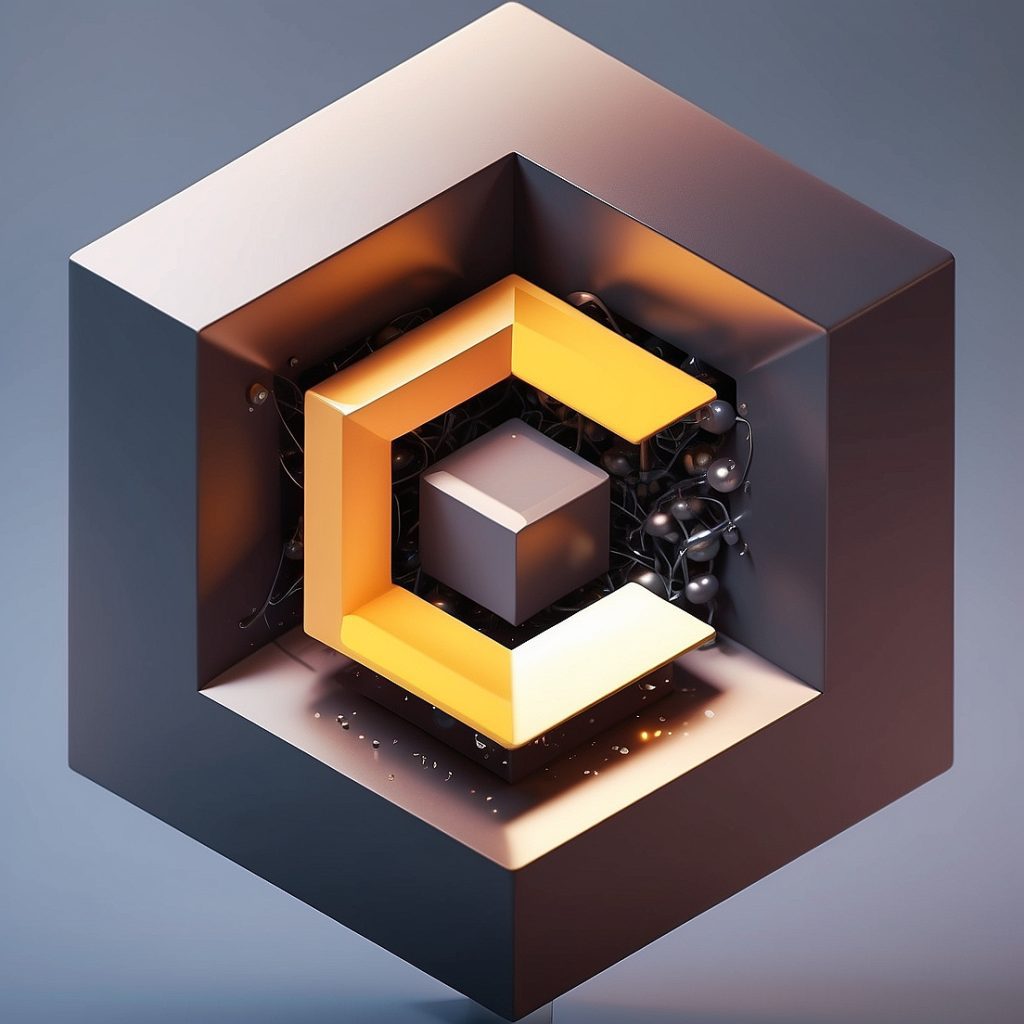
The Future of DeFi on Cronos: Faster, Cheaper, and More Accessible?
Decentralized Finance, or DeFi, has revolutionized the financial landscape by providing decentralized, permissionless, and open alternatives to traditional financial services. At the heart of this revolution is the ability to mint tokens, a process that has gained significant traction on various blockchain platforms. Among these, Cronos Chain stands out with its promise of making DeFi faster, cheaper, and more accessible. This article delves into how Cronos is redefining DeFi, with a focus on the minting process, and includes ample code snippets and scripts to demonstrate its capabilities.
The Cronos Chain Advantage
Speed and Efficiency
One of the primary advantages of the Cronos Chain is its speed. Built on the Cosmos SDK and utilizing the Tendermint consensus mechanism, Cronos is capable of processing transactions at a high throughput. This efficiency is crucial for DeFi applications, where the speed of transaction execution can significantly impact user experience.
Here’s a simple code snippet to illustrate the process of sending a transaction on Cronos:
from web3 import Web3
# Connect to Cronos node
w3 = Web3(Web3.HTTPProvider('https://cronos-testnet-3.crypto.org:8545'))
# Check connection
if w3.isConnected():
print("Connected to Cronos")
# Set up the transaction
tx = {
'nonce': w3.eth.getTransactionCount('0xYourAddress'),
'to': '0xRecipientAddress',
'value': w3.toWei(1, 'ether'),
'gas': 2000000,
'gasPrice': w3.toWei('50', 'gwei')
}
# Sign the transaction
signed_tx = w3.eth.account.signTransaction(tx, private_key='YourPrivateKey')
# Send the transaction
tx_hash = w3.eth.sendRawTransaction(signed_tx.rawTransaction)
print(f"Transaction sent with hash: {tx_hash.hex()}")
Cost-Effectiveness
Transaction fees are a major concern in the DeFi space. High gas fees on networks like Ethereum can be prohibitive for users, particularly those making small transactions. Cronos addresses this issue by offering significantly lower transaction costs, making DeFi more accessible to a broader audience.
Interoperability
Cronos is designed to be interoperable with other blockchains, particularly Ethereum, thanks to its EVM compatibility. This means that developers can easily port their Ethereum-based dApps to Cronos, leveraging its speed and lower costs without having to rewrite their codebases.
Minting Tokens on Cronos
The Basics of Minting
Minting tokens is a fundamental aspect of many DeFi applications. It involves creating new tokens on a blockchain, which can then be used for various purposes, such as staking, trading, or governance. On Cronos, minting tokens is straightforward and can be accomplished with a few lines of code.
Creating an ERC-20 Token
Let’s start by creating a basic ERC-20 token on Cronos. ERC-20 is a widely adopted token standard that defines a set of functions for tokens.
Here’s a simple example in Solidity:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
}
To deploy this contract on Cronos, you can use tools like Remix, Hardhat, or Truffle. Here’s an example using Hardhat:
Hardhat Setup
- Install Hardhat:
npm install --save-dev hardhat
- Create a Hardhat project:
npx hardhat
- Configure Hardhat to use Cronos:
Edithardhat.config.js
to include the Cronos network configuration:
require("@nomiclabs/hardhat-waffle");
module.exports = {
solidity: "0.8.0",
networks: {
cronos: {
url: "https://cronos-testnet-3.crypto.org:8545",
accounts: [`0x${process.env.PRIVATE_KEY}`]
}
}
};
- Deploy the Contract:
Create a new filescripts/deploy.js
:
async function main() {
const [deployer] = await ethers.getSigners();
console.log("Deploying contracts with the account:", deployer.address);
const Token = await ethers.getContractFactory("MyToken");
const token = await Token.deploy(1000000);
console.log("Token deployed to:", token.address);
}
main()
.then(() => process.exit(0))
.catch(error => {
console.error(error);
process.exit(1);
});
Run the deployment script:
npx hardhat run scripts/deploy.js --network cronos
Minting More Tokens
Once your token contract is deployed, you might want to mint additional tokens. This can be done by adding a mint function to your contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyToken is ERC20, Ownable {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
function mint(address to, uint256 amount) public onlyOwner {
_mint(to, amount);
}
}
With this modification, only the contract owner can mint new tokens, ensuring controlled inflation.
Leveraging Cronos for DeFi Applications
Staking Mechanisms
Staking is a popular DeFi application where users lock their tokens to earn rewards. On Cronos, you can easily implement staking mechanisms. Below is a simple example of a staking contract in Solidity:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract Staking is Ownable {
IERC20 public stakingToken;
uint256 public rewardRate;
mapping(address => uint256) public stakingBalance;
mapping(address => uint256) public rewards;
constructor(address _stakingToken, uint256 _rewardRate) {
stakingToken = IERC20(_stakingToken);
rewardRate = _rewardRate;
}
function stake(uint256 _amount) external {
stakingToken.transferFrom(msg.sender, address(this), _amount);
stakingBalance[msg.sender] += _amount;
}
function unstake(uint256 _amount) external {
require(stakingBalance[msg.sender] >= _amount, "Insufficient balance");
stakingBalance[msg.sender] -= _amount;
stakingToken.transfer(msg.sender, _amount);
}
function distributeRewards() external onlyOwner {
for (uint i = 0; i < stakers.length; i++) {
address staker = stakers[i];
uint256 reward = stakingBalance[staker] * rewardRate / 100;
rewards[staker] += reward;
}
}
}
Yield Farming
Yield farming is another DeFi strategy where users earn rewards by providing liquidity to pools. Implementing a yield farming contract on Cronos follows similar principles. Here’s an example:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract YieldFarm is Ownable {
IERC20 public lpToken;
IERC20 public rewardToken;
uint256 public rewardRate;
mapping(address => uint256) public stakedBalance;
mapping(address => uint256) public rewards;
constructor(address _lpToken, address _rewardToken, uint256 _rewardRate) {
lpToken = IERC20(_lpToken);
rewardToken = IERC20(_rewardToken);
rewardRate = _rewardRate;
}
function stake(uint256 _amount) external {
lpToken.transferFrom(msg.sender, address(this), _amount);
stakedBalance[msg.sender] += _amount;
}
function unstake(uint256 _amount) external {
require(stakedBalance[msg.sender] >= _amount, "Insufficient balance");
stakedBalance[msg.sender] -= _amount;
lpToken.transfer(msg.sender, _amount);
}
function distributeRewards() external onlyOwner {
for (uint i = 0; i < stakers.length; i++) {
address staker = stakers[i];
uint256 reward = stakedBalance[staker] * rewardRate / 100;
rewards[staker] += reward;
rewardToken.transfer(staker, reward);
}
}
}
The Future of DeFi on Cronos
Accessibility for Developers
Cronos is committed to lowering the barriers for developers entering the DeFi space. With comprehensive documentation, developer tools, and community support, Cronos makes it easier for developers to build, deploy, and scale their applications.
Community and Ecosystem Growth
The success of a blockchain platform often hinges on its community and ecosystem. Cronos has been fostering a vibrant community of developers, enthusiasts, and users. This collaborative environment accelerates innovation and adoption, driving the platform’s growth.
Regulatory Considerations
As DeFi continues to grow, regulatory scrutiny will likely increase. Cron
os is positioned to navigate these challenges by providing a secure and compliant infrastructure that can adapt to evolving regulatory landscapes.
The future of DeFi on Cronos looks promising. With its emphasis on speed, cost-efficiency, and accessibility, Cronos is well-equipped to lead the next wave of DeFi innovation. Whether you’re a developer looking to mint tokens, build staking mechanisms, or create yield farming contracts, Cronos offers the tools and infrastructure to bring your vision to life.
As we continue to explore the capabilities of Cronos, we can expect to see a new generation of DeFi applications that are faster, cheaper, and more accessible than ever before. The minting mania is just the beginning – the possibilities are limitless.