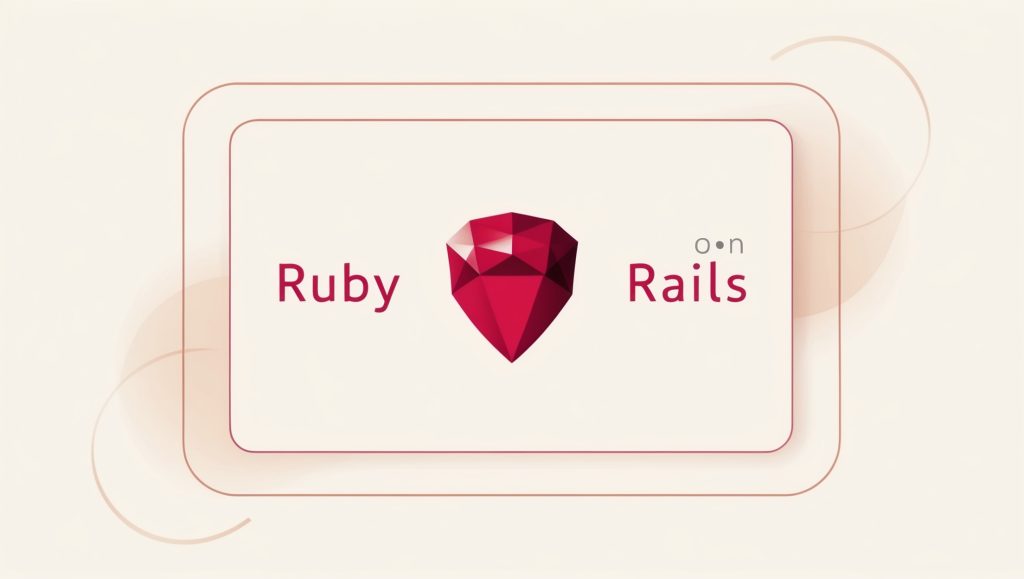
Ruby on Rails and the MVC Paradigm
Ruby on Rails, often simply called Rails, has revolutionized web development since its inception in 2004 by David Heinemeier Hansson. The framework embodies the principles of convention over configuration and don’t repeat yourself (DRY), making it a powerful tool for building modern web applications. Rails has been adopted by numerous prominent companies, including GitHub, Shopify, and Airbnb, demonstrating its capability to handle large-scale, complex applications. The framework’s popularity stems from its elegant syntax, robust ecosystem, and most importantly, its strict adherence to the Model-View-Controller (MVC) architectural pattern, which provides a clear separation of concerns and promotes maintainable, scalable code.
Understanding the MVC Architecture
The Model-View-Controller pattern is a software architectural pattern that divides an application into three interconnected components. Each component serves a specific purpose and maintains a clear separation of concerns, making the application easier to develop, maintain, and scale. Let’s explore each component in detail and understand how Rails implements them.
Models: The Data Layer
Models in Rails represent the business logic and data structure of your application. They serve as an abstraction layer between your application and the database, handling data validation, relationships between different objects, and complex business rules. Models inherit from ActiveRecord::Base, which provides an object-relational mapping (ORM) system that simplifies database operations.
Example of a Rails Model:
class User < ApplicationRecord
has_many :posts
has_many :comments
validates :email, presence: true, uniqueness: true
validates :username, presence: true, length: { minimum: 3, maximum: 50 }
def full_name
"#{first_name} #{last_name}"
end
def recent_posts
posts.where('created_at > ?', 30.days.ago)
end
end
Views: The Presentation Layer
Views in Rails are responsible for presenting data to users in a format they can understand. They contain the HTML, CSS, and JavaScript that create the user interface. Rails uses ERB (Embedded Ruby) by default, allowing you to embed Ruby code within HTML templates.
Example of a Rails View:
<!-- app/views/users/show.html.erb -->
<div class="user-profile">
<h1><%= @user.full_name %></h1>
<div class="user-stats">
<p>Email: <%= @user.email %></p>
<p>Member since: <%= @user.created_at.strftime("%B %d, %Y") %></p>
</div>
<h2>Recent Posts</h2>
<% @user.recent_posts.each do |post| %>
<div class="post">
<h3><%= post.title %></h3>
<p><%= post.content %></p>
</div>
<% end %>
</div>
Controllers: The Logic Layer
Controllers act as intermediaries between models and views. They handle incoming HTTP requests, process user input, interact with models to fetch or modify data, and determine which view to render. Controllers in Rails inherit from ApplicationController and follow RESTful conventions.
Example of a Rails Controller:
class UsersController < ApplicationController
before_action :set_user, only: [#91;:show, :edit, :update, :destroy]#93;
def index
@users = User.all
end
def show
@recent_posts = @user.recent_posts
end
def create
@user = User.new(user_params)
if @user.save
redirect_to @user, notice: 'User was successfully created.'
else
render :new
end
end
private
def set_user
@user = User.find(params[#91;:id]#93;)
end
def user_params
params.require(:user).permit(:email, :username, :first_name, :last_name)
end
end
How Rails Implements MVC
Rails implements the MVC pattern in a way that maximizes developer productivity while maintaining code organization and clarity. The framework provides a structured directory layout where each component has its designated place, making it easy to locate and manage different parts of your application.
Directory Structure
app/
├── models/
├── views/
├── controllers/
├── assets/
├── helpers/
└── mailers/
Rails Convention over Configuration
One of Rails’ core principles is “Convention over Configuration,” which significantly reduces the amount of code developers need to write. Here’s how this principle applies to each MVC component:
Component | Convention | Example |
---|---|---|
Models | Singular, CamelCase | `User`, `BlogPost` |
Controllers | Plural, CamelCase | `UsersController`, `BlogPostsController` |
Views | Matches controller action names | `index.html.erb`, `show.html.erb` |
Database Tables | Plural, snake_case | `users`, `blog_posts` |
Comparison with Other Frameworks
To better understand Rails’ implementation of MVC, let’s compare it with similar patterns in other popular frameworks:
**Django (Python)**:
# models.py
from django.db import models
class User(models.Model):
username = models.CharField(max_length=50)
email = models.EmailField(unique=True)
created_at = models.DateTimeField(auto_now_add=True)
def get_recent_posts(self):
return self.post_set.filter(created_at__gte=timezone.now() - timedelta(days=30))
# views.py
from django.views import View
from django.shortcuts import render
class UserView(View):
def get(self, request, user_id):
user = User.objects.get(id=user_id)
return render(request, 'users/show.html', {'user': user})
**Spring MVC (Java)**:
// Model
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(unique = true)
private String email;
@OneToMany(mappedBy = "user")
private List<Post> posts;
public List<Post> getRecentPosts() {
LocalDateTime thirtyDaysAgo = LocalDateTime.now().minusDays(30);
return posts.stream()
.filter(post -> post.getCreatedAt().isAfter(thirtyDaysAgo))
.collect(Collectors.toList());
}
}
// Controller
@Controller
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public String showUser(@PathVariable Long id, Model model) {
User user = userService.findById(id);
model.addAttribute("user", user);
return "users/show";
}
}
Benefits of Rails’ MVC Implementation
Rapid Development
Rails’ implementation of MVC, combined with its convention over configuration principle, enables developers to build applications quickly. The framework provides:
- Automated database schema migrations
- Built-in testing frameworks
- Asset pipeline for managing JavaScript and CSS
- Automated security features
- RESTful routing conventions
Code Organization and Maintenance
The clear separation of concerns in Rails’ MVC implementation offers several advantages:
- Easier debugging and testing
- Modular code structure
- Simplified collaboration
- Better code reusability
- Clearer application architecture
Advanced MVC Concepts in Rails
Service Objects
For complex business logic that doesn’t fit naturally in models or controllers, Rails developers often use service objects:
class UserRegistrationService
def initialize(params)
@params = params
end
def call
user = User.new(@params)
if user.save
WelcomeMailer.send_welcome_email(user).deliver_later
NotificationService.new(user).notify_admin
true
else
false
end
end
end
Concerns
Rails provides concerns as a way to share common functionality between models and controllers:
module Searchable
extend ActiveSupport::Concern
included do
scope :search, ->(query) {
where("title LIKE ? OR description LIKE ?", "%#{query}%", "%#{query}%")
}
end
def search_excerpt
# Implementation
end
end
class Post < ApplicationRecord
include Searchable
end
Best Practices in Rails MVC
Fat Models, Skinny Controllers
Rails encourages keeping controllers minimal while placing business logic in models:
# Good Practice
class User < ApplicationRecord
def self.active_premium
where(status: 'active', subscription: 'premium')
end
def calculate_subscription_cost
base_price = subscription_plan.price
applied_discounts = discounts.sum(&:amount)
base_price - applied_discounts
end
end
class UsersController < ApplicationController
def premium_users
@users = User.active_premium
end
end
Proper Use of Helpers
View helpers should contain presentation logic that’s reused across views:
module ApplicationHelper
def format_date(date)
date.strftime("%B %d, %Y")
end
def user_avatar(user, size: :medium)
default_url = "default_avatar.png"
image_tag(user.avatar.presence || default_url, class: "avatar-#{size}")
end
end
Security Considerations in Rails MVC
Rails provides several security features that work seamlessly with its MVC implementation:
- CSRF Protection
- SQL Injection Prevention
- XSS Protection
- Mass Assignment Protection
Example of secure parameter handling:
class UsersController < ApplicationController
# Strong Parameters
def user_params
params.require(:user).permit(:username, :email, :password)
end
# CSRF Protection
protect_from_forgery with: :exception
# Authentication
before_action :authenticate_user!
# Authorization
def update
@user = User.find(params[#91;:id]#93;)
authorize @user
if @user.update(user_params)
redirect_to @user
else
render :edit
end
end
end
Testing in Rails MVC
Rails provides a comprehensive testing framework that works well with its MVC structure:
# Model Test
require 'test_helper'
class UserTest < ActiveSupport::TestCase
test "should not save user without email" do
user = User.new
assert_not user.save, "Saved the user without an email"
end
end
# Controller Test
class UsersControllerTest < ActionDispatch::IntegrationTest
test "should get index" do
get users_url
assert_response :success
assert_not_nil assigns(:users)
end
end
# Integration Test
class UserFlowsTest < ActionDispatch::IntegrationTest
test "can create user and then see them" do
get "/users/new"
assert_response :success
post "/users", params: { user: { name: "John", email: "john@example.com" } }
assert_response :redirect
follow_redirect!
assert_response :success
assert_select "h1", "User: John"
end
end
Conclusion
Ruby on Rails’ implementation of the MVC pattern provides a robust foundation for web application development. By following Rails conventions and best practices, developers can build maintainable, secure, and scalable applications efficiently. The framework’s emphasis on convention over configuration, combined with its powerful tools and extensive ecosystem, makes it an excellent choice for both small projects and large-scale applications.
Disclaimer: This article provides general information about Ruby on Rails and the MVC pattern. While we strive for accuracy, the Ruby on Rails framework is continuously evolving, and some features or best practices might have changed. Please refer to the official Rails documentation for the most up-to-date information. If you notice any inaccuracies in this article, please report them so we can make the necessary corrections.