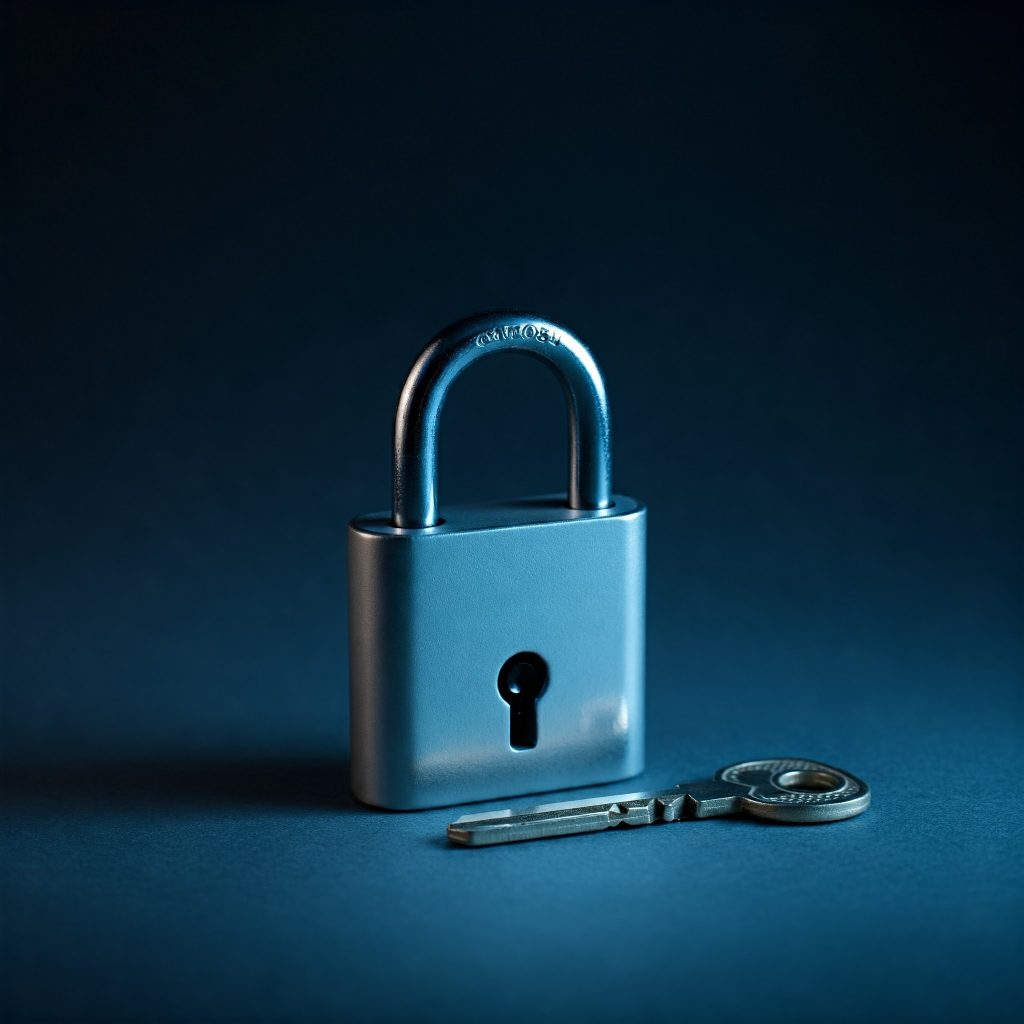
Understanding Primary and Foreign Keys in SQL – Database Relationships and Constraints
In the world of relational databases, primary keys and foreign keys are fundamental elements for managing data integrity and ensuring reliable relationships between tables. Whether you are building a database from scratch or optimizing an existing one, understanding how these keys function is essential for maintaining a structured and logical data model. This blog will delve into the concepts of primary and foreign keys, how they contribute to defining relationships between database tables, and the constraints they impose on data integrity.
By the end of this article, you will have a comprehensive understanding of these key concepts and their critical roles in relational database design.
What is a Primary Key?
A primary key is a column or a combination of columns in a database table that uniquely identifies each record in that table. The primary key ensures that each record in the table is unique and can be referenced efficiently.
Characteristics of a Primary Key:
- Uniqueness: Every value in the primary key column must be unique across the table. This guarantees that no two rows have the same primary key value.
- Non-nullable: A primary key cannot contain NULL values. Every row must have a value for the primary key, as NULL would indicate an unknown or undefined record.
- Single Column or Composite Key: A primary key can be a single column (simple primary key) or a combination of multiple columns (composite primary key).
- Automatic Indexing: Most relational databases automatically create an index for the primary key column, enabling faster query performance.
SQL Example: Defining a Primary Key
Let’s start with a simple example where we create a table with a primary key.
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
Department VARCHAR(50)
);
In this example, the EmployeeID
column is defined as the primary key. Every row in the Employees
table must have a unique, non-null EmployeeID
.
Composite Primary Key Example
A composite primary key involves two or more columns that together create a unique identifier for a record. Here’s an example:
CREATE TABLE ProjectAssignments (
EmployeeID INT,
ProjectID INT,
AssignmentDate DATE,
PRIMARY KEY (EmployeeID, ProjectID)
);
Here, the combination of EmployeeID
and ProjectID
forms the primary key. This ensures that each employee is uniquely assigned to a project without any duplicate assignments.
What is a Foreign Key?
A foreign key is a column or set of columns in one table that establishes a link between the data in two tables. It acts as a reference to the primary key in another table, enforcing referential integrity by ensuring that the value in the foreign key column matches a valid value in the primary key column of the related table.
Characteristics of a Foreign Key:
- Referential Integrity: A foreign key enforces a relationship between tables, ensuring that the data in the foreign key column matches an existing value in the related primary key column.
- Allows Null Values: Unlike primary keys, foreign keys can contain NULL values, meaning that a record does not need to be related to a parent record.
- Can Span Multiple Columns: Like primary keys, foreign keys can also be composed of multiple columns.
SQL Example: Defining a Foreign Key
Below is an example of creating a foreign key in SQL. Consider we have two tables: Departments
and Employees
. We want to create a foreign key in the Employees
table that references the Departments
table.
CREATE TABLE Departments (
DepartmentID INT PRIMARY KEY,
DepartmentName VARCHAR(100)
);
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
DepartmentID INT,
FOREIGN KEY (DepartmentID) REFERENCES Departments(DepartmentID)
);
In this example, the DepartmentID
column in the Employees
table is a foreign key that references the DepartmentID
column in the Departments
table. This relationship ensures that any value entered into the DepartmentID
column in Employees
must correspond to an existing value in the Departments
table.
Understanding Database Relationships with Keys
Keys, particularly primary and foreign keys, are crucial in defining the relationships between different tables in a relational database. These relationships can be classified into the following types:
One-to-One Relationship
In a one-to-one relationship, each record in Table A corresponds to exactly one record in Table B. This relationship is often used when splitting data across tables to reduce redundancy or to separate sensitive information.
For example, consider a scenario where sensitive data like employee salaries are stored separately:
CREATE TABLE EmployeeDetails (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50)
);
CREATE TABLE EmployeeSalaries (
EmployeeID INT PRIMARY KEY,
Salary DECIMAL(10, 2),
FOREIGN KEY (EmployeeID) REFERENCES EmployeeDetails(EmployeeID)
);
Here, both tables share a one-to-one relationship, where each EmployeeID
in EmployeeDetails
has a corresponding EmployeeID
in EmployeeSalaries
.
One-to-Many Relationship
A one-to-many relationship is the most common type in relational databases. In this relationship, a record in Table A can relate to multiple records in Table B, but each record in Table B relates to only one record in Table A.
For example, one department can have multiple employees, but each employee belongs to only one department:
CREATE TABLE Departments (
DepartmentID INT PRIMARY KEY,
DepartmentName VARCHAR(100)
);
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
DepartmentID INT,
FOREIGN KEY (DepartmentID) REFERENCES Departments(DepartmentID)
);
In this case, the relationship between Departments
and Employees
is one-to-many, where one department has many employees, but each employee is associated with only one department.
Many-to-Many Relationship
A many-to-many relationship occurs when a record in Table A can relate to multiple records in Table B, and vice versa. This relationship typically requires the creation of an intermediary table to break down the many-to-many relationship into two one-to-many relationships.
For example, consider a scenario where employees can be assigned to multiple projects, and each project can have multiple employees:
CREATE TABLE Projects (
ProjectID INT PRIMARY KEY,
ProjectName VARCHAR(100)
);
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50)
);
CREATE TABLE EmployeeProjects (
EmployeeID INT,
ProjectID INT,
AssignmentDate DATE,
PRIMARY KEY (EmployeeID, ProjectID),
FOREIGN KEY (EmployeeID) REFERENCES Employees(EmployeeID),
FOREIGN KEY (ProjectID) REFERENCES Projects(ProjectID)
);
In this case, the EmployeeProjects
table acts as a junction table, creating two one-to-many relationships: one between Employees
and EmployeeProjects
, and another between Projects
and EmployeeProjects
.
Primary and Foreign Key Constraints
Primary and foreign keys come with constraints that are critical for maintaining data integrity and preventing errors in the database.
Primary Key Constraints
- Unique Constraint: Ensures that all values in the primary key column are unique. For example:
ALTER TABLE Employees ADD CONSTRAINT PK_EmployeeID UNIQUE (EmployeeID);
- NOT NULL Constraint: Prevents NULL values in the primary key column:
ALTER TABLE Employees MODIFY EmployeeID INT NOT NULL;
Foreign Key Constraints
- Referential Integrity Constraint: Enforces that a foreign key value matches a value in the related table’s primary key:
ALTER TABLE Employees ADD CONSTRAINT FK_DepartmentID FOREIGN KEY (DepartmentID) REFERENCES Departments(DepartmentID);
- ON DELETE CASCADE: Automatically deletes child records when a parent record is deleted:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) ON DELETE CASCADE );
In this example, if a customer is deleted from theCustomers
table, all their orders in theOrders
table will also be deleted. - ON UPDATE CASCADE: Automatically updates the foreign key in child records if the primary key in the parent record changes:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) ON UPDATE CASCADE );
Comparison of Primary Key and Foreign Key
The table below highlights the key differences between primary keys and foreign keys:
Aspect | Primary Key | Foreign Key |
---|---|---|
Purpose | Uniquely identifies each record in a table | Creates a relationship between two tables |
Uniqueness | Values must be unique | Values can be duplicated (except when used in composite key) |
Nullability | Cannot contain NULL values | Can contain NULL values |
Number of Keys | One primary key per table | Can have multiple foreign keys in a table |
Indexing | Automatically indexed | Not automatically indexed |
Referential Integrity | Does not reference another table | References the primary key in another table |
Best Practices for Using Primary and Foreign Keys
Adhering to
best practices ensures the efficient use of primary and foreign keys, enhancing data integrity and database performance.
Choosing Primary Keys
- Use Simple Keys: Whenever possible, opt for simple primary keys (single columns). Composite keys are more complex to manage and may impact query performance.
- Avoid Natural Keys: While natural keys (like social security numbers) can serve as primary keys, it is often better to use surrogate keys (like auto-incremented IDs) to avoid complications if the natural key changes.
Designing Foreign Keys
- Define Referential Constraints: Always specify referential actions (
ON DELETE CASCADE
,ON UPDATE CASCADE
) to maintain data consistency across related tables. - Index Foreign Keys: Although foreign keys are not indexed by default, it’s beneficial to create indexes on foreign keys to improve join performance in queries.
Example: Creating a Full Database with Primary and Foreign Keys
Let’s put everything together by creating a simple database schema with primary and foreign key constraints.
CREATE DATABASE CompanyDB;
USE CompanyDB;
-- Create Departments Table
CREATE TABLE Departments (
DepartmentID INT PRIMARY KEY,
DepartmentName VARCHAR(100) NOT NULL
);
-- Create Employees Table
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50) NOT NULL,
LastName VARCHAR(50) NOT NULL,
DepartmentID INT,
FOREIGN KEY (DepartmentID) REFERENCES Departments(DepartmentID) ON DELETE CASCADE
);
-- Create Projects Table
CREATE TABLE Projects (
ProjectID INT PRIMARY KEY,
ProjectName VARCHAR(100) NOT NULL
);
-- Create EmployeeProjects Junction Table
CREATE TABLE EmployeeProjects (
EmployeeID INT,
ProjectID INT,
AssignmentDate DATE,
PRIMARY KEY (EmployeeID, ProjectID),
FOREIGN KEY (EmployeeID) REFERENCES Employees(EmployeeID) ON DELETE CASCADE,
FOREIGN KEY (ProjectID) REFERENCES Projects(ProjectID) ON DELETE CASCADE
);
In this example, we created four tables: Departments
, Employees
, Projects
, and EmployeeProjects
, using both primary and foreign key constraints to enforce referential integrity.
Conclusion
Understanding primary and foreign keys is critical for creating structured, efficient, and reliable relational databases. Primary keys uniquely identify records, while foreign keys link tables and enforce relationships. Together, they provide the foundation for enforcing data integrity and enabling the structured relationships that make relational databases so powerful. By following best practices and understanding the roles of primary and foreign keys, you can ensure your database design remains robust and scalable.
Disclaimer: The examples provided are for educational purposes. Please report any inaccuracies so we can correct them promptly.