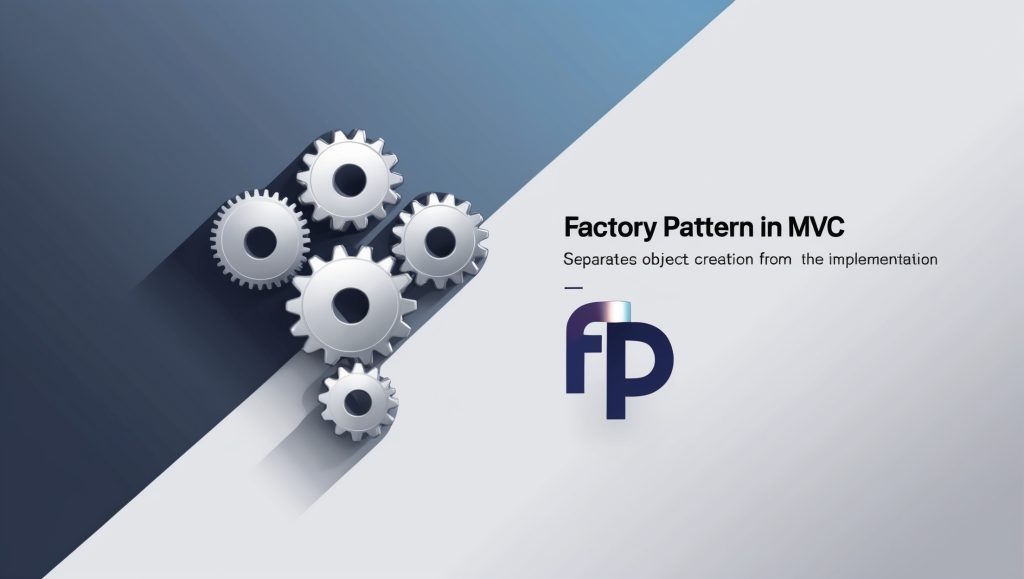
Understanding the Factory Pattern in MVC
The Factory Pattern belongs to the creational pattern family and provides an interface for creating objects without explicitly specifying their exact classes. This pattern becomes particularly valuable in complex systems where object creation logic needs to be centralized and managed efficiently. The pattern introduces a layer of abstraction between the client code and the actual object creation process, allowing for greater flexibility in how objects are instantiated and configured. At its core, the Factory Pattern helps manage the complexity of object creation while maintaining loose coupling between components.
Types of Factory Patterns in MVC
Simple Factory Pattern
The Simple Factory Pattern, while not technically a design pattern but rather a programming idiom, serves as an excellent starting point for understanding factory-based object creation. It encapsulates object creation logic in a single class and provides a simple interface for client code.
# Python Implementation
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
class AnimalFactory:
@staticmethod
def create_animal(animal_type):
if animal_type.lower() == "dog":
return Dog()
elif animal_type.lower() == "cat":
return Cat()
raise ValueError("Invalid animal type")
# Usage in MVC Controller
class AnimalController:
def __init__(self, factory=AnimalFactory()):
self.factory = factory
def get_animal_sound(self, animal_type):
try:
animal = self.factory.create_animal(animal_type)
return animal.speak()
except ValueError as e:
return str(e)
Factory Method Pattern
The Factory Method Pattern defines an interface for creating objects but lets subclasses decide which class to instantiate. This pattern promotes loose coupling by eliminating the need to bind application-specific classes into the code.
// Java Implementation
public abstract class DocumentFactory {
abstract Document createDocument();
public Document orderDocument() {
Document document = createDocument();
document.prepare();
document.validate();
return document;
}
}
public class PDFDocumentFactory extends DocumentFactory {
@Override
Document createDocument() {
return new PDFDocument();
}
}
public class WordDocumentFactory extends DocumentFactory {
@Override
Document createDocument() {
return new WordDocument();
}
}
// Usage in MVC Controller
public class DocumentController {
private DocumentFactory factory;
public DocumentController(DocumentFactory factory) {
this.factory = factory;
}
public Document processDocument() {
return factory.orderDocument();
}
}
Integrating Factory Pattern with MVC Architecture
The Factory Pattern integrates seamlessly with MVC architecture by promoting separation of concerns and maintaining clean boundaries between components. Here’s how each MVC component benefits from the Factory Pattern:
Model Integration
Models in MVC often require complex object creation logic, especially when dealing with domain objects or entities. The Factory Pattern helps manage this complexity by centralizing object creation and configuration.
# Python Implementation of Model Factory
class UserModel:
def __init__(self, name, email, role):
self.name = name
self.email = email
self.role = role
class UserFactory:
@staticmethod
def create_user(user_type, name, email):
if user_type == "admin":
return UserModel(name, email, "administrator")
elif user_type == "customer":
return UserModel(name, email, "customer")
raise ValueError("Invalid user type")
# MVC Controller utilizing the factory
class UserController:
def __init__(self, user_factory=UserFactory()):
self.user_factory = user_factory
def register_user(self, user_type, name, email):
try:
user = self.user_factory.create_user(user_type, name, email)
# Additional registration logic
return {"status": "success", "user": user}
except ValueError as e:
return {"status": "error", "message": str(e)}
Abstract Factory Pattern Implementation
The Abstract Factory Pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes. This pattern is particularly useful when dealing with multiple products that need to maintain consistency across a system.
// Java Implementation of Abstract Factory
public interface GUIFactory {
Button createButton();
TextField createTextField();
}
public class WindowsFactory implements GUIFactory {
@Override
public Button createButton() {
return new WindowsButton();
}
@Override
public TextField createTextField() {
return new WindowsTextField();
}
}
public class MacFactory implements GUIFactory {
@Override
public Button createButton() {
return new MacButton();
}
@Override
public TextField createTextField() {
return new MacTextField();
}
}
// MVC Controller implementation
public class GUIController {
private final GUIFactory factory;
public GUIController(GUIFactory factory) {
this.factory = factory;
}
public void createUI() {
Button button = factory.createButton();
TextField textField = factory.createTextField();
// Additional UI creation logic
}
}
Best Practices and Design Considerations
When implementing the Factory Pattern in an MVC architecture, consider the following best practices:
Best Practice | Description | Benefit |
---|---|---|
Dependency Injection | Inject factories into controllers rather than creating them directly | Improves testability and flexibility |
Interface Segregation | Create specific factory interfaces for different object families | Maintains single responsibility principle |
Configuration Management | Use configuration files to manage factory mappings | Enables easy modification without code changes |
Error Handling | Implement robust error handling in factory methods | Improves system reliability and debugging |
Documentation | Thoroughly document factory interfaces and implementations | Facilitates maintenance and team collaboration |
Advanced Factory Pattern Techniques
Dynamic Factory Registration
Implementing a dynamic factory registration system allows for runtime registration of new factory types without modifying existing code.
# Python Implementation of Dynamic Factory
class DynamicFactory:
_factories = {}
@classmethod
def register_factory(cls, key, factory):
cls._factories[key] = factory
@classmethod
def create(cls, key, *args, **kwargs):
factory = cls._factories.get(key)
if not factory:
raise ValueError(f"Factory not found for key: {key}")
return factory(*args, **kwargs)
# Usage in MVC
class ProductController:
def __init__(self, factory=DynamicFactory):
self.factory = factory
def create_product(self, product_type, *args, **kwargs):
try:
return self.factory.create(product_type, *args, **kwargs)
except ValueError as e:
return {"error": str(e)}
Performance Optimization and Caching
When implementing factories in performance-critical applications, consider incorporating caching mechanisms to improve efficiency.
// Java Implementation with Caching
public class CachedProductFactory {
private static final Map<String, Product> cache = new ConcurrentHashMap<>();
public static Product getProduct(String productId) {
return cache.computeIfAbsent(productId, CachedProductFactory::createProduct);
}
private static Product createProduct(String productId) {
// Complex product creation logic
return new Product(productId);
}
}
// MVC Controller with caching
public class CachedProductController {
private final CachedProductFactory factory;
public CachedProductController(CachedProductFactory factory) {
this.factory = factory;
}
public Product getProduct(String productId) {
return factory.getProduct(productId);
}
}
Testing Strategies for Factory Pattern
Effective testing of factory implementations is crucial for maintaining code quality. Here’s an example of testing factory implementations:
# Python Test Implementation
import unittest
class TestUserFactory(unittest.TestCase):
def setUp(self):
self.factory = UserFactory()
def test_create_admin_user(self):
user = self.factory.create_user("admin", "John Doe", "john@example.com")
self.assertEqual(user.role, "administrator")
def test_create_invalid_user(self):
with self.assertRaises(ValueError):
self.factory.create_user("invalid", "John Doe", "john@example.com")
# Test Controller
class TestUserController(unittest.TestCase):
def setUp(self):
self.controller = UserController()
def test_register_user_success(self):
result = self.controller.register_user("admin", "John Doe", "john@example.com")
self.assertEqual(result["status"], "success")
Common Anti-patterns and How to Avoid Them
When implementing the Factory Pattern, be aware of these common anti-patterns:
- **Over-engineering**: Not every object creation needs a factory. Use factories when there’s genuine need for abstraction and flexibility.
- **Tight Coupling**: Avoid hardcoding factory implementations in controllers. Use dependency injection instead.
- **Violation of Single Responsibility**: Keep factories focused on object creation rather than mixing in business logic.
- **Insufficient Error Handling**: Implement comprehensive error handling and validation in factory methods.
Real-world Application Examples
Let’s examine a practical example of implementing the Factory Pattern in a content management system:
// Java CMS Implementation
public interface ContentFactory {
Content createContent(Map<String, Object> data);
void validateContent(Content content);
}
public class ArticleFactory implements ContentFactory {
@Override
public Content createContent(Map<String, Object> data) {
Article article = new Article();
article.setTitle((String) data.get("title"));
article.setBody((String) data.get("body"));
article.setAuthor((String) data.get("author"));
return article;
}
@Override
public void validateContent(Content content) {
Article article = (Article) content;
if (article.getTitle() == null || article.getTitle().isEmpty()) {
throw new ValidationException("Article title is required");
}
// Additional validation logic
}
}
// MVC Controller Implementation
public class ContentController {
private final Map<String, ContentFactory> factories;
public ContentController(Map<String, ContentFactory> factories) {
this.factories = factories;
}
public Content createContent(String type, Map<String, Object> data) {
ContentFactory factory = factories.get(type);
if (factory == null) {
throw new IllegalArgumentException("Invalid content type: " + type);
}
Content content = factory.createContent(data);
factory.validateContent(content);
return content;
}
}
Future Considerations and Scalability
When implementing the Factory Pattern, consider future scalability needs:
- **Extensibility**: Design factories to be easily extended for new types of objects.
- **Configuration**: Use external configuration for factory mappings.
- **Performance**: Implement caching strategies for frequently created objects.
- **Monitoring**: Add logging and metrics to track factory usage and performance.
Conclusion
The Factory Pattern, when properly implemented within an MVC architecture, provides a robust and flexible approach to object creation. By following the best practices and examples outlined in this guide, developers can create maintainable, scalable, and loosely coupled applications. Remember to consider your specific use case when choosing between different factory pattern implementations and always strive for clean, maintainable code that follows SOLID principles.
Disclaimer: The code examples and implementation strategies provided in this blog post are for educational purposes and may need to be adapted to specific use cases and requirements. While we strive for accuracy, some implementations may need to be modified based on specific framework versions or environmental constraints. Please report any inaccuracies or suggestions for improvement to help us maintain the quality of this content.