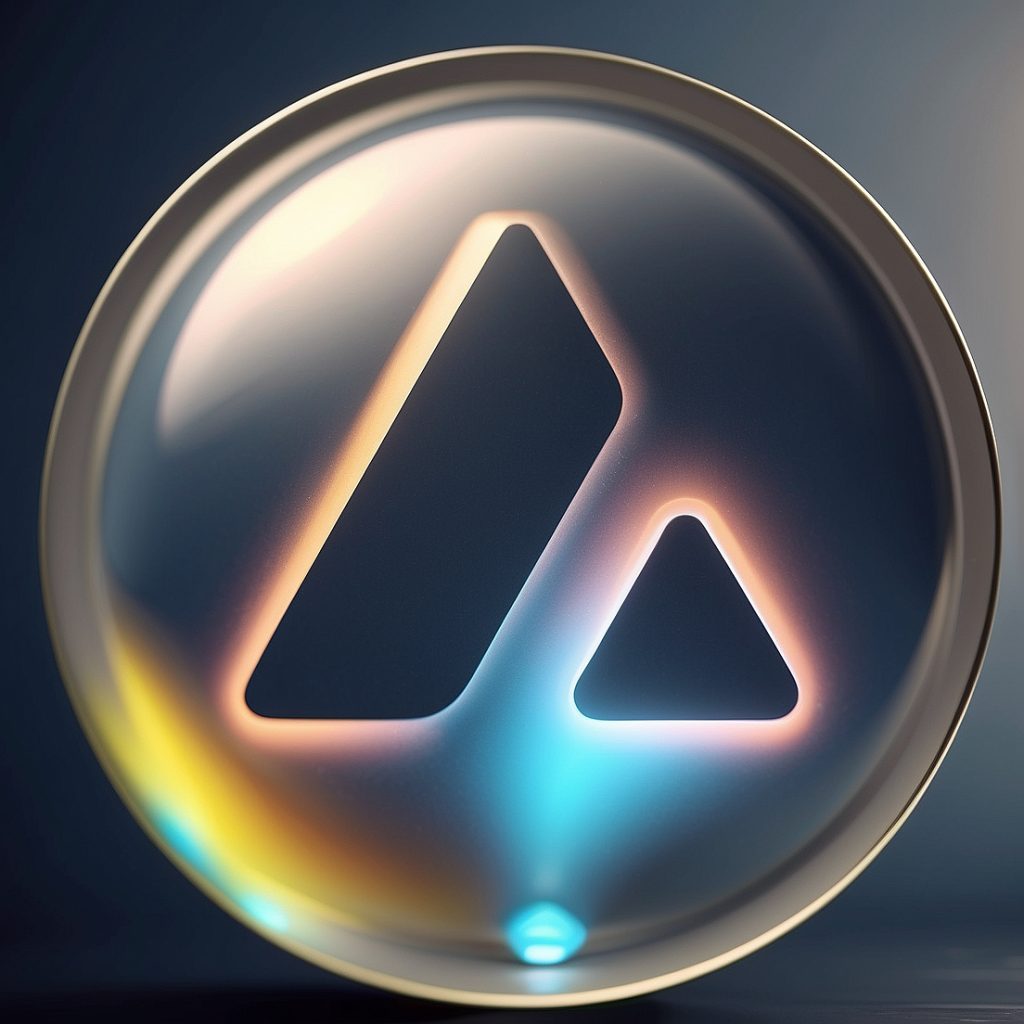
Understanding the Avalanche Consensus Protocol: A Revolution in Blockchain Technology
The world of blockchain technology is continuously evolving, and among the most notable advancements is the Avalanche consensus protocol. This protocol stands out due to its high throughput, low latency, and robust safety guarantees, making it a game-changer for decentralized applications and enterprise blockchain solutions. In this comprehensive blog post, we will delve deep into how the Avalanche consensus protocol works, its key concepts, and its implications for the future of blockchain technology. We will also provide practical code snippets and scripts to illustrate the protocol’s functionality.
The Avalanche Consensus Protocol: An Overview
The Avalanche consensus protocol represents a significant shift from traditional blockchain consensus mechanisms. Unlike conventional Proof-of-Work (PoW) or Proof-of-Stake (PoS) protocols, Avalanche uses a Directed Acyclic Graph (DAG) structure combined with a family of consensus protocols known as Snowball. This unique approach enables Avalanche to achieve high throughput, low latency, and strong safety guarantees.
Key Concepts of Avalanche Consensus
Snowball Family Protocols
The Avalanche consensus is built on a family of protocols called Snowball, which includes Snowflake, Snowball, and Avalanche. These protocols leverage random sampling and repeated sub-sampling to achieve consensus efficiently.
Directed Acyclic Graph (DAG)
Unlike traditional blockchain structures where blocks are added linearly, Avalanche employs a DAG. In a DAG, each transaction or block is a vertex, and edges represent dependencies between them. This structure allows for parallel processing of transactions, significantly enhancing throughput.
Probabilistic Agreement
Consensus in Avalanche is reached through probabilistic agreement rather than absolute certainty. This method allows for faster decision-making without compromising security.
The Consensus Process: Step-by-Step
Proposal Phase
When a new transaction is created, it is broadcast to the network and added to the DAG. Each node in the network receives the transaction and considers it as a candidate for acceptance.
Code Snippet: Broadcasting a Transaction
def broadcast_transaction(transaction, network):
for node in network.nodes:
node.receive_transaction(transaction)
transaction = create_transaction(sender, receiver, amount)
broadcast_transaction(transaction, avalanche_network)
Random Sampling
Nodes randomly sample a small subset of other nodes and query them about their current view of the network, specifically whether they believe the new transaction is valid or not. This sampling process is repeated multiple times.
Code Snippet: Random Sampling
import random
def random_sampling(node, network, sample_size):
sample_nodes = random.sample(network.nodes, sample_size)
responses = []
for sample_node in sample_nodes:
responses.append(sample_node.query_transaction_status(node.current_transaction))
return responses
sample_size = 5
responses = random_sampling(current_node, avalanche_network, sample_size)
Repeated Sub-sampling
Each node keeps track of the responses from its queries. The responses are used to update the node’s confidence in the transaction. This process of querying and updating continues until a threshold is reached where the node is confident enough to make a decision.
Code Snippet: Updating Confidence
def update_confidence(responses):
positive_responses = responses.count(True)
total_responses = len(responses)
confidence = positive_responses / total_responses
return confidence
confidence_threshold = 0.8
current_confidence = update_confidence(responses)
while current_confidence < confidence_threshold:
responses = random_sampling(current_node, avalanche_network, sample_size)
current_confidence = update_confidence(responses)
Decision Phase
- Snowflake: The initial phase involves a single query, and nodes decide based on the majority response.
- Snowball: An extension of Snowflake, where nodes keep track of the number of successful queries to form a confidence level.
- Avalanche: The final phase combines a series of Snowball consensus instances to finalize the decision. Once a transaction has enough confirmations from multiple Snowball instances, it is considered final and irreversible.
Code Snippet: Finalizing the Decision
def finalize_decision(node):
snowflake_decision = make_snowflake_decision(node)
snowball_decision = make_snowball_decision(node, snowflake_decision)
avalanche_decision = make_avalanche_decision(node, snowball_decision)
return avalanche_decision
final_decision = finalize_decision(current_node)
Properties of Avalanche Consensus
High Throughput
The use of DAG allows multiple transactions to be processed in parallel, significantly increasing the network’s throughput. This parallel processing capability is a key advantage of the Avalanche protocol.
Low Latency
Transactions are confirmed within seconds due to the rapid consensus mechanism, providing near-instant finality. This low latency makes Avalanche suitable for a wide range of real-time applications.
Scalability
The protocol is designed to scale efficiently with the number of nodes, making it suitable for large, decentralized networks. As more nodes join the network, the consensus mechanism remains robust and efficient.
Robustness
The probabilistic nature of consensus, combined with multiple sampling rounds, provides strong safety guarantees even in the presence of malicious actors. This robustness is crucial for maintaining the integrity of the network.
Energy Efficiency
Unlike Proof-of-Work (PoW) systems, Avalanche’s consensus does not rely on intensive computational work, making it more environmentally friendly. This energy efficiency is increasingly important in the context of global sustainability efforts.
Example Scenario: Alice Sends 10 AVAX to Bob
Transaction Creation
Alice creates a transaction to send 10 AVAX to Bob. This transaction is broadcast to the network.
Code Snippet: Creating a Transaction
def create_transaction(sender, receiver, amount):
transaction = {
'sender': sender,
'receiver': receiver,
'amount': amount,
'timestamp': time.time()
}
return transaction
alice_to_bob_tx = create_transaction('Alice', 'Bob', 10)
broadcast_transaction(alice_to_bob_tx, avalanche_network)
Network Propagation
Nodes receive Alice’s transaction and add it to their local DAGs.
Code Snippet: Adding Transaction to DAG
def add_to_dag(transaction, dag):
dag.append(transaction)
for node in avalanche_network.nodes:
add_to_dag(alice_to_bob_tx, node.dag)
Sampling and Querying
Each node randomly samples a subset of other nodes, asking whether they believe Alice’s transaction is valid.
Code Snippet: Querying Transaction Validity
def query_transaction_status(transaction, node):
return transaction in node.dag
responses = random_sampling(current_node, avalanche_network, sample_size)
Consensus Formation
Based on the responses, nodes update their confidence in Alice’s transaction. After sufficient rounds of sampling and confirmation, nodes agree on the validity of the transaction.
Code Snippet: Forming Consensus
current_confidence = update_confidence(responses)
while current_confidence < confidence_threshold:
responses = random_sampling(current_node, avalanche_network, sample_size)
current_confidence = update_confidence(responses)
Finalization
The transaction is confirmed and becomes part of the permanent ledger.
Code Snippet: Confirming Transaction
def confirm_transaction(transaction, node):
node.confirmed_transactions.append(transaction)
if final_decision:
for node in avalanche_network.nodes:
confirm_transaction(alice_to_bob_tx, node)
Conclusion
The Avalanche consensus protocol represents a groundbreaking approach to achieving consensus in a decentralized network. By leveraging a Directed Acyclic Graph (DAG) structure and a family of Snowball protocols, Avalanche achieves high throughput, low latency, and strong safety guarantees. These properties make it an ideal choice for building high-performance decentralized applications and platforms.
As blockchain technology continues to evolve, the Avalanche consensus protocol stands as a testament to the power of innovation in creating more efficient, scalable, and robust systems. Whether you are a developer, an entrepreneur, or simply a blockchain enthusiast, understanding the intricacies of Avalanche can provide valuable insights into the future of decentralized technology.
Sample Code and Scripts
To help you get started with Avalanche, here are some additional sample codes and scripts:
Node Initialization
class Node:
def __init__(self, id):
self.id = id
self.dag = []
self.confirmed_transactions = []
def receive_transaction(self, transaction):
self.dag.append(transaction)
def query_transaction_status(self, transaction):
return transaction in self.dag
def confirm_transaction(self, transaction):
self.confirmed_transactions.append(transaction)
# Initialize nodes
nodes = [Node(i) for i in range(10)]
avalanche_network = Network(nodes)
Network Simulation
class Network:
def __init__(self, nodes):
self.nodes = nodes
def broadcast_transaction(self, transaction):
for node in self.nodes:
node.receive_transaction(transaction)
def random_sampling(self, node, sample_size):
sample_nodes = random.sample(self.nodes, sample_size)
responses = []
for sample_node in sample_nodes:
responses.append(sample_node.query_transaction_status(node.current_transaction))
return responses
def update_confidence(self, responses):
positive_responses = responses.count(True)
total_responses = len(responses)
confidence = positive_responses / total_responses
return confidence
# Simulate a network broadcast
transaction = create_transaction('Alice', 'Bob', 10)
avalanche_network.broadcast_transaction(transaction)
# Simulate random sampling and consensus
sample_size = 5
responses = avalanche_network.random_sampling(nodes[0], sample_size)
confidence_threshold = 0.8
current_confidence = avalanche_network.update_confidence(responses)
while current_confidence < confidence_threshold:
responses = avalanche_network.random_sampling(nodes[0], sample_size)
current_confidence = avalanche_network.update_confidence(responses)
By understanding and implementing these principles, you can harness the power of the Avalanche consensus protocol to build the next generation of decentralized applications. The future of blockchain technology is bright, and with innovative protocols like Avalanche, we are well on our way to realizing its full potential.