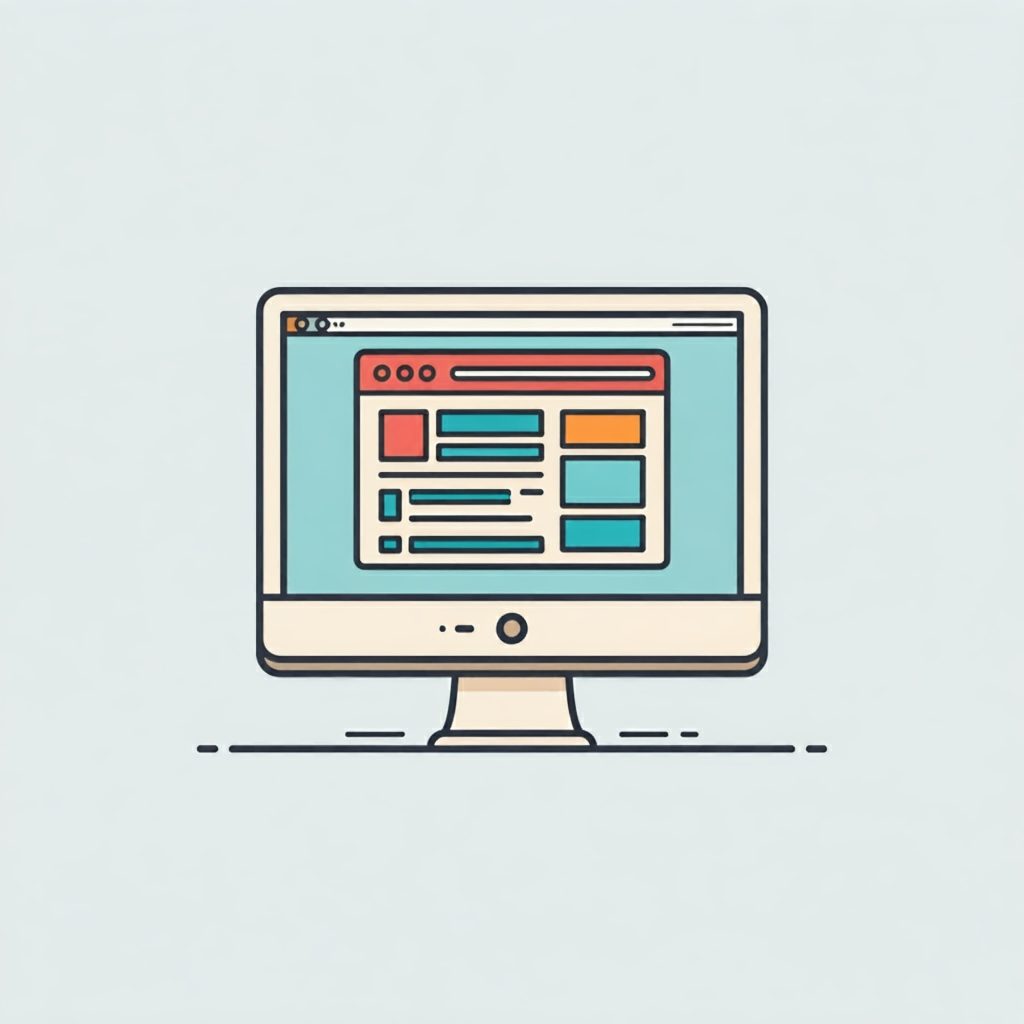
Spring Boot and Thymeleaf – Creating Dynamic Web Pages
In the realm of modern web development, creating dynamic and responsive web pages is crucial for delivering engaging user experiences. Two powerful tools that have gained significant traction in this domain are Spring Boot and Thymeleaf. Spring Boot, a popular framework for building Java-based applications, simplifies the development process by providing a streamlined approach to configuration and deployment. Thymeleaf, on the other hand, is a modern server-side Java template engine that seamlessly integrates with Spring Boot to create dynamic HTML templates. Together, these technologies offer a robust solution for developing sophisticated web applications with ease and efficiency.
This comprehensive guide will delve into the intricacies of using Spring Boot and Thymeleaf to create dynamic web pages. We will explore the fundamental concepts, best practices, and advanced techniques that will empower you to harness the full potential of these technologies. Whether you are a seasoned developer looking to expand your skill set or a newcomer to web development, this blog post will provide valuable insights and practical knowledge to enhance your ability to create compelling web applications.
Understanding Spring Boot
Spring Boot is an open-source Java-based framework designed to simplify the development of stand-alone, production-grade Spring applications. It builds upon the popular Spring Framework, providing a set of tools and conventions that streamline the configuration process and reduce boilerplate code. Spring Boot’s “opinionated” approach to application development allows developers to focus on writing business logic rather than getting bogged down in complex configuration details.
Key Features of Spring Boot:
- Auto-configuration: Spring Boot automatically configures your application based on the dependencies you have added to your project. This feature significantly reduces the amount of manual configuration required, allowing you to get your application up and running quickly.
- Standalone: Spring Boot applications are self-contained and do not require an external web server. They can be run as standalone Java applications, making deployment and testing more straightforward.
- Embedded server: Spring Boot includes an embedded web server (such as Tomcat, Jetty, or Undertow) by default, eliminating the need for external server setup and configuration.
- Production-ready: Spring Boot provides out-of-the-box features for monitoring, health checks, and externalized configuration, making it easier to deploy and manage applications in production environments.
- Spring ecosystem integration: Spring Boot seamlessly integrates with other Spring projects, such as Spring Data, Spring Security, and Spring Cloud, allowing you to leverage a wide range of powerful tools and libraries.
To get started with Spring Boot, you’ll need to set up your development environment and create a new project. The easiest way to do this is by using the Spring Initializer (https://start.spring.io/), which generates a basic project structure with all the necessary dependencies.
Introduction to Thymeleaf
Thymeleaf is a modern server-side Java template engine that excels in processing and creating HTML, XML, JavaScript, CSS, and plain text. It is designed to be easily integrated with Spring Framework, making it an ideal choice for developers working with Spring Boot applications. Thymeleaf’s natural templating approach allows designers and developers to create templates that can be viewed as static prototypes in a browser, while still being fully functional when processed on the server.
Key Features of Thymeleaf:
- Natural templates: Thymeleaf templates are valid HTML files that can be opened in a browser without processing, making it easier for designers and developers to collaborate.
- Spring integration: Thymeleaf offers excellent integration with Spring Framework, providing seamless access to Spring beans and other Spring-specific features.
- Dialect extensibility: Thymeleaf allows you to create custom dialects, enabling you to extend its functionality and create reusable components.
- Layout system: Thymeleaf includes a flexible layout system that facilitates the creation of reusable page layouts and components.
- Internationalization: Thymeleaf provides built-in support for internationalization, making it easy to create multi-language applications.
To use Thymeleaf in your Spring Boot application, you’ll need to add the appropriate dependency to your project. If you’re using Maven, you can include the following dependency in your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
Once you’ve added the dependency, Spring Boot’s auto-configuration will automatically set up Thymeleaf as the template engine for your application.
Setting Up a Spring Boot Project with Thymeleaf
To create a new Spring Boot project with Thymeleaf support, follow these steps:
- Visit the Spring Initializer website (https://start.spring.io/).
- Choose your project settings:
- Project: Maven
- Language: Java
- Spring Boot: (Select the latest stable version)
- Group: com.example
- Artifact: thymeleaf-demo
- Name: thymeleaf-demo
- Description: Demo project for Spring Boot and Thymeleaf
- Package name: com.example.thymeleafdemo
- Packaging: Jar
- Java: (Select your preferred version)
- Add the following dependencies:
- Spring Web
- Thymeleaf
- Spring Boot DevTools (optional, but recommended for development)
- Click “Generate” to download the project files.
- Extract the downloaded ZIP file and import the project into your preferred IDE.
Once you’ve set up your project, you can start creating dynamic web pages using Thymeleaf templates.
Creating Your First Thymeleaf Template
Let’s create a simple “Hello, World!” page to demonstrate how Thymeleaf works with Spring Boot. First, create a new controller class in your project:
package com.example.thymeleafdemo.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("/")
public String hello(Model model) {
model.addAttribute("message", "Hello, World!");
return "hello";
}
}
This controller defines a single endpoint (“/”) that adds a “message” attribute to the model and returns the name of the template to render (“hello”).
Next, create a new Thymeleaf template file named hello.html
in the src/main/resources/templates
directory:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Hello, Thymeleaf!</title>
</head>
<body>
<h1 th:text="${message}">Default Message</h1>
</body>
</html>
In this template, we use the th:text
attribute to display the value of the message
attribute from the model. The “Default Message” text serves as a placeholder that will be displayed when viewing the template statically in a browser.
Now, run your Spring Boot application and navigate to http://localhost:8080
in your web browser. You should see the “Hello, World!” message displayed on the page.
Working with Thymeleaf Expressions
Thymeleaf provides a rich set of expressions and attributes that allow you to create dynamic content in your templates. Let’s explore some common Thymeleaf expressions and their usage:
1. Variable expressions: ${…}
Variable expressions allow you to access attributes from the model or other variables in the template context.
Example:
<p th:text="${user.name}">John Doe</p>
2. Selection expressions: *{…}
Selection expressions are used in conjunction with the th:object
attribute to simplify access to object properties.
Example:
<div th:object="${user}">
<p th:text="*{name}">John Doe</p>
<p th:text="*{email}">john@example.com</p>
</div>
3. Link expressions: @{…}
Link expressions are used to generate URLs, taking into account the context path of your application.
Example:
<a th:href="@{/user/{id}(id=${user.id})}">View User</a>
4. Fragment expressions: ~{…}
Fragment expressions allow you to reuse parts of your templates, promoting modular design and reducing code duplication.
Example:
<div th:insert="~{fragments/header :: header}"></div>
5. Conditional expressions
Thymeleaf provides several attributes for conditional rendering:
th:if
/th:unless
: Renders content based on a conditionth:switch
/th:case
: Implements switch-case logic
Example:
<p th:if="${user.isAdmin}">Welcome, Admin!</p>
<div th:switch="${user.role}">
<p th:case="'admin'">You have full access.</p>
<p th:case="'user'">You have limited access.</p>
<p th:case="*">You have no access.</p>
</div>
6. Iteration
The th:each
attribute allows you to iterate over collections or arrays.
Example:
<ul>
<li th:each="item : ${items}" th:text="${item.name}">Item Name</li>
</ul>
Handling Forms with Thymeleaf and Spring Boot
One of the most common tasks in web development is handling form submissions. Thymeleaf provides excellent support for creating and processing forms in conjunction with Spring Boot. Let’s create a simple user registration form to demonstrate this functionality.
First, create a User
class to represent the form data:
package com.example.thymeleafdemo.model;
public class User {
private String name;
private String email;
private String password;
// Getters and setters
}
Next, create a controller to handle the form:
package com.example.thymeleafdemo.controller;
import com.example.thymeleafdemo.model.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
@Controller
public class UserController {
@GetMapping("/register")
public String showRegistrationForm(Model model) {
model.addAttribute("user", new User());
return "register";
}
@PostMapping("/register")
public String registerUser(@ModelAttribute("user") User user, Model model) {
// Process user registration (e.g., save to database)
model.addAttribute("successMessage", "Registration successful!");
return "register";
}
}
Now, create a Thymeleaf template for the registration form (register.html
):
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>User Registration</title>
</head>
<body>
<h2>User Registration</h2>
<form th:action="@{/register}" th:object="${user}" method="post">
<div>
<label for="name">Name:</label>
<input type="text" id="name" th:field="*{name}" required>
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" th:field="*{email}" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" th:field="*{password}" required>
</div>
<div>
<button type="submit">Register</button>
</div>
</form>
<p th:if="${successMessage}" th:text="${successMessage}"></p>
</body>
</html>
In this example, we use the th:object
attribute to bind the form to the user
object, and th:field
attributes to bind individual form fields to the object’s properties. The th:action
attribute ensures that the form is submitted to the correct endpoint, taking into account the application’s context path.
Integrating CSS and JavaScript with Thymeleaf
To create visually appealing and interactive web pages, you’ll need to integrate CSS and JavaScript into your Thymeleaf templates. Spring Boot makes this process straightforward by providing support for static resources.
1. Adding CSS
Place your CSS files in the src/main/resources/static/css
directory. You can then reference them in your Thymeleaf templates using the th:href
attribute:
<link rel="stylesheet" th:href="@{/css/styles.css}">
2. Adding JavaScript
Similarly, place your JavaScript files in the src/main/resources/static/js
directory and reference them using the th:src
attribute:
<script th:src="@{/js/script.js}"></script>
3. Using inline styles and scripts
Thymeleaf also allows you to use inline styles and scripts with dynamic content:
<style th:inline="css">
.highlight {
background-color: [[${highlightColor}]];
}
</style>
<script th:inline="javascript">
var username = [[${user.name}]];
console.log("Welcome, " + username);
</script>
Thymeleaf Layout Dialect
The Thymeleaf Layout Dialect is an extension that provides powerful layout and decoration features, allowing you to create reusable templates and maintain a consistent structure across your web pages. To use the Layout Dialect, add the following dependency to your pom.xml
file:
<dependency>
<groupId>nz.net.ultraq.thymeleaf</groupId>
<artifactId>thymeleaf-layout-dialect</artifactId>
</dependency>
Now, let’s create a basic layout that we can reuse across multiple pages:
- Create a
layout.html
file in thetemplates
directory:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org" xmlns:layout="http://www.ultraq.net.nz/thymeleaf/layout">
<head>
<meta charset="UTF-8">
<title layout:title-pattern="$LAYOUT_TITLE - $CONTENT_TITLE">My Application</title>
<link rel="stylesheet" th:href="@{/css/styles.css}">
</head>
<body>
<header>
<h1>My Application</h1>
<nav>
<ul>
<li><a th:href="@{/}">Home</a></li>
<li><a th:href="@{/about}">About</a></li>
<li><a th:href="@{/contact}">Contact</a></li>
</ul>
</nav>
</header>
<main layout:fragment="content">
<!-- Page-specific content will be inserted here -->
</main>
<footer>
<p>© 2023 My Application</p>
</footer>
<script th:src="@{/js/script.js}"></script>
</body>
</html>
- Create a page that uses this layout:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org" xmlns:layout="http://www.ultraq.net.nz/thymeleaf/layout" layout:decorate="~{layout}">
<head>
<title>Home</title>
</head>
<body>
<main layout:fragment="content">
<h2>Welcome to My Application</h2>
<p>This is the home page content.</p>
</main>
</body>
</html>
In this example, the layout:decorate
attribute specifies which layout to use, and the layout:fragment
attribute defines the content that will be inserted into the layout’s corresponding fragment.
Advanced Thymeleaf Techniques
As you become more comfortable with Thymeleaf, you can leverage some of its advanced features to create more sophisticated and maintainable templates. Let’s explore some of these techniques:
1. Custom Dialects
Thymeleaf allows you to create custom dialects, which can be used to extend its functionality and create reusable components. A custom dialect consists of one or more processors that define new attributes or tags.
Here’s an example of a simple custom dialect that adds a “capitalize” attribute:
import org.thymeleaf.context.ITemplateContext;
import org.thymeleaf.dialect.AbstractProcessorDialect;
import org.thymeleaf.engine.AttributeName;
import org.thymeleaf.model.IProcessableElementTag;
import org.thymeleaf.processor.element.AbstractAttributeTagProcessor;
import org.thymeleaf.processor.element.IElementTagStructureHandler;
import org.thymeleaf.standard.StandardDialect;
import org.thymeleaf.templatemode.TemplateMode;
public class CapitalizeDialect extends AbstractProcessorDialect {
public CapitalizeDialect() {
super("Capitalize Dialect", "cap", StandardDialect.PROCESSOR_PRECEDENCE);
}
@Override
public Set<IProcessor> getProcessors(String dialectPrefix) {
Set<IProcessor> processors = new HashSet<>();
processors.add(new CapitalizeAttributeProcessor(dialectPrefix));
return processors;
}
}
class CapitalizeAttributeProcessor extends AbstractAttributeTagProcessor {
private static final String ATTR_NAME = "capitalize";
public CapitalizeAttributeProcessor(String dialectPrefix) {
super(TemplateMode.HTML, dialectPrefix, null, false, ATTR_NAME, true, StandardDialect.PROCESSOR_PRECEDENCE, true);
}
@Override
protected void doProcess(ITemplateContext context, IProcessableElementTag tag, AttributeName attributeName,
String attributeValue, IElementTagStructureHandler structureHandler) {
String text = tag.getBody().toString();
structureHandler.setBody(text.toUpperCase(), false);
}
}
To use this custom dialect, you need to register it with the Thymeleaf engine:
@Configuration
public class ThymeleafConfig {
@Bean
public CapitalizeDialect capitalizeDialect() {
return new CapitalizeDialect();
}
}
Now you can use the custom attribute in your templates:
<p cap:capitalize>This text will be capitalized</p>
2. Expression Utility Objects
Thymeleaf provides several utility objects that you can use in expressions to perform common operations. Some of the most useful utility objects include:
#strings
: String manipulation utilities#numbers
: Number formatting utilities#dates
: Date and time utilities#lists
: Collection utilities#arrays
: Array utilities#objects
: Object utilities
Here’s an example of using these utility objects:
<p th:text="${#strings.capitalize(user.name)}">John Doe</p>
<p th:text="${#numbers.formatDecimal(product.price, 1, 2)}">10.00</p>
<p th:text="${#dates.format(order.date, 'dd-MM-yyyy')}">01-01-2023</p>
<p th:text="${#lists.size(items)}">5</p>
3. Inline Processing
Thymeleaf allows you to process expressions inline within text content using double square brackets ([[...]]
) or double parentheses ([(...)]
). This can be useful for creating dynamic text content without using additional attributes.
Example:
<p>Welcome, [[${user.name}]]! You have [(${user.messageCount})] new messages.</p>
4. Fragment Expressions
Fragment expressions allow you to define reusable parts of your templates. You can define fragments using the th:fragment
attribute and then include them in other templates using th:insert
, th:replace
, or th:include
.
Define a fragment:
<!-- fragments/header.html -->
<header th:fragment="pageHeader(title)">
<h1 th:text="${title}">Default Title</h1>
<nav>
<!-- Navigation menu items -->
</nav>
</header>
Use the fragment in another template:
<div th:replace="fragments/header :: pageHeader('Welcome')"></div>
Integrating Spring Boot and Thymeleaf with a Database
To create a fully functional web application, you’ll often need to integrate your Spring Boot and Thymeleaf setup with a database. Let’s look at an example using Spring Data JPA to interact with a database and display the results using Thymeleaf.
First, add the necessary dependencies to your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Next, create an entity class:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Getters and setters
}
Create a repository interface:
import org.springframework.data.jpa.repository.JpaRepository;
public interface ProductRepository extends JpaRepository<Product, Long> {
}
Update your controller to use the repository:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class ProductController {
@Autowired
private ProductRepository productRepository;
@GetMapping("/products")
public String listProducts(Model model) {
model.addAttribute("products", productRepository.findAll());
return "products";
}
}
Finally, create a Thymeleaf template to display the products:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Product List</title>
</head>
<body>
<h1>Product List</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>
<tr th:each="product : ${products}">
<td th:text="${product.id}">1</td>
<td th:text="${product.name}">Product Name</td>
<td th:text="${#numbers.formatDecimal(product.price, 1, 2)}">10.00</td>
</tr>
</tbody>
</table>
</body>
</html>
This example demonstrates how to retrieve data from a database using Spring Data JPA and display it in a Thymeleaf template.
Best Practices for Spring Boot and Thymeleaf Development
To ensure that your Spring Boot and Thymeleaf applications are maintainable, performant, and secure, consider following these best practices:
- Use layout templates: Implement a consistent layout across your application using Thymeleaf’s layout dialect to reduce code duplication and improve maintainability.
- Leverage Spring Boot’s auto-configuration: Take advantage of Spring Boot’s auto-configuration features to minimize manual configuration and streamline your development process.
- Implement proper error handling: Create custom error pages and implement proper exception handling to provide a better user experience and assist with debugging.
- Use Spring Security: Implement authentication and authorization using Spring Security to protect sensitive parts of your application.
- Optimize performance: Use Thymeleaf’s caching features and minimize the use of complex expressions in templates to improve rendering performance.
- Follow SOLID principles: Apply SOLID principles (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) to create more maintainable and extensible code.
- Implement proper logging: Use a logging framework like SLF4J with Logback to implement comprehensive logging throughout your application.
- Write unit and integration tests: Create thorough unit and integration tests to ensure the reliability and correctness of your application.
- Use environment-specific configuration: Leverage Spring Boot’s support for environment-specific configuration to easily manage different settings for development, testing, and production environments.
- Keep your dependencies up to date: Regularly update your project dependencies to benefit from bug fixes, security patches, and new features.
Conclusion
Spring Boot and Thymeleaf provide a powerful combination for creating dynamic web pages with ease and efficiency. By leveraging Spring Boot’s auto-configuration and Thymeleaf’s natural templating approach, developers can focus on building feature-rich web applications without getting bogged down in complex configurations.
Throughout this blog post, we’ve explored the fundamental concepts of both Spring Boot and Thymeleaf, as well as advanced techniques for creating sophisticated templates and integrating with databases. By following the best practices outlined here and continuing to explore the rich ecosystems of both technologies, you’ll be well-equipped to develop robust, maintainable, and scalable web applications.
As web development continues to evolve, the combination of Spring Boot and Thymeleaf remains a strong choice for Java developers looking to create dynamic and responsive web pages. With their active communities and ongoing development, both technologies are likely to remain relevant and powerful tools in the web development landscape for years to come.
Disclaimer: This blog post is intended for educational purposes only. While we strive to provide accurate and up-to-date information, technologies and best practices in the field of web development are constantly evolving. Readers are encouraged to consult official documentation and stay informed about the latest developments in Spring Boot and Thymeleaf. If you notice any inaccuracies in this post, please report them so we can correct them promptly.