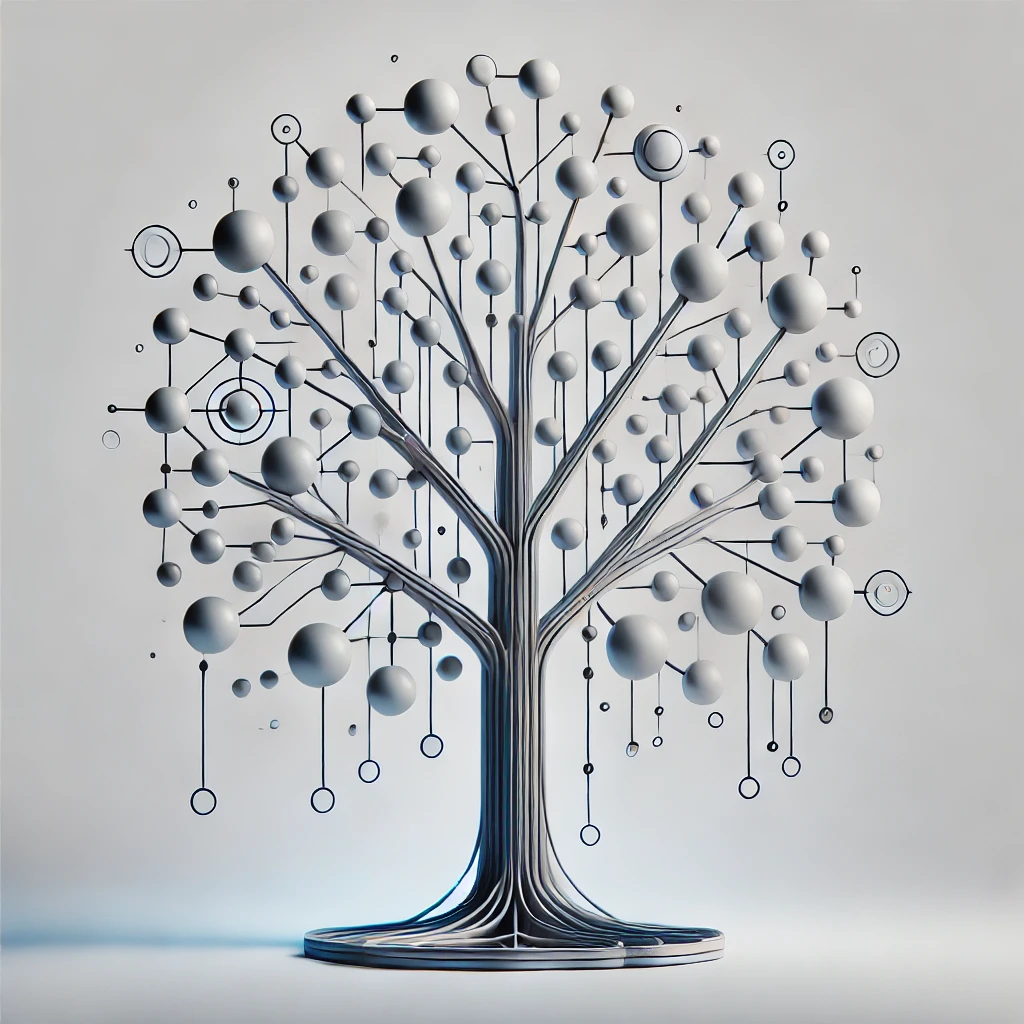
Taming Trees: A Beginner’s Guide to Java Tree Structures
Welcome, fellow code enthusiasts! Today, we’re embarking on an exciting journey into the lush forest of Java tree structures. Don’t worry if you’re feeling a bit lost in the woods – we’ll be your trusty guide, helping you navigate through the dense foliage of nodes, branches, and leaves. Trees aren’t just for nature lovers anymore; they’re a fundamental part of computer science and a crucial tool in any Java developer’s toolkit. Whether you’re a coding sapling just starting to sprout or a seasoned programmer looking to branch out into new territory, this guide will help you cultivate a deep understanding of tree structures in Java. So, grab your virtual axe (or should we say, IDE?), and let’s start chopping away at the complexities of trees!
What Are Tree Structures, Anyway?
Defining the roots of our knowledge
Before we climb too high, let’s plant our feet firmly on the ground and define what we mean by tree structures. In the world of computer science, a tree is a hierarchical data structure that consists of nodes connected by edges. Each tree starts with a single node called the root, which branches out to other nodes, creating parent-child relationships. These child nodes can have their own children, and so on, forming a structure that resembles an upside-down tree (hence the name).
Trees are incredibly versatile and find applications in various domains, from representing file systems and organizational hierarchies to optimizing database queries and powering search algorithms. They provide an efficient way to store and retrieve data, especially when dealing with hierarchical relationships. In Java, we can implement tree structures using classes and objects, allowing us to create powerful and flexible data management systems.
Why should Java developers care about trees?
Now, you might be wondering, “Why should I bother learning about tree structures when I can just use arrays or lists?” Well, my curious friend, trees offer several advantages that make them indispensable in certain situations. First, they provide a natural way to represent hierarchical data, making it easier to model real-world relationships. Second, many tree-based data structures, like binary search trees, offer efficient searching, insertion, and deletion operations, often with time complexities of O(log n) for balanced trees. Lastly, understanding tree structures opens up a world of advanced algorithms and data structures, such as heaps, tries, and balanced trees like AVL and Red-Black trees.
As a Java developer, mastering tree structures will not only expand your problem-solving toolkit but also prepare you for tackling complex coding challenges and optimizing your applications. So, let’s roll up our sleeves and dig into the nitty-gritty of implementing tree structures in Java!
Building Your First Tree: The Basic Node Class
Laying the groundwork
To start our arboreal adventure, we need to create the fundamental building block of any tree structure: the Node class. In its simplest form, a node contains some data and references to its child nodes. Let’s create a basic Node class that we’ll use as the foundation for our tree implementations:
public class Node<T> {
private T data;
private List<Node<T>> children;
public Node(T data) {
this.data = data;
this.children = new ArrayList<>();
}
public void addChild(Node<T> child) {
children.add(child);
}
public void removeChild(Node<T> child) {
children.remove(child);
}
public List<Node<T>> getChildren() {
return children;
}
public T getData() {
return data;
}
public void setData(T data) {
this.data = data;
}
}
This Node class is generic, allowing us to create trees that can hold any type of data. It contains a data field to store the node’s value, and a list of child nodes. We’ve also included methods to add and remove child nodes, as well as getters and setters for the data.
Creating a simple tree
Now that we have our Node class, let’s create a simple tree structure to see how it all fits together:
public class SimpleTree {
public static void main(String[] args) {
Node<String> root = new Node<>("Root");
Node<String> child1 = new Node<>("Child 1");
Node<String> child2 = new Node<>("Child 2");
Node<String> child3 = new Node<>("Child 3");
root.addChild(child1);
root.addChild(child2);
root.addChild(child3);
Node<String> grandchild1 = new Node<>("Grandchild 1");
Node<String> grandchild2 = new Node<>("Grandchild 2");
child1.addChild(grandchild1);
child1.addChild(grandchild2);
// Print the tree structure
printTree(root, 0);
}
private static void printTree(Node<String> node, int level) {
String indent = " ".repeat(level);
System.out.println(indent + node.getData());
for (Node<String> child : node.getChildren()) {
printTree(child, level + 1);
}
}
}
This example creates a simple tree with a root node, three child nodes, and two grandchild nodes. We’ve also included a printTree
method that uses recursion to display the tree structure. When you run this code, you’ll see the following output:
Root
Child 1
Grandchild 1
Grandchild 2
Child 2
Child 3
Congratulations! You’ve just created and traversed your first tree structure in Java. This simple example demonstrates the basic concepts of parent-child relationships and how nodes can be connected to form a hierarchical structure.
Traversing the Forest: Tree Traversal Algorithms
Exploring different paths
Now that we’ve planted our first tree, it’s time to learn how to navigate through its branches. Tree traversal is the process of visiting each node in a tree data structure, exactly once, in a systematic way. There are several traversal algorithms, each with its own unique approach to exploring the tree. The three most common traversal methods are:
- Pre-order traversal
- In-order traversal
- Post-order traversal
Let’s implement these traversal methods in our SimpleTree class to see how they work:
public class SimpleTree {
// ... (previous code remains the same)
public static void preOrderTraversal(Node<String> node) {
if (node == null) return;
System.out.print(node.getData() + " ");
for (Node<String> child : node.getChildren()) {
preOrderTraversal(child);
}
}
public static void postOrderTraversal(Node<String> node) {
if (node == null) return;
for (Node<String> child : node.getChildren()) {
postOrderTraversal(child);
}
System.out.print(node.getData() + " ");
}
public static void levelOrderTraversal(Node<String> root) {
if (root == null) return;
Queue<Node<String>> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
Node<String> node = queue.poll();
System.out.print(node.getData() + " ");
for (Node<String> child : node.getChildren()) {
queue.offer(child);
}
}
}
public static void main(String[] args) {
// ... (tree creation code remains the same)
System.out.println("Pre-order traversal:");
preOrderTraversal(root);
System.out.println("\n\nPost-order traversal:");
postOrderTraversal(root);
System.out.println("\n\nLevel-order traversal:");
levelOrderTraversal(root);
}
}
Let’s break down these traversal methods:
- Pre-order traversal: In this method, we visit the current node first, then recursively traverse its children from left to right. This is useful when you want to create a copy of the tree or generate a prefix expression from an expression tree.
- Post-order traversal: Here, we recursively traverse the children from left to right, and then visit the current node. This is helpful in scenarios like deleting a tree or evaluating a postfix expression.
- Level-order traversal: Also known as breadth-first traversal, this method visits nodes level by level, from left to right. We use a queue to keep track of nodes at each level. This is particularly useful for tasks like finding the minimum depth of a tree or printing the tree level by level.
When you run this code, you’ll see the following output:
Pre-order traversal:
Root Child 1 Grandchild 1 Grandchild 2 Child 2 Child 3
Post-order traversal:
Grandchild 1 Grandchild 2 Child 1 Child 2 Child 3 Root
Level-order traversal:
Root Child 1 Child 2 Child 3 Grandchild 1 Grandchild 2
These traversal methods demonstrate different ways to explore and process the nodes in a tree structure. Each method has its own use cases, and understanding them will help you choose the right approach for your specific problem.
Binary Trees: The Power of Two
Introducing binary trees
Now that we’ve explored general tree structures, let’s narrow our focus to a specific and widely used type of tree: the binary tree. A binary tree is a tree data structure in which each node has at most two children, referred to as the left child and the right child. This simple constraint leads to a powerful and efficient structure that forms the basis for many advanced data structures and algorithms.
Let’s create a BinaryTreeNode class to represent nodes in a binary tree:
public class BinaryTreeNode<T> {
private T data;
private BinaryTreeNode<T> left;
private BinaryTreeNode<T> right;
public BinaryTreeNode(T data) {
this.data = data;
this.left = null;
this.right = null;
}
// Getters and setters
public T getData() { return data; }
public void setData(T data) { this.data = data; }
public BinaryTreeNode<T> getLeft() { return left; }
public void setLeft(BinaryTreeNode<T> left) { this.left = left; }
public BinaryTreeNode<T> getRight() { return right; }
public void setRight(BinaryTreeNode<T> right) { this.right = right; }
}
Now, let’s create a BinaryTree class that uses this node structure:
public class BinaryTree<T> {
private BinaryTreeNode<T> root;
public BinaryTree() {
this.root = null;
}
public void setRoot(BinaryTreeNode<T> root) {
this.root = root;
}
public BinaryTreeNode<T> getRoot() {
return root;
}
// Traversal methods
public void inOrderTraversal(BinaryTreeNode<T> node) {
if (node == null) return;
inOrderTraversal(node.getLeft());
System.out.print(node.getData() + " ");
inOrderTraversal(node.getRight());
}
public void preOrderTraversal(BinaryTreeNode<T> node) {
if (node == null) return;
System.out.print(node.getData() + " ");
preOrderTraversal(node.getLeft());
preOrderTraversal(node.getRight());
}
public void postOrderTraversal(BinaryTreeNode<T> node) {
if (node == null) return;
postOrderTraversal(node.getLeft());
postOrderTraversal(node.getRight());
System.out.print(node.getData() + " ");
}
// Main method for testing
public static void main(String[] args) {
BinaryTree<Integer> tree = new BinaryTree<>();
BinaryTreeNode<Integer> root = new BinaryTreeNode<>(1);
root.setLeft(new BinaryTreeNode<>(2));
root.setRight(new BinaryTreeNode<>(3));
root.getLeft().setLeft(new BinaryTreeNode<>(4));
root.getLeft().setRight(new BinaryTreeNode<>(5));
tree.setRoot(root);
System.out.println("In-order traversal:");
tree.inOrderTraversal(tree.getRoot());
System.out.println("\nPre-order traversal:");
tree.preOrderTraversal(tree.getRoot());
System.out.println("\nPost-order traversal:");
tree.postOrderTraversal(tree.getRoot());
}
}
This BinaryTree class implements the three main traversal methods for binary trees:
- In-order traversal: Visit the left subtree, then the current node, then the right subtree. This is particularly useful for binary search trees, as it visits the nodes in ascending order.
- Pre-order traversal: Visit the current node, then the left subtree, then the right subtree. This is useful for creating a copy of the tree or generating a prefix expression.
- Post-order traversal: Visit the left subtree, then the right subtree, then the current node. This is helpful for deleting a tree or evaluating a postfix expression.
When you run this code, you’ll see the following output:
In-order traversal:
4 2 5 1 3
Pre-order traversal:
1 2 4 5 3
Post-order traversal:
4 5 2 3 1
Binary trees are the foundation for more complex tree structures like binary search trees, AVL trees, and Red-Black trees. Understanding binary trees and their traversal methods is crucial for mastering these advanced data structures.
Binary Search Trees: Efficient Searching and Sorting
Organizing data for quick retrieval
Now that we’ve explored binary trees, let’s take a step further and dive into Binary Search Trees (BST). A Binary Search Tree is a special type of binary tree that maintains a specific ordering of nodes, making it highly efficient for searching, inserting, and deleting elements.
In a BST, for any given node:
- All nodes in the left subtree have values less than the node’s value.
- All nodes in the right subtree have values greater than the node’s value.
- Both the left and right subtrees are also binary search trees.
This ordering property allows for efficient searching, as we can eliminate half of the remaining tree at each step of the search process.
Let’s implement a basic Binary Search Tree:
“`java
public class BinarySearchTree> {
private BinaryTreeNode root;
public BinarySearchTree() {
this.root = null;
}
public void insert(T data) {
root = insertRec(root, data);
}
private BinaryTreeNode<T> insertRec(BinaryTreeNode<T> root, T data) {
if (root == null) {
return new BinaryTreeNode<>(data);
}
if (data.compareTo(root.getData()) < 0) {
root.setLeft(insertRec(root.getLeft(), data));
} else if (data.compareTo(root.getData()) > 0) {
root.setRight(insertRec(root.getRight(), data));
}
return root;
}
public boolean search(T data) {
return searchRec(root, data);
}
private boolean searchRec(BinaryTreeNode<T> root, T data) {
if (root == null) {
return false;
}
if (data.equals(root.getData())) {
return true;
}
if (data.compareTo(root.getData()) < 0) {
return searchRec(root.getLeft(), data);
}
return searchRec(root.getRight(), data);
}
public void inOrderTraversal() {
inOrderTraversalRec(root);
System.out.println();
}
private void inOrderTraversalRec(BinaryTreeNode<T> root) {
if (root != null) {
inOrderTraversalRec(root.getLeft());
System.out.print(root.getData() + " ");
inOrderTraversalRec(root.getRight());
}
}
public static void main(String[] args) {
BinarySearchTree<Integer> bst = new BinarySearchTree<>();
bst.insert(5);
bst.insert(3);
bst.insert(7);
bst.insert(1);
bst.insert(9);
System.out.println("In-order traversal of the BST:");
bst.inOrderTraversal();
System.out.println("Searching for 7: " + bst.search(7));
System.out.println("Searching for 4: " + bst.search(4));
}
}
This implementation of a Binary Search Tree includes methods for inserting elements, searching for a specific value, and performing an in-order traversal. The `insert` method maintains the BST property by recursively finding the correct position for the new node. The `search` method efficiently locates elements by traversing the tree based on the BST property. When you run this code, you’ll see the following output:
In-order traversal of the BST:
1 3 5 7 9
Searching for 7: true
Searching for 4: false
Notice how the in-order traversal of a BST produces a sorted list of elements. This is one of the key benefits of using a BST – it maintains its elements in a sorted order, allowing for efficient searching and retrieval.
Balancing Act: AVL Trees
Keeping trees in shape
While Binary Search Trees are efficient for many operations, their performance can degrade if the tree becomes unbalanced. For example, if you insert elements in sorted order, the BST will degenerate into a linked list, with search operations taking O(n) time instead of the expected O(log n).
This is where self-balancing trees come into play. One popular type of self-balancing binary search tree is the AVL tree, named after its inventors Adelson-Velsky and Landis. AVL trees maintain balance by ensuring that the heights of the left and right subtrees of every node differ by at most one.
Let’s implement a basic AVL tree:
public class AVLTree> {
private class AVLNode {
T data;
AVLNode left, right;
int height;
AVLNode(T data) {
this.data = data;
this.height = 1;
}
}
private AVLNode root;
private int height(AVLNode node) {
return (node == null) ? 0 : node.height;
}
private int balanceFactor(AVLNode node) {
return (node == null) ? 0 : height(node.left) - height(node.right);
}
private AVLNode rightRotate(AVLNode y) {
AVLNode x = y.left;
AVLNode T2 = x.right;
x.right = y;
y.left = T2;
y.height = Math.max(height(y.left), height(y.right)) + 1;
x.height = Math.max(height(x.left), height(x.right)) + 1;
return x;
}
private AVLNode leftRotate(AVLNode x) {
AVLNode y = x.right;
AVLNode T2 = y.left;
y.left = x;
x.right = T2;
x.height = Math.max(height(x.left), height(x.right)) + 1;
y.height = Math.max(height(y.left), height(y.right)) + 1;
return y;
}
public void insert(T data) {
root = insertRec(root, data);
}
private AVLNode insertRec(AVLNode node, T data) {
if (node == null) {
return new AVLNode(data);
}
if (data.compareTo(node.data) < 0) {
node.left = insertRec(node.left, data);
} else if (data.compareTo(node.data) > 0) {
node.right = insertRec(node.right, data);
} else {
return node; // Duplicate keys are not allowed
}
node.height = 1 + Math.max(height(node.left), height(node.right));
int balance = balanceFactor(node);
// Left Left Case
if (balance > 1 && data.compareTo(node.left.data) < 0) {
return rightRotate(node);
}
// Right Right Case
if (balance < -1 && data.compareTo(node.right.data) > 0) {
return leftRotate(node);
}
// Left Right Case
if (balance > 1 && data.compareTo(node.left.data) > 0) {
node.left = leftRotate(node.left);
return rightRotate(node);
}
// Right Left Case
if (balance < -1 && data.compareTo(node.right.data) < 0) {
node.right = rightRotate(node.right);
return leftRotate(node);
}
return node;
}
public void inOrderTraversal() {
inOrderTraversalRec(root);
System.out.println();
}
private void inOrderTraversalRec(AVLNode root) {
if (root != null) {
inOrderTraversalRec(root.left);
System.out.print(root.data + " ");
inOrderTraversalRec(root.right);
}
}
public static void main(String[] args) {
AVLTree<Integer> avl = new AVLTree<>();
avl.insert(10);
avl.insert(20);
avl.insert(30);
avl.insert(40);
avl.insert(50);
avl.insert(25);
System.out.println("In-order traversal of the AVL tree:");
avl.inOrderTraversal();
}
}
This AVL tree implementation includes methods for inserting elements while maintaining balance through rotations. The `balanceFactor` method calculates the difference in height between the left and right subtrees, which is used to determine when and how to rebalance the tree. When you run this code, you’ll see the following output:
In-order traversal of the AVL tree:
10 20 25 30 40 50
Notice that even though we inserted elements in ascending order, the AVL tree maintains balance, preventing the degeneration into a linked list that would occur with a simple BST.
Practical Applications: Real-world Uses of Tree Structures
Putting trees to work
Now that we’ve explored various tree structures, let’s discuss some practical applications where these data structures shine. Understanding these use cases will help you recognize when to reach for a tree structure in your own projects.
1. File Systems
One of the most common real-world examples of a tree structure is your computer’s file system. Directories (folders) can contain files and other directories, creating a hierarchical structure that’s perfectly represented by a tree. Here’s a simple example of how you might model a file system using a tree structure in Java:
class FileSystemNode {
private String name;
private boolean isDirectory;
private List children;
public FileSystemNode(String name, boolean isDirectory) {
this.name = name;
this.isDirectory = isDirectory;
this.children = new ArrayList<>();
}
public void addChild(FileSystemNode child) {
if (isDirectory) {
children.add(child);
} else {
throw new UnsupportedOperationException("Cannot add child to a file");
}
}
public void print(String indent) {
System.out.println(indent + (isDirectory ? "[D] " : "[F] ") + name);
for (FileSystemNode child : children) {
child.print(indent + " ");
}
}
}
public class FileSystem {
public static void main(String[] args) {
FileSystemNode root = new FileSystemNode("root", true);
FileSystemNode documents = new FileSystemNode("Documents", true);
FileSystemNode pictures = new FileSystemNode("Pictures", true);
root.addChild(documents);
root.addChild(pictures);
documents.addChild(new FileSystemNode("resume.docx", false));
documents.addChild(new FileSystemNode("budget.xlsx", false));
pictures.addChild(new FileSystemNode("vacation", true));
pictures.getChildren().get(0).addChild(new FileSystemNode("beach.jpg", false));
root.print("");
}
}
This example demonstrates how a tree structure can naturally represent the hierarchical nature of a file system.
2. HTML DOM (Document Object Model)
Web browsers use a tree structure called the DOM to represent the structure of HTML documents. Each HTML element is a node in the tree, with parent-child relationships mirroring the nesting of elements. This tree structure allows for efficient traversal and manipulation of web page elements.
3. Database Indexing
Many databases use tree structures, particularly B-trees and their variants, for indexing. These structures allow for efficient searching, insertion, and deletion of records, enabling databases to handle large amounts of data while maintaining good performance.
4. Decision Trees in Machine Learning
In the field of machine learning, decision trees are used for both classification and regression tasks. These tree structures represent a series of decisions based on feature values, leading to a final prediction or classification.
5. Syntax Trees in Compilers
Compilers and interpreters often use abstract syntax trees (ASTs) to represent the structure of program code. These trees capture the hierarchical nature of programming language syntax and are crucial for tasks like code analysis, optimization, and translation.
6. Game AI and Pathfinding
Tree structures, particularly game trees, are extensively used in artificial intelligence for games. Algorithms like Minimax use these trees to evaluate possible future game states and make optimal decisions. Similarly, pathfinding algorithms often use tree-like structures to represent possible paths and find the optimal route.
Conclusion
As we’ve seen throughout this journey, tree structures are a fundamental concept in computer science and software development. From simple binary trees to self-balancing AVL trees, these data structures offer powerful tools for organizing and manipulating hierarchical data.
By mastering tree structures, you’ll be better equipped to tackle a wide range of programming challenges. Whether you’re building a file system, optimizing database queries, or developing game AI, understanding trees will give you the ability to create more efficient and elegant solutions.
Remember, the key to becoming proficient with tree structures is practice. Don’t be afraid to implement these structures from scratch, experiment with different traversal methods, and explore how they can be applied to real-world problems. As you continue to grow your skills, you’ll find that trees are an invaluable addition to your programming toolkit.
So go forth, brave coder, and may your trees always be balanced, your traversals efficient, and your algorithms optimal!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information and code examples provided in this blog post, they are intended for educational purposes only. Always thoroughly test and validate any code before using it in production environments. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.