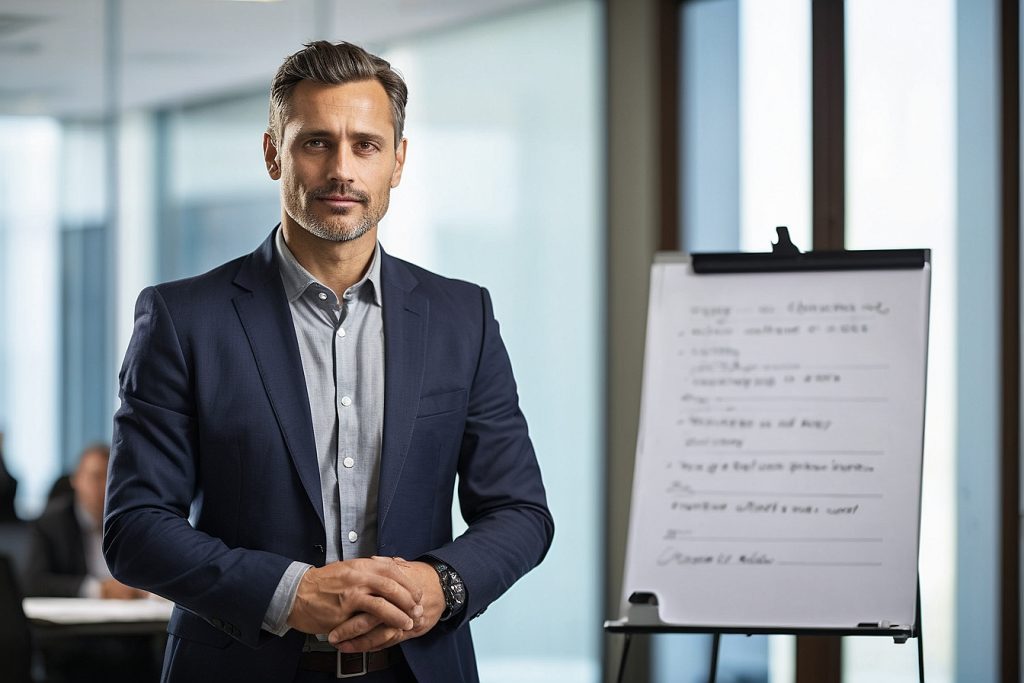
Object Oriented Programming in Python
Object-Oriented Programming (OOP) is a programming paradigm that revolves around the concept of objects, which are instances of classes. In Python, everything is an object (sort of), and OOP provides a way to structure your code by creating reusable and modular components. In this blog post, we’ll explore the core features of OOP in Python and how to leverage them effectively.
- Classes and Objects
A class is a blueprint or template for creating objects. It defines the properties (attributes) and behaviors (methods) that the objects will have. Here’s an example:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} says: Woof!")
# Creating objects
dog1 = Dog("Buddy", "Labrador")
dog2 = Dog("Max", "Poodle")
# Accessing attributes and calling methods
print(dog1.name) # Output: Buddy
print(dog2.breed) # Output: Poodle
dog1.bark() # Output: Buddy says: Woof!
- Inheritance
Inheritance is a mechanism that allows a new class to be based on an existing class, inheriting its attributes and methods. This promotes code reuse and allows for the creation of hierarchical relationships between classes. Here’s an example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print(f"{self.name} makes a sound.")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name)
self.breed = breed
def bark(self):
print(f"{self.name} says: Woof!")
dog = Dog("Buddy", "Labrador")
dog.speak() # Output: Buddy makes a sound.
dog.bark() # Output: Buddy says: Woof!
- Encapsulation
Encapsulation is the concept of bundling data and methods within a single unit (class) and controlling access to them through access modifiers. In Python, there are no strict access modifiers, but we can use naming conventions to indicate whether an attribute or method is meant to be public, protected, or private. Here’s an example:
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self._balance = balance # Protected attribute
def deposit(self, amount):
self._balance += amount
print(f"Deposited {amount}, new balance: {self._balance}")
def withdraw(self, amount):
if amount > self._balance:
print("Insufficient funds")
else:
self._balance -= amount
print(f"Withdrew {amount}, new balance: {self._balance}")
account = BankAccount("123456789", 1000)
account.deposit(500) # Output: Deposited 500, new balance: 1500
account.withdraw(2000) # Output: Insufficient funds
- Polymorphism
Polymorphism is the ability of objects to take on many forms. In Python, this is achieved through method overriding and operator overloading. Method overriding occurs when a subclass provides its own implementation of a method that is already defined in its parent class. Operator overloading allows you to define how operators (+, -, *, /, etc.) work with objects of your class. Here’s an example:
class Shape:
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
import math
return math.pi * self.radius ** 2
shapes = [Rectangle(3, 4), Circle(5)]
for shape in shapes:
print(shape.area()) # Output: 12, 78.53981633974483
In this example, the area
method is overridden in the Rectangle
and Circle
classes, allowing for polymorphic behavior based on the specific shape object.
- Abstraction
Abstraction is the process of hiding complex implementation details and exposing only the essential features to the user. In Python, this can be achieved through abstract classes and interfaces (using the abc
module). Here’s an example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
@abstractmethod
def perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def perimeter(self):
return 2 * (self.width + self.height)
rectangle = Rectangle(3, 4)
print(rectangle.area()) # Output: 12
print(rectangle.perimeter()) # Output: 14
In this example, the Shape
class is an abstract base class that defines the required methods (area
and perimeter
) that any concrete shape class must implement.
These are just a few examples of the core features of OOP in Python. By leveraging these concepts, you can create modular, reusable, and maintainable code that adheres to the principles of object-oriented programming.