instrument your JVM-based application code without vendor lock-in.
Understanding Micrometer Concepts
- Meters: The interface for collecting a set of measurements about your application.
- Tags: Key-value pairs that add dimensions to metrics, allowing for drill-down and aggregation.
- Registries: Where metrics are stored. Spring Boot automatically configures a
SimpleMeterRegistry
by default.
Accessing Metrics
You can access metrics through the /actuator/metrics
endpoint. To view specific metrics, append the metric name to the URL:
GET /actuator/metrics/jvm.memory.used
Creating Custom Metrics
Micrometer makes it easy to create custom metrics. Here are examples of different types of meters:
- Counter: Measures a value that can only increase.
import io.micrometer.core.instrument.Counter;
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.stereotype.Component;
@Component
public class OrderService {
private final Counter orderCounter;
public OrderService(MeterRegistry registry) {
this.orderCounter = Counter.builder("orders.created")
.description("Total number of orders created")
.register(registry);
}
public void createOrder() {
// Order creation logic
orderCounter.increment();
}
}
- Gauge: Measures a value that can increase or decrease.
import io.micrometer.core.instrument.Gauge;
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.stereotype.Component;
@Component
public class QueueManager {
private final Queue<String> queue = new ConcurrentLinkedQueue<>();
public QueueManager(MeterRegistry registry) {
Gauge.builder("queue.size", queue::size)
.description("Number of items in the queue")
.register(registry);
}
// Queue operations
}
- Timer: Measures the time taken for short tasks and the count of these tasks.
import io.micrometer.core.instrument.Timer;
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.stereotype.Component;
@Component
public class DatabaseQueryService {
private final Timer queryTimer;
public DatabaseQueryService(MeterRegistry registry) {
this.queryTimer = Timer.builder("database.query.time")
.description("Time taken for database queries")
.register(registry);
}
public Result performQuery(String query) {
return queryTimer.record(() -> {
// Actual database query logic
return actualQueryMethod(query);
});
}
}
Customizing Metrics
You can customize various aspects of metrics collection and reporting:
- Changing the Metrics Endpoint Base Path:
management.endpoints.web.base-path=/manage
management.endpoints.web.path-mapping.metrics=statistics
This would make metrics available at /manage/statistics
.
- Filtering Metrics:
management.metrics.enable.jvm=false
This disables all JVM metrics.
- Configuring Percentiles:
management.metrics.distribution.percentiles-histogram.http.server.requests=true
management.metrics.distribution.sla.http.server.requests=1ms,5ms
This configures histogram buckets for HTTP request durations and defines SLA boundaries.
Integrating with Monitoring Systems
Spring Boot Actuator and Micrometer make it easy to integrate with various monitoring systems. For example, to use Prometheus:
- Add the Prometheus dependency to your
pom.xml
:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
- Enable the Prometheus endpoint in your
application.properties
:
management.endpoints.web.exposure.include=prometheus
- Prometheus can now scrape metrics from your application at the
/actuator/prometheus
endpoint.
Similar integrations exist for other popular monitoring systems like Graphite, InfluxDB, and New Relic.
Customizing Actuator Endpoints
While Spring Boot Actuator provides a wealth of built-in endpoints, you may sometimes need to customize existing endpoints or create entirely new ones to suit your specific needs.
Customizing Existing Endpoints
You can customize existing endpoints by creating a bean that extends the endpoint you want to modify. For example, to add additional information to the /info
endpoint:
import org.springframework.boot.actuate.info.Info;
import org.springframework.boot.actuate.info.InfoContributor;
import org.springframework.stereotype.Component;
@Component
public class CustomInfoContributor implements InfoContributor {
@Override
public void contribute(Info.Builder builder) {
builder.withDetail("custom",
Map.of(
"key1", "value1",
"key2", "value2"
)
);
}
}
This will add a “custom” section to the /info
endpoint’s response.
Creating Custom Endpoints
You can create entirely new endpoints using the @Endpoint
annotation. Here’s an example of a custom endpoint that provides system time:
import org.springframework.boot.actuate.endpoint.annotation.*;
import org.springframework.stereotype.Component;
@Component
@Endpoint(id = "systemtime")
public class SystemTimeEndpoint {
@ReadOperation
public String getSystemTime() {
return new Date().toString();
}
@WriteOperation
public void setSystemTime(@Selector String newTime) {
// Logic to set system time (if permitted)
}
}
This creates a new endpoint at /actuator/systemtime
that supports both GET (read) and POST (write) operations.
Securing Custom Endpoints
Custom endpoints are secured in the same way as built-in endpoints. You can use Spring Security to control access:
@Configuration
public class ActuatorSecurity extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requestMatcher(EndpointRequest.toAnyEndpoint())
.authorizeRequests()
.anyRequest().hasRole("ACTUATOR")
.and()
.httpBasic();
}
}
This configuration requires users to have the “ACTUATOR” role to access any Actuator endpoint, including custom ones.
Best Practices for Using Spring Boot Actuator
When implementing Spring Boot Actuator in your applications, consider the following best practices:
- Security First: Always secure your Actuator endpoints, especially in production environments. Use Spring Security to control access and consider exposing only necessary endpoints.
- Customize Health Checks: Implement custom health indicators for critical components of your system to get a comprehensive view of your application’s health.
- Use Meaningful Metrics: Focus on collecting metrics that provide actionable insights. Avoid over-instrumenting your code, which can lead to performance overhead.
- Implement Proper Logging: Use the logging endpoint to dynamically adjust log levels. This can be invaluable for debugging issues in production without restarting the application.
- Monitor Endpoint Usage: Keep track of which endpoints are being accessed and how often. This can help you optimize your monitoring strategy.
- Use Tags Effectively: When creating custom metrics, use tags to add dimensions to your data. This allows for more detailed analysis and easier troubleshooting.
- Integrate with Existing Tools: Leverage Actuator’s compatibility with various monitoring systems to integrate with your existing infrastructure.
- Regular Reviews: Periodically review your Actuator configuration and collected metrics to ensure they’re still relevant and providing value.
Conclusion
Spring Boot Actuator is a powerful tool that significantly enhances the monitoring and management capabilities of Spring Boot applications. By providing a wealth of production-ready features out of the box, it allows developers to gain deep insights into their applications’ behavior, performance, and health.
From basic health checks to detailed metrics collection, from environment information to custom endpoints, Spring Boot Actuator offers a comprehensive suite of tools that can be tailored to meet the specific needs of your application. When used effectively, it can greatly improve your ability to monitor, manage, and troubleshoot your Spring Boot applications in production environments.
As with any powerful tool, it’s important to use Spring Boot Actuator judiciously. Always prioritize security, focus on meaningful metrics, and integrate with your existing monitoring infrastructure. By following the best practices outlined in this guide, you can leverage the full potential of Spring Boot Actuator to build more robust, manageable, and observable Spring Boot applications.
Remember, effective application monitoring and management is an ongoing process. Regularly review and refine your Actuator configuration to ensure it continues to meet your evolving needs. With Spring Boot Actuator, you’re well-equipped to tackle the challenges of modern application management and monitoring.
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, technologies and best practices may change over time. Always refer to the official Spring Boot documentation for the most up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.
Additional Endpoints
health: /actuator/health
info: /actuator/info
beans: /actuator/beans
conditions: /actuator/conditions
configprops: /actuator/configprops
env: /actuator/env
metrics: /actuator/metrics
mappings: /actuator/mappings
scheduledtasks: /actuator/scheduledtasks
threaddump: /actuator/threaddump
heapdump: /actuator/heapdump
loggers: /actuator/loggers
httptrace: /actuator/httptrace