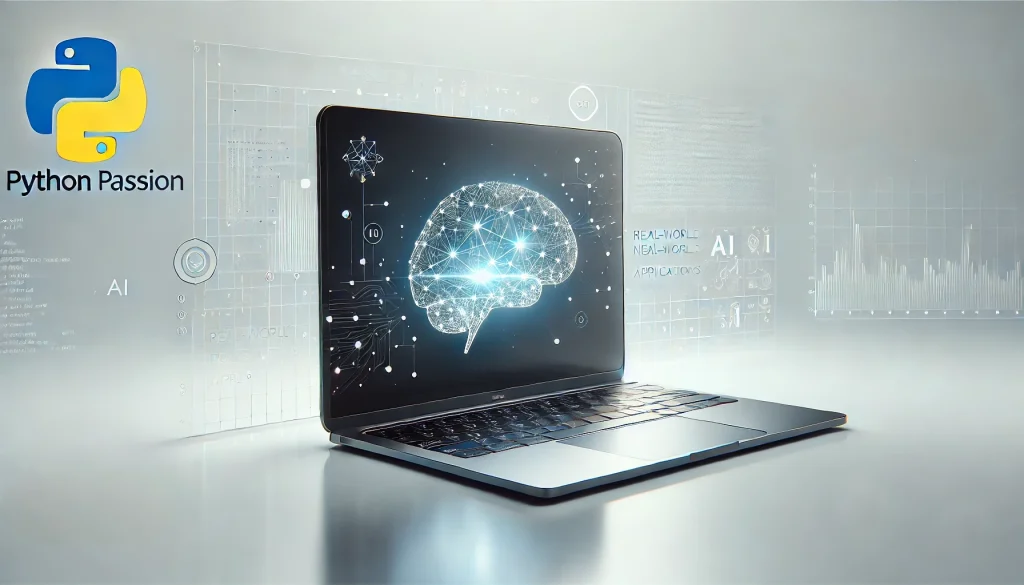
Python Passion: Real-World AI Applications You Can Build
Artificial Intelligence (AI) has become an integral part of our daily lives. From the recommendations on Netflix to the voice recognition on your smartphone, AI is everywhere. For college students and young professionals, diving into the world of AI can seem daunting, but Python makes it incredibly accessible. In this blog, we’ll explore real-world AI applications you can build using Python. We’ll cover several projects with detailed explanations, code snippets, and scripts to help you get started.
Understanding AI and Python
Python is one of the most popular programming languages for AI due to its simplicity, readability, and extensive libraries. Libraries such as TensorFlow, Keras, PyTorch, and scikit-learn provide powerful tools to develop sophisticated AI models. Let’s dive into some exciting projects!
Building a Spam Classifier
Email spam is a persistent problem. Let’s create a spam classifier using machine learning to filter out unwanted emails.
Step 1: Data Collection
First, gather a dataset of emails labeled as spam or not spam. You can find datasets like the Enron email dataset online.
Step 2: Preprocessing the Data
Before feeding the data into a machine learning model, we need to preprocess it. This involves cleaning the text and converting it into numerical data.
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import CountVectorizer
# Load the dataset
emails = pd.read_csv('emails.csv')
# Split the dataset
X_train, X_test, y_train, y_test = train_test_split(emails['text'], emails['spam'], test_size=0.2)
# Vectorize the text data
vectorizer = CountVectorizer()
X_train_vec = vectorizer.fit_transform(X_train)
X_test_vec = vectorizer.transform(X_test)
Step 3: Training the Model
We’ll use a simple logistic regression model for this task.
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Train the model
model = LogisticRegression()
model.fit(X_train_vec, y_train)
# Predict on the test set
y_pred = model.predict(X_test_vec)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy * 100:.2f}%')
With this simple model, you can achieve a decent accuracy in classifying spam emails. You can further improve this by using more advanced models like Random Forest or Neural Networks.
Creating a Chatbot
Chatbots are widely used in customer service, providing 24/7 assistance. Let’s create a basic chatbot using Python’s NLTK library.
Step 1: Installing Required Libraries
First, install the required libraries.
pip install nltk
Step 2: Preparing the Data
We’ll use a simple dataset of questions and answers.
import nltk
from nltk.chat.util import Chat, reflections
pairs = [
['my name is (.*)', ['Hello %1, how can I help you today?']],
['(hi|hello|hey)', ['Hello!', 'Hey there!']],
['what is your name?', ['I am a chatbot created using Python.']],
['(.*) created you?', ['I was created by an aspiring AI developer!']]
]
chatbot = Chat(pairs, reflections)
Step 3: Running the Chatbot
print("Hi! I am your chatbot. Type 'quit' to exit.")
while True:
user_input = input("You: ")
if user_input.lower() == 'quit':
break
response = chatbot.respond(user_input)
print(f'Bot: {response}')
This simple chatbot can be expanded by adding more pairs and integrating it with other NLP techniques to make it more sophisticated.
Image Recognition with Convolutional Neural Networks (CNNs)
Image recognition is a key application of AI. Let’s build an image classifier using a Convolutional Neural Network (CNN).
Step 1: Installing Required Libraries
pip install tensorflow keras
Step 2: Preparing the Dataset
We’ll use the CIFAR-10 dataset, which consists of 60,000 32×32 color images in 10 classes.
import tensorflow as tf
from tensorflow.keras.datasets import cifar10
from tensorflow.keras.utils import to_categorical
# Load and preprocess the dataset
(X_train, y_train), (X_test, y_test) = cifar10.load_data()
X_train, X_test = X_train / 255.0, X_test / 255.0
y_train, y_test = to_categorical(y_train), to_categorical(y_test)
Step 3: Building the CNN Model
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.summary()
Step 4: Training the Model
model.fit(X_train, y_train, epochs=10, validation_data=(X_test, y_test))
Step 5: Evaluating the Model
test_loss, test_acc = model.evaluate(X_test, y_test)
print(f'Test accuracy: {test_acc * 100:.2f}%')
This CNN model can classify images into one of the ten categories in the CIFAR-10 dataset. You can enhance it by adding more layers or using transfer learning with pre-trained models.
Sentiment Analysis with Natural Language Processing (NLP)
Sentiment analysis is widely used to determine the sentiment behind user reviews, social media posts, etc. Let’s build a sentiment analysis tool.
Step 1: Installing Required Libraries
pip install nltk
pip install scikit-learn
Step 2: Preparing the Data
We’ll use the IMDb movie reviews dataset.
import nltk
from nltk.corpus import movie_reviews
import random
nltk.download('movie_reviews')
documents = [(list(movie_reviews.words(fileid)), category)
for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
random.shuffle(documents)
Step 3: Feature Extraction
We need to extract features from the text data.
all_words = nltk.FreqDist(w.lower() for w in movie_reviews.words())
word_features = list(all_words)[:2000]
def document_features(document):
document_words = set(document)
features = {}
for word in word_features:
features[f'contains({word})'] = (word in document_words)
return features
featuresets = [(document_features(d), c) for (d, c) in documents]
train_set, test_set = featuresets[100:], featuresets[:100]
Step 4: Training the Model
We’ll use a Naive Bayes classifier.
classifier = nltk.NaiveBayesClassifier.train(train_set)
# Evaluate the classifier
print(nltk.classify.accuracy(classifier, test_set))
# Show the most informative features
classifier.show_most_informative_features(5)
This sentiment analysis tool can classify movie reviews as positive or negative. You can extend it to other types of text data by retraining the model with relevant datasets.
Creating a Recommendation System
Recommendation systems are used by platforms like Amazon and Netflix to suggest products or content to users. Let’s build a simple recommendation system.
Step 1: Data Collection
We’ll use the MovieLens dataset, which contains millions of movie ratings.
Step 2: Installing Required Libraries
pip install pandas
pip install scikit-learn
Step 3: Loading and Preparing the Data
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.metrics.pairwise import cosine_similarity
# Load the dataset
movies = pd.read_csv('movies.csv')
ratings = pd.read_csv('ratings.csv')
# Merge datasets
data = pd.merge(ratings, movies, on='movieId')
# Create a pivot table
pivot_table = data.pivot_table(index='userId', columns='title', values='rating')
# Fill missing values with 0
pivot_table.fillna(0, inplace=True)
Step 4: Calculating Similarity
We’ll use cosine similarity to calculate the similarity between movies.
# Calculate cosine similarity
cosine_sim = cosine_similarity(pivot_table.T)
cosine_sim_df = pd.DataFrame(cosine_sim, index=pivot_table.columns, columns=pivot_table.columns)
Step 5: Making Recommendations
def get_recommendations(movie_title, similarity_df, n=5):
sim_scores = similarity_df[movie_title]
sim_scores = sim_scores.sort_values(ascending=False)
top_movies = sim_scores.iloc[1:n+1].index
return top_movies
# Get recommendations
print(get_recommendations('Toy Story (1995)', cosine_sim_df))
This simple recommendation system can suggest similar movies based on user
ratings. You can refine this system by incorporating more sophisticated algorithms like collaborative filtering or matrix factorization for better accuracy and scalability.
Building a Voice Assistant
Voice assistants like Siri and Alexa are becoming increasingly popular. Let’s create a basic voice assistant using Python.
Step 1: Installing Required Libraries
pip install speechrecognition
pip install pyttsx3
pip install pyaudio
Step 2: Setting Up the Voice Assistant
We’ll use the speech_recognition
library for speech recognition and pyttsx3
for text-to-speech conversion.
import speech_recognition as sr
import pyttsx3
# Initialize recognizer and engine
recognizer = sr.Recognizer()
engine = pyttsx3.init()
def speak(text):
engine.say(text)
engine.runAndWait()
def listen():
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
command = recognizer.recognize_google(audio)
print(f'You said: {command}')
return command.lower()
except sr.UnknownValueError:
print("Sorry, I did not understand that.")
return "None"
except sr.RequestError:
print("Could not request results; check your network connection.")
return "None"
Step 3: Adding Functionality
We’ll add a few basic functionalities to our voice assistant, like telling the time and opening a website.
import datetime
import webbrowser
def get_time():
now = datetime.datetime.now()
return now.strftime("%H:%M:%S")
def open_website(url):
webbrowser.open(url)
while True:
command = listen()
if 'time' in command:
current_time = get_time()
speak(f'The current time is {current_time}')
elif 'open google' in command:
speak('Opening Google')
open_website('https://www.google.com')
elif 'stop' in command:
speak('Goodbye!')
break
This basic voice assistant can be enhanced with more functionalities, such as controlling smart home devices or retrieving information from the web.
Creating a Face Recognition System
Face recognition technology is used in security systems, social media platforms, and more. Let’s build a face recognition system using Python.
Step 1: Installing Required Libraries
pip install face_recognition
pip install opencv-python
Step 2: Loading and Encoding Faces
import face_recognition
import cv2
# Load a sample picture and learn how to recognize it.
image_of_person = face_recognition.load_image_file('person.jpg')
face_encoding = face_recognition.face_encodings(image_of_person)[0]
known_face_encodings = [face_encoding]
known_face_names = ["Person Name"]
Step 3: Recognizing Faces in Real-Time
video_capture = cv2.VideoCapture(0)
while True:
ret, frame = video_capture.read()
rgb_frame = frame[:, :, ::-1]
face_locations = face_recognition.face_locations(rgb_frame)
face_encodings = face_recognition.face_encodings(rgb_frame, face_locations)
for (top, right, bottom, left), face_encoding in zip(face_locations, face_encodings):
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
name = "Unknown"
if True in matches:
first_match_index = matches.index(True)
name = known_face_names[first_match_index]
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
cv2.putText(frame, name, (left + 6, bottom - 6), cv2.FONT_HERSHEY_DUPLEX, 1.0, (255, 255, 255), 1)
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
video_capture.release()
cv2.destroyAllWindows()
This face recognition system can identify known faces in real-time video streams. You can extend it to recognize multiple people by adding more known faces and encodings.
Conclusion
Python is a versatile and powerful language that makes it easy to dive into the world of AI. The projects we covered—spam classifiers, chatbots, image recognition, sentiment analysis, recommendation systems, voice assistants, and face recognition systems—showcase the breadth of what you can achieve with Python in AI. Each project provides a foundation that you can build upon, adding complexity and features as you grow more comfortable with the tools and techniques.
By starting with these projects, you’ll gain practical experience that can be applied to real-world problems, making you more valuable in today’s tech-driven world. So, pick a project, start coding, and let your Python passion drive your AI journey.
Happy coding!
Disclaimer: The examples and projects discussed in this blog are for educational purposes. Ensure to respect privacy and data protection laws when working on real-world AI applications. Report any inaccuracies so we can correct them promptly.