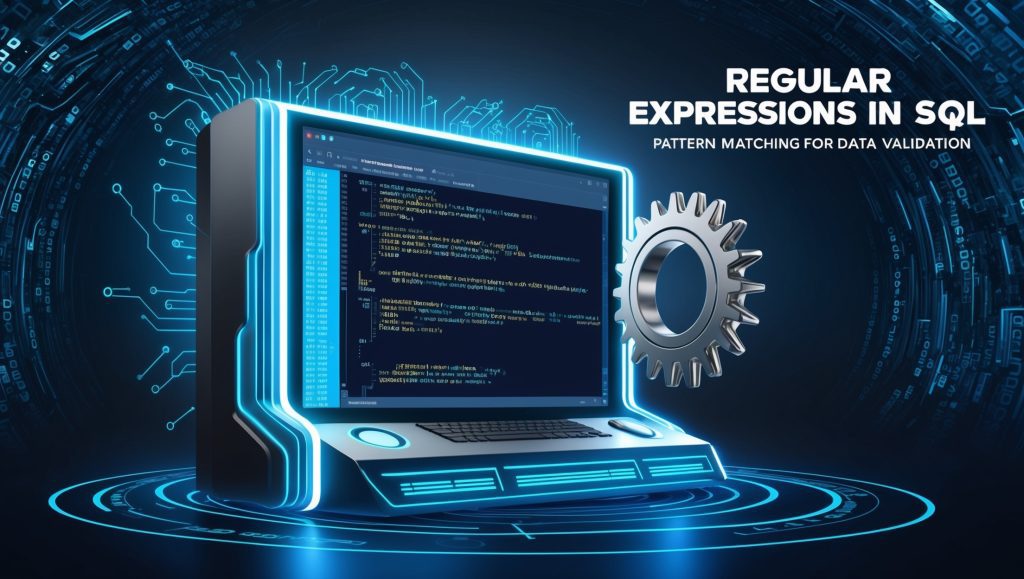
Regular Expressions in SQL: Pattern Matching for Data Validation
Regular expressions (regex) have become an indispensable tool in the modern data professional’s arsenal, offering powerful pattern matching and validation capabilities that extend far beyond simple string operations. In the context of SQL, regular expressions provide a sophisticated mechanism for filtering, validating, and manipulating string data with precision and flexibility. This comprehensive guide explores the implementation of regex in SQL, focusing on practical applications for data validation and advanced filtering techniques. Understanding regex in SQL is crucial for database administrators, data analysts, and developers who work with large datasets containing string patterns that require complex matching and validation rules. The knowledge of regex can significantly enhance query performance, improve data quality, and streamline data cleaning processes.
Understanding Regular Expressions in SQL
Regular expressions in SQL serve as a powerful pattern-matching language that allows users to search, validate, and manipulate text data using sophisticated pattern definitions. Unlike simple wildcards or LIKE operators, regex provides a more granular and flexible approach to pattern matching. The implementation of regex varies across different SQL database management systems (DBMS), with PostgreSQL, Oracle, and MySQL each offering their own syntax and function sets. The fundamental concept remains consistent across platforms: defining patterns using special characters and metacharacters to match specific text patterns within your data. Regular expressions can be particularly useful when dealing with semi-structured data, validating email addresses, phone numbers, or any other text that follows specific patterns. They can also be instrumental in data cleaning operations, helping to identify and filter out invalid or malformed data entries.
Regular Expression Support Across Different SQL Platforms
Different SQL database systems implement regex functionality in unique ways. Here’s a comprehensive overview of regex support across major SQL platforms:
PostgreSQL
-- Using SIMILAR TO
SELECT column_name
FROM table_name
WHERE column_name SIMILAR TO '%(pattern)%';
-- Using ~ operator (POSIX regex)
SELECT column_name
FROM table_name
WHERE column_name ~ 'pattern';
Oracle
-- Using REGEXP_LIKE
SELECT column_name
FROM table_name
WHERE REGEXP_LIKE(column_name, 'pattern');
-- Using REGEXP_SUBSTR
SELECT REGEXP_SUBSTR(column_name, 'pattern')
FROM table_name;
MySQL
-- Using REGEXP or RLIKE
SELECT column_name
FROM table_name
WHERE column_name REGEXP 'pattern';
Common Regex Patterns and Their Applications
Regular expressions employ various special characters and metacharacters to define patterns. Here’s a detailed breakdown of commonly used patterns:
Basic Pattern Matching
Pattern | Description | Example |
---|---|---|
——— | ————- | ——— |
^ | Start of string | ‘^ABC’ matches strings starting with ‘ABC’ |
$ | End of string | ‘XYZ$’ matches strings ending with ‘XYZ’ |
. | Any single character | ‘A.C’ matches ‘ABC’, ‘ADC’, etc. |
* | Zero or more occurrences | ‘AB*C’ matches ‘AC’, ‘ABC’, ‘ABBC’, etc. |
+ | One or more occurrences | ‘AB+C’ matches ‘ABC’, ‘ABBC’, but not ‘AC’ |
? | Zero or one occurrence | ‘AB?C’ matches ‘AC’ and ‘ABC’ |
[] | Character class | ‘[0-9]’ matches any digit |
[^] | Negated character class | ‘[^0-9]’ matches any non-digit |
Practical Examples for Data Validation
Let’s explore practical examples of using regex for common data validation scenarios:
Email Validation
-- PostgreSQL
SELECT email
FROM users
WHERE email ~ '^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$';
-- Oracle
SELECT email
FROM users
WHERE REGEXP_LIKE(email, '^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$');
-- MySQL
SELECT email
FROM users
WHERE email REGEXP '^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$';
Phone Number Validation
-- PostgreSQL (US phone number format)
SELECT phone_number
FROM contacts
WHERE phone_number ~ '^\+?1?\s*\(?([0-9]{3})\)?[-.\s]?([0-9]{3})[-.\s]?([0-9]{4})$';
-- Oracle
SELECT phone_number
FROM contacts
WHERE REGEXP_LIKE(phone_number, '^\+?1?\s*\(?([0-9]{3})\)?[-.\s]?([0-9]{3})[-.\s]?([0-9]{4})$');
Advanced Filtering Techniques
Regular expressions enable sophisticated filtering operations that go beyond simple pattern matching. Here are some advanced filtering techniques:
Complex String Patterns
-- Finding strings with specific patterns
SELECT product_code
FROM products
WHERE product_code ~ '^[A-Z]{2}\d{4}-[A-Z]\d{2}$'; -- Matches format: AA1234-B12
-- Extracting specific patterns
SELECT REGEXP_MATCHES(description, '(\d+\.?\d*)\s*(kg|lbs)', 'g')
FROM product_descriptions;
Multiple Pattern Matching
-- PostgreSQL
SELECT column_name
FROM table_name
WHERE column_name ~ 'pattern1|pattern2|pattern3';
-- Oracle
SELECT column_name
FROM table_name
WHERE REGEXP_LIKE(column_name, 'pattern1|pattern2|pattern3');
Data Cleaning with Regular Expressions
Regular expressions are particularly useful for data cleaning operations. Here are some practical examples:
Removing Special Characters
-- PostgreSQL
UPDATE table_name
SET column_name = REGEXP_REPLACE(column_name, '[^a-zA-Z0-9\s]', '', 'g');
-- Oracle
UPDATE table_name
SET column_name = REGEXP_REPLACE(column_name, '[^a-zA-Z0-9\s]', '');
Standardizing Formats
-- Standardizing phone numbers
UPDATE contacts
SET phone_number = REGEXP_REPLACE(
phone_number,
'^\+?1?\s*\(?([0-9]{3})\)?[-.\s]?([0-9]{3})[-.\s]?([0-9]{4})$',
'($1) $2-$3'
);
Performance Considerations
When implementing regex in SQL queries, consider these performance optimization strategies:
Indexing Considerations
- Regular expressions cannot utilize traditional B-tree indexes
- Consider using functional indexes for frequently used patterns
- Use appropriate index types based on your DBMS capabilities
-- Creating a functional index for pattern matching (PostgreSQL)
CREATE INDEX idx_pattern_match ON table_name (SUBSTRING(column_name FROM 'pattern'));
Query Optimization
-- Instead of
SELECT * FROM table_name WHERE column_name ~ 'complex_pattern';
-- Consider using
SELECT * FROM table_name
WHERE column_name LIKE 'simple_prefix%' -- Use index
AND column_name ~ 'complex_pattern'; -- Then apply regex
Best Practices and Common Pitfalls
When working with regular expressions in SQL, follow these best practices:
Pattern Testing
-- Create a test table for pattern validation
CREATE TABLE pattern_test (
test_string VARCHAR(100)
);
-- Insert test cases
INSERT INTO pattern_test VALUES
('valid_pattern_1'),
('invalid_pattern_1'),
('valid_pattern_2');
-- Test your pattern
SELECT test_string,
CASE WHEN test_string ~ 'your_pattern' THEN 'Match' ELSE 'No Match' END AS result
FROM pattern_test;
Error Handling
-- PostgreSQL
DO $$
BEGIN
-- Attempt pattern matching with error handling
BEGIN
PERFORM 'test_string' ~ 'invalid[pattern';
EXCEPTION
WHEN invalid_regular_expression THEN
RAISE NOTICE 'Invalid regular expression pattern';
END;
END $$;
Common Use Cases and Solutions
Here are some practical applications of regex in SQL for common business scenarios:
Data Validation Rules
-- Validate ZIP codes
CREATE OR REPLACE FUNCTION is_valid_zipcode(zip_code TEXT)
RETURNS BOOLEAN AS $$
BEGIN
RETURN zip_code ~ '^\d{5}(-\d{4})?$';
END;
$$ LANGUAGE plpgsql;
-- Validate URLs
CREATE OR REPLACE FUNCTION is_valid_url(url TEXT)
RETURNS BOOLEAN AS $$
BEGIN
RETURN url ~ '^(https?:\/\/)?([\da-z\.-]+)\.([a-z\.]{2,6})([\/\w \.-]*)*\/?$';
END;
$$ LANGUAGE plpgsql;
Data Extraction
-- Extract domain from email addresses
SELECT
email,
REGEXP_REPLACE(email, '^.*@', '') as domain
FROM users;
-- Extract numbers from text
SELECT
description,
REGEXP_MATCHES(description, '\d+(\.\d+)?', 'g') as numbers
FROM product_descriptions;
Conclusion
Regular expressions in SQL provide a powerful toolkit for pattern matching and data validation. While they require careful consideration of performance implications and proper error handling, the benefits of using regex for complex string operations far outweigh the challenges. By following best practices and understanding the nuances of different SQL platforms, you can effectively implement regex patterns for various data validation and filtering requirements. As data continues to grow in complexity and volume, the ability to leverage regular expressions effectively becomes increasingly valuable for data professionals.
Disclaimer: The code examples and patterns provided in this blog post are intended for educational purposes and may need to be adapted based on your specific database system and requirements. While we strive for accuracy, different SQL implementations may have varying syntax and behavior. Please test thoroughly in your environment before implementing in production. Report any inaccuracies to our technical team for prompt correction.