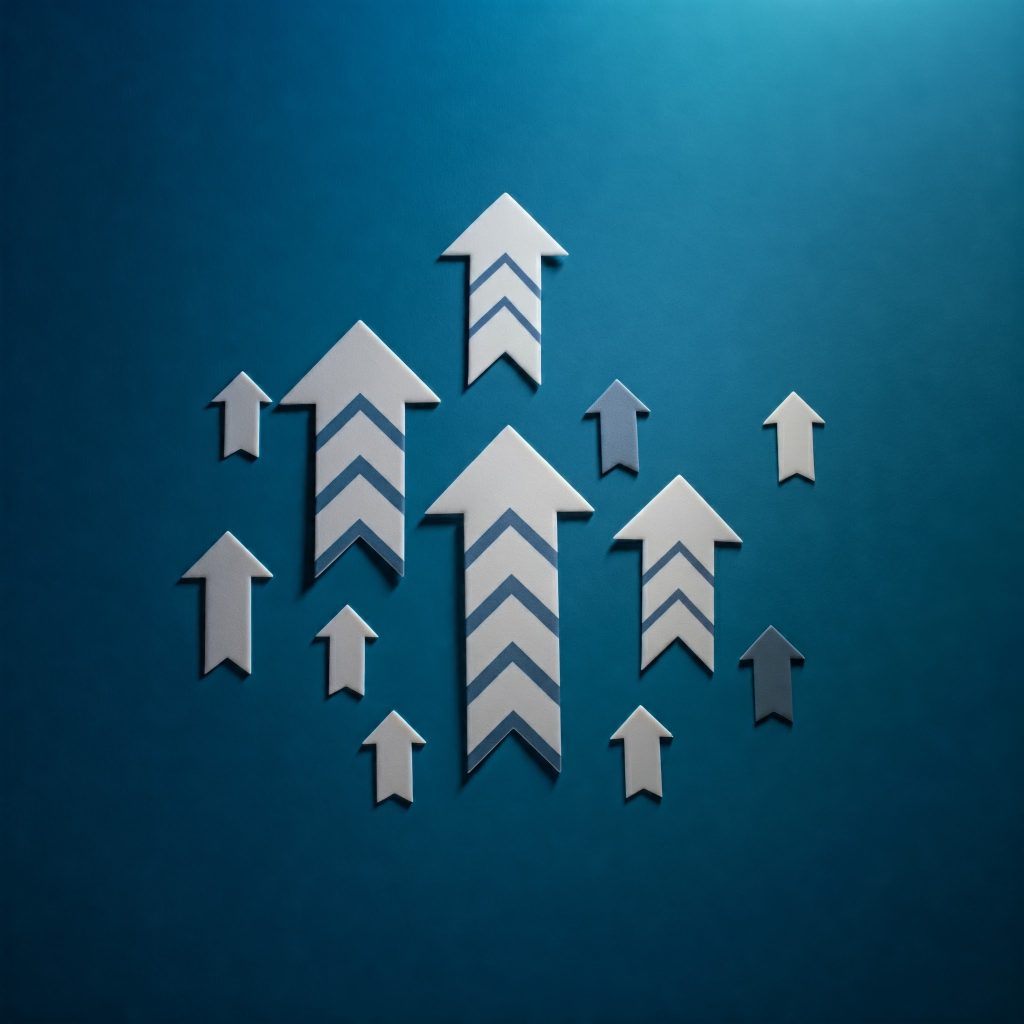
Sorting Data with ORDER BY in SQL: Arranging Results for Better Readability
In the world of databases, effective data retrieval is key to making sense of stored information. SQL (Structured Query Language) offers many features that enable users to interact with data efficiently. One of the most crucial tools for data arrangement is the ORDER BY
clause. This clause allows you to sort your query results in a meaningful order, enhancing readability and making data analysis easier. Whether you need to sort records alphabetically, numerically, or based on dates, understanding the ORDER BY
clause is fundamental to using SQL proficiently.
In this comprehensive guide, we will delve into the ORDER BY
clause, covering everything from its syntax to more advanced sorting techniques. This article is ideal for SQL beginners and intermediate users looking to improve the organization and presentation of their query results.
Understanding the Basics of ORDER BY
The ORDER BY
clause in SQL is used to sort the result set of a query based on one or more columns. By default, SQL returns results in no particular order unless explicitly instructed otherwise. The ORDER BY
clause allows you to control how the rows are ordered in the output.
Here’s the general syntax:
SELECT column1, column2, ...
FROM table_name
ORDER BY column1 [ASC | DESC], column2 [ASC | DESC], ...;
column1
,column2
, …: These are the columns by which you want to sort the data.ASC
: Sorts the data in ascending order (default behavior).DESC
: Sorts the data in descending order.
Ascending vs. Descending Order
SQL allows for sorting in either ascending or descending order. By default, the ORDER BY
clause sorts the results in ascending order. However, if you need the results in descending order, you can explicitly specify this with the DESC
keyword.
Example 1: Sorting in Ascending Order
Let’s retrieve a list of employees sorted by their first names:
SELECT first_name, last_name, salary
FROM employees
ORDER BY first_name ASC;
In this example, the query fetches data from the employees
table and sorts the results by first_name
in ascending order.
Example 2: Sorting in Descending Order
Now, let’s sort the employees by their salaries, in descending order:
SELECT first_name, last_name, salary
FROM employees
ORDER BY salary DESC;
In this case, the ORDER BY
clause ensures that the highest-paid employees appear at the top of the list.
Sorting by Multiple Columns
The ORDER BY
clause can also be used to sort by multiple columns. When you sort by more than one column, SQL will first sort by the first column listed. If there are duplicate values in that column, it will then sort by the second column, and so on.
Example: Sorting by Last Name and First Name
If you want to sort employees by last name and then by first name (in case two employees have the same last name), you can use the following query:
SELECT first_name, last_name, salary
FROM employees
ORDER BY last_name ASC, first_name ASC;
This query ensures that employees are sorted alphabetically by last name first. If two employees share the same last name, it then sorts them by their first name.
First Name | Last Name | Salary |
---|---|---|
John | Doe | 50,000 |
Jane | Doe | 60,000 |
Alice | Smith | 70,000 |
Bob | Smith | 80,000 |
In this case, you can see that the table is first sorted by the last name, and then by the first name where necessary.
Sorting by Column Position
In addition to sorting by column names, SQL also allows you to sort by the position of the columns in the SELECT
statement. This is particularly useful when dealing with long queries, where typing out the column names can be cumbersome.
Example: Sorting by Column Position
In the following query, we sort by the first and third columns, without specifying their names:
SELECT first_name, last_name, salary
FROM employees
ORDER BY 2, 3 DESC;
Here, the query will sort the results by last_name
(the second column in the SELECT
statement) in ascending order, and then by salary
(the third column) in descending order. This technique is helpful when you want to prioritize positional accuracy over clarity.
First Name | Last Name | Salary |
---|---|---|
John | Doe | 60,000 |
Alice | Smith | 70,000 |
Bob | Smith | 50,000 |
Sorting with NULL Values
When sorting data in SQL, NULL
values can present a challenge. By default, NULL
values are considered the lowest possible values and will appear first when sorting in ascending order and last when sorting in descending order.
Example: Sorting with NULL Values
Assume we have a table of employees where some employees do not have an assigned department (NULL value). To sort the employees by their department, the query would look like this:
SELECT first_name, last_name, department
FROM employees
ORDER BY department ASC;
This query will return the employees with no department (NULL values) first:
First Name | Last Name | Department |
---|---|---|
John | Doe | NULL |
Alice | Smith | HR |
Bob | Johnson | Sales |
To reverse this behavior, you can sort the data in descending order:
SELECT first_name, last_name, department
FROM employees
ORDER BY department DESC;
Alternatively, many SQL dialects allow for customization of how NULL
values are treated using the NULLS FIRST
or NULLS LAST
keywords:
SELECT first_name, last_name, department
FROM employees
ORDER BY department ASC NULLS LAST;
This query ensures that rows with NULL
values appear at the end of the result set when sorting in ascending order.
Using Expressions in ORDER BY
The ORDER BY
clause is not limited to sorting by column values alone. You can also sort based on expressions, computations, or even functions.
Example: Sorting by an Expression
Let’s say we want to calculate an employee’s annual salary (monthly salary * 12) and sort the results by that computed value:
SELECT first_name, last_name, salary * 12 AS annual_salary
FROM employees
ORDER BY salary * 12 DESC;
In this example, we multiply the salary
by 12 to compute the annual salary and then sort the results in descending order based on this computed value.
Combining ORDER BY with Other Clauses
The ORDER BY
clause can be used in combination with other SQL clauses such as WHERE
, GROUP BY
, and HAVING
to refine your query results and sort them efficiently.
Example: Using ORDER BY with WHERE
In the following query, we retrieve employees who have a salary greater than 50,000 and sort them by salary in descending order:
SELECT first_name, last_name, salary
FROM employees
WHERE salary > 50000
ORDER BY salary DESC;
This query first filters the results to include only employees with a salary greater than 50,000, then orders them by salary.
Example: Using ORDER BY with GROUP BY
You can also use ORDER BY
in conjunction with the GROUP BY
clause to sort aggregated data. Let’s say we want to group employees by department and calculate the total salary per department. We can then sort these departments by total salary:
SELECT department, SUM(salary) AS total_salary
FROM employees
GROUP BY department
ORDER BY total_salary DESC;
This query groups employees by their departments, calculates the total salary for each department, and orders the result by the total salary in descending order.
Performance Considerations with ORDER BY
While the ORDER BY
clause is highly useful, it’s important to be aware of potential performance issues when sorting large datasets. Sorting can be resource-intensive, especially when working with large tables or multiple columns.
Tips for Optimizing Performance:
- Indexing: Indexing the columns used in the
ORDER BY
clause can significantly improve query performance by allowing SQL to retrieve sorted data more efficiently. - Limit the Results: Use the
LIMIT
clause (or equivalent) to restrict the number of rows returned, which can help reduce the processing time for sorting.
- Example:
SELECT first_name, last_name, salary
FROM employees
ORDER BY salary DESC
LIMIT 10;
- Avoid Complex Expressions: Sorting by simple columns rather than complex expressions or functions can also improve performance.
Practical Examples of ORDER BY in Different Scenarios
1. Sorting Employee Names Alphabetically
SELECT first_name, last_name
FROM employees
ORDER BY last_name ASC, first_name ASC;
In this example, the query will return employees sorted alphabetically by their last name and, where necessary, by their first name.
2. Sorting Products by Price
SELECT product_name, price
FROM products
ORDER BY price DESC;
This
query sorts products by their price in descending order, showing the most expensive products first.
3. Sorting Customers by Registration Date
SELECT customer_name, registration_date
FROM customers
ORDER BY registration_date ASC;
Here, the query sorts customers by their registration date in ascending order, listing the earliest registrations first.
Conclusion
The ORDER BY
clause is a versatile and powerful feature in SQL that enables you to arrange query results in a meaningful and readable manner. By mastering the use of ORDER BY
, you can significantly improve the readability of your result sets and make it easier to derive insights from your data. Whether sorting by a single column, multiple columns, or even expressions, understanding how to leverage this tool is an essential part of becoming proficient in SQL.
Furthermore, it’s important to keep in mind that while the ORDER BY
clause is invaluable for organizing data, it can also impact query performance, especially with large datasets. By optimizing your queries through indexing and limiting results, you can ensure efficient data retrieval without sacrificing performance.
If you have any questions or notice any inaccuracies in this article, please feel free to reach out to us so we can make the necessary corrections.