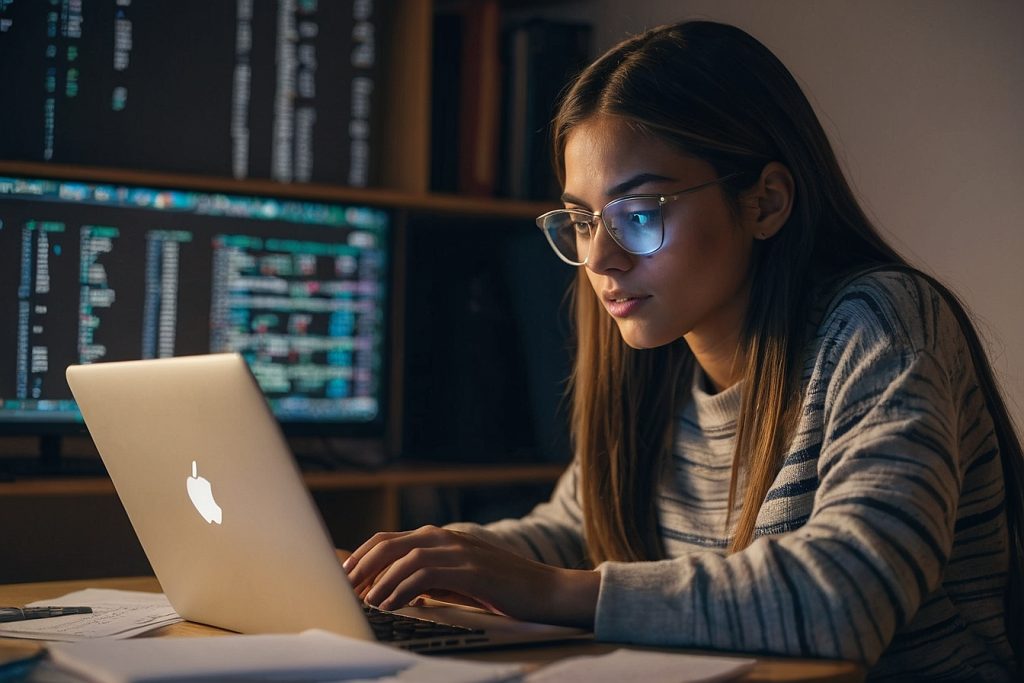
Python Naming Conventions for Beginners
Python is a popular programming language known for its simplicity and readability. One of the key aspects that contribute to Python’s readability is its naming conventions. Adhering to these conventions ensures that your code is consistent, easy to understand, and maintainable. In this blog post, we’ll explore the essential naming conventions in Python, which every beginner should know.
- Variable Names
Variable names in Python should be descriptive and follow the snake_case convention. This means that words are separated by underscores, and all letters are lowercase. For example:
user_name = "John Doe"
total_cost = 25.99
is_active = True
- Function Names
Like variable names, function names should also follow the snake_case convention. However, it’s a common practice to use verb phrases to describe the action performed by the function. For example:
def calculate_area(length, width):
return length * width
def is_palindrome(word):
return word == word[::-1]
- Class Names
Class names in Python follow the CapitalizedWords convention, also known as PascalCase. Each word in the class name should start with a capital letter. For example:
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self.balance = balance
- Module Names
Python modules should have short, all-lowercase names. Underscores can be used to separate words in module names if necessary. For example:
# module_name.py
import math
import os
- Constants
Constants in Python are typically defined as variables with all-uppercase letters and words separated by underscores. This convention makes it easy to distinguish constants from other variables. For example:
PI = 3.14159
MAX_ATTEMPTS = 5
DAYS_IN_WEEK = 7
- Private Attributes and Methods
In Python, there is no strict concept of private attributes or methods. However, it is a convention to prefix private attributes and methods with a single underscore (_
). This indicates that these elements should be treated as private and not accessed directly from outside the class. For example:
class Circle:
def __init__(self, radius):
self.radius = radius
def _calculate_circumference(self):
return 2 * 3.14159 * self.radius
By following these naming conventions, your Python code will be more readable, consistent, and easier to maintain. It also makes collaboration with other developers smoother, as everyone adheres to the same standards. As a beginner, practicing these conventions from the start will help you develop good coding habits and write cleaner, more professional Python code.