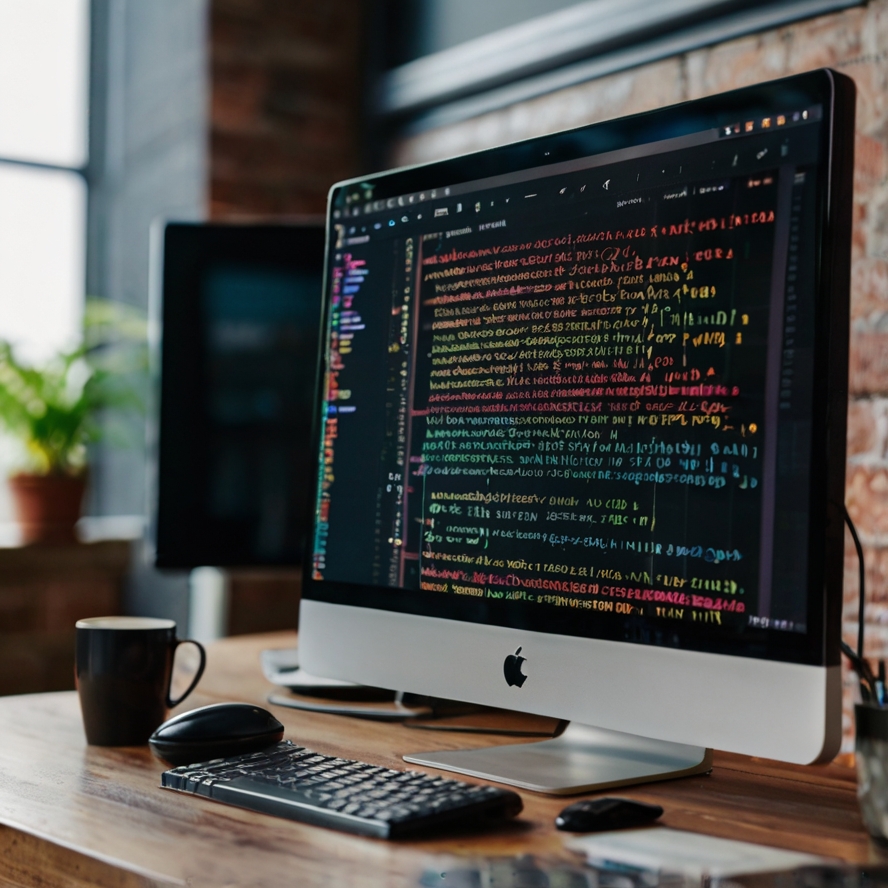
The Benefits of Learning JavaScript
JavaScript is one of the most popular and versatile programming languages in the world. Whether you’re a beginner in coding or an experienced developer looking to expand your skill set, learning JavaScript can open up a myriad of opportunities. In this blog, we’ll explore the numerous benefits of learning JavaScript, from enhancing your career prospects to enabling you to build dynamic websites and applications. Let’s dive into why JavaScript should be your next language to master.
JavaScript is Ubiquitous
JavaScript is everywhere. It is the backbone of web development and powers almost every interactive feature on the internet. From simple form validations to complex single-page applications, JavaScript is used extensively to create dynamic and engaging user experiences.
Example Code: Adding interactivity to a button
<!DOCTYPE html>
<html>
<head>
<title>Button Click Example</title>
</head>
<body>
<button id="myButton">Click Me!</button>
<script>
document.getElementById('myButton').addEventListener('click', function() {
alert('Button was clicked!');
});
</script>
</body>
</html>
In this example, JavaScript is used to add an event listener to a button. When the button is clicked, an alert box pops up, demonstrating a basic interactive feature made possible by JavaScript.
High Demand for JavaScript Developers
One of the key benefits of learning JavaScript is the high demand for developers proficient in this language. Companies across various industries seek JavaScript developers to build and maintain their web applications. This high demand translates into numerous job opportunities and competitive salaries for those skilled in JavaScript.
Job Listings Mentioning JavaScript
- Front-End Developer at TechCorp
- Full Stack Developer at Innovate Inc.
- JavaScript Engineer at Web Solutions
The consistent demand for JavaScript skills makes it a valuable language to learn for anyone looking to enter the tech industry or advance their career.
Easy to Learn and Start with
JavaScript is known for its relatively easy learning curve compared to other programming languages. Its syntax is straightforward and similar to English, making it accessible to beginners. Additionally, there are numerous resources available, including tutorials, documentation, and community support, to help you learn JavaScript effectively.
Example Code: Basic JavaScript Syntax
// This is a comment
let message = "Hello, World!"; // Declare a variable
console.log(message); // Output the message to the console
In the above snippet, you can see how simple it is to declare a variable and output a message using JavaScript. The language’s simplicity encourages beginners to start coding quickly.
Versatile and Powerful
JavaScript is not limited to front-end development. With the advent of Node.js, JavaScript can now be used for back-end development as well. This versatility allows developers to use the same language for both client-side and server-side scripting, streamlining the development process and reducing the need to learn multiple languages.
Example Code: Node.js Server
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
Here, JavaScript is used to create a simple HTTP server using Node.js. This demonstrates how JavaScript extends beyond the browser to server-side development, showcasing its versatility.
Extensive Libraries and Frameworks
JavaScript boasts a rich ecosystem of libraries and frameworks that simplify development and enhance productivity. Libraries like jQuery make DOM manipulation easier, while frameworks like React, Angular, and Vue.js enable the development of complex, scalable web applications.
Example Code: React Component
import React from 'react';
class HelloWorld extends React.Component {
render() {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
}
}
export default HelloWorld;
This React component demonstrates how JavaScript can be used to build reusable UI components, making development more efficient and maintainable.
Community and Support
JavaScript has a massive and active community. This means that there are countless forums, discussion groups, and online resources available to help you when you encounter problems or have questions. Websites like Stack Overflow and GitHub are treasure troves of information where you can find solutions and collaborate with other developers.
Popular JavaScript Communities
- Stack Overflow
- GitHub
- Reddit (r/javascript)
- MDN Web Docs
The strong community support ensures that you are never alone in your learning journey and can always find help when needed.
Continuous Evolution and Improvement
JavaScript is continuously evolving. The language is regularly updated with new features and improvements, ensuring that it remains relevant and powerful. This constant evolution keeps the language exciting and ensures that it can meet the demands of modern web development.
Example Code: ES6 Features
// Arrow functions
const add = (a, b) => a + b;
console.log(add(2, 3)); // Output: 5
// Template literals
let name = "JavaScript";
console.log(`Hello, ${name}!`); // Output: Hello, JavaScript!
The introduction of ES6 (ECMAScript 2015) brought several new features to JavaScript, such as arrow functions and template literals, making the language more concise and expressive.
Great Career Advancement Opportunities
Learning JavaScript can significantly enhance your career advancement opportunities. With the knowledge of JavaScript, you can pursue various roles such as front-end developer, back-end developer, full-stack developer, or even specialize in frameworks like React or Angular. This flexibility allows you to tailor your career path according to your interests and market demand.
Career Paths for JavaScript Developers
- Front-End Developer
- Back-End Developer
- Full-Stack Developer
- Mobile App Developer
- Game Developer
The diverse career paths available to JavaScript developers make it a worthwhile investment in your professional growth.
Building Interactive Websites
JavaScript is essential for creating interactive websites. It enables you to add dynamic content, control multimedia, animate images, and much more. This interactivity is crucial for providing users with a seamless and engaging experience.
Example Code: Image Slider
<!DOCTYPE html>
<html>
<head>
<title>Image Slider</title>
<style>
.slider {
width: 300px;
height: 200px;
overflow: hidden;
}
.slides {
display: flex;
transition: transform 0.5s ease-in-out;
}
.slide {
min-width: 300px;
height: 200px;
}
</style>
</head>
<body>
<div class="slider">
<div class="slides" id="slides">
<img src="image1.jpg" class="slide" />
<img src="image2.jpg" class="slide" />
<img src="image3.jpg" class="slide" />
</div>
</div>
<script>
let index = 0;
const slides = document.getElementById('slides');
const totalSlides = document.querySelectorAll('.slide').length;
setInterval(() => {
index = (index + 1) % totalSlides;
slides.style.transform = `translateX(${-index * 300}px)`;
}, 2000);
</script>
</body>
</html>
This simple image slider uses JavaScript to create an interactive element on a webpage, enhancing the user experience.
Support for Modern Web APIs
JavaScript supports modern web APIs that allow you to build advanced features and functionalities. APIs like the Fetch API for making network requests, the Geolocation API for location-based services, and the Web Storage API for client-side data storage enable developers to create powerful web applications.
Example Code: Fetch API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
In this example, the Fetch API is used to make an HTTP request to an API endpoint, showcasing how JavaScript can be used to interact with web services and retrieve data.
Learning JavaScript is a smart move for anyone interested in web development or looking to enhance their technical skills. Its ubiquity, versatility, and high demand make it a valuable asset in today’s job market. Whether you’re building interactive websites, developing server-side applications, or leveraging modern web APIs, JavaScript equips you with the tools to create dynamic and engaging user experiences. With its supportive community and continuous evolution, JavaScript ensures that you’ll always be at the forefront of web development technology. So, take the plunge and start learning JavaScript today – your future self will thank you!