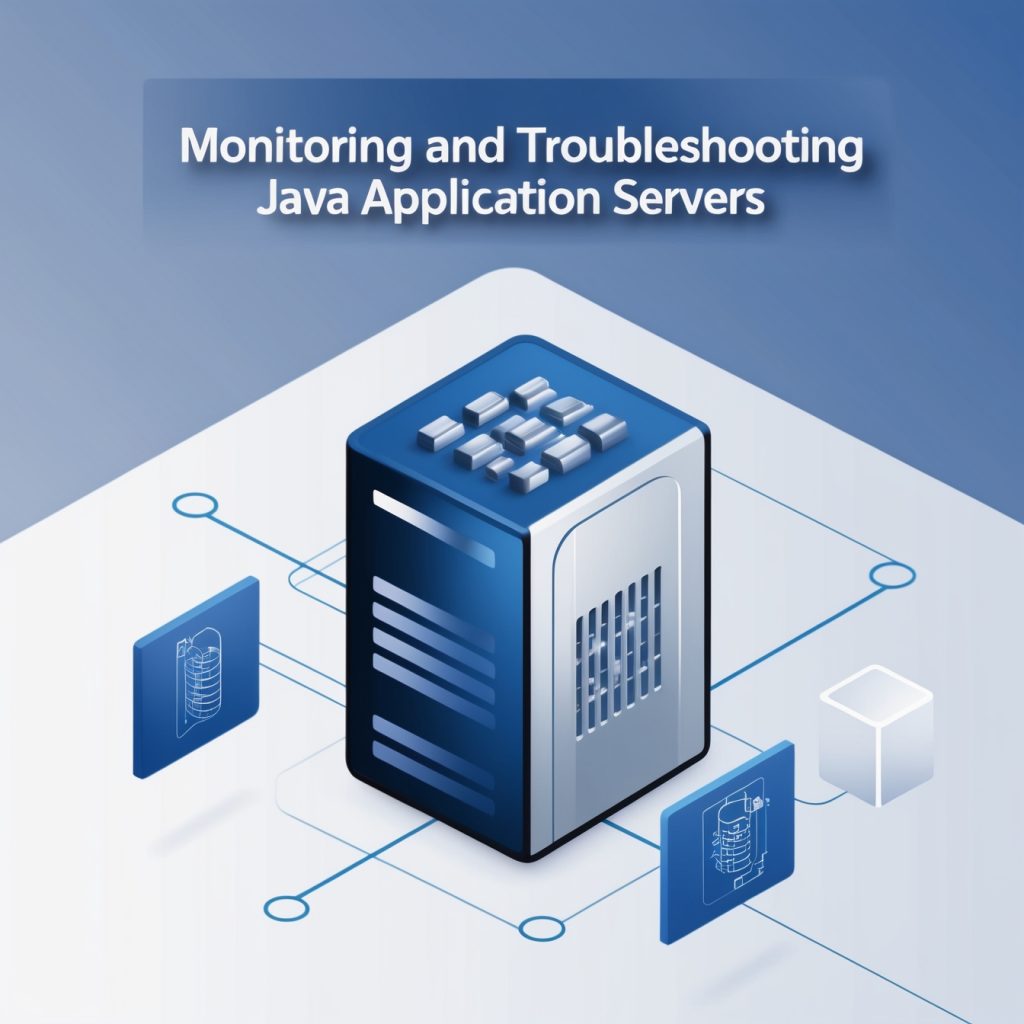
Monitoring and Troubleshooting Java Application Servers
Are you a Java developer or system administrator responsible for keeping your Java applications running smoothly? If so, you’ve probably encountered your fair share of performance issues, memory leaks, and mysterious bugs. Don’t worry – you’re not alone! In this comprehensive guide, we’ll dive deep into the world of monitoring and troubleshooting Java application servers. By the time you finish reading, you’ll be equipped with the knowledge and tools to tackle even the trickiest server problems. So, grab your favorite debugging tool, and let’s get started!
Understanding Java Application Servers
Before we jump into the nitty-gritty of monitoring and troubleshooting, let’s take a moment to understand what Java application servers are and why they’re so important. Java application servers are the backbone of many enterprise applications, providing a runtime environment for Java-based web applications and services. They handle everything from request processing and session management to database connectivity and security. Some popular Java application servers include Apache Tomcat, WildFly (formerly JBoss), IBM WebSphere, and Oracle WebLogic.
These servers are designed to be robust and scalable, but like any complex system, they can sometimes run into issues. That’s where monitoring and troubleshooting come into play. By keeping a close eye on your application server’s performance and knowing how to diagnose problems when they arise, you can ensure your applications run smoothly and your users stay happy.
The Importance of Proactive Monitoring
You know what they say – an ounce of prevention is worth a pound of cure. This couldn’t be truer when it comes to managing Java application servers. Proactive monitoring is all about catching potential issues before they turn into full-blown problems that affect your users. By setting up a comprehensive monitoring strategy, you can:
- Identify performance bottlenecks early on
- Detect and address memory leaks before they cause outages
- Spot unusual patterns in resource usage that might indicate security issues
- Ensure your application is meeting its service level agreements (SLAs)
- Make data-driven decisions about scaling and optimization
Implementing a proactive monitoring approach doesn’t just save you headaches – it can also save your organization time and money. After all, it’s much easier (and cheaper) to fix a small issue than to deal with a major outage or performance crisis.
Essential Metrics to Monitor
Now that we understand the importance of monitoring, let’s talk about what exactly we should be keeping an eye on. Here are some key metrics you should consider monitoring for your Java application server:
1. CPU Usage
Keep track of how much CPU your server is using. High CPU usage can indicate performance bottlenecks or runaway processes.
2. Memory Utilization
Monitor both heap and non-heap memory usage. Memory leaks can cause your server to slow down or crash if left unchecked.
3. Thread Count and States
Watch the number of active threads and their states. Too many blocked or waiting threads could signal concurrency issues.
4. Database Connection Pool
Monitor the number of active and idle connections in your database connection pool. This can help you optimize resource usage and spot connection leaks.
5. Request Processing Time
Track how long it takes to process incoming requests. Slow response times can negatively impact user experience.
6. Garbage Collection Activity
Keep an eye on the frequency and duration of garbage collection events. Excessive GC activity can lead to performance issues.
7. Custom Application Metrics
Don’t forget to monitor metrics specific to your application, such as the number of active users, transaction rates, or error rates.
Let’s summarize these metrics in a table for easy reference:
Metric | Description | Why It’s Important |
---|---|---|
CPU Usage | Percentage of CPU resources used | Identifies performance bottlenecks |
Memory Utilization | Heap and non-heap memory consumption | Detects memory leaks and resource issues |
Thread Count and States | Number and status of active threads | Spots concurrency and deadlock problems |
Database Connection Pool | Active and idle database connections | Optimizes resource usage and identifies connection leaks |
Request Processing Time | Time taken to process incoming requests | Ensures good user experience |
Garbage Collection Activity | Frequency and duration of GC events | Prevents performance degradation due to excessive GC |
Custom Application Metrics | Application-specific measurements | Provides insights into application behavior and usage |
Tools of the Trade: Monitoring Solutions
Now that we know what to monitor, let’s explore some tools that can help us do the job. There’s no shortage of monitoring solutions out there, ranging from open-source tools to enterprise-grade platforms. Here are a few popular options:
1. Java Management Extensions (JMX)
JMX is built into the Java platform and provides a standard way to monitor and manage Java applications. It’s a great starting point for basic monitoring.
2. Java VisualVM
This is a visual tool that comes bundled with the JDK. It provides a user-friendly interface for monitoring and profiling Java applications.
3. Apache Tomcat Manager
If you’re using Tomcat, its built-in Manager application offers basic monitoring and management capabilities.
4. Prometheus and Grafana
This powerful open-source combo allows you to collect and visualize metrics from your Java application server.
5. New Relic
A comprehensive application performance monitoring (APM) solution that provides deep insights into your Java applications and servers.
6. Datadog
Another popular APM platform that offers extensive monitoring capabilities for Java and many other technologies.
7. Dynatrace
An AI-powered observability platform that provides advanced monitoring and automatic problem detection for Java applications.
Remember, the best tool for you will depend on your specific needs, budget, and the complexity of your environment. It’s often a good idea to start with the built-in tools and gradually add more sophisticated solutions as your monitoring needs grow.
Setting Up Basic Monitoring with JMX
Let’s get our hands dirty and set up some basic monitoring using JMX. This will give you a taste of how monitoring works in practice. First, we need to enable JMX remote management on our Java application server. Here’s how you can do it by adding some JVM arguments:
java -Dcom.sun.management.jmxremote \
-Dcom.sun.management.jmxremote.port=9010 \
-Dcom.sun.management.jmxremote.local.only=false \
-Dcom.sun.management.jmxremote.authenticate=false \
-Dcom.sun.management.jmxremote.ssl=false \
-jar your-application.jar
Now that we’ve enabled JMX, we can use a tool like JConsole (which comes with the JDK) to connect to our application and view its metrics. Simply run jconsole
from the command line and connect to your application using the port you specified (9010 in this case).
Once connected, you’ll be able to see various metrics such as heap memory usage, thread count, and CPU usage. You can even invoke operations on your application’s MBeans if you’ve exposed any custom management interfaces.
Creating Custom Monitors
While built-in metrics are great, sometimes you need to monitor aspects of your application that are specific to your business logic. Let’s create a simple custom monitor using JMX. We’ll create a monitor that tracks the number of active users in our application.
First, we’ll define an interface for our monitor:
public interface UserMonitorMBean {
int getActiveUsers();
void setActiveUsers(int count);
}
Then, we’ll implement this interface:
public class UserMonitor implements UserMonitorMBean {
private int activeUsers = 0;
@Override
public int getActiveUsers() {
return activeUsers;
}
@Override
public void setActiveUsers(int count) {
this.activeUsers = count;
}
}
Finally, we’ll register this MBean with the JMX server:
import javax.management.*;
import java.lang.management.ManagementFactory;
public class Main {
public static void main(String[] args) throws Exception {
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("com.example:type=UserMonitor");
UserMonitor mbean = new UserMonitor();
mbs.registerMBean(mbean, name);
// Your application logic here
// Remember to update the activeUsers count as users log in and out
}
}
Now, when you connect to your application with JConsole, you’ll see your custom UserMonitor MBean, and you can monitor the number of active users in real-time!
Troubleshooting Common Java Application Server Issues
Even with the best monitoring setup, issues can still crop up. Let’s look at some common problems you might encounter with Java application servers and how to troubleshoot them.
1. High CPU Usage
If you’re seeing consistently high CPU usage, it could be due to inefficient code, infinite loops, or too many concurrent requests. Here’s how to investigate:
- Use a profiler like Java VisualVM to identify which threads are consuming the most CPU.
- Look for methods that are being called frequently or taking a long time to execute.
- Check for any infinite loops or recursion in your code.
- Consider optimizing your algorithms or adding caching to reduce CPU load.
2. Memory Leaks
Memory leaks can cause your application to slow down over time and eventually crash. Here’s how to detect and fix them:
- Monitor your heap usage over time. If it’s continuously growing without leveling off, you might have a leak.
- Use a memory profiler to take heap dumps at different points in time.
- Compare the heap dumps to see which objects are accumulating.
- Look for objects that should have been garbage collected but weren’t. Common culprits include forgotten event listeners and caches that grow without bounds.
Here’s a simple example of how a memory leak might occur:
public class LeakyClass {
private static final List<byte[]> leakyList = new ArrayList<>();
public void addToList() {
byte[] leakyArray = new byte[1024 * 1024]; // 1MB
leakyList.add(leakyArray);
}
}
In this example, leakyList
is static, so it will never be garbage collected. If addToList()
is called repeatedly, it will keep adding 1MB arrays to the list, eventually causing an OutOfMemoryError.
3. Database Connection Leaks
Database connection leaks can exhaust your connection pool and cause timeouts. Here’s how to address them:
- Monitor the number of active connections in your pool.
- Use a connection pool that can detect and close idle connections.
- Always close your database connections in a finally block or use try-with-resources.
Here’s an example of proper connection handling:
try (Connection conn = dataSource.getConnection();
PreparedStatement ps = conn.prepareStatement("SELECT * FROM users")) {
ResultSet rs = ps.executeQuery();
while (rs.next()) {
// Process results
}
} catch (SQLException e) {
// Handle exception
}
4. Slow Response Times
If your application is responding slowly, there could be several causes:
- Use a profiler to identify which methods are taking the most time.
- Check for any blocking operations, especially in request processing threads.
- Look for N+1 query problems in your database access code.
- Consider adding caching for frequently accessed data.
- Optimize your database queries and indexes.
5. OutOfMemoryError
This dreaded error can bring your application to its knees. Here’s how to deal with it:
- Increase your heap size if you’re genuinely running out of memory.
- Use
-XX:+HeapDumpOnOutOfMemoryError
to generate a heap dump when the error occurs. - Analyze the heap dump to identify which objects are consuming the most memory.
- Look for memory leaks or inefficient data structures in your code.
Advanced Troubleshooting Techniques
Sometimes, the usual troubleshooting methods aren’t enough. That’s when you need to break out the big guns. Here are some advanced techniques you can use:
1. Thread Dumps
Thread dumps can provide valuable insights into what your application is doing at a specific moment. You can generate a thread dump by sending a SIGQUIT signal to your Java process:
kill -3 <pid>
Or, if you’re on Windows, you can use jcmd:
jcmd <pid> Thread.print
Analyze the thread dump to look for blocked threads, deadlocks, or threads stuck in unexpected states.
2. Heap Dumps
Heap dumps capture the state of the heap at a specific point in time. You can generate a heap dump using jmap:
jmap -dump:format=b,file=heap.bin <pid>
Then, use a tool like Eclipse Memory Analyzer (MAT) to analyze the heap dump and identify memory leaks or inefficient memory usage.
3. Flight Recorder
Java Flight Recorder (JFR) is a powerful tool for capturing detailed runtime information about your Java application. Here’s how to start a recording:
jcmd <pid> JFR.start duration=60s filename=myrecording.jfr
After the recording completes, you can analyze it using Java Mission Control or other JFR-compatible tools.
4. Debugging Remote Applications
Sometimes you need to debug an application running in a production-like environment. You can enable remote debugging by adding these JVM arguments:
-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005
Then, connect to the remote JVM from your IDE using the specified port (5005 in this case).
Best Practices for Java Application Server Management
To wrap up our deep dive into monitoring and troubleshooting, let’s look at some best practices that can help you keep your Java application servers running smoothly:
- Implement Comprehensive Logging: Use a logging framework like SLF4J with Logback or Log4j2 to implement structured logging. This will make it easier to search and analyze your logs when troubleshooting issues.
- Set Up Alerting: Configure alerts for critical metrics so you can be notified of potential issues before they impact your users.
- Perform Regular Health Checks: Implement health check endpoints in your application and use them to monitor the overall health of your system.
- Practice Capacity Planning: Regularly review your resource usage trends and plan for future growth to avoid sudden resource shortages.
- Keep Your Server and Dependencies Updated: Regularly update your application server and dependencies to benefit from performance improvements and security patches.
- Use Containerization: Consider using containerization technologies like Docker to ensure consistency across different environments and make it easier to replicate issues.
- Implement Circuit Breakers: Use libraries like Hystrix or Resilience4j to implement circuit breakers, preventing cascading failures in distributed systems.
- Conduct Regular Performance Tests: Periodically run performance tests to catch potential issues before they make it to production.
- Document Your Troubleshooting Processes: Keep a knowledge base of common issues and their solutions to speed up future troubleshooting efforts.
- Foster a Culture of Observability: Encourage your development team to think about monitoring and troubleshooting from the start of the development process.
Conclusion
Monitoring and troubleshooting Java application servers is both an art and a science. It requires a deep understanding of how Java applications work, familiarity with various tools and techniques, and the ability to think critically and solve problems under pressure. By following the practices and techniques we’ve discussed in this guide, you’ll be well-equipped to tackle even the most challenging server issues.
Remember, the key to effective monitoring and troubleshooting is persistence and continuous learning. Technology is always evolving, and new tools and techniques are constantly emerging. Stay curious, keep experimenting, and don’t be afraid to dive deep when issues arise. With practice and experience, you’ll become a true master of Java application server management.
Happy monitoring, and may your servers always run smoothly!
Disclaimer: This blog post is intended for educational purposes only. While we strive to provide accurate and up-to-date information, technologies and best practices in the field of Java application server management are constantly evolving so If you notice any inaccuracies in this post, please report them so we can correct them promptly.