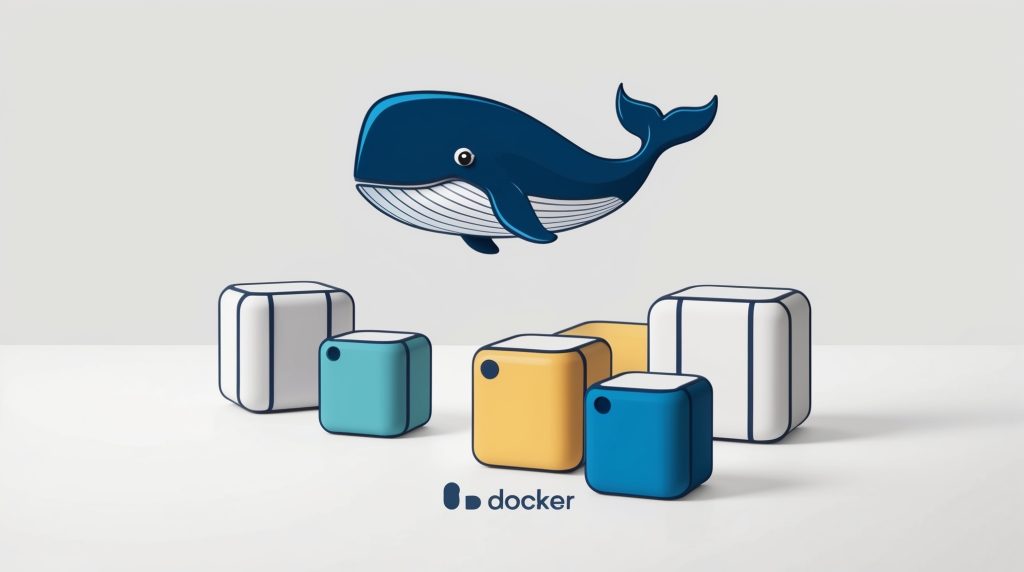
The Basics of Docker
In today’s rapidly evolving technological landscape, containerization has become an essential tool for developers and system administrators alike. At the forefront of this revolution stands Docker, a powerful platform that simplifies the process of creating, deploying, and running applications in isolated environments. This comprehensive guide aims to demystify Docker for beginners, providing a step-by-step approach to understanding its fundamental concepts and practical applications.
Introduction to Docker
Docker has revolutionized the way we develop, ship, and run applications. It provides a standardized unit of software that packages up code and all its dependencies, ensuring that the application runs quickly and reliably from one computing environment to another. This consistency across different environments is one of the primary reasons for Docker’s widespread adoption in the software development industry.
What is Docker?
Docker is an open-source platform that automates the deployment, scaling, and management of applications. It does this by containerizing applications – wrapping up an application with all of its required dependencies into a standardized unit for software development. This container can then be run on any system that supports Docker, regardless of the underlying hardware or operating system.
Why Use Docker?
The benefits of using Docker are numerous and significant:
- Consistency: Docker ensures that your application runs the same way in any environment, from development to production.
- Isolation: Containers are isolated from one another and the host system, providing an additional layer of security.
- Efficiency: Docker containers are lightweight and use resources more efficiently compared to traditional virtual machines.
- Scalability: Docker makes it easy to scale applications up or down quickly.
- Version Control: Docker allows you to track versions of your container images and their dependencies.
Docker vs. Virtual Machines
To better understand Docker, it’s helpful to compare it with virtual machines (VMs). While both technologies provide isolation and virtualization, they do so in fundamentally different ways:
Feature | Docker Containers | Virtual Machines |
---|---|---|
Operating System | Shares the host OS kernel | Requires a full OS |
Performance | Lightweight and fast | More resource-intensive |
Boot Time | Seconds | Minutes |
Storage | Typically megabytes | Often gigabytes |
Portability | Highly portable | Less portable |
Docker containers are more lightweight because they share the host system’s kernel and do not require a full operating system for each application. This results in faster start times, reduced disk space usage, and improved performance compared to traditional VMs.
Docker Architecture
Understanding Docker’s architecture is crucial for grasping how it works and why it’s so effective. Docker uses a client-server architecture, comprising several key components that work together to create and manage containers.
Docker Daemon
The Docker daemon (dockerd) is the persistent process that manages Docker containers on a system. It listens for Docker API requests and manages Docker objects such as images, containers, networks, and volumes. The daemon can also communicate with other daemons to manage Docker services.
Docker Client
The Docker client (docker) is the primary way users interact with Docker. When you use commands such as docker run
, the client sends these commands to dockerd, which carries them out. The Docker client can communicate with more than one daemon.
Docker Registry
A Docker registry stores Docker images. Docker Hub is a public registry that anyone can use, and Docker is configured to look for images on Docker Hub by default. You can also run your own private registry. When you use commands like docker pull
or docker run
, the required images are pulled from your configured registry.
Docker Objects
Docker objects are the various entities used to assemble and run your applications in Docker. The main Docker objects include:
- Images: Read-only templates used to create containers.
- Containers: Runnable instances of images.
- Networks: Provide isolated networking environments for containers.
- Volumes: Persistent data storage for containers.
Understanding these components and how they interact is essential for effectively using Docker in your development and deployment workflows.
Installing Docker
Before diving into Docker’s functionality, you need to install it on your system. The installation process varies depending on your operating system.
For Windows and Mac
- Visit the official Docker website (https://www.docker.com/products/docker-desktop).
- Download Docker Desktop for your operating system.
- Run the installer and follow the prompts.
- Once installed, start Docker Desktop.
For Linux
The installation process for Linux varies depending on the distribution. Here’s a general outline for Ubuntu:
# Update existing packages
sudo apt-get update
# Install prerequisites
sudo apt-get install apt-transport-https ca-certificates curl software-properties-common
# Add Docker's official GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
# Add Docker repository
sudo add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable"
# Update package database
sudo apt-get update
# Install Docker
sudo apt-get install docker-ce
# Start and enable Docker service
sudo systemctl start docker
sudo systemctl enable docker
After installation, verify that Docker is correctly installed by running:
docker --version
This command should display the installed Docker version, confirming a successful installation.
Docker Basic Commands
Now that Docker is installed, let’s explore some fundamental commands that will help you start working with containers.
1. docker run
The docker run
command is used to create and start a new container from an image.
docker run hello-world
This command pulls the ‘hello-world’ image from Docker Hub (if not already present locally) and runs it in a new container.
2. docker ps
Use docker ps
to list running containers:
docker ps
To see all containers (including stopped ones), use:
docker ps -a
3. docker images
To list the Docker images on your system:
docker images
4. docker pull
To download an image from a registry (like Docker Hub) without running it:
docker pull ubuntu
5. docker stop and docker start
To stop a running container:
docker stop <container_id>
To start a stopped container:
docker start <container_id>
6. docker rm and docker rmi
To remove a container:
docker rm <container_id>
To remove an image:
docker rmi <image_id>
These basic commands form the foundation of working with Docker. As you become more comfortable with these, you can explore more advanced Docker functionalities.
Understanding Docker Images
Docker images are the building blocks of containers. An image is a read-only template that contains a set of instructions for creating a Docker container. It’s essentially a snapshot of a container, providing all the necessary information to run it.
Anatomy of a Docker Image
A Docker image consists of multiple layers, each representing an instruction in the image’s Dockerfile. When you change the Dockerfile and rebuild the image, only the layers that have changed are rebuilt. This is part of what makes Docker images so lightweight and fast.
Finding and Using Docker Images
Docker Hub (https://hub.docker.com/) is the default public registry for Docker images. You can search for images directly on Docker Hub or use the Docker command line:
docker search ubuntu
To use an image from Docker Hub, simply reference it in your docker run
command:
docker run ubuntu
This command pulls the latest Ubuntu image (if not already present locally) and runs it in a new container.
Creating Your Own Docker Images
While using pre-built images is convenient, you’ll often need to create custom images for your applications. This is done using a Dockerfile.
A Dockerfile is a text document that contains all the commands a user could call on the command line to assemble an image. Here’s a simple example:
# Use an official Python runtime as a parent image
FROM python:3.7-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
To build an image from this Dockerfile:
docker build -t my-python-app .
This command builds a new image named ‘my-python-app’ based on the instructions in the Dockerfile in the current directory.
Working with Docker Containers
Docker containers are the runnable instances of Docker images. While images are read-only, containers add a writable layer on top of the image, allowing you to modify the running instance.
Creating and Running Containers
To create and start a container from an image:
docker run -d -p 80:80 --name my-container nginx
This command does the following:
-d
: Runs the container in detached mode (in the background)-p 80:80
: Maps port 80 of the host to port 80 in the container--name my-container
: Assigns the name “my-container” to the containernginx
: Specifies the image to use
Interacting with Containers
To execute a command in a running container:
docker exec -it my-container /bin/bash
This opens an interactive bash shell in the container.
Container Lifecycle
Containers have a lifecycle that includes several states:
- Created: The container has been created but not started
- Running: The container is running with all its processes active
- Paused: The container has been paused
- Stopped: The container has been stopped
- Deleted: The container has been deleted
You can manage these states with various Docker commands:
docker pause <container_id>
: Pause a running containerdocker unpause <container_id>
: Unpause a paused containerdocker stop <container_id>
: Stop a running containerdocker start <container_id>
: Start a stopped containerdocker restart <container_id>
: Restart a container
Data Persistence
By default, any data created inside a container is only available within that container and for the duration of its lifecycle. To persist data beyond the life of a container, Docker provides volumes and bind mounts.
Volumes are the preferred mechanism for persisting data generated by and used by Docker containers. To create and use a volume:
# Create a volume
docker volume create my-vol
# Run a container with a volume mounted
docker run -d --name devtest -v my-vol:/app nginx:latest
This creates a volume named ‘my-vol’ and mounts it to the /app
directory in the container.
Docker Networking
Docker networking enables containers to communicate with each other and with the outside world. Docker provides several network drivers to accommodate various use cases.
Default Networks
Docker includes three default networks:
- Bridge: The default network driver. Containers on the same bridge network can communicate.
- Host: Removes network isolation between the container and the Docker host.
- None: Disables all networking for the container.
To list available networks:
docker network ls
Creating Custom Networks
You can create your own networks for more complex scenarios:
docker network create my-network
To run a container on this network:
docker run -d --network my-network --name my-container nginx
Network Troubleshooting
Docker provides several commands to help troubleshoot networking issues:
docker network inspect <network_name>
: Provides detailed information about a networkdocker port <container_name>
: Shows port mappings for a containerdocker network connect <network_name> <container_name>
: Connects a running container to a network
Understanding Docker networking is crucial for building complex, multi-container applications and ensuring they can communicate effectively.
Docker Compose
As your applications grow more complex, you’ll often need to run multiple containers that work together. Docker Compose is a tool for defining and running multi-container Docker applications.
What is Docker Compose?
Docker Compose allows you to define your application’s services, networks, and volumes in a single YAML file, then spin up your entire application with a single command.
Docker Compose File
Here’s an example of a simple docker-compose.yml
file:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
redis:
image: "redis:alpine"
This file defines two services: a web service built from the Dockerfile in the current directory, and a Redis service using the official Redis image.
Using Docker Compose
To start your application:
docker-compose up
To stop it:
docker-compose down
Docker Compose is particularly useful for development environments, automated testing, and single-host deployments.
Best Practices for Using Docker
As you become more proficient with Docker, it’s important to follow best practices to ensure your Docker usage is efficient, secure, and maintainable.
1. Use Official Images
Whenever possible, use official images from Docker Hub as your base images. These are maintained by Docker and the software vendors, ensuring they are up-to-date and secure.
2. Minimize the Number of Layers
Each instruction in a Dockerfile creates a new layer. Try to combine commands (using &&
) to reduce the number of layers and keep your images smaller.
3. Use .dockerignore
Create a .dockerignore
file to exclude files and directories that aren’t necessary for building your image. This can significantly reduce build time and image size.
4. Don’t Run Containers as Root
For security reasons, it’s best to run containers as a non-root user. You can do this by adding a user in your Dockerfile:
RUN adduser --disabled-password --gecos '' myuser
USER myuser
5. Use Multi-Stage Builds
Multi-stage builds allow you to use multiple FROM statements in your Dockerfile. This is useful for creating smaller production images:
# Build stage
FROM golang:1.16 AS build
WORKDIR /app
COPY . .
RUN go build -o main .
# Production stage
FROM alpine:3.14
COPY --from=build /app/main /usr/local/bin/
CMD ["main"]
6. Keep Images Small
Use minimal base images like Alpine when possible. Remove unnecessary files and avoid installing unnecessary packages.
7. Tag Your Images
Always use meaningful tags for your images. The latest
tag can be convenient but can also lead to unexpected behavior if not managed carefully.
8. Use Environment Variables
Use environment variables for configuration that might change between deployments:
ENV APP_HOME /myapp
WORKDIR $APP_HOME
9. Monitor Your Containers
Use Docker’s built-in commands like docker stats
and docker events
, or third-party tools, to monitor your containers’ resource usage and health.
Conclusion
Docker has revolutionized the way we develop, ship, and run applications. Its ability to provide consistent environments across different stages of development and production has made it an indispensable tool in modern software development.
This guide has covered the basics of Docker, from installation to best practices. We’ve explored Docker’s architecture, learned how to work with images and containers, delved into networking and data persistence, and introduced Docker Compose for managing multi-container applications.
As you continue your Docker journey, remember that the ecosystem is vast and constantly evolving. Stay curious, keep experimenting, and don’t hesitate to dive deeper into areas that interest you or are relevant to your projects.
Docker’s official documentation (https://docs.docker.com/) is an excellent resource for more advanced topics and up-to-date information. Additionally, the Docker community is vibrant and helpful, with numerous forums, blogs, and social media channels where you can ask questions and share your experiences.
By mastering Docker, you’re not just learning a tool; you’re embracing a philosophy of software development that prioritizes consistency, efficiency, and scalability. As containerization continues to grow in importance, the skills you develop with Docker will be invaluable in your career as a developer or system administrator.
Remember, the key to mastering Docker, like any technology, is practice. Start small, build your understanding, and gradually tackle more complex scenarios. Before long, you’ll find Docker an indispensable part of your development toolkit.
Disclaimer: This guide is intended for educational purposes and provides a general overview of Docker. While we strive for accuracy, Docker and its ecosystem are constantly evolving. Always refer to the official Docker Documentation for most upto date information.