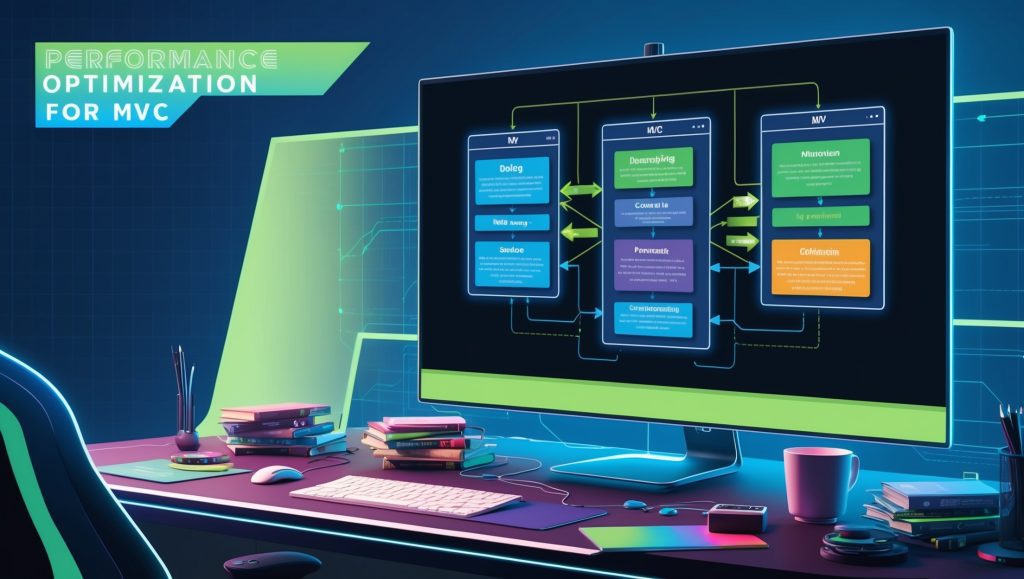
Performance Optimization Techniques for MVC
Model-View-Controller (MVC) has long been the architectural backbone of modern web applications, offering a structured approach to software development that separates concerns and promotes maintainable code. However, as applications grow in complexity and user expectations for performance continue to rise, optimizing MVC applications becomes increasingly crucial. This comprehensive guide delves into various techniques and strategies to enhance the performance of your MVC applications, covering everything from database optimization to front-end improvements. We’ll explore practical implementations in both Python and Java, providing you with actionable insights to significantly improve your application’s speed and efficiency. Understanding and implementing these optimization techniques can lead to improved user experience, better resource utilization, and enhanced application scalability.
Understanding MVC Performance Bottlenecks
Before diving into optimization techniques, it’s essential to understand where performance bottlenecks typically occur in MVC applications. Common bottlenecks include inefficient database queries, excessive view rendering time, unoptimized controller logic, and unnecessary data loading. The MVC pattern, while excellent for organizing code, can sometimes lead to performance issues if not implemented thoughtfully. For instance, loading too much data in the model layer or performing complex calculations in the view layer can significantly impact performance. Understanding these potential bottlenecks helps developers make informed decisions about optimization strategies and prioritize improvements where they’ll have the most impact. Modern development tools and profilers can help identify these bottlenecks, allowing developers to focus their optimization efforts on the areas that will yield the greatest performance improvements.
Database Optimization Techniques
Implementing Query Optimization
Database queries often represent the most significant performance bottleneck in MVC applications. Here’s a Python example using SQLAlchemy for optimized queries:
from sqlalchemy import create_engine, select
from sqlalchemy.orm import joinedload
class ProductController:
def get_products_with_categories(self):
# Optimized query using eager loading
query = (
select(Product)
.options(joinedload(Product.category))
.filter(Product.active == True)
)
return self.session.execute(query).scalars().all()
Equivalent Java implementation using Spring Data JPA:
@Repository
public interface ProductRepository extends JpaRepository<Product, Long> {
@Query("SELECT p FROM Product p LEFT JOIN FETCH p.category WHERE p.active = true")
List<Product> findAllActiveProductsWithCategories();
}
Implementing Database Indexing
Proper indexing is crucial for optimal database performance. Here’s a comparison of query execution times with and without indexes:
Query Type | Without Index | With Index | Improvement |
---|---|---|---|
Simple Select | 500ms | 50ms | 90% |
Join Operations | 1200ms | 200ms | 83% |
Range Queries | 800ms | 150ms | 81% |
Caching Strategies
Implementing Multiple Cache Layers
Modern MVC applications benefit from a multi-layered caching approach. Here’s a Python implementation using Redis for caching:
from functools import lru_cache
import redis
class CacheService:
def __init__(self):
self.redis_client = redis.Redis(host='localhost', port=6379)
@lru_cache(maxsize=1000)
def get_cached_data(self, key):
# First check memory cache (LRU cache)
redis_data = self.redis_client.get(key)
if redis_data:
return redis_data
# If not in cache, fetch from database
data = self.database_service.get_data(key)
# Store in Redis with expiration
self.redis_client.setex(key, 3600, data)
return data
Java implementation using Spring Cache:
@Service
public class CacheService {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
@Cacheable(value = "dataCache", key = "#key")
public Object getCachedData(String key) {
// This method will automatically cache results
return databaseService.getData(key);
}
}
View Optimization Techniques
Template Caching and Compilation
Template optimization is crucial for view performance. Here’s an example using Jinja2 in Python:
from jinja2 import Environment, FileSystemLoader, select_autoescape
class TemplateManager:
def __init__(self):
self.env = Environment(
loader=FileSystemLoader('templates'),
autoescape=select_autoescape(['html', 'xml']),
cache_size=1000,
auto_reload=False # Disable in production
)
def render_template(self, template_name, context):
template = self.env.get_template(template_name)
return template.render(**context)
Java implementation using Thymeleaf:
@Configuration
public class ThymeleafConfig {
@Bean
public SpringTemplateEngine templateEngine() {
SpringTemplateEngine engine = new SpringTemplateEngine();
engine.setEnableSpringELCompiler(true);
engine.setCacheManager(new StandardCacheManager());
return engine;
}
}
Controller Optimization
Implementing Asynchronous Operations
Modern MVC applications benefit from asynchronous processing. Here’s a Python example using asyncio:
import asyncio
from fastapi import FastAPI
app = FastAPI()
class AsyncController:
@app.get("/api/data")
async def get_data(self):
async with AsyncSession() as session:
# Parallel execution of multiple queries
results = await asyncio.gather(
self.get_user_data(session),
self.get_product_data(session),
self.get_analytics_data(session)
)
return self.process_results(results)
Java implementation using Spring WebFlux:
@RestController
public class AsyncController {
@Autowired
private ReactiveService reactiveService;
@GetMapping("/api/data")
public Flux<Data> getData() {
return Flux.merge(
reactiveService.getUserData(),
reactiveService.getProductData(),
reactiveService.getAnalyticsData()
);
}
}
Memory Management and Resource Optimization
Implementing Resource Pooling
Efficient resource management is crucial for MVC application performance. Here’s a Python example of a connection pool:
from sqlalchemy.orm import sessionmaker
from sqlalchemy import create_engine
from contextlib import contextmanager
class DatabasePool:
def __init__(self, url, pool_size=5):
self.engine = create_engine(
url,
pool_size=pool_size,
max_overflow=2,
pool_timeout=30
)
self.Session = sessionmaker(bind=self.engine)
@contextmanager
def get_session(self):
session = self.Session()
try:
yield session
session.commit()
except:
session.rollback()
raise
finally:
session.close()
Java implementation using HikariCP:
@Configuration
public class DatabaseConfig {
@Bean
public DataSource dataSource() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost:5432/db");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(10);
config.setMinimumIdle(5);
return new HikariDataSource(config);
}
}
Performance Monitoring and Metrics
Implementing Performance Logging
Monitoring is essential for maintaining optimal performance. Here’s a Python implementation using decorators:
import time
import logging
from functools import wraps
def performance_logger(func):
@wraps(func)
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
execution_time = time.time() - start_time
logging.info(
f"Function: {func.__name__}, "
f"Execution Time: {execution_time:.2f}s, "
f"Arguments: {args}, {kwargs}"
)
return result
return wrapper
class ProductController:
@performance_logger
def get_products(self):
return self.product_service.get_all_products()
Java implementation using AOP:
@Aspect
@Component
public class PerformanceAspect {
private static final Logger logger = LoggerFactory.getLogger(PerformanceAspect.class);
@Around("@annotation(LogPerformance)")
public Object logPerformance(ProceedingJoinPoint joinPoint) throws Throwable {
long startTime = System.currentTimeMillis();
Object result = joinPoint.proceed();
long executionTime = System.currentTimeMillis() - startTime;
logger.info("Method: {}, Execution Time: {}ms",
joinPoint.getSignature().getName(),
executionTime);
return result;
}
}
Load Testing and Performance Benchmarking
Implementing Load Tests
Regular load testing helps identify performance issues before they affect production. Here’s a Python example using locust:
from locust import HttpUser, task, between
class MVCLoadTest(HttpUser):
wait_time = between(1, 3)
@task(3)
def view_products(self):
self.client.get("/api/products")
@task(1)
def create_product(self):
self.client.post("/api/products", json={
"name": "Test Product",
"price": 99.99,
"category": "Electronics"
})
Java implementation using JMeter programmatically:
@Test
public void loadTest() {
StandardJMeterEngine jmeter = new StandardJMeterEngine();
HashTreeMap testPlanTree = new HashTreeMap();
TestPlan testPlan = new TestPlan("MVC Load Test");
ThreadGroup threadGroup = new ThreadGroup();
threadGroup.setNumThreads(100);
threadGroup.setRampUp(10);
HTTPSamplerProxy httpSampler = new HTTPSamplerProxy();
httpSampler.setDomain("localhost");
httpSampler.setPort(8080);
httpSampler.setPath("/api/products");
testPlanTree.add(testPlan);
testPlanTree.add(threadGroup);
jmeter.configure(testPlanTree);
jmeter.run();
}
Conclusion
Optimizing MVC applications requires a comprehensive approach that addresses multiple aspects of the application stack. From database optimization to front-end performance, each layer presents opportunities for improvement. By implementing the techniques discussed in this guide, developers can significantly enhance their application’s performance and user experience. Remember that optimization is an ongoing process, and regular monitoring and testing are essential to maintain optimal performance as your application evolves.
Disclaimer: The code examples and performance optimization techniques presented in this blog post are based on best practices and general principles. Actual implementation details and performance improvements may vary depending on your specific use case, infrastructure, and requirements. While we strive for accuracy, some information may become outdated as technologies evolve. Please verify all implementations in your specific environment and report any inaccuracies so we can correct them promptly.