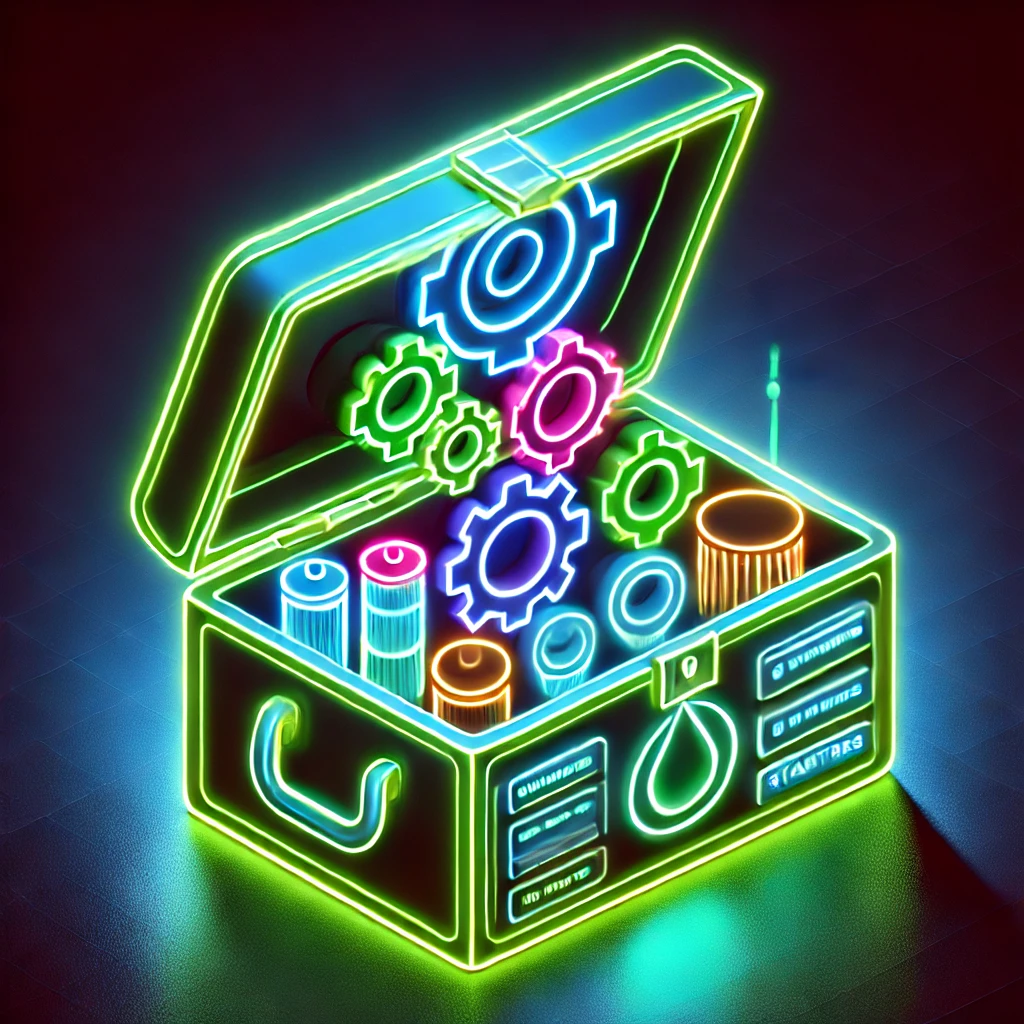
Simplify Dependency Management through Spring Boot Starters
Spring Boot has revolutionized the way Java developers build applications, offering a streamlined approach to creating production-ready applications with minimal configuration. At the heart of this simplification lies Spring Boot Starters, a powerful concept that significantly reduces the complexity of dependency management. This comprehensive guide delves into the world of Spring Boot Starters, exploring their benefits, inner workings, and how they can enhance your development process. Whether you’re a seasoned Spring developer or just starting your journey with Spring Boot, this article will provide valuable insights into leveraging these powerful tools to streamline your projects and boost productivity.
Understanding Spring Boot Starters
What are Spring Boot Starters?
Spring Boot Starters are a set of convenient dependency descriptors that you can include in your application. They are designed to simplify the process of adding common functionality to your Spring Boot projects by bundling related dependencies together. Instead of manually adding and managing multiple dependencies, you can include a single starter that brings in all the necessary libraries and configurations for a particular functionality.
The Purpose of Spring Boot Starters
The primary goal of Spring Boot Starters is to address the “dependency hell” problem that developers often face when setting up new projects. By providing curated sets of dependencies, Spring Boot Starters ensure that compatible versions of required libraries are included in your project. This approach not only saves time but also reduces the likelihood of version conflicts and incompatibilities between different libraries.
How Spring Boot Starters Work
When you include a Spring Boot Starter in your project’s build file (e.g., pom.xml for Maven or build.gradle for Gradle), it automatically pulls in a collection of dependencies that are commonly used together for a specific purpose. These dependencies are transitively resolved, meaning that not only the direct dependencies of the starter are included but also their dependencies, creating a comprehensive and coherent set of libraries for your application.
Key Benefits of Using Spring Boot Starters
Simplified Dependency Management
One of the most significant advantages of Spring Boot Starters is the simplification of dependency management. Instead of manually specifying multiple dependencies and their versions, developers can include a single starter that encapsulates all necessary libraries for a particular functionality. This approach significantly reduces the potential for version conflicts and ensures that all included libraries are compatible with each other.
Rapid Application Development
Spring Boot Starters enable developers to quickly bootstrap applications with specific capabilities. By including the appropriate starter, you can instantly add features like web support, database connectivity, or security to your application without the need for extensive configuration. This rapid setup allows developers to focus more on writing business logic rather than spending time on boilerplate configuration.
Consistency Across Projects
By using Spring Boot Starters, teams can maintain consistency across different projects. Starters provide a standardized way of including common functionalities, ensuring that all projects within an organization follow similar patterns and best practices. This consistency facilitates easier maintenance, knowledge sharing, and onboarding of new team members.
Auto-Configuration
Spring Boot Starters work hand-in-hand with Spring Boot’s auto-configuration feature. When a starter is included, Spring Boot automatically configures the components provided by that starter based on the classpath contents and environment properties. This intelligent configuration reduces the need for manual setup and allows developers to get started with minimal effort.
Common Spring Boot Starters and Their Use Cases
Spring Boot offers a wide range of starters for various purposes. Let’s explore some of the most commonly used starters and their typical use cases:
spring-boot-starter-web
This starter is essential for building web applications, including RESTful applications, using Spring MVC. It includes dependencies for Tomcat as the default embedded container, along with Spring MVC and other web-related components.
Use case: Developing web applications, RESTful services, or any project that requires handling HTTP requests.
spring-boot-starter-data-jpa
This starter provides support for working with relational databases using Spring Data JPA and Hibernate. It includes dependencies for Spring Data JPA, Hibernate, and the Java Persistence API (JPA).
Use case: Implementing data access layers for applications that interact with relational databases.
spring-boot-starter-security
This starter adds Spring Security to your application, providing authentication and authorization support. It includes core Spring Security dependencies and auto-configuration for common security features.
Use case: Implementing security features in your application, such as user authentication, role-based access control, and secure communication.
spring-boot-starter-test
This starter includes dependencies for testing Spring Boot applications, including JUnit, Spring Test, and Mockito. It’s typically used as a test-scoped dependency.
Use case: Writing and running unit and integration tests for your Spring Boot application.
spring-boot-starter-actuator
This starter adds production-ready features to your application, allowing you to monitor and manage your application when it’s running in production. It provides endpoints for health checks, metrics, and other operational information.
Use case: Implementing monitoring and management capabilities for applications running in production environments.
Creating a Custom Spring Boot Starter
While Spring Boot provides a comprehensive set of starters for common use cases, there may be situations where you need to create a custom starter to encapsulate specific functionality or configuration that is unique to your organization or project. Let’s walk through the process of creating a custom Spring Boot Starter.
Step 1: Set Up the Project Structure
Create a new Maven project with the following structure:
my-custom-starter/
├── pom.xml
└── src/
├── main/
│ ├── java/
│ │ └── com/
│ │ └── example/
│ │ └── mycustomstarter/
│ │ ├── MyCustomAutoConfiguration.java
│ │ └── MyCustomProperties.java
│ └── resources/
│ └── META-INF/
│ └── spring.factories
└── test/
└── java/
└── com/
└── example/
└── mycustomstarter/
└── MyCustomStarterTest.java
Step 2: Configure the POM File
In your pom.xml
, include the necessary dependencies and configuration:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-custom-starter</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<java.version>11</java.version>
<spring-boot.version>2.5.0</spring-boot.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>${spring-boot.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
</project>
Step 3: Create the Auto-Configuration Class
Create the MyCustomAutoConfiguration
class to define the beans and configuration for your custom starter:
package com.example.mycustomstarter;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableConfigurationProperties(MyCustomProperties.class)
public class MyCustomAutoConfiguration {
private final MyCustomProperties properties;
public MyCustomAutoConfiguration(MyCustomProperties properties) {
this.properties = properties;
}
@Bean
@ConditionalOnMissingBean
public MyCustomService myCustomService() {
return new MyCustomService(properties.getMessage());
}
}
Step 4: Define Configuration Properties
Create the MyCustomProperties
class to allow users of your starter to customize its behavior:
package com.example.mycustomstarter;
import org.springframework.boot.context.properties.ConfigurationProperties;
@ConfigurationProperties(prefix = "my.custom")
public class MyCustomProperties {
private String message = "Default message";
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
Step 5: Create the Service Class
Implement the MyCustomService
class that provides the actual functionality of your starter:
package com.example.mycustomstarter;
public class MyCustomService {
private final String message;
public MyCustomService(String message) {
this.message = message;
}
public String sayHello() {
return "Hello from MyCustomService: " + message;
}
}
Step 6: Configure spring.factories
Create a spring.factories
file in the src/main/resources/META-INF/
directory to enable auto-configuration:
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.example.mycustomstarter.MyCustomAutoConfiguration
Step 7: Build and Publish
Build your custom starter using Maven:
mvn clean install
You can now include your custom starter in other projects by adding it as a dependency:
<dependency>
<groupId>com.example</groupId>
<artifactId>my-custom-starter</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
Best Practices for Using Spring Boot Starters
To make the most of Spring Boot Starters and ensure smooth development, consider the following best practices:
1. Choose the Right Starters
Carefully select the starters that align with your project requirements. Avoid including unnecessary starters, as they can increase your application’s footprint and potentially introduce unused dependencies.
2. Understand Transitive Dependencies
Be aware of the transitive dependencies that come with each starter. Familiarize yourself with the components included to avoid potential conflicts and to make informed decisions when customizing your application.
3. Override Versions When Necessary
While Spring Boot manages dependency versions, there may be cases where you need to use a specific version of a library. Use your build tool’s dependency management features to override versions when required, but be cautious about potential compatibility issues.
4. Customize Auto-Configuration
Take advantage of Spring Boot’s auto-configuration, but don’t hesitate to customize it when needed. You can exclude specific auto-configuration classes or provide your own beans to override default behavior.
5. Use Property Configuration
Utilize Spring Boot’s property-based configuration to customize the behavior of starters. Many starters expose configuration properties that allow you to fine-tune their functionality without modifying code.
6. Keep Starters Updated
Regularly update your Spring Boot version and associated starters to benefit from the latest features, performance improvements, and security patches. Always review the release notes and migration guides when upgrading.
7. Combine Starters Judiciously
When combining multiple starters, be mindful of potential conflicts or overlapping functionalities. Spring Boot generally handles these scenarios well, but it’s essential to understand the implications of using multiple starters together.
Troubleshooting Common Issues with Spring Boot Starters
While Spring Boot Starters significantly simplify development, you may encounter some challenges. Here are some common issues and their solutions:
Dependency Conflicts
Issue: Version conflicts between dependencies brought in by different starters.
Solution: Use your build tool’s dependency management features to explicitly define versions. For Maven, you can use <dependencyManagement>
in your pom.xml
.
Auto-Configuration Surprises
Issue: Unexpected auto-configuration behavior.
Solution: Enable debug logging for auto-configuration by setting logging.level.org.springframework.boot.autoconfigure=DEBUG
in your application.properties
. This will show you which auto-configurations are being applied or skipped.
Missing Dependencies
Issue: Required dependencies are not included despite using a starter.
Solution: Check if you’ve accidentally excluded a necessary dependency. Review the starter’s documentation to ensure you’re using it correctly.
Custom Starter Not Being Detected
Issue: Your custom starter is not being auto-configured.
Solution: Ensure that your spring.factories
file is correctly placed in the META-INF
directory and that it’s properly formatted.
Conclusion
Spring Boot Starters have revolutionized the way Java developers approach dependency management and application configuration. By providing curated sets of dependencies and leveraging auto-configuration, starters enable rapid application development, promote consistency, and significantly reduce boilerplate code. Understanding how to effectively use Spring Boot Starters, create custom starters, and troubleshoot common issues empowers developers to build robust, scalable applications with ease.
As you continue to work with Spring Boot, remember that starters are just the beginning. They provide a solid foundation upon which you can build complex, feature-rich applications. By following best practices and staying updated with the latest Spring Boot developments, you can maximize the benefits of this powerful framework and focus on delivering value through your applications.
Table: Overview of Common Spring Boot Starters
Starter Name | Purpose | Key Components |
---|---|---|
spring-boot-starter-web | Web application development | Spring MVC, Embedded Tomcat |
spring-boot-starter-data-jpa | Data access with JPA | Spring Data JPA, Hibernate |
spring-boot-starter-security | Application security | Spring Security |
spring-boot-starter-test | Testing support | JUnit, Mockito, Spring Test |
spring-boot-starter-actuator | Production-ready features | Health checks, metrics, monitoring |
spring-boot-starter-thymeleaf | Server-side Java templating | Thymeleaf template engine |
spring-boot-starter-data-mongodb | MongoDB data access | Spring Data MongoDB |
spring-boot-starter-data-redis | Redis data access | Spring Data Redis, Lettuce |
spring-boot-starter-batch | Batch processing | Spring Batch |
spring-boot-starter-websocket | WebSocket support | Spring WebSocket |
This table provides a quick reference for some of the most commonly used Spring Boot Starters, their primary purposes, and key components they include. It can serve as a handy guide when deciding which starters to include in your projects based on your specific requirements.
Disclaimer: The information provided in this blog post is based on the current understanding and implementation of Spring Boot Starters as of the knowledge cutoff date. While every effort has been made to ensure accuracy, the Spring ecosystem is continuously evolving. Readers are encouraged to consult the official Spring Boot documentation for the most up-to-date information. If you notice any inaccuracies or have suggestions for improvement, please report them so we can promptly make corrections and provide the most valuable content to our readers.