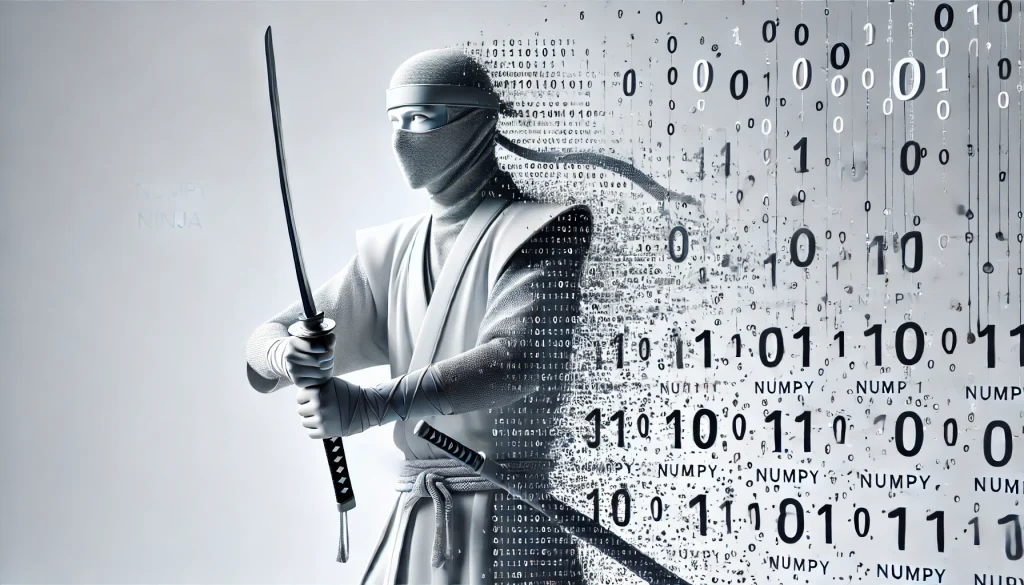
NumPy Ninja: Numerical Operations for AI Made Easy
Hey there, budding data scientists and AI enthusiasts! Are you ready to dive into the world of numerical operations that power Artificial Intelligence? Let’s embark on a journey to explore NumPy, the ninja of numerical operations in Python. By the end of this blog, you’ll see how NumPy makes your AI projects a breeze. Grab your favorite beverage, sit back, and let’s get started!
What is NumPy?
If you’re venturing into data science or AI, you must have stumbled upon the term NumPy. But what exactly is it? NumPy, short for Numerical Python, is a fundamental package for scientific computing in Python. It provides support for arrays, matrices, and a plethora of mathematical functions to operate on these data structures. Imagine handling large datasets and performing complex calculations with ease— that’s what NumPy offers.
Why is NumPy Essential?
- Efficiency: NumPy arrays are more efficient than Python lists.
- Convenience: A variety of functions for easy manipulation of numerical data.
- Foundation: Forms the base for other scientific libraries like Pandas, SciPy, and more.
Let’s dive deeper into how NumPy makes numerical operations easy and efficient.
Installing NumPy
First things first, let’s get NumPy installed. Open your terminal and type:
pip install numpy
This will download and install NumPy on your system. Once installed, you’re ready to unleash the power of numerical computing!
Creating and Manipulating Arrays
Creating Arrays
NumPy provides several ways to create arrays. Here’s how you can create a simple array:
import numpy as np
# Creating a 1D array
arr = np.array([1, 2, 3, 4, 5])
print("1D Array:", arr)
# Creating a 2D array
arr_2d = np.array([[1, 2, 3], [4, 5, 6]])
print("2D Array:\n", arr_2d)
Array Operations
One of the reasons NumPy is powerful is because of its array operations. Let’s look at some examples:
# Element-wise operations
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
# Addition
sum_arr = arr1 + arr2
print("Sum:", sum_arr)
# Multiplication
product_arr = arr1 * arr2
print("Product:", product_arr)
# Broadcasting
broadcast_sum = arr1 + 3
print("Broadcast Sum:", broadcast_sum)
Indexing and Slicing
Indexing
Indexing in NumPy is intuitive and straightforward. Here’s how you can access elements:
arr = np.array([1, 2, 3, 4, 5])
# Accessing the first element
print("First Element:", arr[0])
# Accessing the last element
print("Last Element:", arr[-1])
Slicing
Slicing allows you to access a range of elements in an array. Let’s see how it’s done:
# Slicing the array
print("Elements from index 1 to 3:", arr[1:4])
# Slicing with step
print("Elements from index 0 to 4 with step 2:", arr[0:5:2])
Reshaping Arrays
Reshaping
Reshaping is a powerful feature that allows you to change the shape of an array without changing its data. Here’s an example:
arr = np.array([1, 2, 3, 4, 5, 6])
# Reshaping to 2x3 array
reshaped_arr = arr.reshape(2, 3)
print("Reshaped Array:\n", reshaped_arr)
Mathematical Functions
NumPy comes with a wide range of mathematical functions. Here are some of the most commonly used ones:
Basic Functions
arr = np.array([1, 2, 3, 4, 5])
# Square root
sqrt_arr = np.sqrt(arr)
print("Square Root:", sqrt_arr)
# Exponential
exp_arr = np.exp(arr)
print("Exponential:", exp_arr)
Trigonometric Functions
# Sine function
sin_arr = np.sin(np.pi/2)
print("Sine of 90 degrees:", sin_arr)
# Cosine function
cos_arr = np.cos(0)
print("Cosine of 0 degrees:", cos_arr)
Linear Algebra with NumPy
NumPy also provides functionalities for linear algebra operations, which are crucial for AI and machine learning.
Dot Product
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
# Dot product
dot_product = np.dot(a, b)
print("Dot Product:\n", dot_product)
Determinant and Inverse
# Determinant
det = np.linalg.det(a)
print("Determinant:", det)
# Inverse
inverse = np.linalg.inv(a)
print("Inverse:\n", inverse)
Working with Large Datasets
Handling Large Datasets
NumPy is optimized to handle large datasets efficiently. Let’s simulate working with a large dataset:
# Creating a large array
large_arr = np.random.rand(1000, 1000)
# Performing a sum operation
large_sum = np.sum(large_arr)
print("Sum of Large Array:", large_sum)
Random Number Generation
Random Number Generation
NumPy also provides a module for generating random numbers, which is useful in simulations and AI models.
# Generating random numbers
rand_arr = np.random.rand(5)
print("Random Array:", rand_arr)
# Generating random integers
rand_int_arr = np.random.randint(1, 10, size=5)
print("Random Integer Array:", rand_int_arr)
Fourier Transform
Fourier Transform
Fourier Transform is used in signal processing and NumPy makes it easy to perform.
# Creating a sample signal
signal = np.sin(np.linspace(0, 2*np.pi, 100))
# Performing Fourier Transform
fft_signal = np.fft.fft(signal)
print("Fourier Transform:\n", fft_signal)
Real-World Application: Image Processing
Image Processing with NumPy
NumPy can be used for basic image processing tasks, such as reading, manipulating, and displaying images.
import matplotlib.pyplot as plt
import numpy as np
from PIL import Image
# Reading an image
img = Image.open('sample_image.jpg')
img_arr = np.array(img)
# Displaying the image
plt.imshow(img_arr)
plt.title("Original Image")
plt.show()
# Manipulating the image (inverting colors)
inverted_img_arr = 255 - img_arr
# Displaying the manipulated image
plt.imshow(inverted_img_arr)
plt.title("Inverted Image")
plt.show()
Integrating NumPy with Other Libraries
NumPy works seamlessly with other Python libraries, making it an integral part of the data science ecosystem.
Integration with Pandas
Pandas, a powerful data manipulation library, relies heavily on NumPy.
import pandas as pd
# Creating a DataFrame
data = {
'A': np.random.rand(5),
'B': np.random.rand(5),
'C': np.random.rand(5)
}
df = pd.DataFrame(data)
print("DataFrame:\n", df)
# Performing operations
mean_values = df.mean()
print("Mean Values:\n", mean_values)
Integration with SciPy
SciPy builds on NumPy to provide a large number of higher-level scientific algorithms.
from scipy import stats
# Generating data
data = np.random.normal(0, 1, 1000)
# Performing statistical analysis
mean = np.mean(data)
std_dev = np.std(data)
skewness = stats.skew(data)
kurtosis = stats.kurtosis(data)
print("Mean:", mean)
print("Standard Deviation:", std_dev)
print("Skewness:", skewness)
print("Kurtosis:", kurtosis)
Conclusion
We’ve covered a lot of ground in this blog, from basic array operations to advanced linear algebra and real-world applications. NumPy truly makes numerical operations for AI easy and efficient. Whether you’re handling large datasets, performing complex mathematical calculations, or integrating with other libraries, NumPy is your go-to tool. Happy coding, NumPy Ninja!
Disclaimer: The examples and code snippets provided in this blog are for educational purposes only. Please report any inaccuracies so we can correct them promptly.