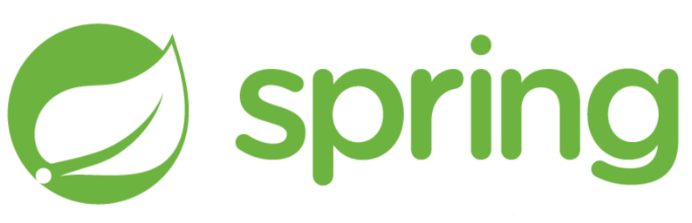
Step By Step: Creating a Basic Spring Boot Application
1. Prerequisites:
- Java Development Kit (JDK) 8 or newer.
- Maven (if not using Spring Initializr or if you prefer Maven).
- An Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or VSCode with the Spring Boot Extension.
2. Initialize a New Project:
Using Spring Initializr:
- Visit Spring Initializr.
- Choose your project type: Maven or Gradle.
- Choose your language: Java, Kotlin, or Groovy.
- Fill in the “Project Metadata”.
- Select required dependencies. For a web application, choose “Spring Web”.
- Click on “Generate” and download the zip file.
- Extract the zip file to your workspace.
Using Spring Boot CLI (alternative method):
spring init --dependencies=web my-springboot-app
3. Importing Project in IDE:
Open your preferred IDE and import the project as a Maven or Gradle project.
4. Project Structure:
Your project will have a default structure. The main parts are:
src/main/java
: Contains the Java source files.src/main/resources
: Contains resources like properties files, static resources, templates, etc.src/test/java
: Contains test cases.
5. Creating a Simple REST Endpoint:
Navigate to src/main/java
and locate the main class named after your project.
Add a new REST Controller:
package com.example.myspringbootapp;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, Spring Boot!";
}
}
6. Configuring application.properties:
In src/main/resources/application.properties
, you can add configuration properties. For this basic setup, we’re leaving it empty, but you might typically set server ports, database connections, etc., here.
7. Running the Application:
Using IDE:
Right-click the main class (@SpringBootApplication
annotated) > Run
Using Maven:
mvn spring-boot:run
8. Accessing the Endpoint:
Once the application is running, open a browser and go to http://localhost:8080/hello
. You should see the message “Hello, Spring Boot!”.
9. Building and Packaging:
To create a standalone JAR with all the dependencies:
mvn clean package
Then, you can run your application with:
java -jar target/myspringbootapp-0.0.1-SNAPSHOT.jar
10. Next Steps:
- Add more dependencies like JPA, Thymeleaf, or Security based on your requirements.
- Connect to databases.
- Implement CRUD operations.
- Add exception handling.
- Write unit and integration tests.
Congratulations, you’ve now created a basic Spring Boot application! As you delve deeper, you’ll find that Spring Boot offers many tools and utilities to simplify most of the common tasks associated with building, testing, and deploying Java applications.