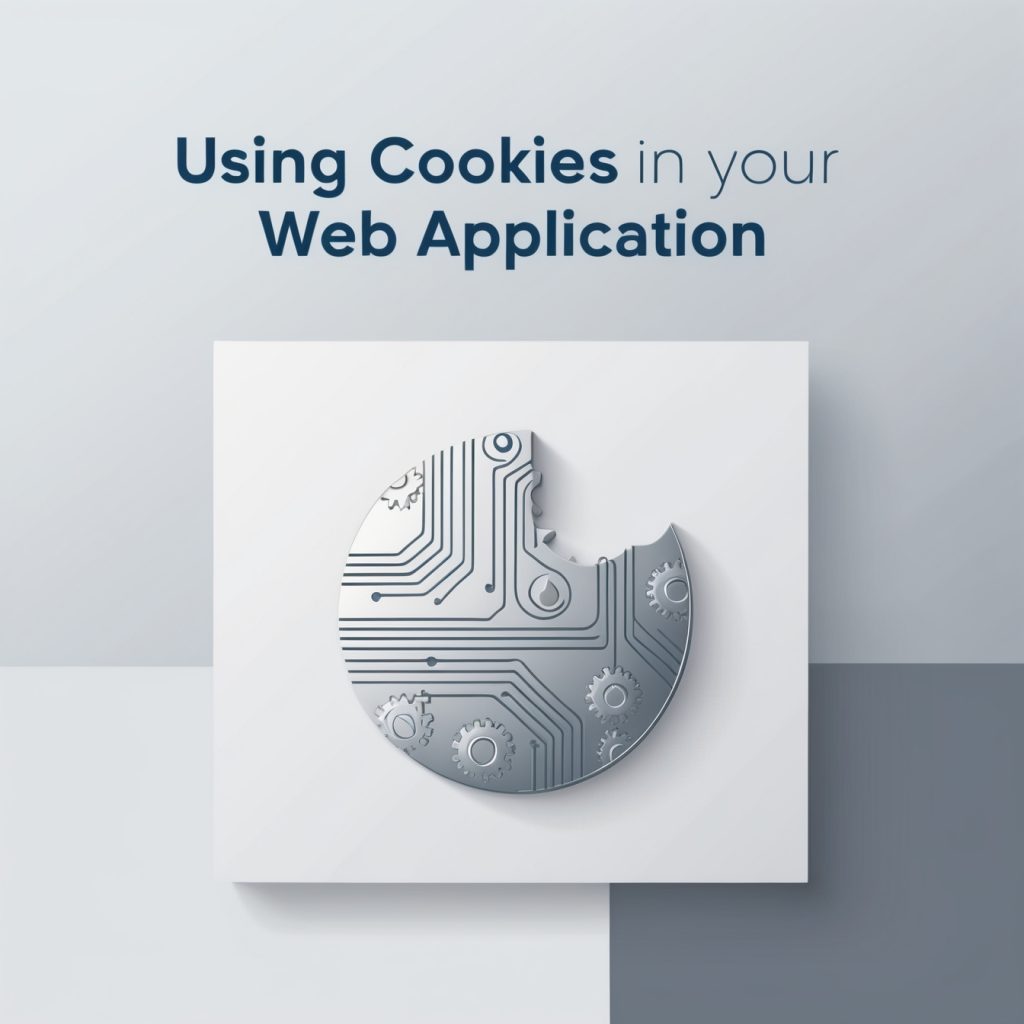
Session Management: Using Cookies in Your Web Application
Hey there, fellow web developers! ๐ Are you ready to dive into the world of session management? Today, we’re going to explore one of the most crucial aspects of web development: implementing session management using cookies. Whether you’re a seasoned pro or just starting out, this guide will help you understand the ins and outs of keeping your users logged in and their data secure. So, grab your favorite beverage, get comfy, and let’s embark on this cookie-filled journey together!
What is Session Management, and Why Should You Care?
Before we jump into the nitty-gritty of implementation, let’s take a moment to understand what session management is all about. In the world of web applications, a session is like a conversation between a user and your website. It’s a way to maintain state and remember information about a user as they navigate through different pages. Think of it as giving each visitor a VIP pass that allows them to have a personalized experience on your site.
Session management is the process of creating, maintaining, and destroying these sessions. It’s what allows users to log in once and stay logged in as they browse, add items to their shopping cart, or interact with various features of your application. Without proper session management, users would need to authenticate themselves on every single page โ talk about a frustrating user experience!
Now, you might be wondering, “Why should I care about session management?” Well, my friend, here are a few compelling reasons:
- Enhanced User Experience: By remembering user preferences and login status, you create a smooth and personalized journey for your visitors.
- Security: Proper session management helps protect user data and prevent unauthorized access to sensitive information.
- State Maintenance: It allows you to keep track of user actions and data across multiple pages without relying solely on server-side storage.
- Scalability: Efficient session management can help your application handle a large number of concurrent users without breaking a sweat.
Now that we’ve covered the basics, let’s dive into the star of our show: cookies!
Cookies: The Sweet Spot of Session Management
What Are Cookies?
Cookies are small pieces of data that websites store on a user’s browser. They’re like little notes that your web application can leave for itself to read later. These digital morsels can contain various types of information, from simple preferences to complex session identifiers.
Why Use Cookies for Session Management?
Cookies are an excellent choice for session management for several reasons:
- Stateless HTTP: The HTTP protocol is stateless by nature, meaning each request is independent. Cookies help bridge this gap by allowing state to be maintained between requests.
- Client-Side Storage: Cookies are stored on the user’s device, reducing the load on your server.
- Automatic Transmission: Browsers automatically send cookies with each request to your server, making them convenient for tracking sessions.
- Customizable Expiration: You can set cookies to expire after a certain time or when the browser session ends, giving you control over session duration.
Now that we’ve established why cookies are the crรจme de la crรจme of session management, let’s roll up our sleeves and get into the implementation!
Implementing Session Management with Cookies: A Step-by-Step Guide
Step 1: Setting Up Your Environment
Before we start baking our session cookies, we need to set up our kitchen (aka development environment). For this guide, we’ll use Node.js with Express as our web framework. If you haven’t already, install Node.js and create a new project directory.
Open your terminal and run the following commands:
mkdir session-management-demo
cd session-management-demo
npm init -y
npm install express cookie-parser
Great! Now we have our basic ingredients ready. Let’s create our main application file:
touch app.js
Open app.js
in your favorite code editor, and let’s start cooking!
Step 2: Creating a Basic Express Server
Let’s set up a simple Express server to get things rolling:
const express = require('express');
const cookieParser = require('cookie-parser');
const app = express();
const port = 3000;
// Middleware
app.use(express.json());
app.use(cookieParser());
// Routes will go here
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
This code sets up a basic Express server with the cookie-parser
middleware, which will help us work with cookies more easily.
Step 3: Implementing User Authentication
Before we can manage sessions, we need a way for users to log in. Let’s create a simple login route:
// Mock user database
const users = [
{ id: 1, username: 'alice', password: 'password123' },
{ id: 2, username: 'bob', password: 'password456' }
];
app.post('/login', (req, res) => {
const { username, password } = req.body;
const user = users.find(u => u.username === username && u.password === password);
if (user) {
// Generate a session ID (in a real app, use a more secure method)
const sessionId = Math.random().toString(36).substring(2, 15);
// Set a cookie with the session ID
res.cookie('sessionId', sessionId, {
httpOnly: true,
maxAge: 3600000 // 1 hour
});
res.json({ message: 'Login successful', user: { id: user.id, username: user.username } });
} else {
res.status(401).json({ message: 'Invalid credentials' });
}
});
In this code, we’re doing a few important things:
- We create a mock user database (in a real application, you’d use a proper database).
- When a user logs in successfully, we generate a simple session ID (in production, use a more secure method like UUID).
- We set a cookie named
sessionId
with the generated ID, making ithttpOnly
for security and setting an expiration time.
Step 4: Creating Protected Routes
Now that we can log users in and set session cookies, let’s create some protected routes that only authenticated users can access:
// Middleware to check if the user is authenticated
const isAuthenticated = (req, res, next) => {
const { sessionId } = req.cookies;
if (sessionId) {
// In a real app, validate the sessionId against a session store
next();
} else {
res.status(401).json({ message: 'Unauthorized' });
}
};
// Protected route
app.get('/dashboard', isAuthenticated, (req, res) => {
res.json({ message: 'Welcome to your dashboard!' });
});
Here, we’ve created a middleware function isAuthenticated
that checks for the presence of a sessionId
cookie. In a real application, you’d validate this ID against a session store to ensure it’s still valid.
Step 5: Logging Out and Destroying Sessions
To complete our session management implementation, we need a way for users to log out:
app.post('/logout', (req, res) => {
res.clearCookie('sessionId');
res.json({ message: 'Logged out successfully' });
});
This simple logout route clears the sessionId
cookie, effectively ending the user’s session.
Best Practices for Secure Session Management
Now that we have a basic implementation up and running, let’s talk about some best practices to keep your session management secure and efficient:
1. Use Secure and HttpOnly Flags
When setting cookies, always use the secure
and httpOnly
flags:
res.cookie('sessionId', sessionId, {
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
maxAge: 3600000
});
The httpOnly
flag prevents client-side access to the cookie, reducing the risk of XSS attacks. The secure
flag ensures the cookie is only sent over HTTPS.
2. Implement Proper Session Storage
In our example, we’re not actually storing session information server-side. In a real application, you should use a session store like Redis or a database to manage sessions:
const session = require('express-session');
const RedisStore = require('connect-redis')(session);
app.use(session({
store: new RedisStore(options),
secret: 'your-secret-key',
resave: false,
saveUninitialized: false,
cookie: { secure: true, httpOnly: true, maxAge: 3600000 }
}));
3. Use Strong Session IDs
Generate strong, unique session IDs to prevent session hijacking:
const crypto = require('crypto');
function generateSessionId() {
return crypto.randomBytes(16).toString('hex');
}
4. Implement Session Expiration
Set reasonable expiration times for your sessions and implement both server-side and client-side expiration checks:
// Server-side expiration check
const isSessionValid = (sessionId) => {
const session = getSessionFromStore(sessionId);
return session && session.expiresAt > Date.now();
};
// Client-side expiration (in milliseconds)
res.cookie('sessionId', sessionId, {
maxAge: 3600000 // 1 hour
});
5. Use CSRF Protection
Implement Cross-Site Request Forgery (CSRF) protection to prevent unauthorized actions on behalf of the authenticated user:
const csrf = require('csurf');
app.use(csrf({ cookie: true }));
app.get('/form', (req, res) => {
res.send(`
<form action="/submit" method="POST">
<input type="hidden" name="_csrf" value="${req.csrfToken()}">
<!-- Other form fields -->
</form>
`);
});
Advanced Session Management Techniques
As your application grows, you might need to implement more advanced session management techniques. Let’s explore a few:
1. Session Rotation
Regularly rotating session IDs can enhance security by limiting the window of opportunity for session hijacking:
app.use((req, res, next) => {
if (req.session && req.session.lastRotated && Date.now() - req.session.lastRotated > 15 * 60 * 1000) {
req.session.regenerate((err) => {
if (err) next(err);
req.session.lastRotated = Date.now();
next();
});
} else {
next();
}
});
This middleware rotates the session every 15 minutes.
2. Multi-Factor Authentication (MFA)
Implement MFA to add an extra layer of security to your session management:
app.post('/login', async (req, res) => {
const { username, password, mfaToken } = req.body;
const user = await authenticateUser(username, password);
if (user) {
if (await verifyMFAToken(user, mfaToken)) {
// Create session and set cookie
} else {
res.status(401).json({ message: 'Invalid MFA token' });
}
} else {
res.status(401).json({ message: 'Invalid credentials' });
}
});
3. Single Sign-On (SSO)
For applications that are part of a larger ecosystem, implementing SSO can provide a seamless experience across multiple services:
const passport = require('passport');
const OIDCStrategy = require('passport-openidconnect').Strategy;
passport.use('oidc', new OIDCStrategy({
issuer: 'https://your-sso-provider.com',
clientID: 'your-client-id',
clientSecret: 'your-client-secret',
callbackURL: 'http://localhost:3000/auth/callback',
scope: 'openid profile email'
}, (issuer, profile, done) => {
// Verify and create or update user
return done(null, profile);
}));
app.get('/auth/sso', passport.authenticate('oidc'));
app.get('/auth/callback', passport.authenticate('oidc', {
successRedirect: '/dashboard',
failureRedirect: '/login'
}));
Troubleshooting Common Session Management Issues
Even with the best implementation, you might encounter some issues. Here are some common problems and how to solve them:
1. Sessions Expiring Too Quickly
If users complain about being logged out too often, check your session expiration settings:
app.use(session({
// ...other options
cookie: { maxAge: 24 * 60 * 60 * 1000 } // Set to 24 hours
}));
2. Sessions Not Working Across Subdomains
To share sessions across subdomains, set the domain option when creating cookies:
res.cookie('sessionId', sessionId, {
domain: '.yourdomain.com',
// other options
});
3. Issues with HTTPS and Secure Cookies
If sessions aren’t persisting when switching between HTTP and HTTPS, ensure you’re setting the secure
flag correctly:
app.use(session({
// ...other options
cookie: { secure: 'auto' } // This sets secure: true in production
}));
Measuring Session Management Performance
To ensure your session management solution is performing well, consider monitoring these key metrics:
Metric | Description | Target |
---|---|---|
Session Creation Time | Time taken to create a new session | < 100ms |
Session Retrieval Time | Time taken to retrieve session data | < 50ms |
Session Store Size | Total size of stored session data | Depends on user base |
Active Sessions | Number of concurrent active sessions | Monitor for unusual spikes |
Session Errors | Rate of session-related errors | < 0.1% of requests |
Regularly reviewing these metrics can help you identify and address performance issues before they impact your users.
Conclusion: Wrapping Up Our Cookie Jar
Phew! We’ve covered a lot of ground in our journey through session management with cookies. From understanding the basics to implementing advanced techniques, you now have the tools to create secure and efficient session management in your web applications.
Remember, session management is an ongoing process. As security threats evolve, so should your implementation. Stay updated with the latest best practices, regularly audit your code, and always prioritize your users’ security and privacy.
I hope this guide has been helpful in sweetening your understanding of session management. Now go forth and create amazing, secure web applications! And remember, like a good cookie, a well-implemented session should be both satisfying and leave your users wanting more (of your app, that is!).
Happy coding, and may your sessions be ever secure! ๐ช๐ป
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, web security is a complex and ever-evolving field. The code examples provided are for educational purposes and may need additional security measures for production use. Always consult the latest security guidelines and best practices when implementing session management in your applications. If you notice any inaccuracies or have suggestions for improvement, please report them so we can update the information promptly. Your security is our priority!