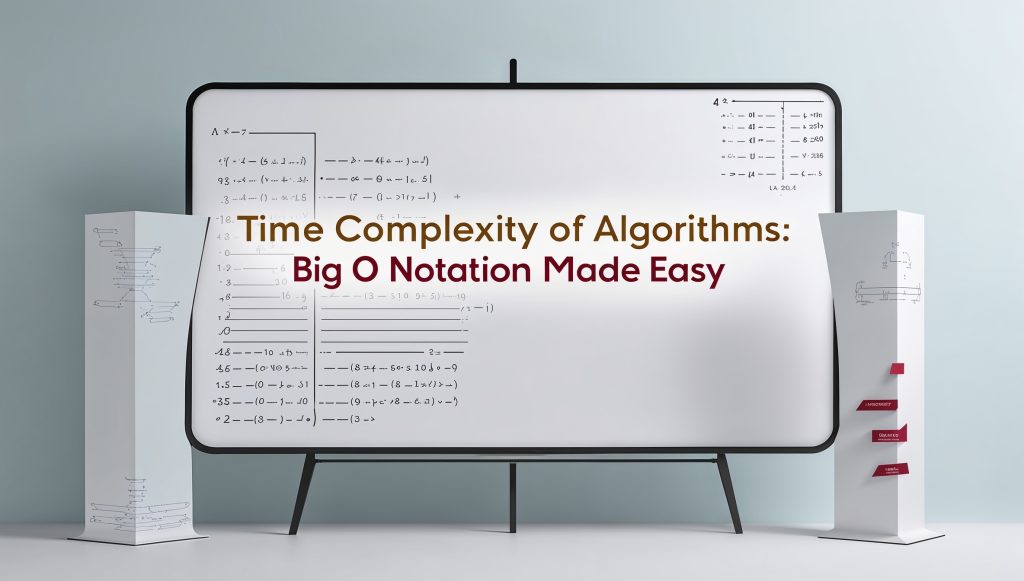
Time Complexity of Algorithms: Big O Notation Made Easy
Introduction: The Great Algorithm Race
Imagine you’re planning a trip from London to Paris. You have several options: a high-speed train that zooms across the Channel in just over two hours, a leisurely bike ride that might take several days, or even a snail-paced walk that could last weeks. Each method will get you there, but the time it takes varies dramatically.
In the world of computer science, algorithms are like these different modes of transportation. They’re methods for solving problems or performing tasks, but just like our travel options, some algorithms are lightning-fast while others move at a snail’s pace. This is where the concept of time complexity comes into play.
Understanding time complexity is crucial for writing efficient code. It helps you choose the right algorithm for the job, ensuring your programs run smoothly even as they handle larger and more complex sets of data. In this blog post, we’ll demystify time complexity and its trusty sidekick, Big O notation, making it easy for you to understand how efficient your code really is.
What is Time Complexity?
Time complexity is a way to describe how the running time of an algorithm grows as the size of its input increases. In simpler terms, it’s about answering the question: “As we give our algorithm more stuff to process, how much longer will it take?”
Let’s break this down with a simple example:
Scenario 1: Finding a name in a phone book
Imagine you’re looking for a specific name in a phone book. If the book is organized alphabetically, you can use a binary search method. You’d start in the middle, see if your name comes before or after, then repeat the process in the correct half. This is very efficient and takes roughly the same amount of time whether the phone book has 100 or 10,000 names.
Scenario 2: Finding a name in an unsorted list
Now imagine the same phone book, but it’s completely unsorted. To find a name, you’d have to check every single entry until you find the right one. If you’re unlucky and the name is at the end, you’d have to go through the entire book. In this case, the time it takes grows directly with the size of the book.
Time complexity focuses on this growth rate. It’s not about the exact number of seconds an algorithm takes, but how the running time increases as the input gets larger.
Introducing Big O Notation
Now that we understand the concept of time complexity, let’s introduce a way to express it concisely: Big O notation.
Big O notation is like a speed rating for algorithms. It gives us a simplified way to talk about how an algorithm’s running time or space requirements grow as the input size gets larger. The “O” stands for “Order of,” so when we say an algorithm has “O(n) time complexity,” we’re saying it has “order of n” complexity.
Let’s look at some common Big O notations:
- O(1) – Constant Time
This is the Usain Bolt of algorithms. No matter how big the input gets, it always takes the same amount of time. Example: Accessing an array element by its index.
def get_first_element(arr):
return arr[0]
Whether the array has 10 elements or 10 million, this function always takes the same amount of time.
- O(n) – Linear Time
This is like a steady jogger. The time increases linearly with the input size. Example: Finding the maximum value in an unsorted array.
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
If the array size doubles, the time it takes roughly doubles too.
- O(log n) – Logarithmic Time
This is the clever sprinter of algorithms. It gets more efficient as the input size grows. Example: Binary search in a sorted array.
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
Each step eliminates half of the remaining elements, making it very efficient for large datasets.
- O(n^2) – Quadratic Time
This is like a tired walker who slows down as the journey gets longer. The time increases quadratically with the input size. Example: Bubble sort algorithm.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
As the array size grows, the time increases much more rapidly.
To visualize these different time complexities, imagine plotting them on a graph where the x-axis is the input size and the y-axis is the time taken:
[Insert a graph showing the growth rates of O(1), O(log n), O(n), and O(n^2)]
As you can see, O(1) remains constant, O(log n) grows very slowly, O(n) increases linearly, and O(n^2) shoots up rapidly as the input size increases.
Calculating Time Complexity
Now that we’re familiar with Big O notation, let’s learn how to calculate the time complexity of an algorithm. The key is to focus on how the number of operations grows with the input size, particularly for large inputs.
Here’s a step-by-step approach:
- Identify the input: Determine what variable represents the size of the input. It’s often denoted as ‘n’.
- Count the operations: Look at how many operations (like comparisons or arithmetic operations) are performed in relation to the input size.
- Find the dominant term: As the input size grows, which term has the most significant impact on the running time?
- Drop constants and lower-order terms: In Big O notation, we’re only concerned with the rate of growth for large inputs, so we can simplify by dropping constants and lower-order terms.
Let’s walk through some examples:
Example 1: Simple loop
def sum_array(arr):
total = 0
for num in arr:
total += num
return total
- The input size is the length of the array, let’s call it n.
- The loop runs n times, performing one addition each time.
- The number of operations grows linearly with n.
- We drop the constants.
Time complexity: O(n)
Example 2: Nested loops
def print_pairs(arr):
n = len(arr)
for i in range(n):
for j in range(n):
print(arr[i], arr[j])
- The input size is the length of the array, n.
- The outer loop runs n times, and for each iteration, the inner loop runs n times.
- The total number of print operations is n * n = n^2.
- We drop any constants.
Time complexity: O(n^2)
Example 3: Logarithmic complexity
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
- The input size is the length of the array, n.
- In each iteration, we eliminate half of the remaining elements.
- The number of iterations is roughly log2(n).
- We drop the base of the logarithm as it’s considered a constant in Big O notation.
Time complexity: O(log n)
When analyzing time complexity, it’s important to consider different scenarios:
- Best-case: The most favorable input, resulting in the quickest execution.
- Average-case: The expected performance for a typical input.
- Worst-case: The most unfavorable input, resulting in the slowest execution.
In Big O notation, we usually focus on the worst-case scenario, as it gives us an upper bound on the algorithm’s performance.
Why Big O Matters
Understanding time complexity and Big O notation isn’t just an academic exercise—it has real-world implications in software development. Here’s why it matters:
- Performance at Scale: An algorithm that works fine for small inputs might become painfully slow as the data size grows. Big O helps you predict how your code will perform with larger datasets.
- Choosing the Right Algorithm: When faced with multiple ways to solve a problem, understanding time complexity helps you choose the most efficient approach for your specific needs.
- Optimization: Knowing the time complexity of your code helps you identify bottlenecks and areas for improvement.
- System Design: When designing large-scale systems, understanding time complexity is crucial for ensuring the system can handle the expected load efficiently.
Let’s look at a practical example:
Imagine you’re building a search function for an e-commerce site with millions of products. You have two algorithms to choose from:
- Linear search (O(n))
- Binary search on a sorted list (O(log n))
For a small shop with 100 products, both might seem fast:
- Linear search: ~100 operations
- Binary search: ~7 operations (log2(100) ≈ 6.64)
But what happens when your shop grows to 1,000,000 products?
- Linear search: ~1,000,000 operations
- Binary search: ~20 operations (log2(1,000,000) ≈ 19.93)
The difference is staggering! The linear search would be noticeably slow, potentially frustrating users and losing sales. The binary search, however, remains lightning-fast even with a million products.
This example illustrates why choosing algorithms with appropriate time complexity is crucial for building scalable and responsive applications.
Beyond the Basics
While we’ve focused on time complexity, it’s worth mentioning that there are other aspects of algorithm analysis:
- Space Complexity: This measures how much memory an algorithm uses in relation to the input size. It’s expressed using the same Big O notation.
- Amortized Analysis: This technique looks at the average performance of a sequence of operations, rather than just the worst-case scenario for a single operation.
- More Time Complexity Notations: We’ve covered some common ones, but there are others you might encounter:
- O(n log n): Common in efficient sorting algorithms like Merge Sort and Quick Sort.
- O(2^n): Exponential time, often seen in brute-force algorithms for NP-hard problems.
- O(n!): Factorial time, seen in algorithms that generate all permutations of an input.
These concepts add depth to your understanding of algorithm efficiency, but mastering the basics of Big O notation will take you a long way in writing and analyzing efficient code.
Conclusion
Time complexity and Big O notation may seem daunting at first, but they’re invaluable tools in a programmer’s toolkit. They provide a common language for discussing algorithm efficiency and help us make informed decisions about our code.
Remember these key takeaways:
- Time complexity describes how an algorithm’s running time grows with input size.
- Big O notation gives us a simplified way to express time complexity.
- Focus on the dominant term and consider the worst-case scenario when analyzing algorithms.
- Understanding time complexity helps in choosing the right algorithm, optimizing code, and designing scalable systems.
As you continue your programming journey, practice analyzing the time complexity of different algorithms you encounter. With time, it will become second nature, helping you write more efficient and scalable code.
Keep exploring, keep learning, and happy coding!
Additional Resources
For those eager to dive deeper into the world of algorithm analysis and Big O notation, here are some excellent resources:
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Grokking Algorithms” by Aditya Bhargava
- Coursera course: “Algorithms Specialization” by Stanford University
- MIT OpenCourseWare: “Introduction to Algorithms”
- YouTube channel: “mycodeschool” for visual explanations of algorithms and their complexities
Remember, mastering these concepts takes practice. Don’t be discouraged if it doesn’t click immediately—keep at it, and you’ll soon find yourself naturally thinking about efficiency as you code.
Disclaimer:
While this blog post aims to simplify the concepts of time complexity and Big O notation, it provides a foundational understanding. Algorithm analysis can be a complex topic with nuances and advanced techniques. Readers are encouraged to explore further resources and delve deeper into the subject for a more comprehensive understanding.