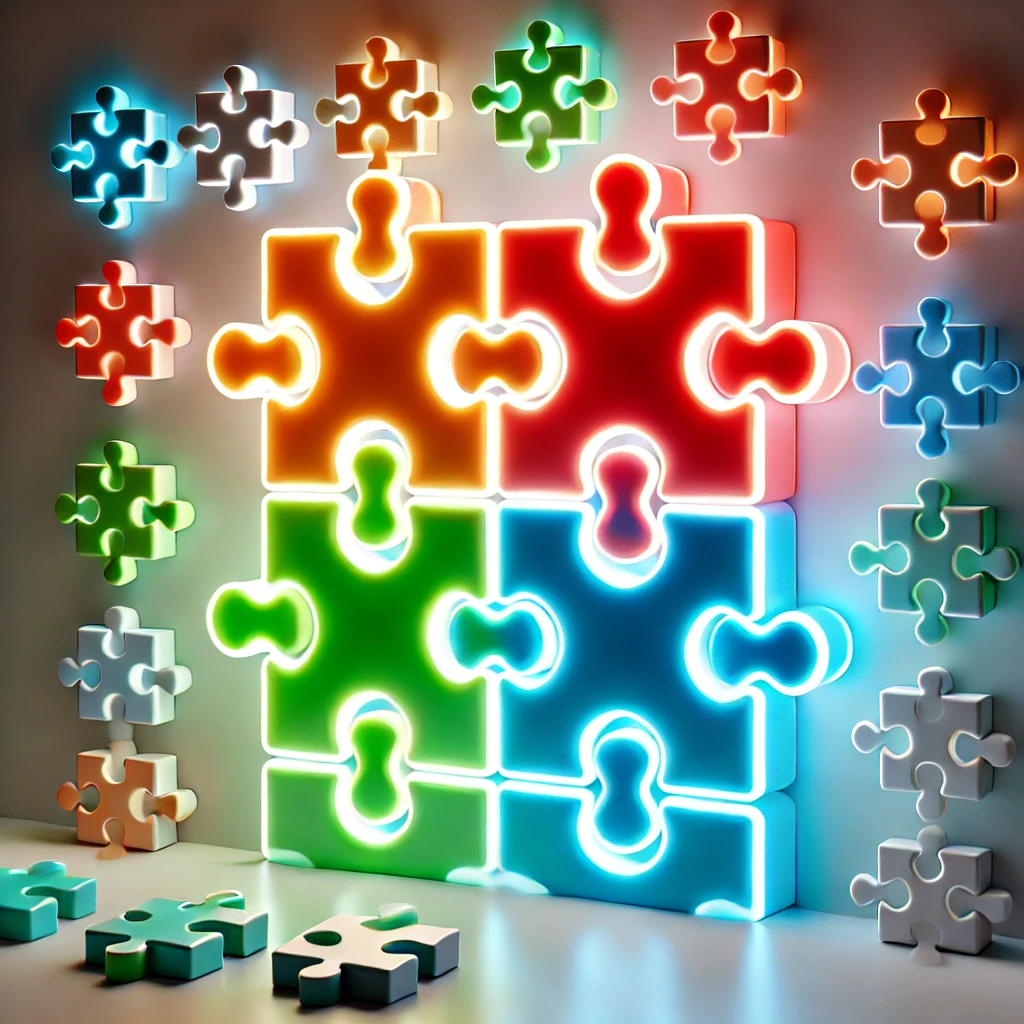
Understanding Auto-Configuration in Spring Boot
Spring Boot has revolutionized the way developers create and deploy Spring applications. One of its most powerful features is auto-configuration, which simplifies the process of setting up and configuring Spring applications. This blog post delves deep into the world of Spring Boot auto-configuration, exploring its mechanics, benefits, and best practices. Whether you’re a seasoned Spring developer or just starting your journey with Spring Boot, this comprehensive guide will enhance your understanding of this crucial feature and help you leverage it effectively in your projects.
What is Auto-Configuration?
Auto-configuration is a cornerstone feature of Spring Boot that aims to reduce the complexity of application setup and configuration. It automatically configures your Spring application based on the dependencies present in your classpath and the properties you’ve defined. This approach significantly reduces the amount of boilerplate code and configuration that developers need to write, allowing them to focus more on business logic and less on infrastructure setup.
The primary goal of auto-configuration is to provide a “convention over configuration” approach. It makes educated guesses about how you want to configure your application based on common use cases and best practices. This means that for many scenarios, you can get a fully functional application up and running with minimal manual configuration.
How Auto-Configuration Works
To truly understand auto-configuration, it’s essential to delve into its inner workings. Spring Boot’s auto-configuration is not magic; it follows a well-defined process to determine what configurations to apply to your application.
The Auto-Configuration Process:
- Classpath Scanning: When your Spring Boot application starts up, it scans the classpath for specific auto-configuration classes.
- Condition Evaluation: Each auto-configuration class contains a set of conditions that must be met for it to be applied.
- Bean Creation: If the conditions are met, Spring Boot creates and configures the necessary beans.
- Property Binding: Auto-configuration classes often use properties from your
application.properties
orapplication.yml
file to customize the created beans.
Let’s break down each of these steps in more detail:
Classpath Scanning
Spring Boot uses the @EnableAutoConfiguration
annotation (which is part of @SpringBootApplication
) to trigger the auto-configuration process. This annotation tells Spring Boot to start looking for auto-configuration classes. These classes are typically found in the spring-boot-autoconfigure
jar, but they can also be provided by other dependencies or even custom-written.
Condition Evaluation
Auto-configuration classes are annotated with @Configuration
and are usually accompanied by one or more condition annotations. These conditions determine whether the configuration should be applied. Common condition annotations include:
@ConditionalOnClass
: Applies the configuration if a specific class is present in the classpath.@ConditionalOnMissingBean
: Applies the configuration if a bean of a specific type is not already in the application context.@ConditionalOnProperty
: Applies the configuration if a specific property has a certain value.
Here’s an example of how conditions are used in an auto-configuration class:
@Configuration
@ConditionalOnClass(DataSource.class)
public class DataSourceAutoConfiguration {
@Bean
@ConditionalOnMissingBean
public DataSource dataSource() {
// Create and configure a DataSource
}
}
In this example, the DataSourceAutoConfiguration
class will only be considered if the DataSource
class is present in the classpath. Furthermore, the dataSource()
bean will only be created if no other DataSource
bean has been defined.
Bean Creation
If all conditions are met, Spring Boot creates the beans defined in the auto-configuration class. These beans are then added to the application context, making them available for use throughout your application.
Property Binding
Many auto-configuration classes use properties to customize the created beans. These properties can be set in your application.properties
or application.yml
file. Spring Boot uses a relaxed binding approach, which means you can use different formats to specify the same property. For example:
spring.datasource.url=jdbc:mysql://localhost/mydb
spring.datasource.username=root
spring.datasource.password=secret
These properties would be used to configure a DataSource
bean created by the DataSourceAutoConfiguration
class.
Benefits of Auto-Configuration
Auto-configuration offers numerous advantages that contribute to increased productivity and simplified application development. Let’s explore some of the key benefits:
Reduced Boilerplate Code
One of the most significant advantages of auto-configuration is the dramatic reduction in boilerplate code. In traditional Spring applications, developers often had to write extensive configuration classes to set up various components. With auto-configuration, much of this setup is handled automatically, allowing developers to focus on writing business logic rather than infrastructure code.
Faster Development
By eliminating the need to manually configure every aspect of the application, auto-configuration significantly speeds up the development process. Developers can quickly prototype and iterate on their applications without getting bogged down in configuration details.
Consistency Across Projects
Auto-configuration promotes consistency across different Spring Boot projects. By providing sensible defaults and following best practices, it ensures that applications are configured in a standardized way. This consistency makes it easier for developers to switch between projects and for teams to maintain a cohesive codebase.
Easy Integration of Third-Party Libraries
Spring Boot’s auto-configuration makes it incredibly easy to integrate third-party libraries into your application. Many popular libraries provide their own auto-configuration modules, which means you can often add new functionality to your application just by including the appropriate dependency in your project.
Flexibility and Customization
While auto-configuration provides sensible defaults, it’s not a one-size-fits-all solution. Spring Boot offers various ways to customize and override the auto-configured beans, giving developers the flexibility to tailor the configuration to their specific needs.
Common Auto-Configuration Modules
Spring Boot comes with a wide range of auto-configuration modules that cover various aspects of application development. Let’s explore some of the most commonly used modules:
Web Applications
Spring Boot’s web auto-configuration sets up an embedded web server (like Tomcat) and configures essential web-related beans. It includes:
- Automatic configuration of
DispatcherServlet
- Setup of view resolvers
- Static resource handling
- Error page generation
Data Access
Spring Boot provides auto-configuration for various data access technologies:
- JPA and Hibernate: Automatically configures an
EntityManagerFactory
andJpaTransactionManager
- JDBC: Sets up a
DataSource
andJdbcTemplate
- Spring Data JPA: Configures repositories and related components
Security
The Spring Security auto-configuration sets up basic security features:
- Form-based authentication
- CSRF protection
- Security headers
Messaging
For applications that use messaging, Spring Boot offers auto-configuration for:
- JMS (Java Message Service)
- AMQP (Advanced Message Queuing Protocol)
- Apache Kafka
Caching
Spring Boot can automatically configure various caching providers:
- EhCache
- Hazelcast
- Infinispan
- Redis
Actuator
The Spring Boot Actuator provides production-ready features to help you monitor and manage your application. Its auto-configuration sets up various endpoints for health checks, metrics, and application information.
Customizing Auto-Configuration
While auto-configuration provides a great starting point, there are often scenarios where you need to customize or override the default behavior. Spring Boot offers several ways to achieve this:
Property Customization
The simplest way to customize auto-configuration is by setting properties in your application.properties
or application.yml
file. For example:
server.port=8081
spring.datasource.url=jdbc:mysql://localhost/mydb
logging.level.org.springframework=DEBUG
These properties will override the default values used by auto-configuration classes.
Excluding Auto-Configuration Classes
If you want to completely disable a specific auto-configuration class, you can use the @EnableAutoConfiguration
annotation’s exclude
attribute:
@SpringBootApplication
@EnableAutoConfiguration(exclude = {DataSourceAutoConfiguration.class})
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Defining Custom Beans
You can override auto-configured beans by defining your own beans of the same type. Spring Boot’s auto-configuration is designed to step back when it detects that you’ve provided your own configuration. For example:
@Configuration
public class MyConfiguration {
@Bean
public DataSource dataSource() {
// Custom DataSource configuration
}
}
Condition Annotations
You can use condition annotations in your own configuration classes to control when they should be applied. This allows you to create configuration that complements or replaces auto-configuration based on specific conditions. For example:
@Configuration
@ConditionalOnMissingBean(DataSource.class)
public class MyDataSourceConfiguration {
@Bean
public DataSource dataSource() {
// Custom DataSource configuration
}
}
Best Practices for Working with Auto-Configuration
To make the most of Spring Boot’s auto-configuration feature, consider the following best practices:
Understand Your Dependencies
Auto-configuration is heavily dependent on the libraries in your classpath. Be aware of what dependencies you’re including in your project and how they might trigger auto-configuration. Use the spring-boot-starter
dependencies to ensure you get the right set of libraries for your use case.
Review Auto-Configuration Reports
Spring Boot can generate a report of all the auto-configuration classes that were applied or skipped. You can enable this by setting the debug
property to true
in your application.properties
:
debug=true
This will log detailed auto-configuration information when you start your application, helping you understand what’s being configured automatically.
Use Configuration Properties
Instead of hardcoding values in your custom configuration classes, use @ConfigurationProperties
. This allows you to externalize configuration and makes it easier to override values:
@Configuration
@ConfigurationProperties(prefix = "myapp.datasource")
public class MyDataSourceProperties {
private String url;
private String username;
private String password;
// Getters and setters
}
@Configuration
public class MyDataSourceConfiguration {
@Autowired
private MyDataSourceProperties properties;
@Bean
public DataSource dataSource() {
// Use properties to configure DataSource
}
}
Test Your Configuration
Write tests to verify that your custom configuration is working as expected. Spring Boot provides testing utilities that make it easy to test auto-configuration and custom configuration:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyConfigurationTest {
@Autowired
private DataSource dataSource;
@Test
public void testDataSourceConfiguration() {
// Assert that dataSource is configured correctly
}
}
Keep Your Configuration Modular
When writing custom configuration, try to keep it modular and focused. Instead of having a single large configuration class, split it into smaller, purpose-specific classes. This makes your configuration more maintainable and easier to understand.
Advanced Auto-Configuration Techniques
For developers looking to take their auto-configuration skills to the next level, here are some advanced techniques:
Creating Custom Auto-Configuration
You can create your own auto-configuration classes for reusable components or libraries. To do this:
- Create a configuration class with the necessary
@Conditional
annotations. - Register your auto-configuration class in
META-INF/spring.factories
:
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.example.MyCustomAutoConfiguration
Using @AutoConfigureAfter and @AutoConfigureBefore
These annotations allow you to control the order in which auto-configuration classes are applied:
@Configuration
@AutoConfigureAfter(DataSourceAutoConfiguration.class)
public class MyDatabaseConfiguration {
// Configuration that depends on DataSource
}
Leveraging Spring Factories
Spring Boot uses the spring.factories
file to discover auto-configuration classes. You can use this mechanism for your own purposes, such as registering custom ApplicationListener
s or EnvironmentPostProcessor
s.
Conditional Auto-Configuration
Create more complex conditions for your auto-configuration by combining multiple @Conditional
annotations or by implementing the Condition
interface:
public class OnCustomCondition implements Condition {
@Override
public boolean matches(ConditionContext context, AnnotatedTypeMetadata metadata) {
// Custom condition logic
}
}
@Configuration
@Conditional(OnCustomCondition.class)
public class MyConditionalConfiguration {
// Configuration that applies only when OnCustomCondition is met
}
Conclusion
Auto-configuration is a powerful feature that sets Spring Boot apart from traditional Spring development. By automatically configuring your application based on the classpath and defined properties, it dramatically reduces the amount of boilerplate code and configuration required. This allows developers to focus on writing business logic rather than infrastructure setup.
Throughout this blog post, we’ve explored the inner workings of auto-configuration, its benefits, and how to work effectively with it. We’ve covered common auto-configuration modules, customization techniques, best practices, and even advanced topics for those looking to dive deeper.
As you continue your journey with Spring Boot, remember that auto-configuration is not magic – it’s a well-designed system that you can understand, customize, and extend. By mastering auto-configuration, you’ll be able to create more efficient, maintainable, and robust Spring applications.
Whether you’re building a simple microservice or a complex enterprise application, understanding and leveraging Spring Boot’s auto-configuration will be invaluable in your development process. Embrace the power of auto-configuration, but don’t be afraid to customize it when needed. With the knowledge gained from this guide, you’re well-equipped to make the most of this fantastic feature in your Spring Boot projects.
Disclaimer: While every effort has been made to ensure the accuracy and completeness of the information presented in this blog post, technology and software specifications can change rapidly. The information provided here is based on the current understanding of Spring Boot auto-configuration as of the date of publication. Readers are encouraged to consult the official Spring Boot documentation for the most up-to-date information. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.