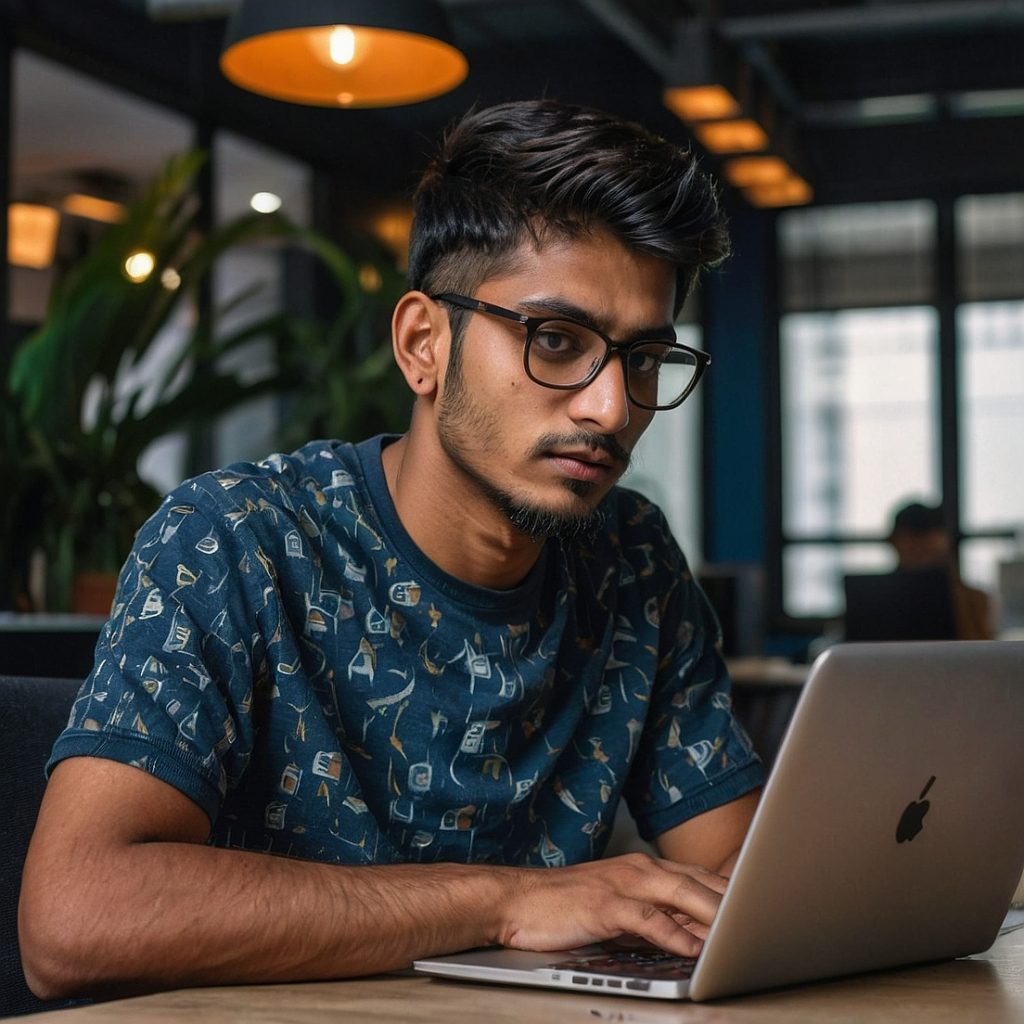
Python vs. the World: When to Choose the Snake for Success
Python, the ubiquitous and versatile programming language named after the British comedy series “Monty Python,” has slithered its way into the hearts of developers and tech enthusiasts worldwide. But how does it stack up against other programming languages? When should you choose Python over alternatives? Let’s dive into the world of programming languages, examining Python’s strengths and when it’s the right tool for the job.
The Swiss Army Knife of Programming Languages
Python is often lauded as the Swiss Army knife of programming languages. Its design philosophy emphasizes code readability and simplicity, making it an excellent choice for both beginners and experienced developers.
Readable and Maintainable Code
Python’s syntax is clean and easy to understand. Compare a simple “Hello, World!” program in Python with other languages:
Python:
print("Hello, World!")
Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
The Python example is concise and straightforward, which is a significant advantage when writing and maintaining code.
Extensive Standard Library
Python’s standard library is extensive, providing modules and packages for a wide range of tasks. This built-in functionality allows developers to accomplish more with less code. For instance, let’s look at reading a CSV file.
Python:
import csv
with open('data.csv', newline='') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
print(row['name'], row['age'])
Java:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ReadCSV {
public static void main(String[] args) {
String csvFile = "data.csv";
String line;
String csvSplitBy = ",";
try (BufferedReader br = new BufferedReader(new FileReader(csvFile))) {
while ((line = br.readLine()) != null) {
String[] data = line.split(csvSplitBy);
System.out.println("Name: " + data[0] + " , Age: " + data[1]);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Python’s code is more concise and readable, showcasing the power of its standard library.
Web Development Wonders
Python is a powerhouse in web development, thanks to frameworks like Django and Flask. These frameworks simplify the process of building robust web applications.
Django: The Full-Stack Framework
Django is a high-level web framework that encourages rapid development and clean, pragmatic design. It comes with an ORM, admin panel, and several built-in features that make web development a breeze.
Creating a Django Project:
pip install django
django-admin startproject mysite
cd mysite
python manage.py runserver
Simple Django View:
from django.http import HttpResponse
def hello_world(request):
return HttpResponse("Hello, World!")
Flask: The Micro Framework
Flask is a micro web framework that’s lightweight and easy to use. It’s perfect for small to medium-sized applications and provides the flexibility to choose components as needed.
Creating a Flask App:
pip install flask
Simple Flask App:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
Data Science Domination
Python has become the go-to language for data science, machine learning, and artificial intelligence. Libraries like NumPy, pandas, and scikit-learn make data manipulation and analysis straightforward.
NumPy for Numerical Computing
NumPy is the foundational package for numerical computing in Python. It provides support for arrays, matrices, and many mathematical functions.
Creating and Manipulating Arrays:
import numpy as np
# Create an array
array = np.array([1, 2, 3, 4, 5])
# Perform arithmetic operations
array = array + 10
print(array)
pandas for Data Manipulation
pandas is a powerful data manipulation library that provides data structures like DataFrames, making it easier to work with structured data.
Reading and Analyzing Data:
import pandas as pd
# Read a CSV file into a DataFrame
df = pd.read_csv('data.csv')
# Display basic statistics
print(df.describe())
# Filter data
filtered_df = df[df['age'] > 30]
print(filtered_df)
scikit-learn for Machine Learning
scikit-learn is a comprehensive library for machine learning. It includes tools for classification, regression, clustering, and more.
Building a Simple Model:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Load dataset
data = pd.read_csv('data.csv')
# Split data into features and target variable
X = data[['feature1', 'feature2']]
y = data['target']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, predictions)
print(f'Mean Squared Error: {mse}')
Scripting and Automation
Python’s simplicity and readability make it an ideal choice for scripting and automation tasks. Whether it’s automating repetitive tasks or writing small scripts, Python gets the job done efficiently.
Automating a Task
Imagine you need to rename multiple files in a directory. Python can handle this with ease:
Renaming Files:
import os
# Define the directory and the new name format
directory = '/path/to/files'
new_name = 'file'
# Iterate over the files and rename them
for count, filename in enumerate(os.listdir(directory)):
new_filename = f"{new_name}_{count}.txt"
os.rename(os.path.join(directory, filename), os.path.join(directory, new_filename))
Scientific Computing and Visualization
Python excels in scientific computing and visualization, thanks to libraries like SciPy, Matplotlib, and Seaborn.
SciPy for Scientific Computing
SciPy builds on NumPy and provides additional functionality for scientific computing, including optimization, integration, and signal processing.
Optimization Example:
from scipy.optimize import minimize
# Define a simple quadratic function
def func(x):
return x**2 + 5*x + 4
# Minimize the function
result = minimize(func, x0=0)
print(result)
Matplotlib and Seaborn for Visualization
Matplotlib is a plotting library that produces high-quality graphs, while Seaborn builds on Matplotlib to provide a high-level interface for drawing attractive statistical graphics.
Plotting with Matplotlib:
import matplotlib.pyplot as plt
import numpy as np
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Plot the data
plt.plot(x, y)
plt.title('Sine Wave')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
Plotting with Seaborn:
import seaborn as sns
import pandas as pd
# Load dataset
df = sns.load_dataset('iris')
# Create a scatter plot
sns.scatterplot(data=df, x='sepal_length', y='sepal_width', hue='species')
plt.title('Iris Dataset')
plt.show()
When Not to Choose Python
Despite its versatility, Python isn’t always the best choice. There are scenarios where other languages might be more suitable.
Performance-Intensive Applications
Python is an interpreted language, which means it can be slower than compiled languages like C or C++. For performance-critical applications, these languages might be a better choice.
Example: C++ for Performance
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
for (int i = 0; i < 1000000; ++i) {
vec.push_back(i);
}
std::cout << "Done!" << std::endl;
return 0;
}
Mobile Development
While Python has frameworks like Kivy for mobile development, it’s not the dominant player. Languages like Swift for iOS and Kotlin for Android are more commonly used.
Example: Swift for iOS
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
print("Hello, World!")
}
}
Conclusion
Python is a versatile, powerful, and easy-to-learn language that excels in various domains, from web development and data science to automation and scientific computing. Its readability and extensive standard library make it a favorite among developers. However, it’s essential to recognize its limitations and choose the right tool for the job. By understanding when to choose Python, you’ll be well-equipped to harness its power for success in your projects.
In the end, whether you’re building a web application, analyzing data, or automating tasks, Python’s got your back. So, embrace the snake and let it guide you to programming success!