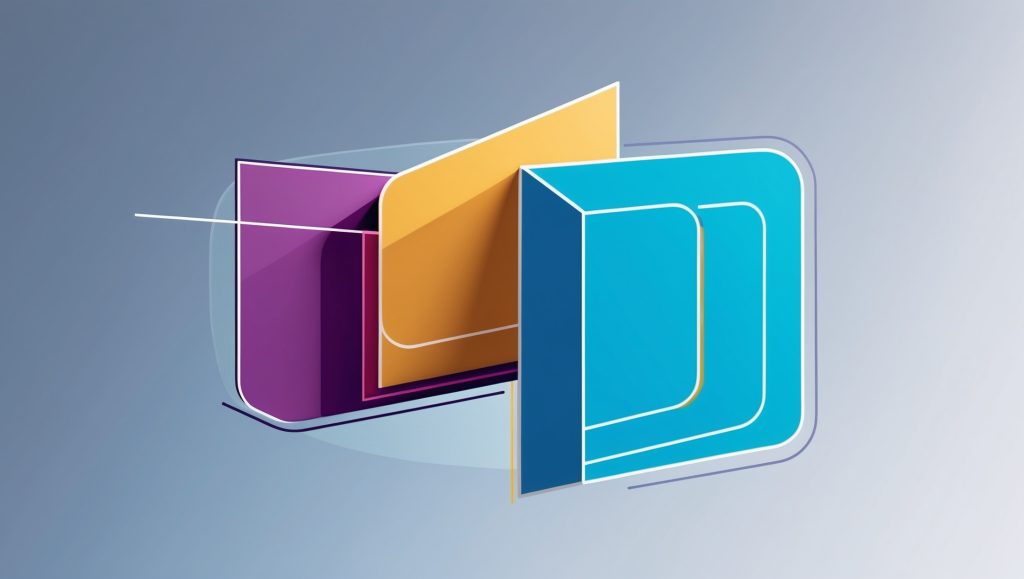
The Model-View-Presenter (MVP) Pattern
The evolution of software architecture patterns has consistently aimed to improve code organization, maintainability, and testability. Among these patterns, the Model-View-Presenter (MVP) pattern stands out as a powerful variation of the traditional Model-View-Controller (MVC) pattern. MVP has gained significant traction in modern application development due to its ability to handle complex presentation logic more effectively while maintaining a clear separation of concerns. This comprehensive guide explores the MVP pattern’s core concepts, implementation strategies, and practical applications in both desktop and mobile development environments. By understanding MVP’s nuances and advantages, developers can make informed decisions about incorporating this architectural pattern into their projects, ultimately leading to more maintainable and testable applications.
Understanding MVP Architecture
The Model-View-Presenter pattern is an architectural pattern that evolved from the MVC pattern, specifically designed to address some of the limitations and complexities found in traditional MVC implementations. MVP introduces a more defined separation between the presentation logic and the view, making it particularly suitable for applications with complex user interfaces and interaction requirements. The pattern consists of three main components: Model, View, and Presenter, each with distinct responsibilities and interactions.
Core Components of MVP
- **Model**: The Model component represents the data and business logic layer of the application. It contains the business rules, data validation, and data manipulation logic. The Model is completely independent of the user interface and is unaware of how its data will be displayed.
- **View**: The View component is responsible for displaying data to the user and capturing user interactions. In MVP, the View is passive and delegates all user actions to the Presenter. It should contain minimal logic, primarily focusing on UI-related code.
- **Presenter**: The Presenter acts as an intermediary between the Model and View. It contains the presentation logic, handles user input forwarded by the View, and updates the View with data from the Model. The Presenter is independent of the specific UI implementation, making it easier to test and maintain.
- Delegate all user interactions to the Presenter
- Contain no business logic
- Not directly modify the Model
- Focus solely on UI-related code
- Define clear View interfaces that the Presenter can work with
- Use interface segregation to keep interfaces focused and minimal
- Consider using events or callbacks for communication between components
- Model: Business logic and data
- View: Display and user input
- Presenter: Coordination and presentation logic
- Write unit tests for the Presenter without UI dependencies
- Mock the View interface for testing presentation logic
- Test the Model independently of the UI
Key Differences Between MVP and MVC
Understanding the distinctions between MVP and MVC is crucial for implementing the right pattern for your specific use case. Here’s a detailed comparison:
Aspect | MVP | MVC |
---|---|---|
View-Logic Coupling | Loose coupling through interface | Direct coupling |
Controller/Presenter Role | Handles presentation logic | Handles navigation and flow |
View Independence | View is passive | View can be active |
Testing Complexity | Easier to test | More complex testing |
Data Flow | Bidirectional through Presenter | Can be multidirectional |
UI Logic Location | Concentrated in Presenter | Distributed between View and Controller |
Implementing MVP in Python
Let’s explore a practical implementation of the MVP pattern in Python using a simple task management application.
# Model
class TaskModel:
def __init__(self):
self.tasks = []
def add_task(self, task):
if task and isinstance(task, str):
self.tasks.append({"title": task, "completed": False})
return True
return False
def get_tasks(self):
return self.tasks.copy()
def complete_task(self, index):
if 0 <= index < len(self.tasks):
self.tasks[index]["completed"] = True
return True
return False
# View Interface
from abc import ABC, abstractmethod
class TaskView(ABC):
@abstractmethod
def display_tasks(self, tasks):
pass
@abstractmethod
def get_user_input(self):
pass
@abstractmethod
def show_message(self, message):
pass
# Concrete View Implementation
class ConsoleTaskView(TaskView):
def display_tasks(self, tasks):
print("\n=== Task List ===")
for i, task in enumerate(tasks):
status = "✓" if task["completed"] else " "
print(f"{i+1}. [{status}] {task['title']}")
def get_user_input(self):
return input("Enter new task (or 'q' to quit): ")
def show_message(self, message):
print(message)
# Presenter
class TaskPresenter:
def __init__(self, model, view):
self.model = model
self.view = view
def add_new_task(self, task_title):
if self.model.add_task(task_title):
self.view.show_message("Task added successfully!")
else:
self.view.show_message("Failed to add task!")
self.update_view()
def complete_task(self, task_number):
if self.model.complete_task(task_number - 1):
self.view.show_message("Task marked as completed!")
else:
self.view.show_message("Invalid task number!")
self.update_view()
def update_view(self):
tasks = self.model.get_tasks()
self.view.display_tasks(tasks)
def run(self):
while True:
self.update_view()
user_input = self.view.get_user_input()
if user_input.lower() == 'q':
break
try:
if user_input.startswith('complete '):
task_num = int(user_input.split()[1])
self.complete_task(task_num)
else:
self.add_new_task(user_input)
except ValueError:
self.view.show_message("Invalid input!")
# Usage Example
if __name__ == "__main__":
model = TaskModel()
view = ConsoleTaskView()
presenter = TaskPresenter(model, view)
presenter.run()
Implementing MVP in Java
Here’s an equivalent implementation of the task management application in Java, demonstrating MVP pattern principles:
// Model
import java.util.ArrayList;
import java.util.List;
class Task {
private String title;
private boolean completed;
public Task(String title) {
this.title = title;
this.completed = false;
}
public String getTitle() {
return title;
}
public boolean isCompleted() {
return completed;
}
public void setCompleted(boolean completed) {
this.completed = completed;
}
}
class TaskModel {
private List<Task> tasks;
public TaskModel() {
tasks = new ArrayList<>();
}
public boolean addTask(String taskTitle) {
if (taskTitle != null && !taskTitle.trim().isEmpty()) {
tasks.add(new Task(taskTitle));
return true;
}
return false;
}
public List<Task> getTasks() {
return new ArrayList<>(tasks);
}
public boolean completeTask(int index) {
if (index >= 0 && index < tasks.size()) {
tasks.get(index).setCompleted(true);
return true;
}
return false;
}
}
// View Interface
interface TaskView {
void displayTasks(List<Task> tasks);
String getUserInput();
void showMessage(String message);
}
// Concrete View Implementation
class ConsoleTaskView implements TaskView {
private Scanner scanner;
public ConsoleTaskView() {
scanner = new Scanner(System.in);
}
@Override
public void displayTasks(List<Task> tasks) {
System.out.println("\n=== Task List ===");
for (int i = 0; i < tasks.size(); i++) {
Task task = tasks.get(i);
String status = task.isCompleted() ? "✓" : " ";
System.out.printf("%d. [%s] %s%n", i + 1, status, task.getTitle());
}
}
@Override
public String getUserInput() {
System.out.print("Enter new task (or 'q' to quit): ");
return scanner.nextLine();
}
@Override
public void showMessage(String message) {
System.out.println(message);
}
}
// Presenter
class TaskPresenter {
private TaskModel model;
private TaskView view;
public TaskPresenter(TaskModel model, TaskView view) {
this.model = model;
this.view = view;
}
public void addNewTask(String taskTitle) {
if (model.addTask(taskTitle)) {
view.showMessage("Task added successfully!");
} else {
view.showMessage("Failed to add task!");
}
updateView();
}
public void completeTask(int taskNumber) {
if (model.completeTask(taskNumber - 1)) {
view.showMessage("Task marked as completed!");
} else {
view.showMessage("Invalid task number!");
}
updateView();
}
private void updateView() {
view.displayTasks(model.getTasks());
}
public void run() {
while (true) {
updateView();
String userInput = view.getUserInput();
if (userInput.toLowerCase().equals("q")) {
break;
}
try {
if (userInput.startsWith("complete ")) {
int taskNum = Integer.parseInt(userInput.split(" ")[1]);
completeTask(taskNum);
} else {
addNewTask(userInput);
}
} catch (NumberFormatException e) {
view.showMessage("Invalid input!");
}
}
}
}
// Main Application
public class TaskManagementApp {
public static void main(String[] args) {
TaskModel model = new TaskModel();
TaskView view = new ConsoleTaskView();
TaskPresenter presenter = new TaskPresenter(model, view);
presenter.run();
}
}
Best Practices and Design Considerations
When implementing the MVP pattern, several best practices should be followed to ensure maximum benefit from the architecture:
1. Keep the View Passive
The View should be as passive as possible, implementing a simple interface that the Presenter can use to update it. This approach, known as the Passive View pattern, makes the View easier to test and maintain. The View should:
2. Design Clear Interfaces
Well-defined interfaces between components are crucial for maintaining loose coupling:
3. Maintain Proper Separation of Concerns
Each component should have a single responsibility:
4. Consider Testing Requirements
MVP’s architecture naturally supports testing:
Common Implementation Patterns
Several common patterns emerge when implementing MVP in real-world applications:
1. Supervising Presenter Pattern
This variation allows the View to handle simple data bindings directly while the Presenter handles complex logic:
class SupervisingPresenter:
def __init__(self, model, view):
self.model = model
self.view = view
# Set up basic data bindings
self.view.set_data_bindings(self.model)
# Handle complex logic
self.view.set_complex_action_handler(self.handle_complex_action)
2. Passive View Pattern
This pattern maximizes testability by making the View completely passive:
class PassiveView:
def __init__(self):
self.display_value = None
def set_display_value(self, value):
self.display_value = value
self.update_display()
def update_display(self):
# Only handle UI updates
pass
Testing MVP Applications
One of MVP’s major advantages is its testability. Here’s an example of testing a Presenter:
import unittest
from unittest.mock import Mock
class TaskPresenterTests(unittest.TestCase):
def setUp(self):
self.model = Mock()
self.view = Mock()
self.presenter = TaskPresenter(self.model, self.view)
def test_add_task_success(self):
# Arrange
self.model.add_task.return_value = True
# Act
self.presenter.add_new_task("Test Task")
# Assert
self.model.add_task.assert_called_once_with("Test Task")
self.view.show_message.assert_called_with("Task added successfully!")
Conclusion
The Model-View-Presenter pattern provides a robust architecture for applications with complex user interfaces and presentation logic. Its clear separation of concerns, enhanced testability, and flexible implementation options make it an excellent choice for many modern applications. While it may require more initial setup than simpler patterns, the benefits in terms of maintainability, testability, and code organization often outweigh the initial investment. By following the best practices and implementation patterns outlined in this guide, developers can successfully leverage MVP to create more maintainable and scalable applications.
Disclaimer: The code examples and implementation patterns presented in this article are for educational purposes and may need to be adapted for specific use cases and requirements. While we strive for accuracy, software development practices and patterns continue to evolve. Please report any inaccuracies or suggest improvements to help us maintain the quality of this content. The implementations shown may be simplified for clarity and might need additional error handling and security considerations for production use.