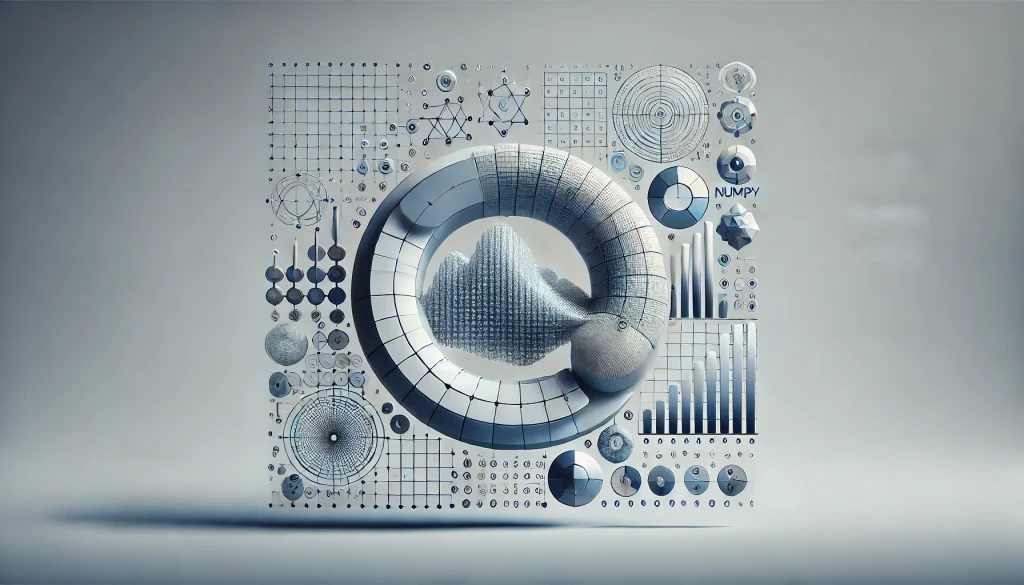
The Ultimate Guide to NumPy for AI Enthusiasts
Hey there, AI enthusiasts! If you’re diving into the exciting world of artificial intelligence (AI), you’ve probably heard about NumPy. It’s a fundamental package for scientific computing in Python and a game-changer when working with data. Whether you’re dealing with linear algebra, random number generation, or simply performing array operations, NumPy is your go-to library. So, let’s embark on this journey and explore why NumPy is indispensable for anyone venturing into AI.
What is NumPy?
NumPy stands for Numerical Python, and it’s a powerful library that provides support for arrays, matrices, and many mathematical functions. It is designed for efficient operations on large datasets, making it an essential tool in the AI toolkit.
Why Use NumPy?
- Performance: NumPy operations are executed at the C level, making them extremely fast.
- Convenience: It provides a plethora of built-in functions for array manipulations, which are both easy to use and efficient.
- Community: Being open-source, it boasts a large and active community, ensuring continuous improvement and support.
Installing NumPy
Getting started with NumPy is a breeze. You can install it using pip, the Python package installer.
pip install numpy
Once installed, you can start using it by importing it into your Python scripts:
import numpy as np
Basic NumPy Operations
Let’s start with some fundamental operations. We’ll cover array creation, basic arithmetic, and some handy functions.
Creating Arrays
Creating arrays in NumPy is simple. Here’s how you can create different types of arrays:
# Creating a 1D array
array_1d = np.array([1, 2, 3, 4, 5])
# Creating a 2D array
array_2d = np.array([[1, 2, 3], [4, 5, 6]])
# Creating an array of zeros
zeros_array = np.zeros((3, 3))
# Creating an array of ones
ones_array = np.ones((2, 4))
# Creating an array with a range of values
range_array = np.arange(10)
# Creating an array with random values
random_array = np.random.random((3, 3))
Array Arithmetic
NumPy allows you to perform arithmetic operations element-wise. This means you can add, subtract, multiply, and divide arrays with ease.
array_a = np.array([1, 2, 3])
array_b = np.array([4, 5, 6])
# Adding arrays
sum_array = array_a + array_b
# Subtracting arrays
diff_array = array_b - array_a
# Multiplying arrays
prod_array = array_a * array_b
# Dividing arrays
quot_array = array_b / array_a
Useful Array Functions
NumPy comes packed with a variety of useful functions that make array manipulation a breeze.
# Reshaping an array
reshaped_array = np.reshape(array_1d, (5, 1))
# Transposing an array
transposed_array = np.transpose(array_2d)
# Finding the maximum value
max_value = np.max(array_2d)
# Finding the minimum value
min_value = np.min(array_2d)
# Calculating the sum of all elements
sum_all = np.sum(array_2d)
# Calculating the mean of all elements
mean_value = np.mean(array_2d)
Advanced NumPy Techniques for AI
Now that we’ve covered the basics, let’s dive into some advanced techniques that are particularly useful in AI applications.
Broadcasting
Broadcasting is a powerful feature in NumPy that allows you to perform arithmetic operations on arrays of different shapes. It automatically expands the smaller array to match the shape of the larger one.
array_c = np.array([1, 2, 3])
array_d = np.array([[4], [5], [6]])
# Broadcasting allows this operation
broadcast_sum = array_c + array_d
Vectorization
Vectorization is the process of converting iterative operations into vector operations, which can be executed much faster. NumPy supports vectorized operations, making computations more efficient.
# Traditional loop-based approach
result = []
for i in range(len(array_a)):
result.append(array_a[i] + array_b[i])
# Vectorized approach
vectorized_result = array_a + array_b
Matrix Operations
In AI, matrices are often used to represent data. NumPy provides robust support for matrix operations, making it easy to work with these data structures.
matrix_a = np.array([[1, 2], [3, 4]])
matrix_b = np.array([[5, 6], [7, 8]])
# Matrix multiplication
matrix_product = np.dot(matrix_a, matrix_b)
# Element-wise multiplication
elementwise_product = matrix_a * matrix_b
# Inverse of a matrix
matrix_inverse = np.linalg.inv(matrix_a)
# Determinant of a matrix
matrix_determinant = np.linalg.det(matrix_a)
Working with Large Datasets
NumPy excels at handling large datasets efficiently, a crucial capability in AI where data size can be substantial.
Memory Management
Efficient memory management is vital when working with large datasets. NumPy provides several functions to optimize memory usage.
# Creating an array with a specific data type
large_array = np.array([1, 2, 3], dtype=np.int32)
# Checking the memory size of an array
memory_size = large_array.nbytes
Loading and Saving Data
NumPy makes it easy to load and save data, which is essential for working with large datasets.
# Saving an array to a file
np.save('my_array.npy', array_1d)
# Loading an array from a file
loaded_array = np.load('my_array.npy')
Real-World AI Applications
Let’s explore some real-world AI applications where NumPy plays a pivotal role.
Data Preprocessing
Before feeding data into an AI model, it often needs to be preprocessed. NumPy provides various functions to simplify this process.
# Normalizing data
data = np.random.random((5, 5))
normalized_data = (data - np.mean(data)) / np.std(data)
# Splitting data into training and testing sets
data_length = len(data)
train_size = int(0.8 * data_length)
train_data = data[:train_size]
test_data = data[train_size:]
Feature Engineering
Feature engineering involves creating new features from raw data to improve the performance of machine learning models. NumPy is invaluable in this process.
# Generating polynomial features
from sklearn.preprocessing import PolynomialFeatures
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1)
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
Model Evaluation
Evaluating the performance of AI models is crucial. NumPy makes it easy to calculate various metrics.
# Calculating Mean Squared Error
predictions = np.array([2.5, 0.0, 2, 8])
targets = np.array([3, -0.5, 2, 7])
mse = np.mean((predictions - targets) ** 2)
Integrating NumPy with Other Libraries
NumPy seamlessly integrates with many other libraries commonly used in AI.
Pandas
Pandas is a powerful library for data manipulation and analysis. It integrates well with NumPy, allowing for efficient data processing.
import pandas as pd
# Creating a DataFrame from a NumPy array
df = pd.DataFrame(data, columns=['A', 'B', 'C', 'D', 'E'])
# Converting a DataFrame to a NumPy array
array_from_df = df.values
Matplotlib
Matplotlib is a plotting library for creating static, animated, and interactive visualizations in Python. It works hand-in-hand with NumPy for data visualization.
import matplotlib.pyplot as plt
# Plotting a simple graph
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('sin(x)')
plt.title('Sine Wave')
plt.show()
Scikit-learn
Scikit-learn is a popular machine learning library that leverages NumPy for efficient computations.
from sklearn.linear_model import LinearRegression
# Generating some data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1, 2, 3, 4, 5])
# Creating and training a linear regression model
model = LinearRegression()
model.fit(X, y)
# Making predictions
predictions = model.predict(X)
Practical Tips for Using NumPy
To wrap things up, here are some practical tips to get the most out of NumPy in your AI projects.
Use Vectorized Operations
Whenever possible, use vectorized operations instead of loops. They are faster and more efficient.
Leverage Broadcasting
Understand and leverage broadcasting to simplify your code and improve performance.
Profile Your Code
Use profiling tools to identify bottlenecks in your code and optimize them. NumPy offers functions like np.einsum
for complex operations, which can be more efficient than traditional methods.
Stay Updated
NumPy is continuously evolving. Stay updated with the latest releases and best practices by following the official documentation and community forums.
Documentation and Community
NumPy has extensive documentation and a vibrant community. Whenever you encounter issues or need advanced tips, refer to the NumPy Documentation or participate in forums like Stack Overflow.
Efficient Data Handling
When dealing with large datasets, be mindful of memory consumption. Use functions like np.memmap
for memory-mapped file support, allowing you to work with large arrays without loading them entirely into memory.
Debugging and Testing
Use debugging tools and write tests for your NumPy-based code. Libraries like pytest
can help ensure your code is robust and reliable. NumPy itself offers various testing utilities under numpy.testing
.
Array Types
Understand the different array types and data types in NumPy. Using the right data type (like np.float32
instead of np.float64
) can save memory and improve performance.
Interoperability with Other Libraries
NumPy arrays are the standard for numerical data in Python. Ensure smooth interoperability with other libraries like Pandas, SciPy, TensorFlow, and PyTorch by converting data to and from NumPy arrays as needed.
Real-World Projects Using NumPy
Let’s look at some real-world projects where NumPy is heavily utilized, providing a practical context for its application in AI.
Image Processing
NumPy is often used in image processing tasks. Images are typically represented as arrays, making NumPy a natural choice for handling image data.
import matplotlib.pyplot as plt
import numpy as np
import imageio
# Load an image
image = imageio.imread('path/to/image.jpg')
# Convert to grayscale
gray_image = np.dot(image[...,:3], [0.2989, 0.5870, 0.1140])
# Display the image
plt.imshow(gray_image, cmap=plt.get_cmap('gray'))
plt.show()
Natural Language Processing (NLP)
NumPy is also used in NLP tasks for handling text data, embeddings, and more.
# Example: Converting sentences to embeddings using a hypothetical function
def sentence_to_vector(sentence):
# This is a placeholder function
return np.random.random(300)
sentences = ["Hello, world!", "NumPy is awesome."]
embeddings = np.array([sentence_to_vector(s) for s in sentences])
# Calculating cosine similarity between embeddings
from numpy.linalg import norm
def cosine_similarity(vec1, vec2):
return np.dot(vec1, vec2) / (norm(vec1) * norm(vec2))
similarity = cosine_similarity(embeddings[0], embeddings[1])
print("Cosine Similarity:", similarity)
Financial Analysis
In finance, NumPy is used for various analyses, including time-series analysis, portfolio optimization, and risk management.
# Example: Calculating daily returns of a stock
prices = np.array([100, 101, 102, 105, 107])
returns = np.diff(prices) / prices[:-1]
# Calculate mean and standard deviation of returns
mean_return = np.mean(returns)
std_return = np.std(returns)
print("Mean Return:", mean_return)
print("Standard Deviation of Return:", std_return)
NumPy in AI and Machine Learning Pipelines
Data Preparation
In AI pipelines, preparing and cleaning data is a critical step. NumPy simplifies operations like handling missing values, normalizing data, and transforming features.
# Handling missing values
data = np.array([1, 2, np.nan, 4, 5])
cleaned_data = np.nan_to_num(data, nan=np.mean(data))
# Normalizing data
normalized_data = (cleaned_data - np.min(cleaned_data)) / (np.max(cleaned_data) - np.min(cleaned_data))
Feature Selection
NumPy aids in feature selection by providing tools for statistical analysis and matrix operations.
# Correlation matrix
correlation_matrix = np.corrcoef(data)
# Selecting features based on correlation threshold
threshold = 0.5
selected_features = np.where(correlation_matrix > threshold)
Model Evaluation and Improvement
After training models, NumPy helps in evaluating and improving them by computing metrics and performing statistical tests.
# Computing R-squared for regression models
actual_values = np.array([1, 2, 3, 4, 5])
predicted_values = np.array([1.1, 1.9, 3.05, 3.9, 5.1])
r_squared = 1 - (np.sum((actual_values - predicted_values) ** 2) / np.sum((actual_values - np.mean(actual_values)) ** 2))
print("R-squared:", r_squared)
Conclusion
NumPy is a cornerstone of the AI ecosystem, providing the performance and versatility required to handle complex data operations efficiently. Whether you’re preprocessing data, performing matrix operations, or evaluating models, NumPy is the tool that will streamline your workflow and enhance your productivity.
This guide has covered the essentials and some advanced techniques to help you get started with NumPy in your AI projects. Remember, the key to mastering NumPy is practice. So, roll up your sleeves, write some code, and see the magic unfold!
Happy coding, and may your AI projects be ever successful!
Disclaimer: This guide is meant for educational purposes. While every effort has been made to ensure the accuracy of the content, we welcome feedback and corrections. Please report any inaccuracies so we can correct them promptly.