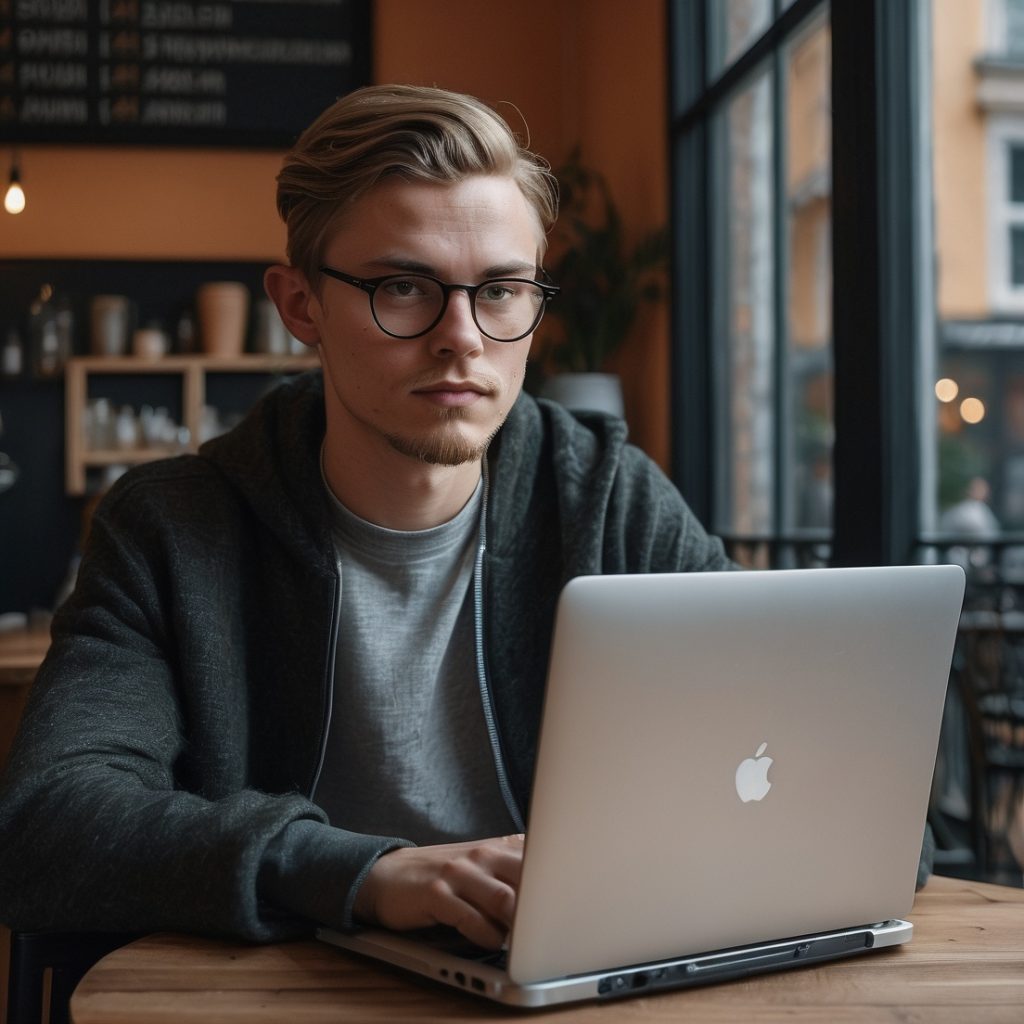
Python for the Perplexed: Taming Data Dragons with Elegance
Welcome, fellow data wranglers and Python enthusiasts, to a mystical journey where we transform raw data into polished, actionable insights. If you’ve ever felt overwhelmed by the unruly data dragons in your datasets, fear not! With Python’s elegant tools and libraries, we will tame these beasts and make data manipulation not just manageable but enjoyable.
Why Python for Data Analysis?
Python has emerged as a go-to language for data analysis, and for good reason. It’s versatile, easy to learn, and supported by a rich ecosystem of libraries that make data processing a breeze. Whether you’re dealing with CSV files, databases, or web data, Python has you covered.
The Power of Simplicity
Python’s syntax is clean and readable, making it an excellent choice for both beginners and experienced programmers. Its simplicity allows you to focus on solving problems rather than getting bogged down by complex syntax. Here’s a quick example:
# Read a CSV file
import pandas as pd
data = pd.read_csv('data.csv')
print(data.head())
In just a few lines, you can read and display the first few rows of a CSV file. This ease of use is one of Python’s greatest strengths.
A Rich Ecosystem
Python’s ecosystem is second to none when it comes to data analysis. Libraries like Pandas, NumPy, Matplotlib, and Scikit-learn provide robust tools for data manipulation, visualization, and machine learning. Each of these libraries is designed to handle specific aspects of data analysis, making your workflow more efficient.
Getting Started with Pandas
Pandas is the backbone of data manipulation in Python. It provides data structures and functions needed to manipulate structured data seamlessly. Let’s dive into some basic operations.
Reading Data
Reading data into Pandas is straightforward. You can read from various formats such as CSV, Excel, SQL databases, and more.
import pandas as pd
# Read a CSV file
data = pd.read_csv('data.csv')
# Read an Excel file
data_excel = pd.read_excel('data.xlsx')
# Read from a SQL database
import sqlite3
conn = sqlite3.connect('database.db')
data_sql = pd.read_sql_query('SELECT * FROM table_name', conn)
Exploring Data
Once you have your data, Pandas provides several ways to explore it. You can inspect the first few rows, get a summary of the data, and check for missing values.
# Display the first few rows
print(data.head())
# Summary statistics
print(data.describe())
# Check for missing values
print(data.isnull().sum())
Data Cleaning
Data is rarely clean and ready for analysis. Pandas offers powerful tools for cleaning data, such as handling missing values, removing duplicates, and transforming data types.
# Drop rows with missing values
data_clean = data.dropna()
# Fill missing values with a specific value
data_filled = data.fillna(0)
# Remove duplicate rows
data_unique = data.drop_duplicates()
# Convert data types
data['column_name'] = data['column_name'].astype('int')
Advanced Data Manipulation with Pandas
Once you’ve got the basics down, it’s time to explore more advanced data manipulation techniques with Pandas. These techniques will help you reshape, merge, and aggregate your data efficiently.
Reshaping Data
Reshaping data involves changing its structure without altering the actual data. Pandas provides functions like pivot
, melt
, and stack
to make this process easier.
# Pivoting data
pivot_table = data.pivot(index='date', columns='category', values='value')
# Melting data
melted_data = data.melt(id_vars=['date'], value_vars=['category1', 'category2'])
# Stacking data
stacked_data = data.stack()
Merging DataFrames
Merging data from multiple DataFrames is a common task in data analysis. Pandas offers functions like merge
, join
, and concat
to combine DataFrames in various ways.
# Merging DataFrames
merged_data = pd.merge(data1, data2, on='common_column')
# Joining DataFrames
joined_data = data1.join(data2.set_index('common_column'), on='common_column')
# Concatenating DataFrames
concatenated_data = pd.concat([data1, data2], axis=0)
Group By and Aggregation
Grouping and aggregating data is essential for summarizing and analyzing large datasets. Pandas’ groupby
function allows you to group data by specific columns and apply aggregate functions to each group.
# Group by a column and aggregate
grouped_data = data.groupby('category').agg({'value': 'sum', 'another_value': 'mean'})
# Applying multiple aggregations
multiple_aggregations = data.groupby('category').agg({'value': ['sum', 'mean'], 'another_value': 'max'})
Visualization with Matplotlib and Seaborn
Visualizing data is crucial for understanding and communicating insights. Python’s Matplotlib and Seaborn libraries provide powerful tools for creating a wide range of visualizations.
Matplotlib Basics
Matplotlib is a versatile plotting library that allows you to create static, animated, and interactive visualizations. Here’s a simple example of a line plot.
import matplotlib.pyplot as plt
# Simple line plot
plt.plot(data['date'], data['value'])
plt.title('Line Plot')
plt.xlabel('Date')
plt.ylabel('Value')
plt.show()
Seaborn for Statistical Plots
Seaborn builds on Matplotlib and provides a high-level interface for drawing attractive and informative statistical graphics. It is particularly useful for visualizing data distributions and relationships between variables.
import seaborn as sns
# Histogram
sns.histplot(data['value'], bins=30)
plt.title('Histogram')
plt.show()
# Scatter plot with regression line
sns.lmplot(x='value', y='another_value', data=data)
plt.title('Scatter Plot with Regression Line')
plt.show()
# Heatmap
pivot_table = data.pivot('date', 'category', 'value')
sns.heatmap(pivot_table, annot=True, fmt="g", cmap='viridis')
plt.title('Heatmap')
plt.show()
Working with Time Series Data
Time series data is ubiquitous in many fields, from finance to weather forecasting. Python’s Pandas library provides excellent support for handling time series data.
Parsing Dates
When working with time series data, the first step is often to ensure that date columns are parsed correctly.
# Parse dates during CSV reading
data = pd.read_csv('time_series_data.csv', parse_dates=['date_column'])
# Set the date column as the index
data.set_index('date_column', inplace=True)
Resampling and Rolling Windows
Resampling is the process of converting a time series to a different frequency. Rolling windows allow you to compute statistics over a moving window.
# Resample to monthly frequency
monthly_data = data.resample('M').mean()
# Rolling window calculations
rolling_mean = data['value'].rolling(window=12).mean()
Machine Learning with Scikit-learn
Python’s Scikit-learn library provides simple and efficient tools for data mining and data analysis. It is built on NumPy, SciPy, and Matplotlib and integrates well with Pandas.
Preprocessing Data
Before applying machine learning algorithms, it’s crucial to preprocess your data. This includes handling missing values, encoding categorical variables, and scaling features.
from sklearn.preprocessing import StandardScaler, OneHotEncoder
from sklearn.compose import ColumnTransformer
from sklearn.pipeline import Pipeline
# Define a pipeline for preprocessing
numeric_features = ['numeric_column1', 'numeric_column2']
numeric_transformer = Pipeline(steps=[
('scaler', StandardScaler())])
categorical_features = ['categorical_column']
categorical_transformer = Pipeline(steps=[
('encoder', OneHotEncoder(handle_unknown='ignore'))])
preprocessor = ColumnTransformer(
transformers=[
('num', numeric_transformer, numeric_features),
('cat', categorical_transformer, categorical_features)])
# Fit and transform the data
X = data.drop('target', axis=1)
y = data['target']
X_preprocessed = preprocessor.fit_transform(X)
Training and Evaluating Models
Scikit-learn provides a consistent interface for training and evaluating machine learning models. Here’s an example of training a logistic regression model.
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X_preprocessed, y, test_size=0.2, random_state=42)
# Train a logistic regression model
model = LogisticRegression()
model.fit(X_train, y_train)
# Predict on the test set
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
Conclusion
Python is an exceptional tool for taming the dragons of data. Its simplicity, combined with a powerful ecosystem of libraries, makes it an ideal choice for data analysis and machine learning. From reading and cleaning data with Pandas to visualizing insights with Matplotlib and Seaborn, and even building machine learning models with Scikit-learn, Python equips you with everything you need to conquer your data challenges.
So, whether you’re a novice data enthusiast or an experienced analyst, Python offers elegant solutions to complex problems. Embrace its capabilities, explore its vast libraries, and let Python