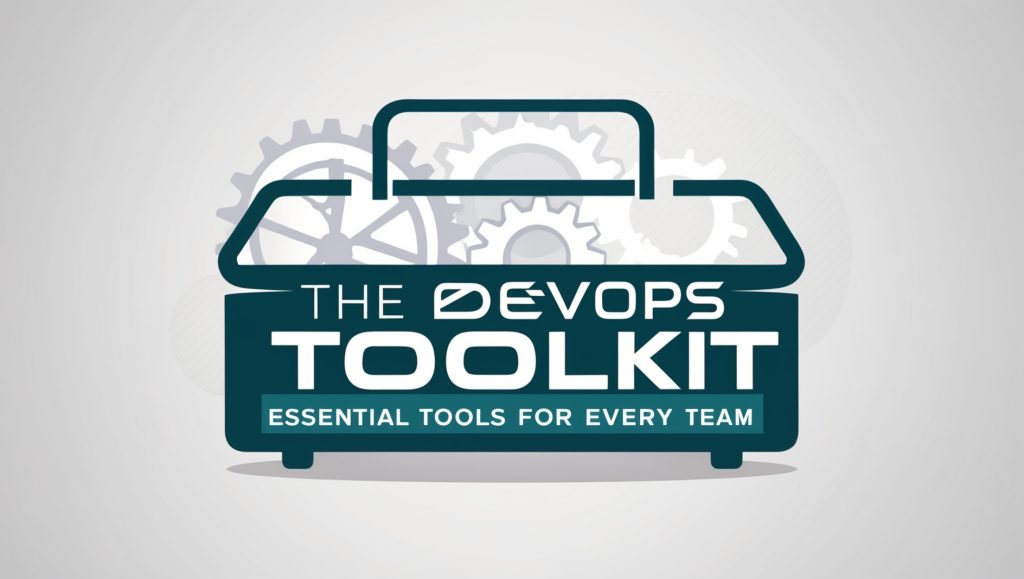
The DevOps Toolkit: Essential Tools for Every Team
In today’s fast-paced tech world, DevOps has become more than just a buzzword – it’s a necessity. Whether you’re a startup or an enterprise, having the right DevOps toolkit can make or break your team’s efficiency and productivity. But with so many tools out there, how do you know which ones are truly essential? Don’t worry, we’ve got you covered! In this comprehensive guide, we’ll explore the must-have tools that every DevOps team should have in their arsenal. From version control to monitoring, we’ll dive deep into each category and help you build a toolkit that’ll supercharge your development and operations processes. So, grab a cup of coffee, and let’s embark on this DevOps journey together!
Version Control: The Foundation of Collaboration
Let’s kick things off with the cornerstone of any DevOps toolkit: version control. It’s like the air we breathe in the development world – you can’t live without it. Version control systems (VCS) allow teams to track changes, collaborate seamlessly, and maintain a history of their codebase. While there are several options out there, Git has emerged as the undisputed champion in this arena.
Why Git?
Git’s distributed nature, powerful branching and merging capabilities, and widespread adoption make it the go-to choice for most teams. It’s not just about storing code; it’s about fostering collaboration and maintaining a clear history of your project’s evolution. With Git, you can easily experiment with new features, roll back changes if things go south, and work on multiple aspects of your project simultaneously.
Git in Action
Let’s look at some basic Git commands that every developer should know:
# Initialize a new Git repository
git init
# Add files to staging
git add .
# Commit changes
git commit -m "Add new feature: user authentication"
# Create and switch to a new branch
git checkout -b feature/payment-gateway
# Merge changes from another branch
git merge develop
# Push changes to remote repository
git push origin main
These commands are just the tip of the iceberg. As you delve deeper into Git, you’ll discover more advanced features like rebasing, cherry-picking, and hooks that can further streamline your workflow. Remember, mastering Git is an ongoing process, but even knowing the basics can significantly boost your team’s productivity.
Continuous Integration/Continuous Deployment (CI/CD): Automating Success
Now that we’ve got our code under control, let’s talk about getting it from your developer’s laptop to production seamlessly. This is where CI/CD pipelines come into play. These automated processes are the bread and butter of DevOps, ensuring that code changes are automatically built, tested, and deployed.
Popular CI/CD Tools
While there are numerous CI/CD tools available, some of the most popular ones include:
- Jenkins
- GitLab CI/CD
- CircleCI
- Travis CI
- GitHub Actions
Each of these tools has its strengths, but let’s focus on GitHub Actions as an example, given its tight integration with GitHub repositories.
GitHub Actions: CI/CD Made Simple
GitHub Actions allows you to automate your software development workflows right in your GitHub repository. It’s incredibly flexible and can handle everything from simple tasks to complex CI/CD pipelines. Here’s a basic example of a GitHub Actions workflow that builds and tests a Node.js application:
name: Node.js CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [14.x, 16.x, 18.x]
steps:
- uses: actions/checkout@v3
- name: Use Node.js ${{ matrix.node-version }}
uses: actions/setup-node@v3
with:
node-version: ${{ matrix.node-version }}
- run: npm ci
- run: npm run build --if-present
- run: npm test
This workflow is triggered on pushes to the main branch or when a pull request is opened. It runs on the latest Ubuntu environment, tests against multiple Node.js versions, and executes the build and test scripts. By automating these processes, you catch issues early and ensure that only quality code makes it to production.
Configuration Management: Taming the Infrastructure Beast
As your applications grow, so does the complexity of your infrastructure. This is where configuration management tools come in, helping you manage and provision your infrastructure as code. These tools ensure consistency across your environments, reduce manual errors, and make it easy to scale your infrastructure.
Popular Configuration Management Tools
Some of the leading players in this space include:
- Ansible
- Puppet
- Chef
- SaltStack
Let’s take a closer look at Ansible, known for its simplicity and agentless architecture.
Ansible: Infrastructure as Code Made Easy
Ansible uses YAML-based playbooks to define infrastructure configurations. Here’s a simple example of an Ansible playbook that installs and starts Nginx on a group of web servers:
---
- hosts: webservers
become: yes
tasks:
- name: Install Nginx
apt:
name: nginx
state: present
- name: Start Nginx service
service:
name: nginx
state: started
enabled: yes
- name: Copy custom Nginx configuration
template:
src: templates/nginx.conf.j2
dest: /etc/nginx/nginx.conf
notify:
- Restart Nginx
handlers:
- name: Restart Nginx
service:
name: nginx
state: restarted
This playbook installs Nginx, starts the service, copies a custom configuration file, and restarts Nginx if the configuration changes. The beauty of Ansible lies in its readability and ease of use. Even team members without extensive DevOps experience can understand and contribute to these playbooks.
Containerization: Packaging Applications for Consistency
In the world of DevOps, consistency is key. Containerization technologies like Docker have revolutionized how we package and deploy applications. Containers encapsulate an application and its dependencies, ensuring that it runs consistently across different environments – from a developer’s laptop to production servers.
Docker: The Container King
Docker has become synonymous with containerization, offering a simple yet powerful way to build, ship, and run applications. Let’s look at a basic Dockerfile that sets up a Python web application:
# Use an official Python runtime as the base image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
This Dockerfile creates a container that runs a Python application. It starts with a base Python image, sets up the working directory, copies the application code, installs dependencies, exposes a port, and specifies the command to run the application.
Container Orchestration: Managing the Container Zoo
As your application grows, you’ll likely find yourself managing multiple containers across multiple hosts. This is where container orchestration tools like Kubernetes come into play. Kubernetes automates the deployment, scaling, and management of containerized applications.
Here’s a simple example of a Kubernetes deployment manifest:
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp-deployment
labels:
app: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: myapp:1.0
ports:
- containerPort: 80
This manifest creates a deployment of three replicas of our application, ensuring high availability and easy scalability. Kubernetes handles the nitty-gritty details of scheduling these containers across your cluster, managing their lifecycle, and even rolling updates when you deploy new versions.
Monitoring and Logging: Keeping Your Finger on the Pulse
In the fast-paced world of DevOps, knowing what’s happening in your systems at all times is crucial. This is where monitoring and logging tools come into play. They help you track performance, detect issues before they become critical, and troubleshoot problems when they occur.
Prometheus: The Monitoring Maestro
Prometheus has emerged as a popular choice for monitoring in the cloud-native world. It’s an open-source system monitoring and alerting toolkit that integrates well with Kubernetes and other cloud platforms. Here’s a simple example of a Prometheus configuration file:
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'prometheus'
static_configs:
- targets: ['localhost:9090']
- job_name: 'node_exporter'
static_configs:
- targets: ['node_exporter:9100']
- job_name: 'app'
static_configs:
- targets: ['app:8080']
This configuration sets up Prometheus to scrape metrics from itself, a Node Exporter (for host-level metrics), and our application. Prometheus’s powerful query language, PromQL, allows you to create complex queries and alerts based on these metrics.
ELK Stack: The Logging Leviathan
When it comes to logging, the ELK stack (Elasticsearch, Logstash, and Kibana) is a popular choice. It allows you to collect logs from various sources, process them, and visualize them in real-time. Here’s a simple Logstash configuration that collects logs from a file and sends them to Elasticsearch:
input {
file {
path => "/var/log/myapp.log"
start_position => "beginning"
}
}
filter {
grok {
match => { "message" => "%{COMBINEDAPACHELOG}" }
}
date {
match => [ "timestamp" , "dd/MMM/yyyy:HH:mm:ss Z" ]
}
}
output {
elasticsearch {
hosts => ["localhost:9200"]
index => "myapp-logs-%{+YYYY.MM.dd}"
}
}
This configuration reads logs from a file, parses them using a predefined pattern, extracts the timestamp, and sends the structured logs to Elasticsearch. You can then use Kibana to create visualizations and dashboards based on these logs.
Infrastructure as Code: Defining Your World in Text
Infrastructure as Code (IaC) is a key DevOps practice that allows you to manage and provision your infrastructure through code instead of manual processes. This approach brings the benefits of version control, peer review, and automated testing to your infrastructure management.
Terraform: The IaC Powerhouse
Terraform has become the de facto standard for Infrastructure as Code. It allows you to define your infrastructure across multiple cloud providers using a declarative language. Here’s a simple example of a Terraform configuration that creates an AWS EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "MyWebServer"
}
}
resource "aws_security_group" "allow_http" {
name = "allow_http"
description = "Allow HTTP inbound traffic"
ingress {
description = "HTTP from VPC"
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
tags = {
Name = "allow_http"
}
}
This Terraform code creates an EC2 instance and a security group that allows HTTP traffic. The beauty of Terraform is that you can version control this code, collaborate on it with your team, and use it to create consistent environments across development, staging, and production.
Secrets Management: Keeping Your Secrets… Secret
In the world of DevOps, managing secrets (like API keys, database passwords, and other sensitive information) securely is crucial. Hardcoding secrets in your application code or configuration files is a recipe for disaster. This is where secrets management tools come in handy.
HashiCorp Vault: The Fort Knox of Secrets
HashiCorp Vault is a powerful tool for securely accessing secrets. It provides a unified interface to any secret, while providing tight access control and recording a detailed audit log. Here’s a simple example of how you might use Vault in a Python application:
import hvac
# Initialize the Vault client
client = hvac.Client(url='http://localhost:8200', token='my-root-token')
# Write a secret
client.secrets.kv.v2.create_or_update_secret(
path='my-secret',
secret=dict(password='my-super-secret-password'),
)
# Read a secret
secret = client.secrets.kv.v2.read_secret_version(path='my-secret')
password = secret['data']['data']['password']
print(f"The secret password is: {password}")
This code demonstrates writing a secret to Vault and then reading it back. In a real-world scenario, you’d use more secure authentication methods and would likely integrate Vault with your CI/CD pipeline to inject secrets into your applications at runtime.
Collaboration and Communication: Bringing It All Together
DevOps isn’t just about tools and processes – it’s also about people and communication. Effective collaboration tools are essential for any DevOps team. While there are many options available, let’s look at a couple that are particularly well-suited for DevOps workflows.
Slack: The Communication Hub
Slack has become the go-to communication platform for many tech teams. Its real-time messaging, channel-based organization, and extensive integration capabilities make it ideal for DevOps teams. You can set up channels for different projects or teams, integrate with your CI/CD tools to get build notifications, and even create custom bots to automate routine tasks.
Here’s a simple example of a Slack bot that posts a message when a new Git commit is pushed:
import os
from slack_sdk import WebClient
from slack_sdk.errors import SlackApiError
slack_token = os.environ["SLACK_API_TOKEN"]
client = WebClient(token=slack_token)
try:
response = client.chat_postMessage(
channel="#devops-alerts",
text="New commit pushed to main branch: https://github.com/your-repo/commit/abc123"
)
print(f"Message posted: {response['ts']}")
except SlackApiError as e:
print(f"Error posting message: {e}")
This script uses the Slack SDK to post a message to a specific channel whenever a new commit is pushed. You could easily integrate this into your CI/CD pipeline to keep your team informed of important events.
Jira: Tracking Work and Progress
For more structured project management and issue tracking, many DevOps teams turn to tools like Jira. Jira allows you to create and track tasks, bugs, and features, and its Agile boards are great for managing sprints and visualizing workflow.
Jira also offers a robust API that allows you to integrate it with your other DevOps tools. For example, you could automatically create a Jira ticket when a certain type of error is logged in your monitoring system:
from jira import JIRA
# Initialize the Jira client
jira = JIRA(server="https://your-domain.atlassian.net", basic_auth=("email@example.com", "api-token"))
# Create a new issue
new_issue = jira.create_issue(project='PROJ',
summary='High CPU Usage Detected',
description='CPU usage exceeded 90% for more than 5 minutes.',
issuetype={'name': 'Bug'})
print(f"Created issue: {new_issue.key}")
This script uses the Jira Python library to create a new issue when a high CPU usage event is detected. By automating the creation of tickets, you can ensure that important issues are tracked and addressed promptly.
Conclusion
As we wrap up our journey through the essential DevOps toolkit, it’s important to remember that these tools are just the beginning. The world of DevOps is constantly evolving, with new tools and practices emerging all the time. The key is to build a toolkit that works for your team and your specific needs.
Remember, DevOps is not just about the tools – it’s about fostering a culture of collaboration, continuous improvement, and shared responsibility. The tools we’ve discussed are meant to support and enhance these cultural aspects, not replace them.
Let’s recap the key areas we’ve covered:
- Version Control with Git
- CI/CD with tools like GitHub Actions
- Configuration Management with Ansible
- Containerization with Docker and Kubernetes
- Monitoring and Logging with Prometheus and ELK Stack
- Infrastructure as Code with Terraform
- Secrets Management with HashiCorp Vault
- Collaboration and Communication with Slack and Jira
Each of these tools and practices plays a crucial role in the DevOps lifecycle, from planning and development to deployment and monitoring. By integrating these tools effectively, you can create a smooth, automated pipeline that takes your code from commit to production with minimal friction.
But remember, implementing these tools is just the first step. The real power of DevOps comes from how you use these tools to improve your processes, foster collaboration, and deliver value to your customers more quickly and reliably.
As you build your DevOps toolkit, keep these principles in mind:
- Start small and iterate: Don’t try to implement everything at once. Start with the tools that will have the biggest impact on your current pain points, and gradually expand your toolkit as you become more comfortable with DevOps practices.
- Focus on automation: Look for opportunities to automate repetitive tasks. The more you can automate, the more time your team will have to focus on high-value work.
- Embrace continuous learning: The DevOps landscape is always changing. Encourage your team to stay up-to-date with new tools and practices, and be open to evolving your toolkit over time.
- Prioritize security: As you implement new tools and processes, always consider the security implications. Tools like HashiCorp Vault can help, but security should be a consideration in every aspect of your DevOps practice.
- Measure and improve: Use the monitoring and logging tools we discussed to gather data about your processes. Use this data to continually refine and improve your DevOps practices.
Building an effective DevOps toolkit is a journey, not a destination. As your team grows and your projects evolve, your toolkit will need to evolve too. The tools and practices we’ve discussed here provide a solid foundation, but don’t be afraid to explore other tools that might better suit your specific needs.
Remember, the goal of DevOps is to improve collaboration, increase efficiency, and deliver better software faster. If the tools you’re using are helping you achieve these goals, you’re on the right track. If not, don’t be afraid to reevaluate and make changes.
So, are you ready to supercharge your development and operations processes? Start building your DevOps toolkit today, and watch as your team’s productivity and efficiency soar to new heights. The future of software development is here, and it’s powered by DevOps. Happy coding!
Disclaimer: This blog post is intended for informational purposes only. The tools and practices discussed here are based on current industry trends and the author’s experience. However, the field of DevOps is rapidly evolving, and what works best for one team may not be ideal for another. Always evaluate tools and practices in the context of your own team’s needs and capabilities. If you notice any inaccuracies or have suggestions for improvement, please report them so we can update the content promptly.