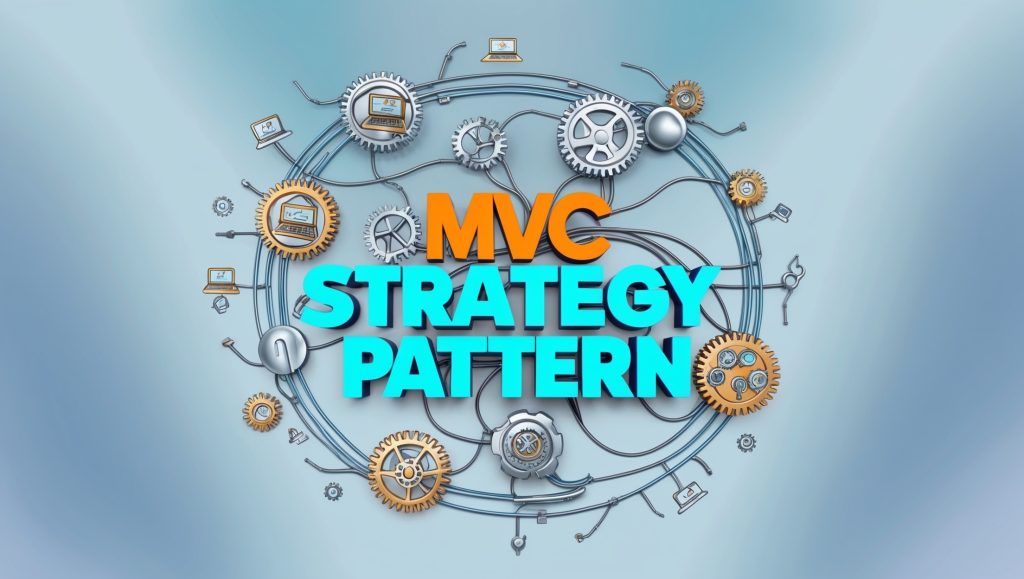
The Strategy Pattern in MVC
The Strategy Pattern stands as one of the most powerful and flexible design patterns in software engineering, particularly within the Model-View-Controller (MVC) architecture. This behavioral design pattern enables applications to dynamically select and switch between different algorithms or strategies at runtime, promoting code reusability, maintainability, and adherence to the Open-Closed Principle. In modern software development, where applications must adapt to changing requirements and support multiple algorithmic approaches, the Strategy Pattern provides an elegant solution for managing complexity and ensuring code flexibility. This comprehensive guide delves deep into the implementation, benefits, and practical applications of the Strategy Pattern within MVC architecture, supported by real-world examples in both Python and Java.
Understanding the Strategy Pattern
Core Concepts
The Strategy Pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It lets the algorithm vary independently from clients that use it. This pattern involves three key components: the Context, the Strategy interface, and Concrete Strategies. The Context maintains a reference to a Strategy object and delegates the actual algorithm execution to this object. The Strategy interface defines a common interface for all supported algorithms, while Concrete Strategies implement specific algorithms following this interface. This separation of concerns allows for easy addition of new algorithms without modifying existing code, exemplifying the Open-Closed Principle of SOLID design principles.
Integration with MVC Architecture
MVC Components and Strategy Pattern Synergy
The Model-View-Controller architecture naturally complements the Strategy Pattern, creating a robust framework for managing complex application logic. In an MVC application, the Strategy Pattern typically resides within the Model layer, where it handles various business logic algorithms. The Controller acts as the coordinator, selecting appropriate strategies based on user input or system conditions, while the View remains completely isolated from the algorithmic implementations. This separation creates a clean, maintainable codebase where algorithmic variations can be managed effectively without impacting the presentation layer.
Let’s examine a practical implementation in Python:
from abc import ABC, abstractmethod
# Strategy Interface
class SortingStrategy(ABC):
@abstractmethod
def sort(self, data):
pass
# Concrete Strategies
class QuickSort(SortingStrategy):
def sort(self, data):
if len(data) <= 1:
return data
pivot = data[len(data) // 2]
left = [x for x in data if x < pivot]
middle = [x for x in data if x == pivot]
right = [x for x in data if x > pivot]
return self.sort(left) + middle + self.sort(right)
class MergeSort(SortingStrategy):
def sort(self, data):
if len(data) <= 1:
return data
mid = len(data) // 2
left = self.sort(data[:mid])
right = self.sort(data[mid:])
return self._merge(left, right)
def _merge(self, left, right):
result = []
i = j = 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Context (Model)
class SortingModel:
def __init__(self, strategy: SortingStrategy):
self._strategy = strategy
self._data = []
def set_strategy(self, strategy: SortingStrategy):
self._strategy = strategy
def set_data(self, data):
self._data = data
def sort_data(self):
return self._strategy.sort(self._data)
# Controller
class SortingController:
def __init__(self, model: SortingModel):
self._model = model
def handle_sorting_request(self, data, strategy_name):
strategies = {
'quick': QuickSort(),
'merge': MergeSort()
}
if strategy_name in strategies:
self._model.set_strategy(strategies[strategy_name])
self._model.set_data(data)
return self._model.sort_data()
else:
raise ValueError(f"Unknown sorting strategy: {strategy_name}")
Real-World Applications
Dynamic Algorithm Selection
In real-world applications, the Strategy Pattern proves invaluable when dealing with scenarios requiring dynamic algorithm selection. Consider an e-commerce platform that needs to calculate shipping costs based on different carrier services, each with its unique pricing algorithm. Here’s a Java implementation demonstrating this concept:
// Strategy Interface
public interface ShippingStrategy {
double calculateShippingCost(Order order);
}
// Concrete Strategies
public class FedExStrategy implements ShippingStrategy {
@Override
public double calculateShippingCost(Order order) {
double baseCost = 15.00;
double weightMultiplier = 0.5;
return baseCost + (order.getWeight() * weightMultiplier);
}
}
public class UPSStrategy implements ShippingStrategy {
@Override
public double calculateShippingCost(Order order) {
double baseCost = 12.00;
double weightMultiplier = 0.6;
return baseCost + (order.getWeight() * weightMultiplier);
}
}
// Model
public class ShippingModel {
private ShippingStrategy strategy;
private Order order;
public void setStrategy(ShippingStrategy strategy) {
this.strategy = strategy;
}
public void setOrder(Order order) {
this.order = order;
}
public double calculateShipping() {
return strategy.calculateShippingCost(order);
}
}
// Controller
public class ShippingController {
private ShippingModel model;
public ShippingController(ShippingModel model) {
this.model = model;
}
public double processShippingCalculation(Order order, String carrier) {
ShippingStrategy strategy;
switch (carrier.toLowerCase()) {
case "fedex":
strategy = new FedExStrategy();
break;
case "ups":
strategy = new UPSStrategy();
break;
default:
throw new IllegalArgumentException("Unknown carrier: " + carrier);
}
model.setStrategy(strategy);
model.setOrder(order);
return model.calculateShipping();
}
}
Best Practices and Implementation Guidelines
Pattern Implementation Considerations
When implementing the Strategy Pattern in an MVC architecture, several best practices should be followed to ensure optimal results. Here’s a comprehensive table outlining key considerations:
Aspect | Recommendation | Rationale |
---|---|---|
Strategy Interface | Keep it focused and minimal | Ensures easy implementation of new strategies |
Context Class | Implement strategy switching mechanism | Allows runtime algorithm selection |
Strategy Creation | Use Factory Pattern or DI | Reduces coupling and improves testability |
Error Handling | Implement robust validation | Prevents runtime errors from invalid strategy selection |
Performance | Consider strategy caching | Improves performance for frequently used strategies |
Advanced Implementation Techniques
Combining with Other Design Patterns
The Strategy Pattern can be enhanced by combining it with other design patterns. Here’s an example implementing a Strategy Factory with Registry pattern in Python:
from typing import Dict, Type
from abc import ABC, abstractmethod
# Strategy Interface
class PaymentStrategy(ABC):
@abstractmethod
def process_payment(self, amount: float) -> bool:
pass
# Concrete Strategies
class CreditCardStrategy(PaymentStrategy):
def process_payment(self, amount: float) -> bool:
print(f"Processing credit card payment of ${amount}")
return True
class PayPalStrategy(PaymentStrategy):
def process_payment(self, amount: float) -> bool:
print(f"Processing PayPal payment of ${amount}")
return True
# Strategy Factory with Registry
class PaymentStrategyFactory:
_strategies: Dict[str, Type[PaymentStrategy]] = {}
@classmethod
def register_strategy(cls, name: str, strategy: Type[PaymentStrategy]):
cls._strategies[name] = strategy
@classmethod
def create_strategy(cls, name: str) -> PaymentStrategy:
if name not in cls._strategies:
raise ValueError(f"Unknown payment strategy: {name}")
return cls._strategies[name]()
# Register strategies
PaymentStrategyFactory.register_strategy("credit_card", CreditCardStrategy)
PaymentStrategyFactory.register_strategy("paypal", PayPalStrategy)
# Model
class PaymentModel:
def __init__(self):
self._strategy = None
def set_strategy(self, strategy: PaymentStrategy):
self._strategy = strategy
def process_payment(self, amount: float) -> bool:
if not self._strategy:
raise ValueError("Payment strategy not set")
return self._strategy.process_payment(amount)
# Controller
class PaymentController:
def __init__(self, model: PaymentModel):
self._model = model
def handle_payment(self, amount: float, payment_method: str) -> bool:
try:
strategy = PaymentStrategyFactory.create_strategy(payment_method)
self._model.set_strategy(strategy)
return self._model.process_payment(amount)
except ValueError as e:
print(f"Error: {e}")
return False
Performance Optimization and Scaling
Strategy Caching and Lazy Loading
For applications requiring high performance, implementing strategy caching and lazy loading can significantly improve efficiency. Here’s an example in Java demonstrating these concepts:
public class StrategyCache {
private static final Map<String, ShippingStrategy> strategyCache = new ConcurrentHashMap<>();
public static ShippingStrategy getStrategy(String carrier) {
return strategyCache.computeIfAbsent(carrier, StrategyCache::createStrategy);
}
private static ShippingStrategy createStrategy(String carrier) {
switch (carrier.toLowerCase()) {
case "fedex":
return new FedExStrategy();
case "ups":
return new UPSStrategy();
default:
throw new IllegalArgumentException("Unknown carrier: " + carrier);
}
}
}
Testing and Maintenance
Unit Testing Strategies
Testing implementations of the Strategy Pattern requires thorough coverage of both individual strategies and their integration within the MVC architecture. Here’s an example of unit tests in Python using pytest:
import pytest
from typing import List
def test_sorting_strategies():
# Test data
data: List[int] = [64, 34, 25, 12, 22, 11, 90]
expected_result = sorted(data)
# Test QuickSort strategy
model = SortingModel(QuickSort())
model.set_data(data.copy())
assert model.sort_data() == expected_result
# Test MergeSort strategy
model.set_strategy(MergeSort())
model.set_data(data.copy())
assert model.sort_data() == expected_result
def test_invalid_strategy():
controller = SortingController(SortingModel(QuickSort()))
with pytest.raises(ValueError):
controller.handle_sorting_request([1, 2, 3], "invalid_strategy")
Conclusion
The Strategy Pattern, when properly implemented within an MVC architecture, provides a powerful mechanism for managing algorithmic complexity and maintaining code flexibility. Through careful consideration of implementation details, performance optimization, and testing strategies, developers can create robust applications that efficiently handle varying business requirements. The pattern’s ability to separate algorithmic implementation from business logic, combined with its support for runtime algorithm selection, makes it an invaluable tool in modern software development.
Disclaimer: The code examples and implementations provided in this blog post are for educational purposes and may require additional error handling, security measures, and optimizations for production use. While we strive for accuracy, technology evolves rapidly, and some information may become outdated. Please report any inaccuracies to our editorial team for prompt correction.