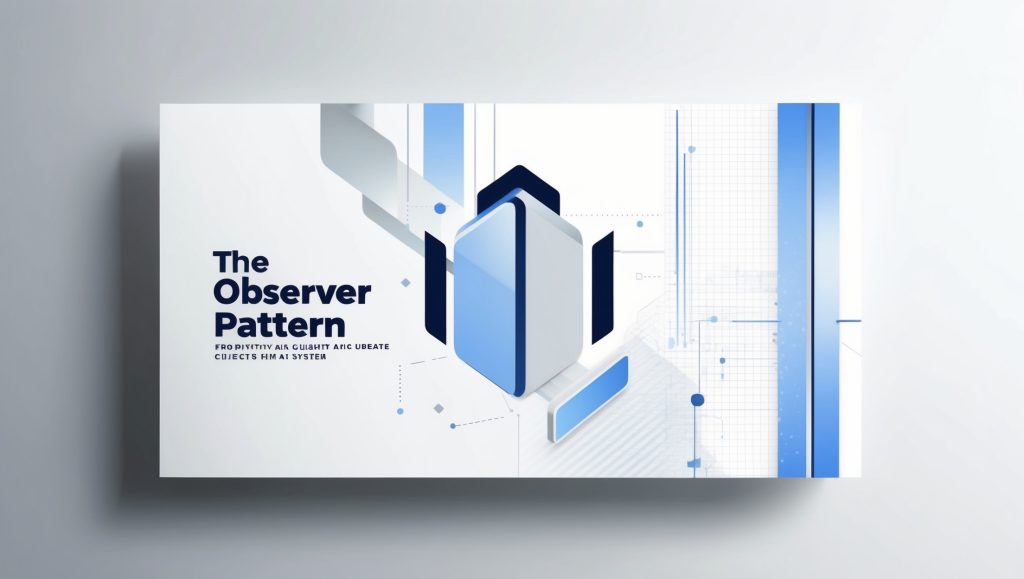
Understanding MVC Architecture and the Observer Pattern
The Model-View-Controller (MVC) architectural pattern and the Observer pattern are fundamental concepts in software engineering that have stood the test of time. These patterns continue to influence modern application development, from web applications to desktop software and mobile apps. In this comprehensive guide, we’ll explore how these patterns work independently and how they complement each other to create robust, maintainable applications. We’ll delve into their implementation details, best practices, and real-world applications, supported by concrete examples in both Python and Java. Understanding these patterns is crucial for developers who want to create scalable, maintainable, and well-structured applications that can adapt to changing requirements while maintaining code quality and reducing technical debt.
The Model-View-Controller (MVC) Pattern
The MVC pattern is an architectural design pattern that separates an application into three main components: Model, View, and Controller. This separation of concerns allows for better code organization, maintainability, and scalability. Each component has its specific responsibilities and interacts with other components in well-defined ways.
Components of MVC
-
Model: The Model represents the application’s data and business logic. It is responsible for:
- Managing data
- Processing business rules
- Handling data validation
- Maintaining application state
-
View: The View is responsible for presenting data to users. It:
- Renders the user interface
- Displays data from the Model
- Captures user input
- Sends user actions to the Controller
-
Controller: The Controller acts as an intermediary between Model and View. It:
- Processes user input
- Updates the Model
- Selects appropriate Views
- Coordinates application flow
Let’s look at a simple implementation of MVC in Python:
# Model
class UserModel:
def __init__(self):
self.name = ""
self.email = ""
self._observers = []
def set_user_details(self, name, email):
self.name = name
self.email = email
self.notify_observers()
def add_observer(self, observer):
self._observers.append(observer)
def notify_observers(self):
for observer in self._observers:
observer.update(self)
# View
class UserView:
def display_user_details(self, user):
print(f"\nUser Details:")
print(f"Name: {user.name}")
print(f"Email: {user.email}")
def get_user_input(self):
name = input("Enter name: ")
email = input("Enter email: ")
return name, email
# Controller
class UserController:
def __init__(self, model, view):
self.model = model
self.view = view
def update_user(self):
name, email = self.view.get_user_input()
self.model.set_user_details(name, email)
def display_user(self):
self.view.display_user_details(self.model)
# Usage
if __name__ == "__main__":
model = UserModel()
view = UserView()
controller = UserController(model, view)
controller.update_user()
controller.display_user()
The Observer Pattern
The Observer pattern is a behavioral design pattern that establishes a one-to-many relationship between objects. When one object (the subject) changes its state, all its dependents (observers) are notified and updated automatically. This pattern is particularly useful in implementing distributed event handling systems.
Key Components of the Observer Pattern
-
Subject (Observable):
- Maintains a list of observers
- Provides methods to add/remove observers
- Notifies observers of state changes
-
Observer:
- Defines an updating interface
- Receives updates from the subject
- Maintains consistency with the subject
Here’s an implementation of the Observer pattern in Java:
import java.util.ArrayList;
import java.util.List;
// Subject interface
interface Subject {
void registerObserver(Observer observer);
void removeObserver(Observer observer);
void notifyObservers();
}
// Observer interface
interface Observer {
void update(String message);
}
// Concrete Subject
class NewsAgency implements Subject {
private List<Observer> observers = new ArrayList<>();
private String news;
@Override
public void registerObserver(Observer observer) {
observers.add(observer);
}
@Override
public void removeObserver(Observer observer) {
observers.remove(observer);
}
@Override
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(news);
}
}
public void setNews(String news) {
this.news = news;
notifyObservers();
}
}
// Concrete Observer
class NewsChannel implements Observer {
private String name;
public NewsChannel(String name) {
this.name = name;
}
@Override
public void update(String news) {
System.out.println(name + " received news: " + news);
}
}
// Usage Example
public class ObserverDemo {
public static void main(String[] args) {
NewsAgency newsAgency = new NewsAgency();
NewsChannel channel1 = new NewsChannel("Channel 1");
NewsChannel channel2 = new NewsChannel("Channel 2");
newsAgency.registerObserver(channel1);
newsAgency.registerObserver(channel2);
newsAgency.setNews("Breaking News: Important Update!");
}
}
Integrating MVC with the Observer Pattern
The integration of MVC with the Observer pattern creates a powerful combination that enhances application flexibility and maintainability. The Model typically acts as the Subject, while Views act as Observers. This integration enables automatic UI updates when the underlying data changes.
Benefits of Integration
Benefit | Description |
---|---|
Loose Coupling | Views are not directly dependent on Models |
Multiple Views | Same data can be displayed in different formats |
Consistency | All Views automatically reflect Model changes |
Scalability | Easy to add new Views without modifying existing code |
Here’s an example of integrated MVC and Observer pattern in Python:
from abc import ABC, abstractmethod
from typing import List
# Observer interface
class Observer(ABC):
@abstractmethod
def update(self, subject):
pass
# Subject interface
class Subject(ABC):
@abstractmethod
def attach(self, observer: Observer):
pass
@abstractmethod
def detach(self, observer: Observer):
pass
@abstractmethod
def notify(self):
pass
# Model (Subject)
class DataModel(Subject):
def __init__(self):
self._observers: List[Observer] = []
self._data = None
def attach(self, observer: Observer):
if observer not in self._observers:
self._observers.append(observer)
def detach(self, observer: Observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self)
@property
def data(self):
return self._data
@data.setter
def data(self, value):
self._data = value
self.notify()
# Views (Observers)
class TableView(Observer):
def update(self, subject: DataModel):
print(f"\nTable View - Data Updated:")
print("-" * 20)
print(f"| {subject.data} |")
print("-" * 20)
class ChartView(Observer):
def update(self, subject: DataModel):
print(f"\nChart View - Data Updated:")
print(f"{'*' * int(subject.data)}")
print(f"Value: {subject.data}")
# Controller
class DataController:
def __init__(self, model: DataModel):
self.model = model
def set_data(self, value):
self.model.data = value
# Usage
if __name__ == "__main__":
# Create MVC components
model = DataModel()
controller = DataController(model)
# Create views
table_view = TableView()
chart_view = ChartView()
# Register views as observers
model.attach(table_view)
model.attach(chart_view)
# Update data through controller
controller.set_data(5)
controller.set_data(10)
Best Practices and Design Considerations
When implementing MVC with the Observer pattern, several best practices should be followed to ensure optimal results:
Architecture Guidelines
-
Keep the Model Independent:
- Models should not have direct references to Views or Controllers
- Models should not depend on UI-specific code
- Business logic should be contained within the Model
-
Minimize View Logic:
- Views should focus on display logic only
- Complex data transformations should be handled by the Model
- Views should not modify Model data directly
-
Controller Responsibilities:
- Controllers should be thin
- Avoid business logic in Controllers
-
Focus on coordination and user input handling
Implementation Considerations
Consideration | Description | Solution |
---|---|---|
Memory Leaks | Observer references not properly cleaned | Implement proper observer removal |
Performance | Too many updates | Use batch updates or throttling |
Complexity | Cascade of updates | Implement update priority system |
Threading | Concurrent modifications | Use thread-safe observer lists |
The basic MVC and Observer patterns can be extended and modified to meet specific requirements:
MVVM (Model-View-ViewModel)
class ViewModel:
def __init__(self, model):
self.model = model
self._observers = []
self.processed_data = None
def process_data(self):
# Transform model data for view consumption
raw_data = self.model.get_data()
self.processed_data = self.transform_data(raw_data)
self.notify_observers()
def transform_data(self, data):
# Apply view-specific transformations
return f"Processed: {data}"
def add_observer(self, observer):
self._observers.append(observer)
def notify_observers(self):
for observer in self._observers:
observer.update(self.processed_data)
MVP (Model-View-Presenter)
// Presenter interface
interface UserPresenter {
void onUserDataChanged(String name, String email);
void onDisplayRequest();
}
// Concrete Presenter
class UserPresenterImpl implements UserPresenter {
private UserModel model;
private UserView view;
public UserPresenterImpl(UserModel model, UserView view) {
this.model = model;
this.view = view;
}
@Override
public void onUserDataChanged(String name, String email) {
model.setUserDetails(name, email);
view.displayUserDetails(model.getName(), model.getEmail());
}
@Override
public void onDisplayRequest() {
view.displayUserDetails(model.getName(), model.getEmail());
}
}
Testing Strategies
Testing applications built with MVC and Observer patterns requires careful consideration of component isolation and interaction:
Unit Testing
import unittest
from unittest.mock import Mock
class TestUserModel(unittest.TestCase):
def setUp(self):
self.model = UserModel()
self.observer = Mock()
self.model.add_observer(self.observer)
def test_set_user_details_notifies_observers(self):
self.model.set_user_details("John", "john@example.com")
self.observer.update.assert_called_once_with(self.model)
def test_user_details_stored_correctly(self):
self.model.set_user_details("John", "john@example.com")
self.assertEqual(self.model.name, "John")
self.assertEqual(self.model.email, "john@example.com")
Conclusion
The combination of MVC architecture and the Observer pattern provides a robust foundation for building maintainable and scalable applications. By following the principles and best practices outlined in this guide, developers can create applications that are easier to test, modify, and extend. The examples provided in both Python and Java demonstrate practical implementations that can be adapted to various use cases and requirements. Understanding these patterns and their interactions is crucial for modern software development, enabling developers to create more organized and efficient code structures.
Disclaimer: The code examples and implementations provided in this blog post are for educational purposes and may need to be adapted for production use. While we strive for accuracy, technology evolves rapidly, and best practices may change. Please report any inaccuracies or suggestions for improvement to our editorial team.