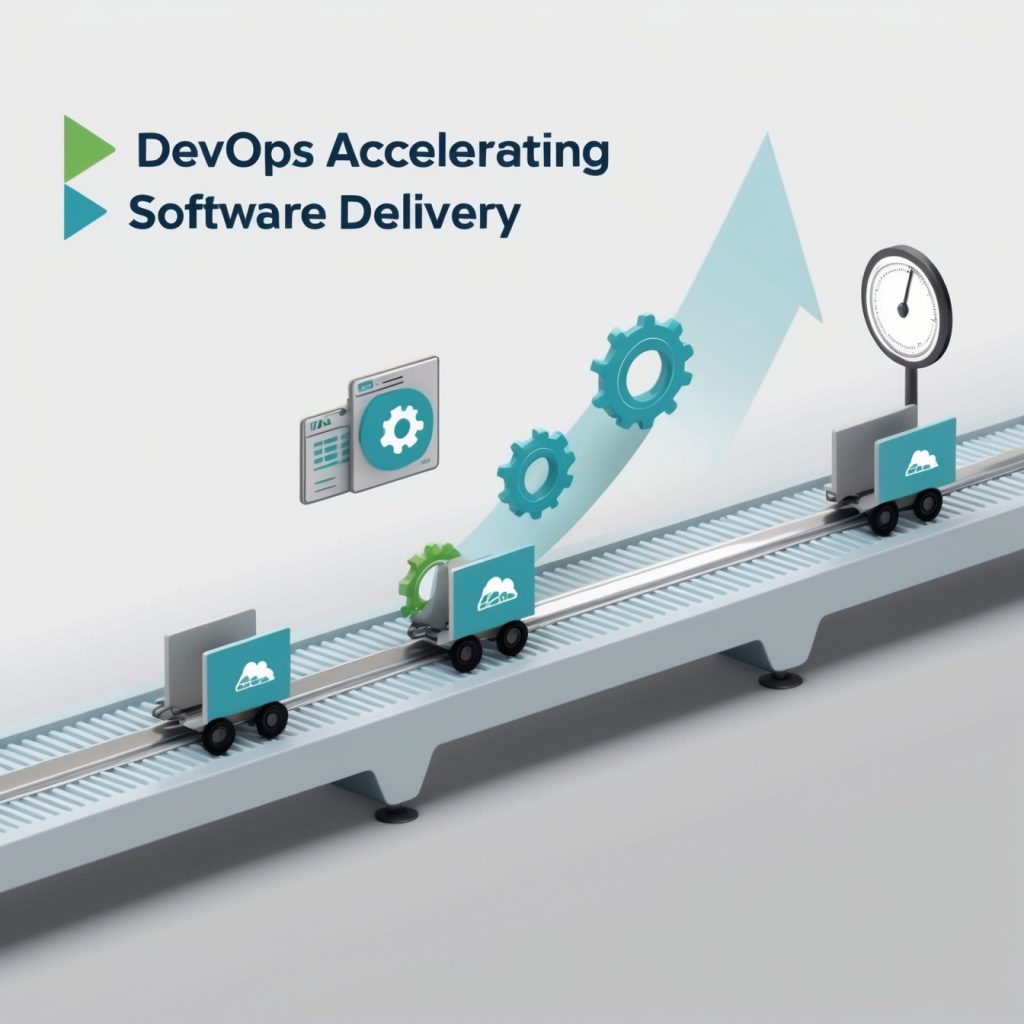
The Fast Track to Software Delivery: How DevOps Speeds Things Up
Businesses are constantly seeking ways to innovate and deliver software solutions quickly and efficiently. Enter DevOps – a game-changing approach that’s revolutionizing the way we develop, deploy, and maintain software. But what exactly is DevOps, and how does it accelerate software delivery? Let’s dive into the world of DevOps and explore how it’s transforming the software development landscape.
What is DevOps, and Why Should You Care?
DevOps, a portmanteau of “Development” and “Operations,” is more than just a buzzword – it’s a cultural shift in how organizations approach software development and IT operations. At its core, DevOps is about breaking down the traditional silos between development teams and operations teams, fostering collaboration, and automating processes to deliver high-quality software faster and more reliably.
But why should you care about DevOps? Well, if you’re involved in software development or IT operations in any capacity, DevOps has the potential to transform your work life. It’s not just about speeding things up (although that’s a significant benefit); it’s about improving the quality of your software, reducing errors, and creating a more efficient and enjoyable work environment. Whether you’re a developer, system administrator, project manager, or business leader, understanding and implementing DevOps practices can give you a competitive edge in today’s fast-moving tech landscape.
The DevOps Lifecycle: A Continuous Journey
To understand how DevOps speeds up software delivery, we need to look at the DevOps lifecycle. Unlike traditional software development models, DevOps embraces a continuous, cyclical approach that integrates development, testing, deployment, and monitoring.
Continuous Planning: The journey begins with continuous planning, where teams collaborate to define project goals, requirements, and timelines. This ongoing process ensures that everyone is aligned and can adapt to changing needs quickly.
Continuous Development: Developers write code in short iterations, often using version control systems like Git to manage changes and collaborate effectively.
Continuous Integration: As code is written, it’s regularly merged into a shared repository. Automated build and test processes catch integration issues early, preventing the dreaded “integration hell” that often plagues traditional development approaches.
Continuous Testing: Automated testing is a cornerstone of DevOps. Unit tests, integration tests, and acceptance tests are run continuously to catch bugs early and ensure code quality.
Continuous Deployment: Once code passes all tests, it can be automatically deployed to staging or production environments. This eliminates manual deployment processes and reduces the risk of human error.
Continuous Monitoring: After deployment, systems are continuously monitored for performance issues, security vulnerabilities, and user feedback. This information feeds back into the planning phase, creating a true continuous improvement cycle.
By embracing this continuous lifecycle, DevOps teams can deliver software updates faster, more frequently, and with greater confidence. But how exactly does this speed things up? Let’s explore some key DevOps practices that accelerate software delivery.
Automation: The Engine of DevOps Speed
If DevOps were a race car, automation would be its high-performance engine. Automation is at the heart of DevOps, enabling teams to move faster by eliminating manual, error-prone processes. Here are some key areas where automation accelerates software delivery:
Build Automation: Gone are the days of manually compiling code and creating build artifacts. With tools like Jenkins, GitLab CI, or GitHub Actions, teams can automate the entire build process. Here’s a simple example of a Jenkins pipeline that automates building and testing a Java application:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
This script automatically compiles the code, runs tests, and packages the application, saving developers countless hours of manual work.
Testing Automation: Manual testing is time-consuming and prone to human error. Automated testing tools like Selenium, JUnit, or Pytest allow teams to run comprehensive test suites in minutes, catching bugs early and often. Here’s a simple Python example using Pytest:
def add(a, b):
return a + b
def test_add():
assert add(2, 3) == 5
assert add(-1, 1) == 0
assert add(0, 0) == 0
With just a few lines of code, we can create tests that run automatically, ensuring our function works as expected across multiple scenarios.
Deployment Automation: Deploying software manually is slow and risky. Tools like Ansible, Puppet, or Kubernetes automate the deployment process, ensuring consistency across environments and reducing the chance of human error. Here’s a basic Ansible playbook for deploying a web application:
---
- hosts: webservers
tasks:
- name: Ensure Apache is installed
apt:
name: apache2
state: present
- name: Copy web files
copy:
src: /path/to/web/files
dest: /var/www/html
- name: Restart Apache
service:
name: apache2
state: restarted
This playbook automatically installs Apache, copies web files, and restarts the server – tasks that could take hours if done manually.
By automating these processes, DevOps teams can dramatically reduce the time it takes to go from code commit to production deployment. But automation is just one piece of the puzzle. Let’s explore some other key DevOps practices that contribute to faster software delivery.
Continuous Integration and Continuous Delivery (CI/CD): The Fast Lane to Production
Continuous Integration and Continuous Delivery (CI/CD) are fundamental DevOps practices that significantly speed up software delivery. CI/CD creates an automated pipeline that takes code from commit to production with minimal manual intervention.
Continuous Integration: CI involves frequently merging code changes into a central repository, where automated builds and tests are run. This practice catches integration issues early, preventing the buildup of conflicts that can slow down development. Tools like Jenkins, Travis CI, or GitLab CI make it easy to set up CI pipelines. Here’s an example of a simple .travis.yml file for a Node.js project:
language: node_js
node_js:
- "14"
script:
- npm install
- npm test
This configuration automatically runs tests whenever code is pushed to the repository, ensuring that new changes don’t break existing functionality.
Continuous Delivery: CD takes CI a step further by automatically deploying code to staging or production environments after it passes all tests. This eliminates manual deployment processes and allows teams to release new features and bug fixes quickly and reliably. Here’s an example of a GitHub Actions workflow that deploys a Node.js application to Heroku:
name: Deploy to Heroku
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Deploy to Heroku
uses: akhileshns/heroku-deploy@v3.12.12
with:
heroku_api_key: ${{secrets.HEROKU_API_KEY}}
heroku_app_name: "your-app-name"
heroku_email: "your-email@example.com"
This workflow automatically deploys the application to Heroku whenever changes are pushed to the main branch, eliminating the need for manual deployments.
By implementing CI/CD, teams can dramatically reduce the time between writing code and getting it into the hands of users. This not only speeds up delivery but also improves software quality by catching issues early and enabling rapid feedback loops.
Infrastructure as Code: Building the Fast Track
Infrastructure as Code (IaC) is another key DevOps practice that accelerates software delivery. IaC involves managing and provisioning infrastructure through code and automation, rather than manual processes. This approach brings several benefits:
- Consistency: IaC ensures that infrastructure is provisioned consistently every time, eliminating configuration drift and “works on my machine” issues.
- Speed: Provisioning infrastructure through code is much faster than manual setup, especially for complex environments.
- Version Control: Infrastructure code can be version-controlled like application code, making it easy to track changes and roll back if needed.
- Scalability: IaC makes it easy to scale infrastructure up or down as needed, supporting rapid growth and efficient resource use.
Tools like Terraform, AWS CloudFormation, or Ansible are commonly used for IaC. Here’s a simple Terraform example that provisions an AWS EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "example-instance"
}
}
This code defines an EC2 instance with specific parameters, allowing you to create, modify, or destroy infrastructure with simple commands. By treating infrastructure as code, DevOps teams can provision and manage complex environments quickly and reliably, further accelerating the software delivery process.
Microservices: Breaking Down the Monolith for Faster Delivery
While not exclusive to DevOps, the microservices architecture is often adopted alongside DevOps practices to speed up software delivery. Microservices involve breaking down large, monolithic applications into smaller, independently deployable services. This approach offers several advantages:
- Faster Development: Smaller services are easier to understand and modify, allowing developers to work more quickly.
- Independent Deployment: Services can be deployed independently, enabling teams to release updates to specific components without affecting the entire application.
- Scalability: Individual services can be scaled independently based on demand, improving resource utilization.
- Technology Flexibility: Different services can use different technologies, allowing teams to choose the best tool for each job.
Implementing microservices often involves using containerization technologies like Docker and orchestration platforms like Kubernetes. Here’s a simple example of a Dockerfile for a Python microservice:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["python", "app.py"]
This Dockerfile defines a lightweight container for a Python application, making it easy to deploy and scale the service independently.
While microservices can add complexity to system architecture, when implemented thoughtfully alongside DevOps practices, they can significantly speed up software delivery by enabling more frequent, targeted updates and easier scaling.
Monitoring and Feedback: Fueling Continuous Improvement
DevOps isn’t just about speeding up initial delivery – it’s about continuously improving software quality and performance. This is where monitoring and feedback loops come into play. By implementing comprehensive monitoring and rapid feedback mechanisms, DevOps teams can:
- Detect and resolve issues quickly, minimizing downtime and user impact.
- Gather real-time data on system performance and user behavior to inform future development.
- Continuously optimize applications based on actual usage patterns.
Tools like Prometheus, Grafana, or ELK stack (Elasticsearch, Logstash, Kibana) are commonly used for monitoring and log analysis in DevOps environments. Here’s a simple example of a Prometheus configuration file:
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'prometheus'
static_configs:
- targets: ['localhost:9090']
- job_name: 'app'
static_configs:
- targets: ['app:8080']
This configuration sets up Prometheus to scrape metrics from itself and an application every 15 seconds, providing real-time data on system performance.
By implementing robust monitoring and feedback systems, DevOps teams can respond quickly to issues, make data-driven decisions, and continuously improve their software, leading to faster, more reliable delivery over time.
Collaboration and Communication: The Human Side of Speed
While we’ve focused a lot on technical practices, it’s important to remember that DevOps is as much about culture and collaboration as it is about tools and processes. Effective communication and collaboration between development, operations, and other stakeholders are crucial for speeding up software delivery. Here are some ways DevOps fosters better collaboration:
- Shared Responsibility: DevOps breaks down silos, encouraging developers and operations teams to work together throughout the software lifecycle.
- Continuous Feedback: Regular communication and feedback loops ensure that issues are caught and addressed quickly.
- Cross-Functional Teams: DevOps often involves creating cross-functional teams that can handle all aspects of software delivery, reducing handoffs and improving efficiency.
- Knowledge Sharing: DevOps culture encourages sharing knowledge and best practices across the organization, leading to continuous improvement.
Tools like Slack, Microsoft Teams, or Jira can facilitate this collaboration, but the key is fostering a culture of open communication and shared responsibility. By improving how teams work together, DevOps can significantly speed up decision-making and problem-solving, further accelerating software delivery.
Overcoming Challenges: Navigating the Fast Lane
While DevOps offers numerous benefits, implementing it successfully can be challenging. Organizations often face hurdles such as:
- Resistance to Change: Shifting to a DevOps culture can be met with resistance from team members accustomed to traditional methods.
- Skill Gaps: DevOps requires a broad skill set that spans development, operations, and automation.
- Tool Overload: With so many DevOps tools available, choosing and integrating the right ones can be overwhelming.
- Security Concerns: Rapid delivery must be balanced with robust security practices.
Overcoming these challenges requires a strategic approach:
- Start Small: Begin with pilot projects to demonstrate the value of DevOps before scaling across the organization.
- Invest in Training: Provide comprehensive training to help team members acquire the necessary skills.
- Choose Tools Wisely: Select tools that integrate well and meet your specific needs, rather than adopting every new technology.
- Prioritize Security: Implement “DevSecOps” practices that integrate security throughout the development lifecycle.
By addressing these challenges head-on, organizations can successfully implement DevOps and reap the benefits of faster, more reliable software delivery.
The Future of DevOps: Accelerating Towards Tomorrow
As technology continues to evolve, so too will DevOps practices. Looking ahead, we can expect to see:
- Increased AI and Machine Learning Integration: AI-powered tools will help automate more complex tasks and provide deeper insights into system performance and user behavior.
- Serverless Architecture: The growth of serverless computing will further abstract infrastructure management, allowing teams to focus even more on code and features.
- GitOps: Version control systems like Git will play an even larger role in managing not just code, but entire system configurations and deployments.
- DevOps for Edge Computing: As edge computing grows, DevOps practices will need to adapt to manage distributed systems at scale.
- Continued Focus on Security: DevSecOps will become increasingly important as organizations seek to balance rapid delivery with robust security measures.
These trends promise to further accelerate software delivery, enabling organizations to innovate and respond to market changes even faster.
Embracing the DevOps Fast Track
DevOps has fundamentally changed the way we approach software delivery, offering a fast track to innovation and customer value. By breaking down silos, automating processes, and fostering a culture of collaboration and continuous improvement, DevOps enables organizations to deliver high-quality software faster and more reliably than ever before.
From continuous integration and delivery to infrastructure as code, from microservices to comprehensive monitoring, DevOps practices provide a toolkit for accelerating every stage of the software lifecycle. And while implementing DevOps can be challenging, the benefits in terms of speed, quality, and team satisfaction make it a worthwhile journey.
As we look to the future, it’s clear that DevOps will continue to evolve, offering even more ways to speed up software delivery. Organizations that embrace DevOps now will be well-positioned to take advantage of these advances, staying ahead in an increasingly competitive digital landscape.
So, are you ready to hit the fast track? Whether you’re just starting your DevOps journey or looking to optimize your existing practices, remember that DevOps is not just about tools or processes – it’s about creating a culture of collaboration, automation, and continuous improvement. By embracing these principles, you can accelerate your software delivery and drive your organization towards greater success in the digital age.
Disclaimer: This blog post is intended for informational purposes only. While we strive to provide accurate and up-to-date information, the field of DevOps is rapidly evolving, and specific practices and tools may change over time. Always consult with IT professionals and refer to official documentation when implementing DevOps practices in your organization. If you notice any inaccuracies in this post, please report them so we can correct them promptly.