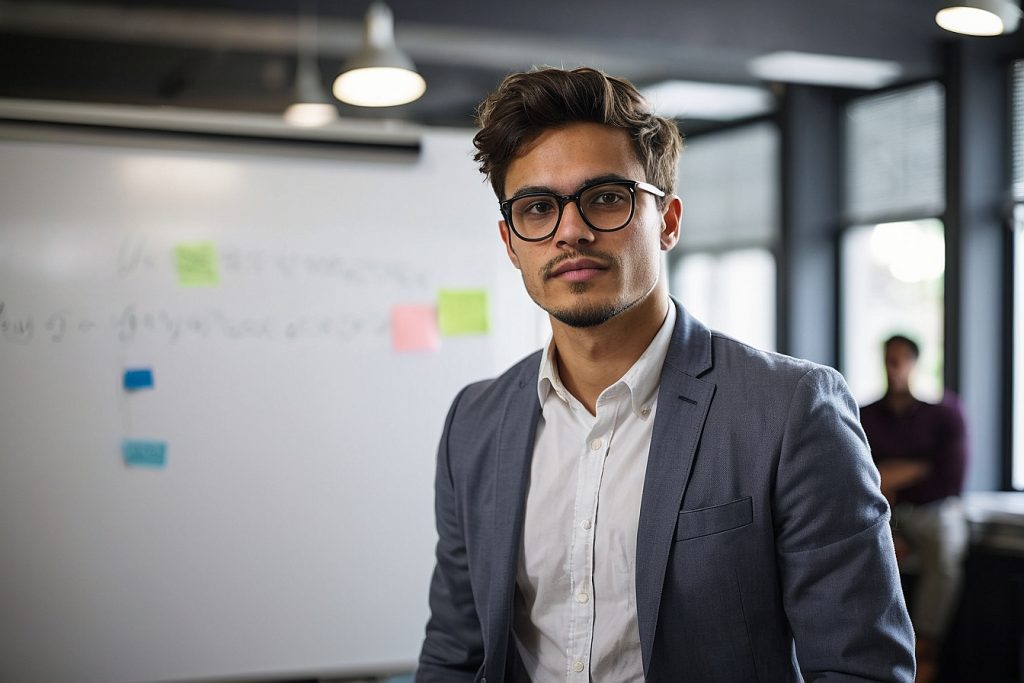
Top 10 Commonly Used Design Patterns Every Software Engineer Should Know
Design patterns are reusable solutions to common recurring problems in software design. They represent best practices that have been developed and refined by experienced software engineers over many years of software development. Design patterns are not specific pieces of code, but rather conceptual models that provide a general approach to solving a particular design problem.
The use of design patterns in software development offers numerous benefits. They promote code reusability, maintainability, and flexibility, as well as provide a common vocabulary for developers to communicate more effectively. By leveraging proven solutions to common problems, developers can focus on the unique aspects of their applications, rather than reinventing the wheel. Additionally, design patterns can improve code readability and make it easier for new developers to understand and maintain existing codebases. Overall, mastering design patterns is an essential skill for any software engineer looking to write high-quality, scalable, and maintainable code.
1. Singleton
The Singleton design pattern ensures that a class has only one instance and provides a global point of access to it. This pattern is particularly useful when you need to control access to shared resources or when you want to manage a single instance of an object throughout your application.
2. Factory Method
The Factory Method pattern is a creational pattern that provides an interface for creating objects in a super class while allowing subclasses to alter the type of objects that will be created. This pattern promotes loose coupling and provides a more flexible way of creating objects, as the client doesn’t need to know the exact class of the object being created.
3. Abstract Factory
The Abstract Factory pattern is an extension of the Factory Method pattern. It provides a way to create families of related or dependent objects without specifying their concrete classes. This pattern is particularly useful when you want to create a set of objects that share a common theme, such as UI components in a specific style.
4. Prototype
The Prototype pattern allows you to create new objects by cloning existing ones, instead of creating them from scratch. This can be particularly useful when object creation is expensive or complex, as it promotes a more efficient way to create new instances.
5. Builder
The Builder pattern is a creational pattern that allows you to separate the construction of a complex object from its representation. The pattern promotes the creation of different representations of an object using the same construction process. This is particularly useful when you need to create multiple variations of an object with different configurations.
6. Observer
The Observer pattern is a behavioral design pattern that defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is commonly used in event-driven systems, as it helps you decouple the components that produce events from those that consume them.
7. Strategy
The Strategy pattern is a behavioral design pattern that enables you to define a family of algorithms, encapsulate each one, and make them interchangeable. This pattern allows the algorithm to vary independently from clients that use it, promoting flexibility and maintainability in your software.
8. Command
The Command pattern is a behavioral design pattern that turns a request into a stand-alone object that contains all information about the request. This pattern allows you to decouple the sender of a request from its receiver, enabling you to parameterize objects with different requests and support undoable operations.
9. Adapter
The Adapter pattern is a structural design pattern that allows you to make incompatible interfaces compatible. This pattern is particularly useful when you need to integrate existing components into a new system without modifying their source code. The Adapter pattern acts as a wrapper, providing a new interface that translates the original interface into one that the new system can work with.
10. Decorator
The Decorator pattern is a structural design pattern that allows you to attach new responsibilities to an object dynamically. This pattern provides a flexible alternative to subclassing for extending functionality, as it allows you to add or remove responsibilities from an object at runtime.
Design patterns are a powerful tool for software engineers, providing proven solutions to common problems and promoting the development of maintainable, flexible, and scalable software. By mastering these top 10 commonly used design patterns, you’ll be well-equipped to tackle the challenges that come your way in the world of software engineering.