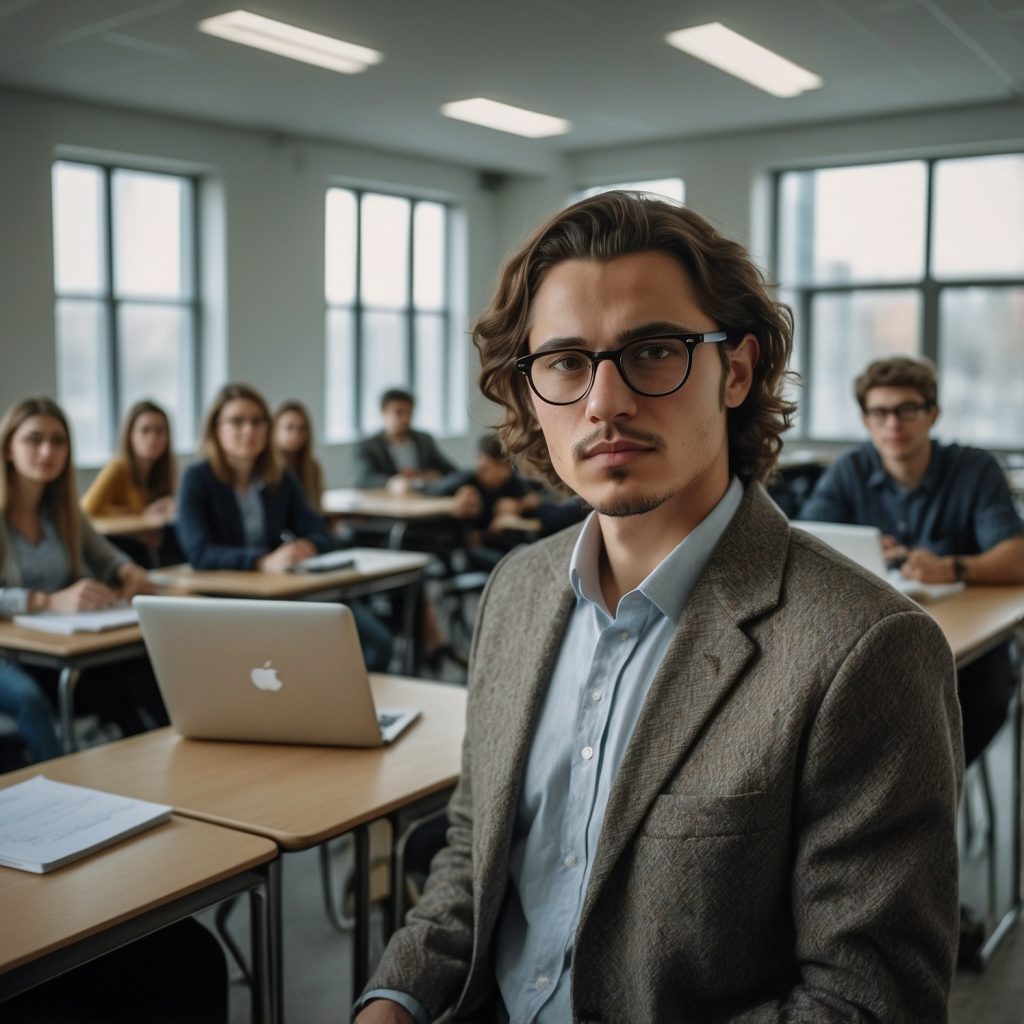
Understanding Functional Programming
Functional programming (FP) is a paradigm that has gained significant traction in recent years, especially with the rise of multi-core processors and the need for efficient, scalable code. While object-oriented programming (OOP) has dominated the software development landscape for decades, FP offers a different approach that can lead to more predictable and maintainable code. In this blog, we’ll delve into the fundamentals of functional programming, its principles, and its advantages, and provide plenty of code examples to illustrate its concepts.
What is Functional Programming?
Functional programming is a programming paradigm where computation is treated as the evaluation of mathematical functions and avoids changing state and mutable data. In essence, FP focuses on what to solve rather than how to solve it, which is often the focus in imperative programming.
Key Concepts of Functional Programming
- Pure Functions: Functions that always produce the same output for the same input and do not have any side effects.
- Immutability: Once a data structure is created, it cannot be changed.
- First-Class Functions: Functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
- Higher-Order Functions: Functions that can take other functions as arguments or return them as results.
- Recursion: The primary method of looping, as opposed to iterative constructs like
for
orwhile
loops in imperative languages. - Function Composition: Combining simple functions to build more complex ones.
Benefits of Functional Programming
Functional programming offers several advantages, particularly for modern software development challenges.
Predictability and Ease of Testing
Pure functions are predictable because they always produce the same output for the same input. This predictability makes functional programs easier to test and debug. For example, consider the following Python function:
def add(a, b):
return a + b
The add
function is pure. It always returns the sum of a
and b
without any side effects. Testing this function is straightforward because there are no external dependencies or state changes to consider.
Immutability and Concurrency
Immutability ensures that data cannot be changed once it is created. This property is particularly useful in concurrent programming, where multiple threads or processes might try to modify the same data simultaneously, leading to race conditions and other concurrency issues. In FP, since data is immutable, such issues are inherently avoided.
Consider this example in JavaScript:
const person = { name: 'Alice', age: 25 };
// Instead of modifying the original object, create a new one
const updatedPerson = { ...person, age: 26 };
console.log(person); // { name: 'Alice', age: 25 }
console.log(updatedPerson); // { name: 'Alice', age: 26 }
In this example, instead of modifying the person
object directly, a new object is created with the updated age, preserving the immutability principle.
Modularity and Reusability
Functional programs are often more modular because they consist of small, reusable functions. This modularity makes code easier to understand, maintain, and reuse. For example, in Haskell, you can compose functions to build complex operations from simple ones:
-- Define two simple functions
square x = x * x
increment x = x + 1
-- Compose them into a new function
squareAndIncrement = increment . square
-- Usage
main = print (squareAndIncrement 5) -- Output: 26
Key Features of Functional Programming Languages
Several programming languages support functional programming either exclusively or alongside other paradigms. Let’s explore some features of popular functional programming languages.
Haskell
Haskell is a purely functional programming language that enforces immutability and pure functions.
-- Example of a pure function in Haskell
double x = x * 2
-- Higher-order function: map
doubleList = map double [1, 2, 3, 4]
main = print doubleList -- Output: [2,4,6,8]
In this example, the double
function is pure, and map
is a higher-order function that applies double
to each element of the list.
Scala
Scala is a language that blends functional and object-oriented programming. It runs on the Java Virtual Machine (JVM) and can interoperate with Java code.
object FunctionalProgramming extends App {
// Example of a pure function
def add(a: Int, b: Int): Int = a + b
// Higher-order function
def applyFunction(f: (Int, Int) => Int, a: Int, b: Int): Int = f(a, b)
val result = applyFunction(add, 5, 3)
println(result) // Output: 8
}
Here, add
is a pure function, and applyFunction
demonstrates the use of higher-order functions by accepting another function as an argument.
JavaScript
JavaScript, primarily known for its imperative and object-oriented features, also supports functional programming.
// Pure function
const multiply = (a, b) => a * b;
// Higher-order function: filter
const isEven = (n) => n % 2 === 0;
const evenNumbers = [1, 2, 3, 4, 5, 6].filter(isEven);
console.log(evenNumbers); // Output: [2, 4, 6]
In this example, multiply
is a pure function, and filter
is a higher-order function that takes another function isEven
as its argument.
Common Functional Programming Techniques
Currying
Currying is the process of transforming a function that takes multiple arguments into a sequence of functions that each take a single argument.
// Curried function in JavaScript
const add = (a) => (b) => a + b;
const addFive = add(5);
console.log(addFive(3)); // Output: 8
Partial Application
Partial application refers to the process of fixing a few arguments of a function and producing another function of smaller arity.
def multiply(a: Int, b: Int, c: Int): Int = a * b * c
// Partially applied function
val multiplyBy2And3 = multiply(2, 3, _: Int)
println(multiplyBy2And3(4)) // Output: 24
Lazy Evaluation
Lazy evaluation is a strategy where expressions are not evaluated until their values are needed. This can lead to performance improvements by avoiding unnecessary computations.
-- Haskell example of lazy evaluation
ones = 1 : ones
take 5 ones -- Output: [1,1,1,1,1]
Real-World Applications of Functional Programming
Functional programming is not just a theoretical concept but has practical applications in various domains.
Web Development
Languages like Elm and frameworks like React (which uses a functional approach) have popularized FP in web development.
// React functional component
const Greeting = ({ name }) => <h1>Hello, {name}!</h1>;
ReactDOM.render(<Greeting name="Alice" />, document.getElementById('root'));
Data Science
Languages like R and libraries in Python (like pandas and NumPy) leverage functional programming for data manipulation and analysis.
import pandas as pd
# Sample data
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
# Create DataFrame
df = pd.DataFrame(data)
# Use a lambda function with map
df['AgeInFiveYears'] = df['Age'].map(lambda x: x + 5)
print(df)
# Output:
# Name Age AgeInFiveYears
# 0 Alice 25 30
# 1 Bob 30 35
# 2 Charlie 35 40
Functional programming offers a powerful alternative to traditional imperative programming, with its focus on pure functions, immutability, and higher-order functions. These principles can lead to more predictable, maintainable, and scalable code, making it particularly well-suited for modern software development challenges.
By understanding and applying the concepts of functional programming, you can improve the quality of your code and take advantage of the benefits it offers. Whether you’re working on web development, data science, or any other field, the principles of FP can help you write cleaner, more efficient, and more reliable software.
Embrace functional programming and explore its potential in your projects. Happy coding!