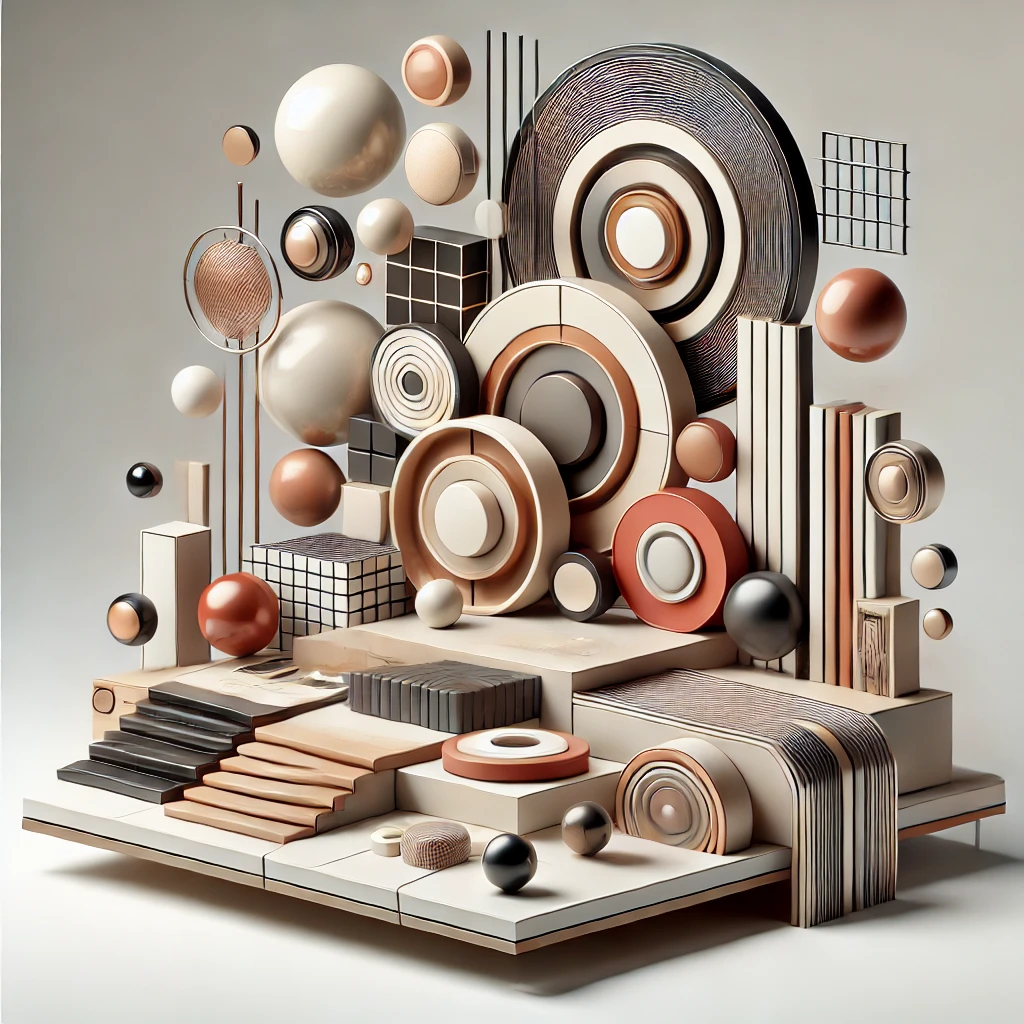
Sets in Java: Unique Collections Simplified
Introduction: The Power of Uniqueness
Have you ever found yourself in a situation where you needed to store a collection of unique elements? Maybe you were working on a project that required keeping track of distinct user IDs, or perhaps you needed to eliminate duplicates from a list of email addresses. If you’ve encountered such scenarios, then you’re in for a treat! Today, we’re diving deep into the world of Sets in Java โ a powerful tool that makes handling unique collections a breeze.
Sets are one of the fundamental data structures in Java, and they’re designed with one primary goal in mind: to store unique elements. Unlike lists or arrays, which can contain duplicate values, Sets ensure that each element appears only once. This unique property makes Sets incredibly useful for a wide range of programming tasks, from removing duplicates to efficiently checking for the presence of specific elements.
In this blog post, we’ll explore the ins and outs of Sets in Java, discussing their characteristics, implementation types, and practical applications. We’ll also dive into code examples to help you understand how to leverage Sets in your own projects. So, whether you’re a Java novice or a seasoned developer looking to refresh your knowledge, buckle up and get ready for an exciting journey through the world of unique collections!
What Are Sets in Java?
Before we dive into the nitty-gritty details, let’s start with the basics. In Java, a Set is an interface that extends the Collection interface. It represents a collection of elements where each element is unique โ no duplicates allowed! This uniqueness is the key characteristic that sets Sets apart from other collection types like Lists or Queues.
Sets in Java are part of the Java Collections Framework, which provides a unified architecture for representing and manipulating collections. The Set interface defines the contract for all Set implementations, ensuring that they adhere to the fundamental principles of uniqueness and unordered storage.
One important thing to note is that Sets don’t maintain any particular order of elements by default. When you iterate through a Set, the order in which elements are returned may not be the same as the order in which they were inserted. However, some Set implementations, like LinkedHashSet, do maintain insertion order.
Key characteristics of Sets:
- Uniqueness: Each element in a Set is unique. If you try to add a duplicate element, the Set will simply ignore it.
- No indexing: Unlike Lists, Sets don’t provide index-based access to elements. You can’t retrieve an element by its position.
- Null elements: Most Set implementations allow null elements, but only one null element can be present due to the uniqueness constraint.
- Fast operations: Sets typically offer fast add, remove, and contains operations, making them efficient for membership testing.
Now that we have a basic understanding of what Sets are, let’s explore the different types of Set implementations available in Java.
Types of Set Implementations in Java
Java provides several implementations of the Set interface, each with its own characteristics and use cases. Let’s take a closer look at the three most commonly used Set implementations:
1. HashSet
HashSet is the most widely used Set implementation in Java. It stores elements in a hash table, which allows for constant-time performance for basic operations like add, remove, and contains (assuming the hash function disperses elements properly).
Here’s an example of how to create and use a HashSet:
import java.util.HashSet;
import java.util.Set;
public class HashSetExample {
public static void main(String[] args) {
Set<String> fruits = new HashSet<>();
// Adding elements
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
fruits.add("Apple"); // This won't be added (duplicate)
System.out.println("Fruits in the set: " + fruits);
System.out.println("Number of fruits: " + fruits.size());
// Checking if an element exists
System.out.println("Contains 'Banana'? " + fruits.contains("Banana"));
// Removing an element
fruits.remove("Orange");
System.out.println("After removing 'Orange': " + fruits);
}
}
In this example, we create a HashSet of strings to store fruit names. Notice how adding a duplicate “Apple” doesn’t increase the size of the Set.
2. TreeSet
TreeSet is an implementation of the SortedSet interface. It stores elements in a sorted tree structure, which means elements are always in sorted order. This makes TreeSet ideal for scenarios where you need to maintain elements in a specific order.
Here’s an example of using TreeSet:
import java.util.Set;
import java.util.TreeSet;
public class TreeSetExample {
public static void main(String[] args) {
Set<Integer> numbers = new TreeSet<>();
// Adding elements
numbers.add(5);
numbers.add(2);
numbers.add(8);
numbers.add(1);
numbers.add(9);
System.out.println("Numbers in sorted order: " + numbers);
// Getting the first and last elements
System.out.println("First number: " + ((TreeSet<Integer>) numbers).first());
System.out.println("Last number: " + ((TreeSet<Integer>) numbers).last());
// Getting a subset
Set<Integer> subset = ((TreeSet<Integer>) numbers).subSet(3, 8);
System.out.println("Subset (3 to 8): " + subset);
}
}
In this example, we create a TreeSet of integers. Notice how the elements are automatically sorted in ascending order. We also demonstrate some TreeSet-specific methods like first(), last(), and subSet().
3. LinkedHashSet
LinkedHashSet is a hybrid between HashSet and LinkedList. It maintains a linked list of entries in the order in which they were inserted, while still providing the O(1) time complexity for basic operations like HashSet.
Here’s an example of using LinkedHashSet:
import java.util.LinkedHashSet;
import java.util.Set;
public class LinkedHashSetExample {
public static void main(String[] args) {
Set<String> colors = new LinkedHashSet<>();
// Adding elements
colors.add("Red");
colors.add("Green");
colors.add("Blue");
colors.add("Yellow");
System.out.println("Colors in insertion order: " + colors);
// Removing and re-adding an element
colors.remove("Green");
colors.add("Green");
System.out.println("After removing and re-adding 'Green': " + colors);
}
}
In this example, we create a LinkedHashSet of strings to store color names. Notice how the order of elements is maintained, even when we remove and re-add an element.
When to Use Each Set Implementation
Now that we’ve explored the different types of Set implementations, you might be wondering when to use each one. Let’s break it down:
- Use HashSet when you need the fastest performance for add, remove, and contains operations, and you don’t care about the order of elements.
- Use TreeSet when you need to maintain elements in a sorted order or when you frequently need to perform range queries (e.g., finding all elements between two values).
- Use LinkedHashSet when you want to maintain insertion order while still benefiting from the fast operations of a hash-based structure.
Choosing the right Set implementation can have a significant impact on your application’s performance and behavior, so it’s essential to consider your specific requirements when making a decision.
Common Set Operations and Their Time Complexity
Understanding the time complexity of common Set operations is crucial for writing efficient code. Let’s take a look at some of the most frequently used operations and their time complexities for each Set implementation:
HashSet:
- add(E e): O(1) average case, O(n) worst case
- remove(Object o): O(1) average case, O(n) worst case
- contains(Object o): O(1) average case, O(n) worst case
- size(): O(1)
TreeSet:
- add(E e): O(log n)
- remove(Object o): O(log n)
- contains(Object o): O(log n)
- size(): O(1)
LinkedHashSet:
- add(E e): O(1)
- remove(Object o): O(1)
- contains(Object o): O(1)
- size(): O(1)
As you can see, HashSet and LinkedHashSet generally offer better performance for basic operations compared to TreeSet. However, TreeSet provides additional functionality like maintaining sorted order and efficient range queries, which can be valuable in certain scenarios.
Practical Applications of Sets in Java
Sets have numerous practical applications in real-world programming scenarios. Let’s explore some common use cases where Sets can simplify your code and improve efficiency:
1. Removing duplicates from a collection
One of the most straightforward applications of Sets is removing duplicates from a collection. Here’s an example:
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class DuplicateRemoval {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>(List.of(1, 2, 3, 2, 4, 1, 5, 3, 6));
Set<Integer> uniqueNumbers = new HashSet<>(numbers);
System.out.println("Original list: " + numbers);
System.out.println("List without duplicates: " + uniqueNumbers);
}
}
In this example, we create a List with duplicate elements and then use a HashSet constructor to remove duplicates efficiently.
2. Checking for unique elements
Sets are excellent for quickly checking if an element exists in a collection. This is particularly useful when you need to ensure uniqueness across a large dataset:
import java.util.HashSet;
import java.util.Set;
public class UniqueChecker {
public static void main(String[] args) {
Set<String> usernames = new HashSet<>();
String[] newUsers = {"alice", "bob", "charlie", "alice", "david"};
for (String username : newUsers) {
if (usernames.add(username)) {
System.out.println("User " + username + " successfully registered.");
} else {
System.out.println("Username " + username + " is already taken.");
}
}
}
}
In this example, we use a Set to keep track of registered usernames and quickly check for duplicates when registering new users.
3. Finding common elements between collections
Sets provide efficient methods for finding common elements between collections. Here’s an example using the retainAll() method:
import java.util.HashSet;
import java.util.Set;
public class CommonElementsFinder {
public static void main(String[] args) {
Set<String> set1 = new HashSet<>(Set.of("a", "b", "c", "d"));
Set<String> set2 = new HashSet<>(Set.of("b", "d", "e", "f"));
Set<String> commonElements = new HashSet<>(set1);
commonElements.retainAll(set2);
System.out.println("Common elements: " + commonElements);
}
}
This example demonstrates how to find common elements between two Sets using the retainAll() method.
4. Implementing a simple spell checker
Sets can be used to implement a basic spell checker by storing a dictionary of valid words:
import java.util.HashSet;
import java.util.Set;
public class SimpleSpellChecker {
private Set<String> dictionary;
public SimpleSpellChecker() {
dictionary = new HashSet<>();
// Add words to the dictionary
dictionary.add("hello");
dictionary.add("world");
dictionary.add("java");
dictionary.add("programming");
}
public boolean isWordValid(String word) {
return dictionary.contains(word.toLowerCase());
}
public static void main(String[] args) {
SimpleSpellChecker spellChecker = new SimpleSpellChecker();
System.out.println("Is 'hello' valid? " + spellChecker.isWordValid("hello"));
System.out.println("Is 'Java' valid? " + spellChecker.isWordValid("Java"));
System.out.println("Is 'python' valid? " + spellChecker.isWordValid("python"));
}
}
This example demonstrates a simple spell checker using a Set to store valid words and quickly check if a given word is in the dictionary.
Advanced Set Techniques: Views and Immutability
As you become more comfortable with Sets, you might want to explore some advanced techniques that can enhance your code’s flexibility and safety. Let’s look at two important concepts: Set views and immutable Sets.
Set Views
Java provides several ways to create views of existing Sets. These views allow you to work with a subset of elements or combine multiple Sets without creating new Set instances. Here are a few examples:
- Unmodifiable Set view:
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
public class UnmodifiableSetExample {
public static void main(String[] args) {
Set<String> mutableSet = new HashSet<>(Set.of("a", "b", "c"));
Set<String> unmodifiableSet = Collections.unmodifiableSet(mutableSet);
System.out.println("Unmodifiable set: " + unmodifiableSet);
try {
unmodifiableSet.add("d"); // This will throw an UnsupportedOperationException
} catch (UnsupportedOperationException e) {
System.out.println("Cannot modify an unmodifiable set!");
}
}
}
This example demonstrates how to create an unmodifiable view of a Set using Collections.unmodifiableSet(). This view prevents modifications to the Set, providing a level of immutability.
- Subset view:
import java.util.SortedSet;
import java.util.TreeSet;
public class SubsetViewExample {
public static void main(String[] args) {
SortedSet<Integer> numbers = new TreeSet<>(Set.of(1, 2, 3, 4, 5, 6, 7, 8, 9, 10));
SortedSet<Integer> subset = numbers.subSet(3, 8);
System.out.println("Original set: " + numbers);
System.out.println("Subset (3 to 8): " + subset);
numbers.add(6); // This will also affect the subset
System.out.println("After adding 6 to the original set:");
System.out.println("Original set: " + numbers);
System.out.println("Subset (3 to 8): " + subset);
}
}
This example shows how to create a subset view of a SortedSet using the subSet() method. Changes to the original Set are reflected in the subset view.
Immutable Sets
While Set views provide a way to create read-only views of existing Sets, sometimes you need truly immutable Sets. Java 9 introduced convenient factory methods for creating immutable Sets:
import java.util.Set;
public class ImmutableSetExample {
public static void main(String[] args) {
Set<String> immutableSet = Set.of("red", "green", "blue");
System.out.println("Immutable set: " + immutableSet);
try {
immutableSet.add("yellow"); // This will throw an UnsupportedOperationException
} catch (UnsupportedOperationException e) {
System.out.println("Cannot modify an immutable set!");
}
}
}
In this example, we use the Set.of() factory method to create an immutable Set. This Set cannot be modified after creation, providing true immutability.
Best Practices and Performance Considerations
To make the most of Sets in your Java projects, it’s important to follow some best practices and keep performance considerations in mind. Here are some tips to help you write efficient and maintainable code:
- Choose the right Set implementation: As we discussed earlier, different Set implementations have different characteristics. Choose the one that best fits your use case to optimize performance.
- Use the diamond operator: When creating a Set, use the diamond operator (<>) to improve code readability and reduce verbosity:
Set<String> set = new HashSet<>(); // Instead of Set<String> set = new HashSet<String>();
- Specify initial capacity: If you know the approximate number of elements your Set will contain, specify an initial capacity to avoid costly resizing operations:
Set<Integer> set = new HashSet<>(1000); // Creates a HashSet with an initial capacity of 1000
- Use Sets for membership testing: When you need to frequently check if an element exists in a collection, using a Set can be much more efficient than using a List, especially for large collections.
- Leverage Set operations: Take advantage of Set methods like addAll(), retainAll(), and removeAll() for efficient set operations instead of implementing them manually.
- Be cautious with custom objects: If you’re using custom objects in a Set, make sure to properly implement the hashCode() and equals() methods to ensure correct behavior:
public class Person {
private String name;
private int age;
// Constructor, getters, and setters omitted for brevity
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
return age == person.age && Objects.equals(name, person.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
- Use streams with Sets: Java 8 introduced streams, which work well with Sets and can make your code more concise and readable:
Set<Integer> numbers = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10));
Set<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toSet());
System.out.println("Even numbers: " + evenNumbers);
- Consider using EnumSet for enum types: If you’re working with enum types, use EnumSet instead of HashSet for better performance and memory usage:
enum Day { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY }
EnumSet<Day> weekdays = EnumSet.range(Day.MONDAY, Day.FRIDAY);
System.out.println("Weekdays: " + weekdays);
Conclusion
We’ve taken a deep dive into the world of Sets in Java, exploring their unique properties, different implementations, and practical applications. From removing duplicates to implementing efficient membership testing, Sets offer a powerful tool for handling collections of unique elements in your Java projects.
Remember, the key to effectively using Sets lies in understanding their strengths and choosing the right implementation for your specific needs. Whether you opt for the blazing-fast HashSet, the ordered TreeSet, or the insertion-order-preserving LinkedHashSet, each has its place in a Java developer’s toolkit.
As you continue to work with Sets, don’t forget to leverage advanced techniques like Set views and immutable Sets to enhance the safety and flexibility of your code. And always keep best practices and performance considerations in mind to ensure your Set-based solutions are as efficient as possible.
Sets may seem simple at first glance, but their ability to handle unique collections elegantly and efficiently makes them an indispensable part of Java programming. So the next time you find yourself dealing with a collection of distinct elements, remember the power of Sets โ they might just be the perfect tool for the job!
Happy coding, and may your collections always be uniquely awesome!
Disclaimer: While every effort has been made to ensure the accuracy and completeness of the information in this blog post, programming practices and Java specifications may change over time. Always refer to the official Java documentation for the most up-to-date and accurate information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.