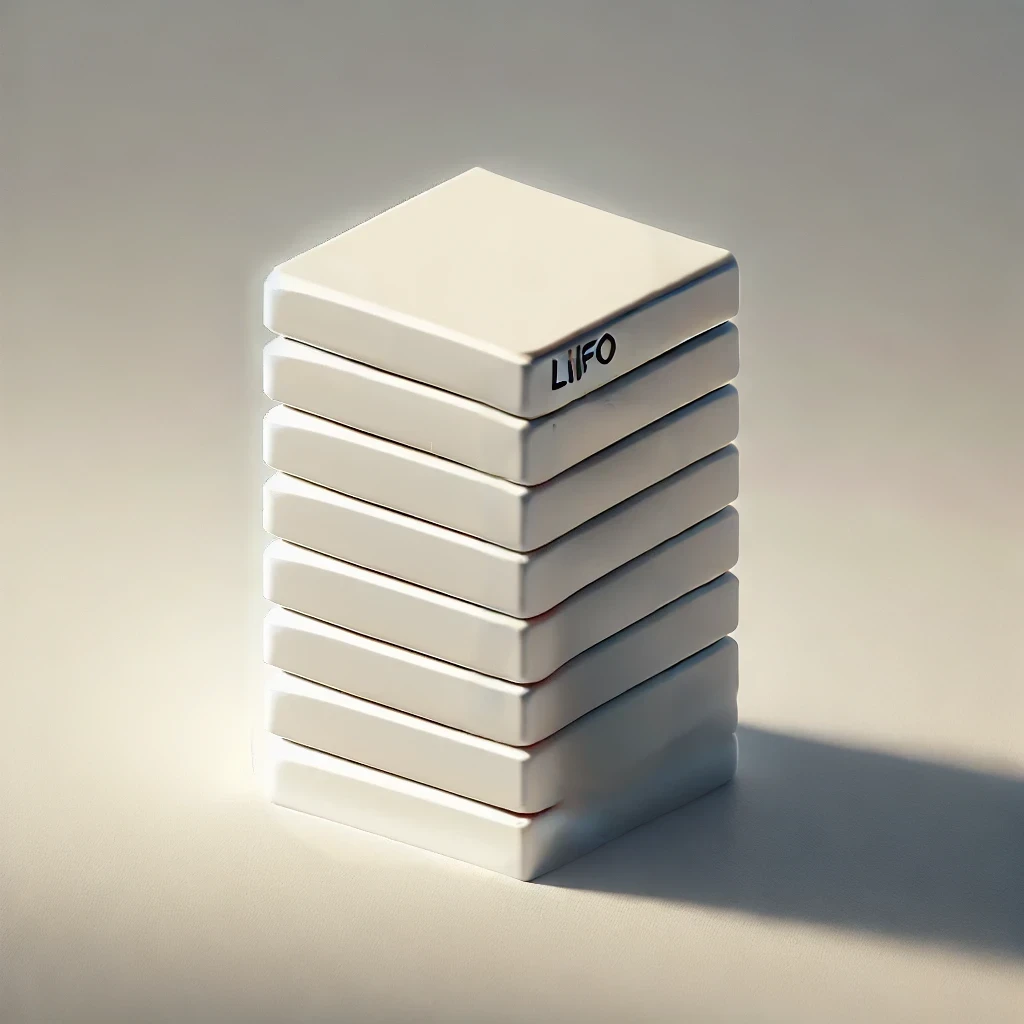
Stacks in Java: The LIFO Principle Made Easy
Have you ever wondered how your browser’s back button works? Or how undo functionality is implemented in your favorite text editor? These seemingly simple features rely on a fundamental data structure called a stack. In the world of computer science and programming, stacks play a crucial role in solving various problems efficiently. Today, we’re diving deep into the concept of stacks in Java, exploring their inner workings, and uncovering their practical applications. Whether you’re a beginner programmer or an experienced developer looking to refresh your knowledge, this blog post will equip you with a solid understanding of stacks and their implementation in Java. So, grab your favorite beverage, get comfortable, and let’s embark on this exciting journey through the world of stacks!
What is a Stack? Understanding the LIFO Principle
Defining the Stack Data Structure
At its core, a stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. Imagine a stack of books on your desk. When you add a new book, you place it on top of the pile. When you want to remove a book, you take the one from the top. This simple analogy perfectly illustrates how a stack operates in the world of programming. In a stack, elements are added and removed from the same end, typically referred to as the “top” of the stack. This behavior gives rise to two primary operations: push (to add an element) and pop (to remove an element). The LIFO principle ensures that the last element added to the stack is always the first one to be removed, creating a predictable and efficient way of managing data.
The LIFO Principle in Action
To better understand the LIFO principle, let’s consider a real-world scenario. Think about a stack of clean plates in a cafeteria. As new plates are washed and dried, they’re added to the top of the stack. When a customer needs a plate, they take it from the top of the stack. This ensures that the most recently cleaned plates are used first, while older plates gradually make their way to the top. In programming, this principle is particularly useful for managing function calls, tracking state changes, and implementing algorithms that require backtracking or reversal of operations. The LIFO nature of stacks makes them an ideal choice for scenarios where you need to keep track of the most recent data or actions, with the ability to quickly access and remove the latest entry.
Implementing Stacks in Java: From Theory to Practice
Java’s Built-in Stack Class
Java provides a built-in Stack
class as part of the java.util
package. This class extends the Vector
class and provides a convenient way to work with stacks in your Java programs. While the Stack
class is still supported for backward compatibility, it’s worth noting that it’s considered somewhat outdated, and for most modern applications, you might want to consider using more flexible alternatives. Nevertheless, let’s take a look at how to use the Stack
class to get a feel for stack operations:
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
Stack<String> bookStack = new Stack<>();
// Pushing elements onto the stack
bookStack.push("Java Programming");
bookStack.push("Data Structures");
bookStack.push("Algorithms");
System.out.println("Stack: " + bookStack);
// Peeking at the top element
System.out.println("Top book: " + bookStack.peek());
// Popping an element from the stack
String removedBook = bookStack.pop();
System.out.println("Removed book: " + removedBook);
System.out.println("Updated stack: " + bookStack);
// Checking if the stack is empty
System.out.println("Is stack empty? " + bookStack.isEmpty());
}
}
In this example, we create a stack of books, push some titles onto it, peek at the top element, pop an element, and check if the stack is empty. This demonstrates the basic operations you can perform with Java’s built-in Stack
class.
Implementing a Custom Stack
While the built-in Stack
class is convenient, implementing your own stack can provide a deeper understanding of how this data structure works. Let’s create a simple generic stack implementation using an array:
public class CustomStack<T> {
private static final int DEFAULT_CAPACITY = 10;
private Object[] elements;
private int size;
public CustomStack() {
elements = new Object[DEFAULT_CAPACITY];
}
public void push(T item) {
ensureCapacity();
elements[size++] = item;
}
@SuppressWarnings("unchecked")
public T pop() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
T item = (T) elements[--size];
elements[size] = null; // Help garbage collection
return item;
}
@SuppressWarnings("unchecked")
public T peek() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
return (T) elements[size - 1];
}
public boolean isEmpty() {
return size == 0;
}
public int size() {
return size;
}
private void ensureCapacity() {
if (size == elements.length) {
int newCapacity = elements.length * 2;
elements = Arrays.copyOf(elements, newCapacity);
}
}
}
This custom implementation provides the core functionality of a stack, including push
, pop
, peek
, isEmpty
, and size
methods. It uses an array to store elements and dynamically resizes when necessary. By implementing your own stack, you gain a deeper appreciation for the inner workings of this data structure and can tailor it to your specific needs.
Common Operations and Time Complexity
Push Operation
The push operation adds an element to the top of the stack. In our custom implementation, this is achieved by simply adding the element to the end of the array and incrementing the size. The time complexity of the push operation is typically O(1), meaning it takes constant time regardless of the stack’s size. However, in cases where the underlying array needs to be resized, the operation can occasionally take O(n) time, where n is the number of elements in the stack.
Pop Operation
Popping an element removes and returns the top item from the stack. This operation decrements the size and returns the last element. Like push, the pop operation generally has a time complexity of O(1). It’s important to note that popping from an empty stack should be handled carefully, usually by throwing an exception or returning a special value to indicate the error condition.
Peek Operation
The peek operation allows you to view the top element of the stack without removing it. This is useful when you need to inspect the most recently added item without modifying the stack’s state. Peek also has a time complexity of O(1), as it simply returns the last element in the array without any additional processing.
IsEmpty and Size Operations
Checking if a stack is empty (isEmpty
) and getting its current size (size
) are both O(1) operations. These methods provide quick ways to inspect the stack’s state without traversing its contents.
Practical Applications of Stacks in Java Programming
Function Call Management
One of the most fundamental uses of stacks in programming is managing function calls. When a function is called, information about the call (such as local variables and the return address) is pushed onto a call stack. When the function returns, this information is popped off the stack. This mechanism allows for efficient handling of nested function calls and recursion. Let’s look at a simple example of how a stack might be used to simulate function call management:
import java.util.Stack;
public class FunctionCallSimulator {
private static class FunctionCall {
String functionName;
int localVariable;
FunctionCall(String name, int var) {
functionName = name;
localVariable = var;
}
@Override
public String toString() {
return functionName + " (local var: " + localVariable + ")";
}
}
public static void main(String[] args) {
Stack<FunctionCall> callStack = new Stack<>();
// Simulating function calls
callStack.push(new FunctionCall("main", 0));
System.out.println("Calling main(): " + callStack);
callStack.push(new FunctionCall("functionA", 5));
System.out.println("Calling functionA(): " + callStack);
callStack.push(new FunctionCall("functionB", 10));
System.out.println("Calling functionB(): " + callStack);
// Simulating function returns
callStack.pop();
System.out.println("Returning from functionB(): " + callStack);
callStack.pop();
System.out.println("Returning from functionA(): " + callStack);
callStack.pop();
System.out.println("Returning from main(): " + callStack);
}
}
This example demonstrates how a stack can be used to keep track of function calls, including local variables. As functions are called, they’re pushed onto the stack, and as they return, they’re popped off, mimicking the behavior of a real call stack.
Expression Evaluation
Stacks are instrumental in evaluating mathematical expressions, particularly when dealing with infix, postfix, or prefix notations. For instance, converting an infix expression (e.g., “3 + 4 * 2”) to postfix notation (e.g., “3 4 2 * +”) can be efficiently done using a stack. Let’s implement a simple infix to postfix converter:
import java.util.Stack;
public class InfixToPostfixConverter {
public static String infixToPostfix(String infix) {
StringBuilder postfix = new StringBuilder();
Stack<Character> operatorStack = new Stack<>();
for (char c : infix.toCharArray()) {
if (Character.isLetterOrDigit(c)) {
postfix.append(c);
} else if (c == '(') {
operatorStack.push(c);
} else if (c == ')') {
while (!operatorStack.isEmpty() && operatorStack.peek() != '(') {
postfix.append(operatorStack.pop());
}
if (!operatorStack.isEmpty() && operatorStack.peek() == '(') {
operatorStack.pop();
}
} else {
while (!operatorStack.isEmpty() && precedence(c) <= precedence(operatorStack.peek())) {
postfix.append(operatorStack.pop());
}
operatorStack.push(c);
}
}
while (!operatorStack.isEmpty()) {
postfix.append(operatorStack.pop());
}
return postfix.toString();
}
private static int precedence(char operator) {
switch (operator) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
}
return -1;
}
public static void main(String[] args) {
String infix = "a+b*(c^d-e)^(f+g*h)-i";
System.out.println("Infix expression: " + infix);
System.out.println("Postfix expression: " + infixToPostfix(infix));
}
}
This example showcases how a stack can be used to convert an infix expression to postfix notation, which is easier for computers to evaluate. The stack helps manage operator precedence and parentheses, ensuring the correct order of operations in the resulting postfix expression.
Advanced Stack Concepts: Balancing Act and Two-Stack Queues
Balancing Parentheses and Brackets
One classic problem that beautifully demonstrates the power of stacks is checking for balanced parentheses and brackets in an expression. This problem is commonly encountered in code editors and compilers to ensure proper syntax. Let’s implement a solution using a stack:
import java.util.Stack;
public class BalancedBracketChecker {
public static boolean isBalanced(String expression) {
Stack<Character> stack = new Stack<>();
for (char c : expression.toCharArray()) {
if (c == '(' || c == '[' || c == '{') {
stack.push(c);
} else if (c == ')' || c == ']' || c == '}') {
if (stack.isEmpty()) {
return false;
}
char top = stack.pop();
if ((c == ')' && top != '(') ||
(c == ']' && top != '[') ||
(c == '}' && top != '{')) {
return false;
}
}
}
return stack.isEmpty();
}
public static void main(String[] args) {
String[] expressions = {
"((()))",
"({[]})",
"(()",
"({[}])",
""
};
for (String expr : expressions) {
System.out.println("Expression: " + expr);
System.out.println("Is balanced: " + isBalanced(expr));
System.out.println();
}
}
}
This implementation uses a stack to keep track of opening brackets. When a closing bracket is encountered, it’s checked against the most recent opening bracket (the top of the stack). If they match, the opening bracket is popped off the stack. The expression is considered balanced if all brackets are matched and the stack is empty at the end.
Implementing a Queue Using Two Stacks
While stacks follow the LIFO principle, queues operate on a First-In-First-Out (FIFO) basis. Interestingly, we can implement a queue using two stacks, showcasing the versatility of the stack data structure. Here’s how we can do it:
import java.util.Stack;
public class QueueUsingTwoStacks<T> {
private Stack<T> inbox = new Stack<>();
private Stack<T> outbox = new Stack<>();
public void enqueue(T item) {
inbox.push(item);
}
public T dequeue() {
if (outbox.isEmpty()) {
while (!inbox.isEmpty()) {
outbox.push(inbox.pop());
}
}
if (outbox.isEmpty()) {
throw new IllegalStateException("Queue is empty");
}
return outbox.pop();
}
public T peek() {
if (outbox.isEmpty()) {
while (!inbox.isEmpty()) {
outbox.push(inbox.pop());
}
}
if (outbox.isEmpty()) {
throw new IllegalStateException("Queue is empty");
}
return outbox.peek();
}
public boolean isEmpty() {
return inbox.isEmpty() && outbox.isEmpty();
}
public int size() {
return inbox.size() + outbox.size();
}
public static void main(String[] args) {
QueueUsingTwoStacks<Integer> queue = new QueueUsingTwoStacks<>();
queue.enqueue(1);
queue.enqueue(2);
queue.enqueue(3);
System.out.println("Dequeued: " + queue.dequeue());
System.out.println("Peek: " + queue.peek());
queue.enqueue(4);
while (!queue.isEmpty()) {
System.out.println("Dequeued: " + queue.dequeue());
}
}
}
This implementation uses two stacks: an “inbox” for enqueuing elements and an “outbox” for dequeuing. When dequeuing or peeking, if the outbox is empty, all elements from the inbox are moved to the outbox, effectively reversing their order. This clever use of two stacks allows us to achieve queue-like behavior, demonstrating the flexibility and power of the stack data structure.
Best Practices and Performance Considerations
Choosing the Right Implementation
When working with stacks in Java, it’s important to choose the right implementation for your specific needs. While the built-in Stack
class is available, it’s generally recommended to use ArrayDeque
as a more efficient and flexible alternative. ArrayDeque
can be used as both a stack and a queue, and it offers better performance characteristics. Here’s a quick comparison:
import java.util.ArrayDeque;
import java.util.Stack;
public class StackPerformanceComparison {
public static void main(String[] args) {
int iterations = 1000000;
// Using Stack
long startTime = System.nanoTime();
Stack<Integer> stack = new Stack<>();
for (int i = 0; i < iterations; i++) {
stack.push(i);
}
for (int i = 0; i < iterations; i++) {
stack.pop();
}
long endTime = System.nanoTime();
System.out.println("Stack time: " + (endTime - startTime) / 1000000 + " ms");
// Using ArrayDeque as a stack
startTime = System.nanoTime();
ArrayDeque<Integer> deque = new ArrayDeque<>();
for (int i = 0; i < iterations; i++) {
deque.push(i);
}
for (int i = 0; i < iterations; i++) {
deque.pop();
}
endTime = System.nanoTime();
System.out.println("ArrayDeque time: " + (endTime - startTime) / 1000000 + " ms");
}
}
This comparison typically shows that `ArrayDeque` performs better than `Stack` for large numbers of operations. The `ArrayDeque` class is not synchronized, making it more efficient for single-threaded applications. If you need thread-safety, you can use `Collections.synchronizedDeque()` to create a synchronized version of `ArrayDeque`. **Memory Management and Efficiency** When implementing your own stack or using built-in classes, it’s crucial to consider memory management. Here are some tips to ensure efficient use of memory:
- Proper sizing: If you know the approximate size of your stack in advance, initialize it with that capacity to avoid unnecessary resizing operations.
2. Clearing references: When popping elements from a stack, especially if they’re objects, it’s good practice to set the reference to null to help the garbage collector. This is particularly important if you’re implementing your own stack:
public T pop() {
if (isEmpty()) {
throw new EmptyStackException();
}
T item = (T) elements[--size];
elements[size] = null; // Clear the reference
return item;
}
3. Using primitive types: When possible, use primitive types instead of their wrapper classes to reduce memory overhead. For example, use `int` instead of `Integer` if you’re storing numbers.
4. Avoiding unnecessary object creation: Be mindful of creating unnecessary objects within stack operations, especially in frequently called methods.
Common Pitfalls and How to Avoid Them
Stack Overflow
One of the most common issues when working with stacks is stack overflow. This occurs when you try to push more elements onto the stack than it can hold. To prevent this
1. Use a dynamically resizing implementation (like `ArrayList` or `ArrayDeque`).
2. If using a fixed-size stack, always check for available space before pushing:
public void push(T item) {
if (size >= capacity) {
throw new StackOverflowError("Stack is full");
}
elements[size++] = item;
}
Underflow Conditions
Stack underflow occurs when you try to pop or peek at an empty stack. Always check for empty stacks before performing these operations:
public T pop() {
if (isEmpty()) {
throw new EmptyStackException();
}
// … rest of pop implementation
}
public T peek() {
if (isEmpty()) {
throw new EmptyStackException();
}
// … rest of peek implementation
}
Misusing Stack Operations
Ensure you’re using stack operations correctly. For example, don’t use `get(index)` to access elements in the middle of the stack, as this violates the LIFO principle and can lead to unexpected behavior.
Real-world Applications: Beyond the Basics
Undo/Redo Functionality
Stacks are perfect for implementing undo/redo functionality in applications. Each action is pushed onto an undo stack, and when the user wants to undo, the action is popped and reversed. Here’s a simple example:
public class UndoRedoManager {
private Stack undoStack = new Stack<>();
private Stack redoStack = new Stack<>();
public void performAction(String action) {
undoStack.push(action);
redoStack.clear(); // Clear redo stack when a new action is performed
System.out.println("Performed: " + action);
}
public void undo() {
if (!undoStack.isEmpty()) {
String action = undoStack.pop();
redoStack.push(action);
System.out.println("Undone: " + action);
} else {
System.out.println("Nothing to undo");
}
}
public void redo() {
if (!redoStack.isEmpty()) {
String action = redoStack.pop();
undoStack.push(action);
System.out.println("Redone: " + action);
} else {
System.out.println("Nothing to redo");
}
}
public static void main(String[] args) {
UndoRedoManager manager = new UndoRedoManager();
manager.performAction("Type 'Hello'");
manager.performAction("Type ' World'");
manager.undo();
manager.undo();
manager.redo();
}
}
This example demonstrates a basic undo/redo system using two stacks. It’s a simplified version of what you might find in text editors or graphic design software.
Depth-First Search in Graph Traversal
Stacks are fundamental in implementing depth-first search (DFS) algorithms for graph traversal. Here’s a simple example of DFS using a stack:
import java.util.*;
public class DepthFirstSearch {
private static class Graph {
private int vertices;
private LinkedList[] adjacencyList;
Graph(int v) {
vertices = v;
adjacencyList = new LinkedList[vertices];
for (int i = 0; i < vertices; i++) {
adjacencyList[i] = new LinkedList<>();
}
}
void addEdge(int v, int w) {
adjacencyList[v].add(w);
}
void DFS(int startVertex) {
boolean[] visited = new boolean[vertices];
Stack<Integer> stack = new Stack<>();
stack.push(startVertex);
while (!stack.isEmpty()) {
int vertex = stack.pop();
if (!visited[vertex]) {
System.out.print(vertex + " ");
visited[vertex] = true;
}
for (int adjacentVertex : adjacencyList[vertex]) {
if (!visited[adjacentVertex]) {
stack.push(adjacentVertex);
}
}
}
}
}
public static void main(String[] args) {
Graph graph = new Graph(4);
graph.addEdge(0, 1);
graph.addEdge(0, 2);
graph.addEdge(1, 2);
graph.addEdge(2, 0);
graph.addEdge(2, 3);
graph.addEdge(3, 3);
System.out.println("Depth First Traversal (starting from vertex 2):");
graph.DFS(2);
}
}
This implementation uses a stack to keep track of vertices to visit next, demonstrating how stacks can be used in graph algorithms.
Conclusion
As we’ve explored throughout this blog post, stacks are a fundamental data structure with a wide range of applications in Java programming. From managing function calls to implementing complex algorithms, the simplicity and efficiency of the LIFO principle make stacks an indispensable tool in a developer’s arsenal. By understanding the core concepts, operations, and best practices associated with stacks, you’ve taken a significant step towards becoming a more proficient Java programmer.
Remember, the key to mastering stacks lies in practice and application. Try implementing your own stack, experiment with different use cases, and always consider whether a stack might be the right solution for the problem at hand. As you continue your journey in Java development, you’ll find that a solid grasp of data structures like stacks will serve you well in crafting efficient, elegant solutions to complex programming challenges.
So, the next time you’re faced with a problem that involves managing data in a last-in, first-out manner, don’t hesitate to reach for your newfound knowledge of stacks. Happy coding!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information and code examples presented in this blog post, they are provided “as is” without warranty of any kind. The author and publisher do not assume any responsibility or liability for any errors or omissions. Readers are encouraged to test and verify the code in their own environments before using it in production. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.