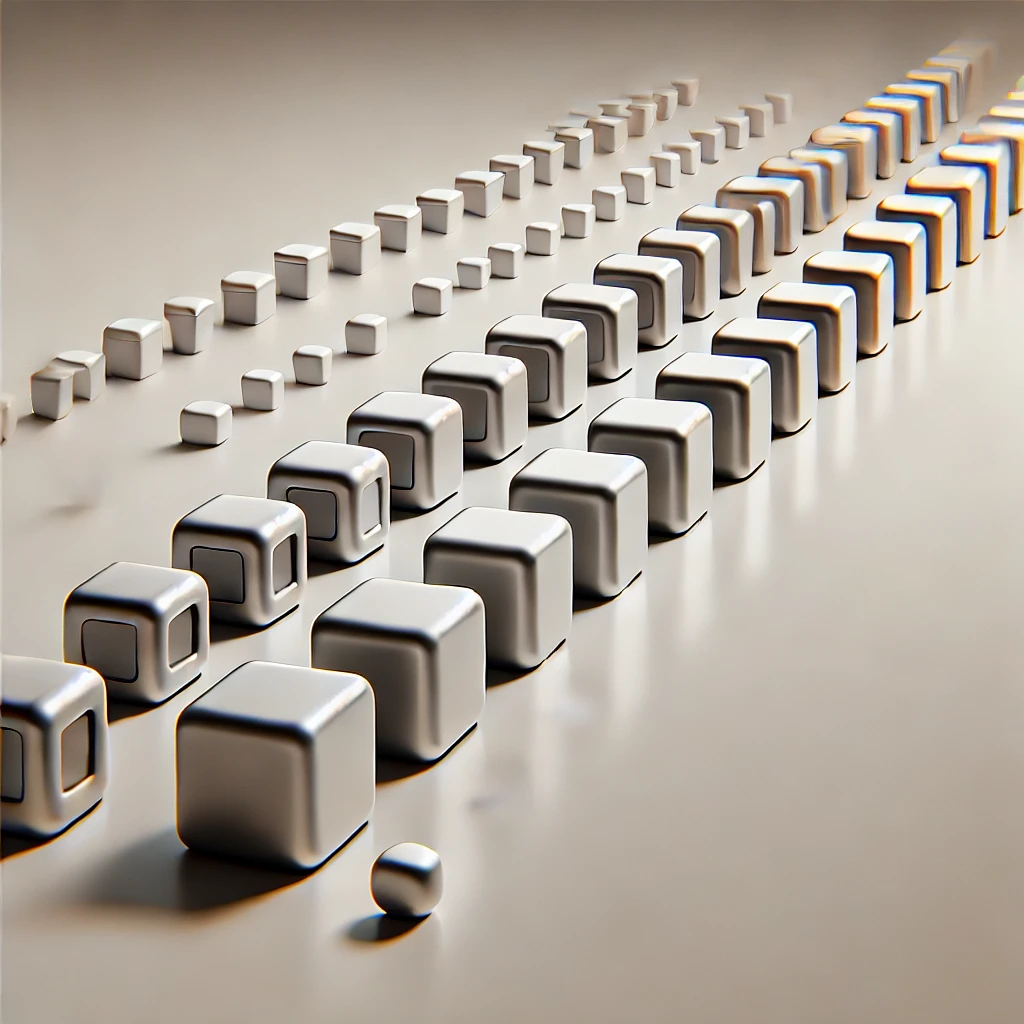
Queues Demystified: FIFO in Java
Have you ever found yourself scratching your head over data structures in Java? You’re not alone. Among the myriad of data structures, queues stand out as both simple and powerful. But what exactly are queues, and why should you care about them? Well, buckle up, because we’re about to embark on a journey through the fascinating world of queues in Java. We’ll explore their inner workings, uncover their practical applications, and even dive into some code to see them in action. By the end of this post, you’ll not only understand queues but also appreciate their elegance in solving real-world problems. So, whether you’re a coding newbie or a seasoned developer looking to refresh your knowledge, this guide is for you. Let’s jump in and demystify queues, shall we?
What is a Queue? Understanding the Basics
The Essence of FIFO
At its core, a queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. Imagine you’re standing in line at your favorite coffee shop. The first person who joined the line gets served first, right? That’s exactly how a queue works in programming. The first element added to the queue is the first one to be removed. This simple yet powerful concept forms the foundation of many algorithms and real-world applications. From managing print jobs to handling requests in web servers, queues are everywhere in the world of computing.
Queue Operations: The Building Blocks
Now that we’ve got the basic idea, let’s break down the fundamental operations of a queue. There are primarily two key operations: enqueue and dequeue. Enqueue is the process of adding an element to the rear of the queue, while dequeue removes an element from the front. Think of it as people joining the back of a line and leaving from the front. These operations maintain the FIFO order, ensuring that elements are processed in the sequence they were added. Additionally, queues often support operations like peek (to view the front element without removing it) and isEmpty (to check if the queue is empty). Understanding these operations is crucial as they form the basis of how we interact with queues in Java.
Queues in Java: The Interface and Implementations
The Queue Interface: A Blueprint for Order
In Java, the Queue interface is part of the Java Collections Framework, providing a contract for queue implementations. It extends the Collection interface, inheriting methods for basic collection operations. The Queue interface defines methods specific to queue behavior, such as offer(), poll(), and peek(). These methods allow for more flexible queue operations, handling scenarios like queue capacity limits or empty queues gracefully. By using the Queue interface, you’re setting up your code to work with any queue implementation, promoting flexibility and ease of maintenance.
Queue Implementations: Choosing Your Weapon
Java offers several implementations of the Queue interface, each with its own characteristics and use cases. Let’s explore some of the most common ones:
- LinkedList: A doubly-linked list implementation of the Queue interface. It’s unbounded and allows for null elements.
- ArrayDeque: A resizable-array implementation that’s more efficient than LinkedList for most operations.
- PriorityQueue: An implementation based on a priority heap, where elements are ordered according to their natural order or a specified comparator.
- BlockingQueue: An interface for thread-safe operations, with implementations like LinkedBlockingQueue and ArrayBlockingQueue.
Each of these implementations has its strengths and ideal use cases. For instance, LinkedList is great for frequent add and remove operations, while ArrayDeque offers better performance for most scenarios. PriorityQueue is perfect when you need elements to be processed based on priority, and BlockingQueue implementations are essential for concurrent programming.
Implementing a Basic Queue in Java
Creating a Simple Queue: Step by Step
Let’s roll up our sleeves and implement a basic queue in Java. We’ll use the LinkedList class, which provides a convenient implementation of the Queue interface. Here’s a step-by-step guide:
import java.util.LinkedList;
import java.util.Queue;
public class BasicQueueExample {
public static void main(String[] args) {
// Create a queue
Queue<String> queue = new LinkedList<>();
// Add elements to the queue (enqueue)
queue.offer("Alice");
queue.offer("Bob");
queue.offer("Charlie");
System.out.println("Queue: " + queue);
// Remove and print the front element (dequeue)
String frontElement = queue.poll();
System.out.println("Removed element: " + frontElement);
// Peek at the front element without removing it
String peekedElement = queue.peek();
System.out.println("Front element (after peek): " + peekedElement);
System.out.println("Queue after operations: " + queue);
}
}
In this example, we create a queue of strings, add elements to it, remove the front element, and peek at the new front element. This demonstrates the basic operations of a queue in action. Running this code will give you a clear picture of how elements flow through a queue.
Understanding the Output
When you run this code, you’ll see the queue’s contents at different stages, illustrating how elements are added and removed. The FIFO principle becomes evident as “Alice” (the first element added) is the first to be removed. This simple example serves as a foundation for understanding more complex queue implementations and use cases.
Advanced Queue Operations: Beyond the Basics
Queue Methods: A Deeper Dive
While enqueue and dequeue are the core operations, Java’s Queue interface offers additional methods that provide more control and flexibility. Let’s explore some of these methods:
- add(E e): Inserts an element, throwing an exception if the queue is full.
- remove(): Retrieves and removes the head, throwing an exception if the queue is empty.
- element(): Retrieves, but does not remove, the head, throwing an exception if the queue is empty.
- offer(E e): Inserts an element if possible, returning false if the queue is full.
- poll(): Retrieves and removes the head, returning null if the queue is empty.
- peek(): Retrieves, but does not remove, the head, returning null if the queue is empty.
Understanding when to use each method is crucial for effective queue management. For instance, offer(), poll(), and peek() are often preferred in scenarios where queue capacity or emptiness needs to be handled gracefully without throwing exceptions.
Implementing a Circular Queue
A circular queue, also known as a ring buffer, is an advanced queue implementation where the rear of the queue wraps around to the front when it reaches the end of the underlying array. This allows for efficient space utilization. Let’s implement a basic circular queue:
public class CircularQueue<E> {
private E[] elements;
private int front;
private int rear;
private int size;
private int capacity;
@SuppressWarnings("unchecked")
public CircularQueue(int capacity) {
this.capacity = capacity;
elements = (E[]) new Object[capacity];
front = 0;
rear = -1;
size = 0;
}
public boolean offer(E element) {
if (isFull()) {
return false;
}
rear = (rear + 1) % capacity;
elements[rear] = element;
size++;
return true;
}
public E poll() {
if (isEmpty()) {
return null;
}
E element = elements[front];
elements[front] = null;
front = (front + 1) % capacity;
size--;
return element;
}
public E peek() {
if (isEmpty()) {
return null;
}
return elements[front];
}
public boolean isEmpty() {
return size == 0;
}
public boolean isFull() {
return size == capacity;
}
public int size() {
return size;
}
}
This circular queue implementation uses an array to store elements and wraps around when it reaches the end, providing efficient space usage. It’s particularly useful in scenarios with a fixed buffer size, such as in embedded systems or when implementing a producer-consumer pattern.
Practical Applications: Queues in the Real World
Breadth-First Search: Exploring Graphs
One of the most common applications of queues is in breadth-first search (BFS) algorithms. BFS is used to traverse or search tree or graph data structures, exploring all the neighboring nodes at the present depth before moving on to nodes at the next depth level. Here’s a simple implementation of BFS using a queue:
import java.util.*;
public class BreadthFirstSearch {
private int V; // Number of vertices
private LinkedList<Integer>[] adj; // Adjacency Lists
@SuppressWarnings("unchecked")
BreadthFirstSearch(int v) {
V = v;
adj = new LinkedList[v];
for (int i = 0; i < v; ++i)
adj[i] = new LinkedList<>();
}
void addEdge(int v, int w) {
adj[v].add(w);
}
void BFS(int s) {
boolean visited[] = new boolean[V];
Queue<Integer> queue = new LinkedList<>();
visited[s] = true;
queue.offer(s);
while (!queue.isEmpty()) {
s = queue.poll();
System.out.print(s + " ");
for (int n : adj[s]) {
if (!visited[n]) {
visited[n] = true;
queue.offer(n);
}
}
}
}
public static void main(String args[]) {
BreadthFirstSearch g = new BreadthFirstSearch(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
System.out.println("Breadth First Traversal (starting from vertex 2):");
g.BFS(2);
}
}
This BFS implementation demonstrates how queues can be used to systematically explore graph structures, which is fundamental in many algorithms and applications, from social network analysis to pathfinding in GPS systems.
Task Scheduling: Managing Priorities
Another practical application of queues is in task scheduling systems. PriorityQueue in Java is particularly useful for this purpose, as it allows tasks to be processed based on their priority. Here’s a simple task scheduler using PriorityQueue:
import java.util.PriorityQueue;
class Task implements Comparable<Task> {
private String name;
private int priority;
public Task(String name, int priority) {
this.name = name;
this.priority = priority;
}
@Override
public int compareTo(Task other) {
return Integer.compare(other.priority, this.priority); // Higher priority first
}
@Override
public String toString() {
return name + " (Priority: " + priority + ")";
}
}
public class TaskScheduler {
public static void main(String[] args) {
PriorityQueue<Task> taskQueue = new PriorityQueue<>();
taskQueue.offer(new Task("Write report", 3));
taskQueue.offer(new Task("Fix critical bug", 1));
taskQueue.offer(new Task("Attend meeting", 2));
taskQueue.offer(new Task("Refactor code", 4));
System.out.println("Tasks will be processed in this order:");
while (!taskQueue.isEmpty()) {
System.out.println(taskQueue.poll());
}
}
}
This task scheduler demonstrates how PriorityQueue can be used to manage tasks based on their priority, ensuring that high-priority tasks are processed first. This concept is widely used in operating systems, job scheduling in distributed systems, and many other applications where task prioritization is crucial.
Performance Considerations: Choosing the Right Queue
Time Complexity: The Need for Speed
When working with queues, understanding the time complexity of different operations is crucial for optimizing performance. Here’s a quick rundown of the time complexities for common queue operations in different implementations:
- LinkedList:
- Offer (enqueue): O(1)
- Poll (dequeue): O(1)
- Peek: O(1)
- ArrayDeque:
- Offer (enqueue): O(1) amortized
- Poll (dequeue): O(1)
- Peek: O(1)
- PriorityQueue:
- Offer (enqueue): O(log n)
- Poll (dequeue): O(log n)
- Peek: O(1)
As you can see, LinkedList and ArrayDeque offer constant-time performance for basic operations, making them suitable for most general-purpose queue applications. PriorityQueue, while slightly slower for enqueue and dequeue operations, provides the added benefit of priority-based ordering.
Space Efficiency: Memory Matters
Space efficiency is another important factor to consider when choosing a queue implementation. LinkedList, while offering good time complexity, can be less space-efficient due to the overhead of storing node references. ArrayDeque, on the other hand, provides a good balance of time and space efficiency, making it a popular choice for many applications. When dealing with a large number of elements or in memory-constrained environments, choosing the right implementation can make a significant difference in your application’s performance and resource usage.
Concurrent Queues: Threading the Needle
Thread-Safe Queues: Ensuring Consistency
In multi-threaded applications, using thread-safe queue implementations is crucial to maintain data consistency and prevent race conditions. Java provides several concurrent queue implementations in the java.util.concurrent package. Let’s explore one of the most commonly used concurrent queues: BlockingQueue.
BlockingQueue is an interface that represents a queue which is thread-safe to put into and take from. It provides additional operations that wait for the queue to become non-empty when retrieving an element, and wait for space to become available in the queue when storing an element. Here’s an example using LinkedBlockingQueue:
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.TimeUnit;
public class ConcurrentQueueExample {
public static void main(String[] args) {
BlockingQueue<String> queue = new LinkedBlockingQueue<>(5); // Capacity of 5
// Producer thread
new Thread(() -> {
try {
String[] messages = {"Hello", "World", "Concurrent", "Queue", "Example", "Overflow"};
for (String msg : messages) {
queue.offer(msg, 1, TimeUnit.SECONDS); // Wait up to 1 second to insert
System.out.println("Produced: " + msg);
Thread.sleep(100); // Simulate some work
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
// Consumer thread
new Thread(() -> {
try {
while (true) {
String msg = queue.poll(1, TimeUnit.SECONDS); // Wait up to 1 second to retrieve
if (msg == null) break; // Exit if no more messages
System.out.println("Consumed: " + msg);
Thread.sleep(200); // Simulate some work
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
}
}
This example demonstrates a producer-consumer scenario using LinkedBlockingQueue. The producer thread adds messages to the queue, while the consumer thread retrieves and processes them. The BlockingQueue ensures thread-safe operations, handling synchronization internally and providing methods like offer(E e, long timeout, TimeUnit unit) and poll(long timeout, TimeUnit unit) for timed operations.
Choosing the Right Concurrent Queue
Java offers several implementations of BlockingQueue, each with its own characteristics:
- LinkedBlockingQueue: An optionally-bounded queue based on linked nodes.
- ArrayBlockingQueue: A bounded queue backed by an array.
- PriorityBlockingQueue: An unbounded priority queue.
- DelayQueue: A queue of elements that become available for consumption only after a delay.
Choosing the right concurrent queue depends on your specific requirements. For example, if you need a bounded queue with fairness guarantees, ArrayBlockingQueue might be the best choice. If you need an unbounded queue with priority ordering, PriorityBlockingQueue would be more appropriate.
Best Practices and Common Pitfalls
Queue Design Patterns: Structuring for Success
When working with queues, several design patterns can help you structure your code more effectively:
- Producer-Consumer Pattern: This pattern decouples the production of data from its consumption, often using a queue as the intermediate storage. We saw an example of this in our concurrent queue section.
- Command Queue Pattern: Used to queue up commands or tasks to be executed sequentially or in parallel. This is particularly useful in GUI applications or game development.
- Work Queue Pattern: Similar to the producer-consumer pattern, but typically used in scenarios where multiple workers process tasks from a shared queue.
- Priority Queue Pattern: Used when tasks or elements need to be processed based on their priority rather than their order of arrival.
Implementing these patterns can lead to more modular, maintainable, and scalable code. For instance, here’s a simple implementation of the Command Queue Pattern:
import java.util.LinkedList;
import java.util.Queue;
interface Command {
void execute();
}
class CommandQueue {
private Queue<Command> commandQueue = new LinkedList<>();
public void addCommand(Command command) {
commandQueue.offer(command);
}
public void processCommands() {
while (!commandQueue.isEmpty()) {
Command command = commandQueue.poll();
command.execute();
}
}
}
// Usage example
public class CommandQueueExample {
public static void main(String[] args) {
CommandQueue queue = new CommandQueue();
queue.addCommand(() -> System.out.println("First command"));
queue.addCommand(() -> System.out.println("Second command"));
queue.addCommand(() -> System.out.println("Third command"));
queue.processCommands();
}
}
This pattern allows you to queue up commands and execute them later, which can be particularly useful in scenarios where you want to batch operations or implement undo/redo functionality.
Common Pitfalls: Avoiding Queue Quagmires
While queues are powerful tools, there are some common pitfalls to watch out for:
- Ignoring Queue Capacity: When using bounded queues, always consider what happens when the queue reaches its capacity. Implement appropriate error handling or blocking behavior.
- Neglecting Thread Safety: In multi-threaded environments, using non-thread-safe queue implementations can lead to data races and inconsistencies. Always use concurrent queue implementations when working with multiple threads.
- Inefficient Iteration: Avoid using iterator() or enhanced for-loop to process queue elements if you intend to remove them. Instead, use poll() or remove() to efficiently dequeue elements.
- Memory Leaks: Be cautious when using queues with object references. Ensure that objects are properly dequeued and dereferenced when no longer needed to prevent memory leaks.
- Blocking Queue Deadlocks: When using blocking queues, be aware of potential deadlock scenarios where producers are waiting for space and consumers are waiting for elements.
Here’s an example demonstrating proper handling of a bounded queue:
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.TimeUnit;
public class BoundedQueueExample {
public static void main(String[] args) {
BlockingQueue<String> boundedQueue = new ArrayBlockingQueue<>(3);
try {
// Adding elements
boundedQueue.offer("First", 1, TimeUnit.SECONDS);
boundedQueue.offer("Second", 1, TimeUnit.SECONDS);
boundedQueue.offer("Third", 1, TimeUnit.SECONDS);
// This will fail as the queue is full
boolean added = boundedQueue.offer("Fourth", 1, TimeUnit.SECONDS);
if (!added) {
System.out.println("Failed to add: queue is full");
}
// Removing elements
System.out.println(boundedQueue.poll(1, TimeUnit.SECONDS));
System.out.println(boundedQueue.poll(1, TimeUnit.SECONDS));
System.out.println(boundedQueue.poll(1, TimeUnit.SECONDS));
// This will return null as the queue is empty
String element = boundedQueue.poll(1, TimeUnit.SECONDS);
if (element == null) {
System.out.println("No more elements in the queue");
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
System.out.println("Operation interrupted");
}
}
}
This example demonstrates proper handling of both queue capacity limits and empty queue scenarios, using timed operations to avoid indefinite blocking.
Conclusion
As we wrap up our deep dive into queues in Java, it’s clear that these data structures are far more than simple lists. They’re powerful tools that, when used correctly, can significantly enhance the efficiency and elegance of your code. From managing task priorities to facilitating concurrent operations, queues offer solutions to a wide array of programming challenges.
We’ve explored the basic concepts of FIFO queues, delved into various implementations provided by Java, and even implemented our own circular queue. We’ve seen how queues play crucial roles in algorithms like breadth-first search and in real-world applications like task scheduling. Moreover, we’ve discussed the importance of choosing the right queue implementation based on performance considerations and thread-safety requirements.
Remember, the key to mastering queues lies not just in understanding their mechanics, but in recognizing when and how to apply them effectively in your projects. As you continue your coding journey, keep an eye out for problems that exhibit FIFO behavior or require ordered processing – chances are, a queue might be the perfect solution.
So, the next time you find yourself tackling a complex data flow problem or designing a concurrent system, don’t forget to consider the humble yet powerful queue. It might just be the key to queuing up your next big success in software development.
Happy coding, and may your queues always be perfectly balanced!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information and code examples presented in this blog post, they are provided “as is” without warranty of any kind. The author and the website do not guarantee the accuracy, completeness, or suitability of the content for any particular purpose. Users should use this information at their own risk and are encouraged to verify and test any code in their own development environments. If you notice any inaccuracies or have suggestions for improvements, please report them so we can correct them promptly.