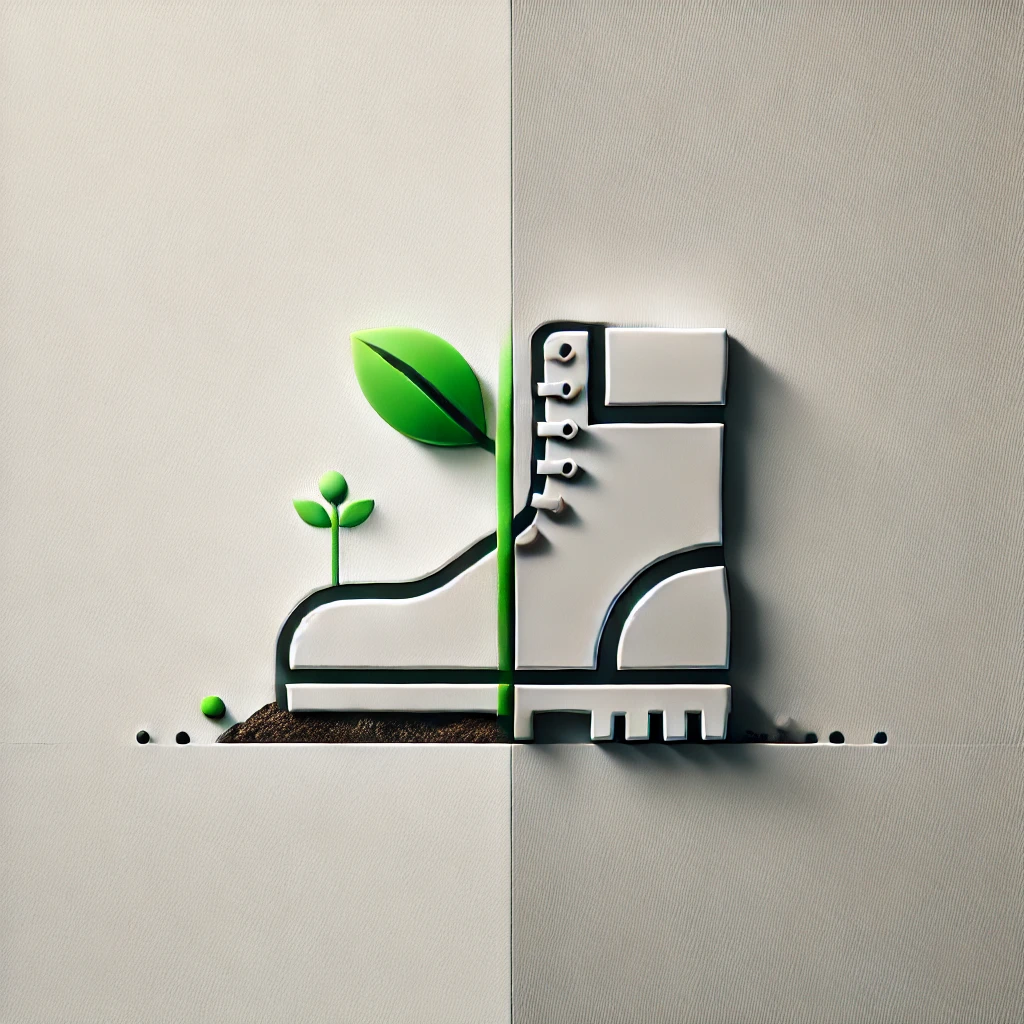
Spring Boot vs. Spring – Understanding the Key Differences
Spring Boot and Spring are two core frameworks within the Spring ecosystem, both designed to simplify Java development. However, they serve distinct purposes and have different capabilities, leading to widespread use across various types of applications. Understanding their differences is crucial for developers when deciding which one to use for specific scenarios.
In this blog, we will explore the key differences between Spring Boot and Spring, focusing on their unique characteristics, features, and ideal use cases. We will also provide clear examples and comparisons to help guide your decisions effectively.
What is Spring?
Spring is a comprehensive Java-based framework that simplifies enterprise Java development by providing various features, such as Dependency Injection (DI), Aspect-Oriented Programming (AOP), transaction management, and more. It offers a wide range of tools and libraries to create flexible, modular, and testable applications.
Spring allows developers to use POJOs (Plain Old Java Objects), which means you can build enterprise-level Java applications without requiring heavy enterprise frameworks like Enterprise JavaBeans (EJB).
Core Features of Spring:
- Dependency Injection (DI): A technique that allows the development of loosely coupled code.
- Aspect-Oriented Programming (AOP): Helps manage cross-cutting concerns like logging and security.
- Transaction Management: Ensures that data integrity is maintained, especially in multi-threaded applications.
- Data Access Integration: Supports JDBC, Hibernate, JPA, and other data access frameworks.
- Testing: Provides excellent integration with testing frameworks like JUnit.
Code Example: Traditional Spring Configuration
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="myService" class="com.example.MyService">
<property name="repository" ref="myRepository" />
</bean>
<bean id="myRepository" class="com.example.MyRepository" />
</beans>
In traditional Spring applications, XML configurations like this one were commonly used. Over time, Spring evolved to offer annotation-based configurations to simplify this process.
What is Spring Boot?
Spring Boot, on the other hand, is an extension of the Spring framework designed to simplify the development of production-ready applications by providing default configurations. It allows developers to focus on the application’s functionality rather than dealing with the complexities of Spring configuration.
Spring Boot follows the “Convention over Configuration” philosophy, making it easier to start a project with minimal configuration. With Spring Boot, you can set up a fully running Spring application quickly, thanks to features like an embedded server (Tomcat, Jetty, or Undertow), auto-configuration, and starter dependencies.
Core Features of Spring Boot:
- Auto-Configuration: Automatically configures Spring components based on classpath settings, properties, and other factors.
- Starter Dependencies: Pre-defined dependencies that simplify build configuration (e.g.,
spring-boot-starter-web
,spring-boot-starter-data-jpa
). - Embedded Servers: Bundles Tomcat, Jetty, or Undertow, removing the need to deploy WAR files separately.
- Actuator: Provides production-ready features such as monitoring and health checks.
- Externalized Configuration: Easily manages configuration properties using
application.properties
orapplication.yml
.
Code Example: Spring Boot Application
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In this example, the @SpringBootApplication
annotation enables several features like component scanning, autoconfiguration, and allows the application to run on an embedded server.
Key Differences Between Spring and Spring Boot
While both Spring and Spring Boot share the same core Spring features, they are different in how they approach application development. Below, we explore their differences in greater detail.
Aspect | Spring | Spring Boot |
---|---|---|
Purpose | General-purpose framework for building any Java app. | Framework to simplify Spring application development. |
Configuration | Requires extensive XML or Java-based configurations. | Auto-configuration with convention over configuration. |
Embedded Server | Requires external server setup (Tomcat, Jetty). | Comes with embedded server (Tomcat, Jetty, Undertow). |
Dependencies | Manually manage dependencies. | Provides starter dependencies to simplify management. |
Deployment | Typically packaged as WAR and deployed on external server. | Packaged as JAR, includes embedded server for direct execution. |
Startup Time | Can take longer due to extensive configurations. | Faster startup due to auto-configuration and embedded server. |
Production Features | Requires manual setup for monitoring, health checks, etc. | Includes Actuator for out-of-the-box production features. |
Deep Dive into the Differences
1. Configuration Approach
Spring: Traditional Spring applications often require a lot of manual configuration. XML files or Java-based annotations like @Configuration
, @ComponentScan
, and @Bean
are used to wire up beans and set up the application context.
For example:
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
Spring Boot: Spring Boot significantly reduces the configuration burden by using auto-configuration. By including specific libraries in the classpath, Spring Boot automatically configures beans based on available dependencies.
For example:
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
In this case, Spring Boot detects web dependencies on the classpath and automatically configures web-related components such as Tomcat and Spring MVC.
2. Dependency Management
Spring: Developers have full control over dependencies. This means they must include the necessary libraries and configure them individually, which can sometimes lead to version conflicts.
For example, a typical Maven dependency for Spring MVC:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.10</version>
</dependency>
Spring Boot: Spring Boot simplifies dependency management with the use of starter dependencies. Starters are pre-configured sets of dependencies for different functionalities, such as web, JPA, or security.
For example, the Maven dependency for a Spring Boot web project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Spring Boot’s starters eliminate the need to explicitly define versions for Spring dependencies, and they ensure compatibility between different modules.
3. Embedded Server
Spring: Traditional Spring applications require you to set up an external server like Tomcat or Jetty. You need to package your application as a WAR and deploy it to the server.
Spring Boot: Spring Boot includes an embedded server by default, allowing developers to package their application as a JAR and run it independently. This embedded server approach simplifies deployment and reduces configuration complexity.
For instance, with Spring Boot, you can start your application using a simple command:
mvn spring-boot:run
This makes development faster and reduces the time needed to deploy and test your application in different environments.
4. Production-Ready Features
Spring: Out-of-the-box, Spring does not offer many features specifically geared toward production environments. Features like health checks, metrics, and logging need to be manually integrated.
Spring Boot: Spring Boot provides a production-ready set of features through Spring Boot Actuator. Actuator includes endpoints for monitoring, health checks, and metrics that allow you to easily manage your application in production.
For example, you can expose a health endpoint in Spring Boot with Actuator:
management:
endpoints:
web:
exposure:
include: health
This will provide a /actuator/health
endpoint, which reports on the application’s health status.
Which One Should You Choose?
The decision to use Spring or Spring Boot depends on your project requirements and your team’s experience with the Spring framework.
- Use Spring if:
- You need fine-grained control over your application’s configuration.
- Your project requires customization beyond what Spring Boot offers out-of-the-box.
- You are working on a legacy project or enterprise system where you need full control over the setup.
- Use Spring Boot if:
- You need to develop a Spring-based application quickly without dealing with complex configuration.
- Your project is relatively small to medium in size and would benefit from an embedded server.
- You need out-of-the-box production features like health monitoring, metrics, or logging.
Spring and Spring Boot in Practice
To illustrate when to use Spring vs. Spring Boot, let’s look at two real-world scenarios:
Scenario 1: Building a Microservice
If you are developing a microservice that needs to be lightweight, run independently, and have minimal configuration, Spring Boot is the ideal choice. It allows rapid setup and deployment with minimal fuss. The embedded server, along with Spring Boot’s auto-configuration, simplifies microservice architecture development.
Scenario 2: Building an Enterprise-Level Application
For large, enterprise-level applications that require complex configurations, multiple layers of security, or integration with multiple external services, Spring (without Boot) might be a better fit. It gives you complete control over how the application is structured, how transactions are managed, and how data is processed.
Conclusion
Spring and Spring Boot are both powerful tools in the Java ecosystem, but they cater to different needs. Spring offers full control over your application, making it suitable for complex, large-scale projects. Spring Boot, on the other hand, simplifies development and configuration, making it ideal for building microservices or small-to-medium applications.
The choice between Spring and Spring Boot ultimately comes down to the complexity and requirements of your project. While Spring offers more customization, Spring Boot provides simplicity and speed, enabling developers to create production-ready applications with minimal effort.
Regardless of your choice, both frameworks are robust, widely used, and capable of supporting enterprise-level development needs.
Disclaimer: This blog aims to provide an accurate comparison based on available information. Please report any inaccuracies so we can correct them promptly.