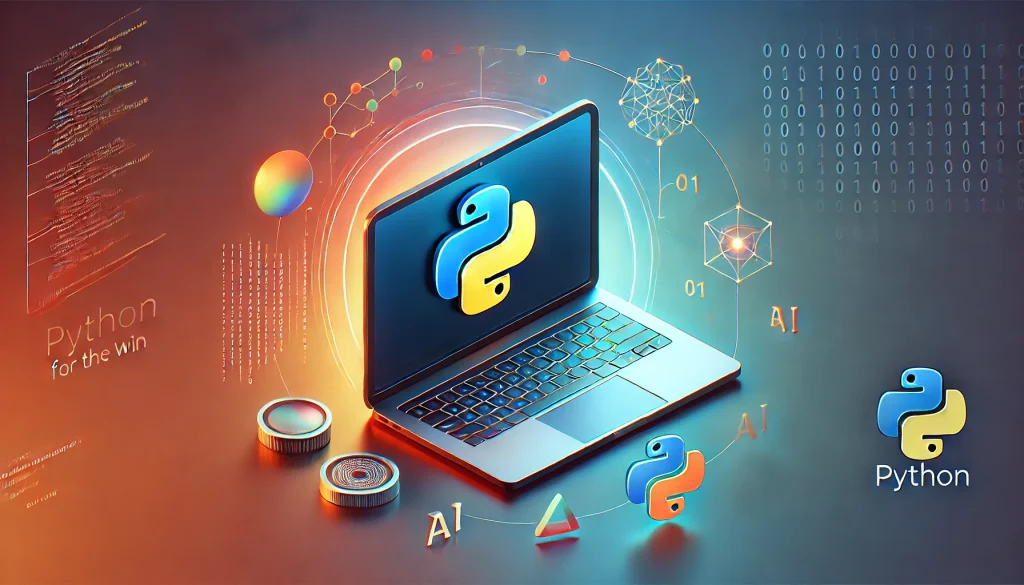
Python for the Win: Why It’s the Hottest Skill in AI
Artificial Intelligence (AI) has taken the world by storm, revolutionizing industries from healthcare to finance, entertainment to transportation. If you’re looking to break into this dynamic field, there’s one skill that stands out above the rest: Python. But why is Python considered the hottest skill in AI? Let’s dive into the reasons why Python has become the go-to language for AI enthusiasts and professionals alike.
The Rise of Python in AI
Python’s journey to becoming the favorite language for AI isn’t a fluke. Its growth is fueled by several key factors that make it an ideal choice for developing AI applications. From its simplicity to its extensive libraries, Python offers numerous advantages that set it apart.
Ease of Learning and Use
Python’s syntax is clear and intuitive, making it accessible to beginners and experts alike. Unlike languages with steep learning curves, Python’s readable code allows developers to focus on solving problems rather than struggling with complex syntax. This ease of use is particularly valuable in AI, where the focus is on creating and refining algorithms rather than getting bogged down by language intricacies.
# Example: Simple Python program to add two numbers
def add_numbers(a, b):
return a + b
result = add_numbers(3, 5)
print(f"The sum is: {result}")
Extensive Libraries and Frameworks
One of Python’s greatest strengths in AI development is its extensive collection of libraries and frameworks. These tools simplify the implementation of complex algorithms and data processing tasks, saving valuable time and effort. Here are a few of the most popular ones:
- NumPy: Essential for numerical computing and handling arrays.
- Pandas: Ideal for data manipulation and analysis.
- Matplotlib: Useful for creating visualizations.
- SciPy: Provides advanced mathematical functions and algorithms.
- Scikit-learn: A robust library for machine learning.
- TensorFlow and Keras: Powerful frameworks for deep learning.
# Example: Using NumPy to perform basic operations
import numpy as np
array = np.array([1, 2, 3, 4, 5])
print("Original array:", array)
squared_array = np.square(array)
print("Squared array:", squared_array)
Python’s Role in Machine Learning and Deep Learning
Machine Learning (ML) and Deep Learning (DL) are two of the most exciting areas in AI, and Python plays a crucial role in both. Let’s explore how Python facilitates the development and deployment of ML and DL models.
Machine Learning with Python
Machine Learning involves training algorithms to make predictions or decisions based on data. Python’s simplicity and readability make it a favorite among data scientists. Libraries like Scikit-learn provide all the tools needed to implement and evaluate machine learning models, from regression to classification, clustering to dimensionality reduction.
# Example: Implementing a simple linear regression with Scikit-learn
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 6, 8, 10])
# Splitting data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Training the model
model = LinearRegression()
model.fit(X_train, y_train)
# Making predictions
predictions = model.predict(X_test)
print("Predictions:", predictions)
# Evaluating the model
mse = mean_squared_error(y_test, predictions)
print("Mean Squared Error:", mse)
Deep Learning with Python
Deep Learning, a subset of Machine Learning, uses neural networks with many layers (hence the term “deep”) to model complex patterns in data. Python’s TensorFlow and Keras frameworks make it easier to build, train, and deploy deep neural networks.
# Example: Building a simple neural network with Keras
from keras.models import Sequential
from keras.layers import Dense
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 6, 8, 10])
# Defining the model
model = Sequential()
model.add(Dense(units=1, input_dim=1, activation='linear'))
# Compiling the model
model.compile(optimizer='adam', loss='mean_squared_error')
# Training the model
model.fit(X, y, epochs=100, verbose=1)
# Making predictions
predictions = model.predict(X)
print("Predictions:", predictions)
Python’s Versatility in AI Applications
Python isn’t just limited to academic research or prototype development. Its versatility extends to a wide range of AI applications in real-world scenarios.
Natural Language Processing (NLP)
Natural Language Processing is a branch of AI focused on the interaction between computers and human language. Python’s NLTK and spaCy libraries are powerful tools for NLP tasks, enabling developers to process and analyze large volumes of text data.
# Example: Basic NLP with NLTK
import nltk
from nltk.tokenize import word_tokenize
text = "Python is a powerful language for AI."
tokens = word_tokenize(text)
print("Tokens:", tokens)
Computer Vision
Computer Vision is another exciting field where Python shines. Libraries like OpenCV and frameworks like TensorFlow enable developers to create applications that can interpret and understand visual data from the world.
# Example: Basic image processing with OpenCV
import cv2
# Load an image
image = cv2.imread('path_to_image.jpg')
# Convert to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Save the grayscale image
cv2.imwrite('gray_image.jpg', gray_image)
print("Grayscale image saved.")
Reinforcement Learning
Reinforcement Learning (RL) is a type of machine learning where an agent learns to make decisions by performing actions and receiving rewards. Python’s Gym library, developed by OpenAI, provides a wide range of environments to develop and test RL algorithms.
# Example: Basic reinforcement learning setup with Gym
import gym
# Create the CartPole environment
env = gym.make('CartPole-v1')
# Reset the environment
state = env.reset()
# Take a random action
action = env.action_space.sample()
next_state, reward, done, info = env.step(action)
print(f"Next state: {next_state}, Reward: {reward}, Done: {done}")
The Future of Python in AI
Python’s popularity in AI is unlikely to wane anytime soon. Its continuous evolution and the vibrant community supporting it ensure that Python will remain at the forefront of AI development. As new algorithms and techniques emerge, Python will adapt, providing developers with the tools they need to push the boundaries of what’s possible.
Community and Support
Python’s vast and active community is one of its greatest assets. Whether you’re a beginner or an experienced developer, there’s always someone willing to help. Online forums, tutorials, and documentation make it easy to find solutions to problems and learn new skills.
Integration with Other Technologies
Python’s ability to integrate with other technologies and languages enhances its utility in AI. Whether you’re working with big data platforms like Apache Spark or deploying models with cloud services like AWS and Google Cloud, Python’s flexibility makes it an ideal choice.
# Example: Integrating Python with Spark for big data processing
from pyspark.sql import SparkSession
# Create a Spark session
spark = SparkSession.builder.appName("PythonAIExample").getOrCreate()
# Load a dataset
data = spark.read.csv('path_to_data.csv', header=True, inferSchema=True)
# Show the first few rows
data.show()
Conclusion
Python’s dominance in AI is no accident. Its ease of learning, extensive libraries, and versatility make it the perfect language for developing AI applications. Whether you’re just starting out or looking to advance your career, mastering Python will give you a significant edge in the rapidly evolving field of AI.
So, what are you waiting for? Dive into Python, experiment with its libraries, and start building the AI of the future. Python for the win, indeed!
Disclaimer: The code snippets provided in this blog are for educational purposes only. Please ensure you understand and test the code in your own environment. Report any inaccuracies so we can correct them promptly.