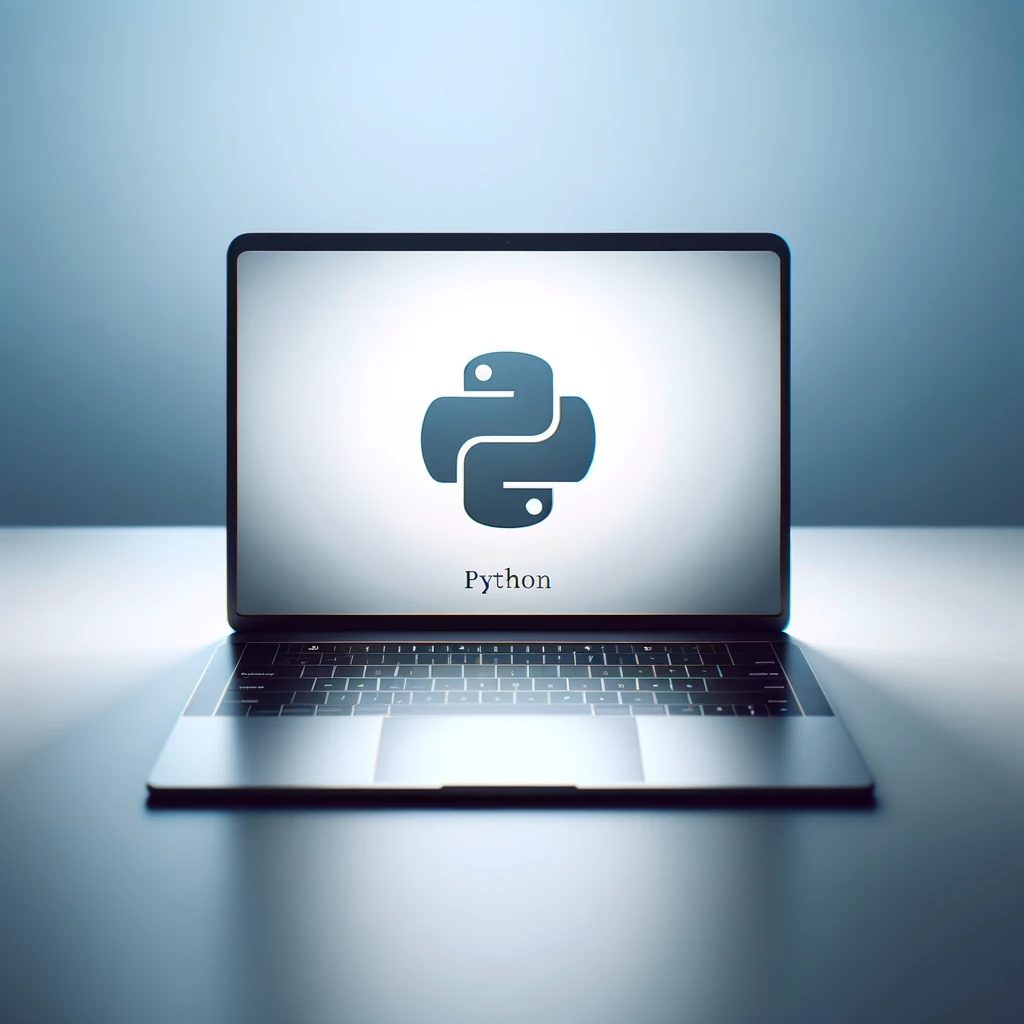
Python 101: Your First Step Towards AI Mastery
Hey there, future AI maestro! Are you ready to embark on an exhilarating journey into the world of artificial intelligence? Well, buckle up because we’re about to dive headfirst into the language that’s powering some of the most groundbreaking AI technologies out there – Python. Whether you’re a complete coding newbie or you’ve tinkered with a few lines here and there, this guide is your golden ticket to unlocking the vast potential of AI through Python. So, grab your favorite beverage, get comfy, and let’s unravel the mysteries of Python together. Trust me, by the time we’re done, you’ll be itching to create your very own AI masterpieces!
Why Python for AI?
Before we jump into the nitty-gritty of Python, let’s address the elephant in the room – why Python for AI? Well, my curious friend, Python has become the go-to language for AI and machine learning for some pretty compelling reasons. First off, it’s incredibly user-friendly and readable, making it perfect for beginners and experts alike. Imagine a programming language that reads almost like plain English – that’s Python for you! But don’t let its simplicity fool you; Python packs a serious punch when it comes to functionality.
The AI powerhouse
Python’s extensive library ecosystem is like a treasure trove for AI enthusiasts. With powerhouse libraries like TensorFlow, PyTorch, and scikit-learn at your fingertips, you can build sophisticated AI models without reinventing the wheel. These libraries offer pre-built functions and tools that make implementing complex AI algorithms a breeze. Plus, Python’s flexibility allows it to integrate seamlessly with other languages and technologies, making it a versatile choice for AI development across various platforms and industries.
Community and resources galore
Another feather in Python’s cap is its massive, vibrant community. When you’re learning AI with Python, you’re never alone. There’s a wealth of resources, tutorials, and forums where you can find answers to your questions, share your projects, and collaborate with fellow AI enthusiasts. This supportive ecosystem is invaluable, especially when you’re just starting out and need a helping hand or a burst of inspiration. So, are you convinced yet? Let’s dive into the basics and get you started on your Python journey!
Setting Up Your Python Environment
Alright, let’s get our hands dirty! Before we can start coding, we need to set up our Python environment. Don’t worry; it’s not as daunting as it sounds. Think of it as preparing your artist’s studio before creating a masterpiece. We want everything in its right place so we can focus on the fun part – coding!
Choosing your Python version
First things first, we need to decide which version of Python to use. As of now, Python 3.x is the way to go. It’s the most up-to-date version and is widely used in the AI community. Head over to the official Python website (python.org) and download the latest stable version for your operating system. The installation process is straightforward – just follow the prompts, and you’ll be done in no time.
Installing an Integrated Development Environment (IDE)
Now that we have Python installed, let’s talk about where we’ll be writing our code. While you could use a simple text editor, I highly recommend using an Integrated Development Environment (IDE) for a smoother coding experience. PyCharm and Visual Studio Code are popular choices among Python developers. These IDEs offer features like code completion, debugging tools, and project management, which can be incredibly helpful as you progress in your Python journey.
Setting up virtual environments
Before we wrap up our setup, let’s talk about virtual environments. These are isolated spaces where you can install packages and dependencies for specific projects without affecting your system-wide Python installation. It might sound a bit advanced, but trust me, it’s a good habit to get into from the start. You can create a virtual environment using the venv
module that comes with Python. This way, you can experiment freely without worrying about messing up your main Python installation. Pretty neat, right?
Python Basics: The Building Blocks of AI
Now that we’ve got our environment set up, it’s time to dive into the heart of Python. Don’t worry if you feel a bit overwhelmed at first – we’ll take it step by step, and before you know it, you’ll be writing Python code like a pro!
Variables and data types
Let’s start with the basics – variables and data types. In Python, variables are like containers that hold different types of data. You don’t need to declare the type of a variable explicitly; Python figures it out based on the value you assign. For example:
# Assigning values to variables
name = "Alice" # String
age = 25 # Integer
height = 5.7 # Float
is_student = True # Boolean
# Printing variables
print(f"Name: {name}, Age: {age}, Height: {height}, Student: {is_student}")
In this example, we’ve used four common data types: strings (for text), integers (for whole numbers), floats (for decimal numbers), and booleans (for true/false values). Python has many more data types, but these will get you started on your AI journey.
Control structures: If statements and loops
Control structures are the backbone of any programming language, allowing you to make decisions and repeat actions. Let’s look at if statements and loops:
# If statement
age = 20
if age >= 18:
print("You're an adult!")
else:
print("You're a minor.")
# For loop
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(f"I like {fruit}!")
# While loop
count = 0
while count < 5:
print(f"Count is {count}")
count += 1
These control structures allow you to create more complex and dynamic programs. You’ll use them extensively when working with AI algorithms to make decisions based on data and iterate through large datasets.
Functions: Your code’s best friend
Functions are reusable blocks of code that perform specific tasks. They’re crucial in AI programming for organizing your code and making it more modular. Here’s a simple function example:
def greet(name):
return f"Hello, {name}! Welcome to the world of AI."
# Using the function
message = greet("Alice")
print(message)
As you delve deeper into AI, you’ll create and use more complex functions to handle data preprocessing, model training, and result analysis. Functions help keep your code clean, readable, and maintainable – all essential qualities when working on large AI projects.
Data Structures: The Backbone of AI Algorithms
Now that we’ve covered the basics, let’s talk about data structures. In AI and machine learning, you’ll be working with vast amounts of data, and understanding how to efficiently store and manipulate this data is crucial. Python offers several built-in data structures that are incredibly useful for AI applications.
Lists: Your versatile data container
Lists are one of the most versatile data structures in Python. They can hold multiple items of different types and are mutable, meaning you can change their contents after creation. Here’s how you can work with lists:
# Creating a list
fruits = ["apple", "banana", "cherry"]
# Adding an item to the list
fruits.append("date")
# Accessing items
print(fruits[0]) # Prints "apple"
# Slicing
print(fruits[1:3]) # Prints ["banana", "cherry"]
# List comprehension
squared_numbers = [x**2 for x in range(5)]
print(squared_numbers) # Prints [0, 1, 4, 9, 16]
Lists are great for storing sequences of data, like time series or features in a machine learning model. The list comprehension shown above is a powerful tool for creating new lists based on existing ones – a common operation in data preprocessing for AI.
Dictionaries: Key-value pairs for the win
Dictionaries are another essential data structure in Python. They store key-value pairs, making them perfect for representing complex data structures. Here’s a quick example:
# Creating a dictionary
person = {
"name": "Alice",
"age": 25,
"skills": ["Python", "Machine Learning", "Data Analysis"]
}
# Accessing values
print(person["name"]) # Prints "Alice"
# Adding new key-value pairs
person["location"] = "New York"
# Iterating through a dictionary
for key, value in person.items():
print(f"{key}: {value}")
Dictionaries are incredibly useful in AI for storing model parameters, configuration settings, or representing complex data structures like JSON responses from APIs.
NumPy arrays: The powerhouse of numerical computing
While not a built-in Python data structure, NumPy arrays deserve a special mention. NumPy is a fundamental library for scientific computing in Python and is extensively used in AI and machine learning. Here’s a taste of what NumPy can do:
import numpy as np
# Creating a NumPy array
arr = np.array([1, 2, 3, 4, 5])
# Basic operations
print(arr * 2) # Prints [2 4 6 8 10]
# Creating a 2D array
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Matrix operations
print(matrix.T) # Transpose of the matrix
NumPy arrays are optimized for numerical operations, making them much faster than regular Python lists when dealing with large amounts of data. As you progress in your AI journey, you’ll find yourself using NumPy arrays extensively for tasks like data preprocessing, linear algebra operations, and implementing neural networks.
Object-Oriented Programming: Structuring Your AI Projects
As your AI projects grow in complexity, you’ll need a way to organize your code more efficiently. This is where Object-Oriented Programming (OOP) comes in. OOP is a programming paradigm that allows you to structure your code around objects, which are instances of classes. This approach can make your AI projects more modular, reusable, and easier to maintain.
Classes and objects: The building blocks of OOP
A class is like a blueprint for creating objects. It defines the attributes (data) and methods (functions) that the objects of that class will have. Here’s a simple example:
class AIModel:
def __init__(self, name, algorithm):
self.name = name
self.algorithm = algorithm
self.is_trained = False
def train(self, data):
print(f"Training {self.name} using {self.algorithm} algorithm...")
# Training logic would go here
self.is_trained = True
def predict(self, input_data):
if not self.is_trained:
print("Error: Model not trained yet!")
return None
print(f"Making predictions using {self.name}...")
# Prediction logic would go here
return "Prediction result"
# Creating an instance of the AIModel class
my_model = AIModel("SuperAI", "Deep Learning")
# Using the object
my_model.train("training_data")
result = my_model.predict("input_data")
print(result)
In this example, we’ve defined an AIModel
class with attributes like name
and algorithm
, and methods like train
and predict
. We can create multiple instances of this class, each representing a different AI model with its own set of attributes and behaviors.
Inheritance: Building on existing foundations
Inheritance is a powerful OOP concept that allows you to create new classes based on existing ones. This is particularly useful in AI when you want to create specialized versions of more general algorithms. Let’s extend our previous example:
class ImageClassifier(AIModel):
def __init__(self, name, algorithm, image_size):
super().__init__(name, algorithm)
self.image_size = image_size
def preprocess_image(self, image):
print(f"Resizing image to {self.image_size}x{self.image_size}")
# Image preprocessing logic would go here
def predict(self, image):
self.preprocess_image(image)
return super().predict(image)
# Creating an instance of the ImageClassifier class
img_classifier = ImageClassifier("ImageNet", "CNN", 224)
# Using the specialized object
img_classifier.train("image_dataset")
result = img_classifier.predict("test_image.jpg")
print(result)
Here, we’ve created an ImageClassifier
class that inherits from AIModel
. It adds a new attribute (image_size
) and a new method (preprocess_image
), while also overriding the predict
method to include image preprocessing.
Encapsulation and abstraction: Hiding complexity
OOP allows you to encapsulate complex logic within objects and provide a simpler interface for using them. This is particularly useful in AI, where you often want to hide the intricate details of algorithms behind easy-to-use methods. For example:
class NeuralNetwork:
def __init__(self, layers):
self.layers = layers
self._weights = None # Private attribute
def _initialize_weights(self):
# Complex weight initialization logic
pass
def train(self, data):
self._initialize_weights()
# Complex training logic
pass
def predict(self, input_data):
# Complex prediction logic
pass
# Using the NeuralNetwork class
nn = NeuralNetwork([64, 32, 10])
nn.train("training_data")
result = nn.predict("test_input")
In this example, the user of the NeuralNetwork
class doesn’t need to know about the complex weight initialization or the intricacies of the training algorithm. They can simply call train
and predict
methods, making the neural network easy to use while hiding its complexity.
Working with Data: Pandas for AI Preprocessing
When it comes to AI and machine learning, data is king. But raw data is rarely in the perfect format for our models. This is where Pandas, a powerful data manipulation library, comes into play. Pandas provides high-performance, easy-to-use data structures and data analysis tools for Python. Let’s explore how Pandas can help us prepare data for our AI models.
Introducing DataFrames
The main data structure in Pandas is the DataFrame, which you can think of as a spreadsheet in Python. It’s a 2-dimensional labeled data structure with columns of potentially different types. Here’s how you can create and manipulate DataFrames:
import pandas as pd
# Creating a DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'San Francisco', 'Los Angeles']
}
df = pd.DataFrame(data)
# Displaying the DataFrame
print(df)
# Accessing columns
print(df['Name'])
# Adding a new column
df['Occupation'] = ['Engineer', 'Designer', 'Manager']
# Filtering data
young_people = df[df['Age'] < 30]
print(young_people)
DataFrames make it easy to handle structured data, which is crucial for many AI tasks. You can load data from various sources (CSV, Excel, databases) into a DataFrame, manipulate it, and then feed it into your AI models.
Data cleaning and preprocessing
Real-world data is often messy. It may contain missing values, outliers, or be in the wrong format. Pandas provides numerous tools to clean and preprocess your data:
# Handling missing values
df['Salary'] = [50000, None, 75000]
df['Salary'].fillna(df['Salary'].mean(), inplace=True)
# Converting data types
df['Age'] = df['Age'].astype(float)
# Removing duplicates
df.drop_duplicates(inplace=True)
# Renaming columns
df.rename(columns={'Name': 'Full Name'}, inplace=True)
# Applying functions to columns
df['Age'] = df['Age'].apply(lambda x: x + 1)
These operations allow you to transform your data into a format that’s suitable for your AI models. For example, many machine learning algorithms can’t handle missing values, so you need to either remove them or fill them in before training your model.
Data aggregation and grouping
# Grouping data
grouped = df.groupby('City')
# Aggregating data
city_stats = grouped.agg({
'Age': ['mean', 'max'],
'Salary': ['mean', 'min', 'max']
})
print(city_stats)
# Pivot tables
pivot = df
pivot = df.pivot_table(values='Salary', index='City', columns='Occupation', aggfunc='mean')
print(pivot)
These aggregation and pivoting capabilities are invaluable when preparing data for machine learning models. They allow you to create new features, summarize large datasets, and uncover patterns in your data that could be useful for your AI algorithms.
Visualizing Data: Matplotlib and Seaborn
In AI and machine learning, visualizing your data is crucial. It helps you understand your dataset, identify patterns, and communicate your results effectively. Python offers excellent libraries for data visualization, with Matplotlib and Seaborn being two of the most popular. Let’s explore how to use these libraries to create insightful visualizations.
Getting started with Matplotlib
Matplotlib is the foundation for data visualization in Python. It provides a MATLAB-like interface for creating a wide range of plots. Here’s a simple example:
import matplotlib.pyplot as plt
import numpy as np
# Creating data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Creating the plot
plt.figure(figsize=(10, 6))
plt.plot(x, y, label='Sine wave')
plt.title('A Simple Sine Wave')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.grid(True)
plt.show()
This code creates a simple sine wave plot. Matplotlib gives you fine-grained control over every aspect of your plots, from colors and line styles to axes and legends.
Advanced plotting with Seaborn
While Matplotlib is powerful, Seaborn builds on top of it to provide a higher-level interface for creating attractive and informative statistical graphics. It’s particularly useful for visualizing complex datasets:
import seaborn as sns
import pandas as pd
# Creating a sample dataset
data = pd.DataFrame({
'x': np.random.randn(100),
'y': np.random.randn(100),
'category': np.random.choice(['A', 'B', 'C'], 100)
})
# Creating a scatter plot with Seaborn
plt.figure(figsize=(10, 6))
sns.scatterplot(data=data, x='x', y='y', hue='category', style='category')
plt.title('Scatter Plot with Categories')
plt.show()
# Creating a box plot
plt.figure(figsize=(10, 6))
sns.boxplot(data=data, x='category', y='y')
plt.title('Box Plot of Y by Category')
plt.show()
Seaborn excels at creating statistical visualizations like scatter plots, box plots, and heatmaps, which are often used in exploratory data analysis for AI projects.
Introduction to Machine Learning with Scikit-learn
Now that we’ve covered the basics of Python, data handling, and visualization, let’s dip our toes into the exciting world of machine learning. Scikit-learn is a powerful library that provides simple and efficient tools for data analysis and modeling. It includes various machine learning algorithms and utilities for tasks like preprocessing, model selection, and evaluation.
Preparing data for machine learning
Before we can train a model, we need to prepare our data. Scikit-learn provides tools for this:
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Assuming we have features X and target y
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Scaling the features
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
Here, we’ve split our data into training and testing sets, and scaled our features to have zero mean and unit variance, which is often beneficial for many machine learning algorithms.
Training a simple model
Let’s train a simple classification model using Scikit-learn:
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, classification_report
# Creating and training the model
model = LogisticRegression()
model.fit(X_train_scaled, y_train)
# Making predictions
y_pred = model.predict(X_test_scaled)
# Evaluating the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
print(classification_report(y_test, y_pred))
This code trains a logistic regression model, makes predictions on the test set, and evaluates the model’s performance using accuracy and a classification report.
Model selection and evaluation
Scikit-learn also provides tools for more advanced model selection and evaluation:
from sklearn.model_selection import cross_val_score, GridSearchCV
from sklearn.svm import SVC
# Cross-validation
scores = cross_val_score(model, X_train_scaled, y_train, cv=5)
print(f"Cross-validation scores: {scores}")
print(f"Mean accuracy: {scores.mean():.2f}")
# Grid search for hyperparameter tuning
param_grid = {'C': [0.1, 1, 10], 'kernel': ['rbf', 'linear']}
grid_search = GridSearchCV(SVC(), param_grid, cv=5)
grid_search.fit(X_train_scaled, y_train)
print(f"Best parameters: {grid_search.best_params_}")
print(f"Best cross-validation score: {grid_search.best_score_:.2f}")
These techniques help you validate your model’s performance and find the best hyperparameters for your algorithm.
Next Steps: Diving Deeper into AI
Congratulations! You’ve taken your first steps into the world of AI with Python. But this is just the beginning of your journey. Here are some suggestions for your next steps:
- Deep Learning: Explore deep learning libraries like TensorFlow or PyTorch to build neural networks and tackle more complex AI tasks.
- Natural Language Processing: Dive into libraries like NLTK or spaCy to work with text data and build language models.
- Computer Vision: Learn about image processing and computer vision using libraries like OpenCV and explore how to build image classification and object detection models.
- Reinforcement Learning: Discover how to create AI agents that learn from their environment using libraries like OpenAI Gym.
- Big Data: Explore tools like Apache Spark with PySpark for handling large-scale data processing for AI applications.
- AI Ethics: As you progress, don’t forget to learn about the ethical implications of AI and how to develop responsible AI systems.
Remember, the field of AI is vast and constantly evolving. The key is to stay curious, keep learning, and practice regularly. Build projects, participate in AI competitions on platforms like Kaggle, and engage with the AI community. With Python as your foundation, you’re well-equipped to explore the exciting frontiers of artificial intelligence.
As you embark on this thrilling journey into the world of AI, remember that every expert was once a beginner. Embrace the challenges, celebrate your successes, and don’t be afraid to make mistakes – they’re all part of the learning process. The AI revolution is happening right now, and with your newfound Python skills, you’re ready to be a part of it. So go forth, code fearlessly, and let your AI adventures begin!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, the field of AI is rapidly evolving. Some details may become outdated over time. We encourage readers to verify information and consult official documentation for the most up-to-date guidance. If you notice any inaccuracies, please report them so we can correct them promptly.