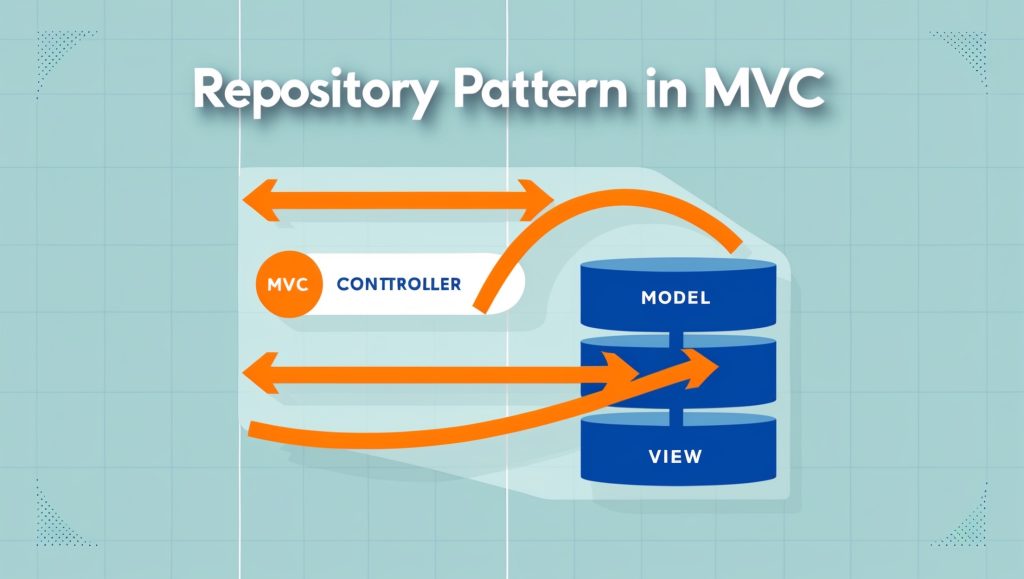
Using the Repository Pattern in MVC
The Repository Pattern has become an essential architectural pattern in modern software development, particularly within the Model-View-Controller (MVC) framework. This pattern serves as a mediator between the domain and data mapping layers, effectively abstracting the complexities of data persistence from the rest of the application. By implementing the Repository Pattern, developers can create a more maintainable, testable, and scalable application architecture. This comprehensive guide will explore the fundamentals of the Repository Pattern, its implementation in both Python and Java, and its practical applications within the MVC architecture.
Understanding the Repository Pattern
The Repository Pattern is designed to create an abstraction layer between the data access and business logic layers of an application. It encapsulates the logic required to access data sources, centralizing common data access functionality and promoting better separation of concerns. This pattern helps in managing domain objects and their lifecycle, from creation to deletion, while maintaining a clean separation between the domain model and data access logic.
Key Benefits of the Repository Pattern
- Centralized data access logic
- Improved maintainability and testability
- Reduced duplication of code
- Better separation of concerns
- Enhanced application scalability
- Simplified unit testing through dependency injection
- Keep repositories focused on single entity types
- Use dependency injection for better testability
- Implement proper error handling and logging
- Consider implementing the Unit of Work pattern for transaction management
- Use asynchronous operations where appropriate
Core Components of the Repository Pattern
The Repository Pattern consists of several key components that work together to provide a robust data access abstraction. Understanding these components is crucial for successful implementation.
Repository Interface
The repository interface defines the contract for data access operations. It typically includes methods for basic CRUD (Create, Read, Update, Delete) operations and any additional data access methods specific to the domain.
Here’s an example in Python:
from abc import ABC, abstractmethod
from typing import List, Optional
from models.user import User
class IUserRepository(ABC):
@abstractmethod
def get_by_id(self, user_id: int) -> Optional[User]:
pass
@abstractmethod
def get_all(self) -> List[User]:
pass
@abstractmethod
def add(self, user: User) -> User:
pass
@abstractmethod
def update(self, user: User) -> bool:
pass
@abstractmethod
def delete(self, user_id: int) -> bool:
pass
And in Java:
public interface IUserRepository {
Optional<User> getById(Long userId);
List<User> getAll();
User add(User user);
boolean update(User user);
boolean delete(Long userId);
}
Implementing the Repository Pattern
The implementation of the Repository Pattern involves creating concrete repository classes that implement the defined interfaces. These classes contain the actual data access logic and can work with different data sources.
Concrete Repository Implementation
Here’s an example of a concrete repository implementation in Python using SQLAlchemy:
from typing import List, Optional
from sqlalchemy.orm import Session
from models.user import User
from repositories.interfaces import IUserRepository
class SQLAlchemyUserRepository(IUserRepository):
def __init__(self, session: Session):
self._session = session
def get_by_id(self, user_id: int) -> Optional[User]:
return self._session.query(User).filter(User.id == user_id).first()
def get_all(self) -> List[User]:
return self._session.query(User).all()
def add(self, user: User) -> User:
self._session.add(user)
self._session.commit()
return user
def update(self, user: User) -> bool:
try:
self._session.merge(user)
self._session.commit()
return True
except Exception:
self._session.rollback()
return False
def delete(self, user_id: int) -> bool:
try:
user = self.get_by_id(user_id)
if user:
self._session.delete(user)
self._session.commit()
return True
return False
except Exception:
self._session.rollback()
return False
And in Java using JPA:
import org.springframework.stereotype.Repository;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import java.util.List;
import java.util.Optional;
@Repository
public class JpaUserRepository implements IUserRepository {
@PersistenceContext
private EntityManager entityManager;
@Override
public Optional<User> getById(Long userId) {
return Optional.ofNullable(entityManager.find(User.class, userId));
}
@Override
public List<User> getAll() {
return entityManager.createQuery("SELECT u FROM User u", User.class)
.getResultList();
}
@Override
public User add(User user) {
entityManager.persist(user);
return user;
}
@Override
public boolean update(User user) {
try {
entityManager.merge(user);
return true;
} catch (Exception e) {
return false;
}
}
@Override
public boolean delete(Long userId) {
try {
User user = entityManager.find(User.class, userId);
if (user != null) {
entityManager.remove(user);
return true;
}
return false;
} catch (Exception e) {
return false;
}
}
}
Integration with MVC Architecture
The Repository Pattern integrates seamlessly with the MVC architecture, providing a clean separation between data access and business logic. Let’s examine how this integration works in practice.
Controller Implementation
Here’s an example of a controller using the repository pattern in Python:
from fastapi import APIRouter, Depends, HTTPException
from typing import List
from models.user import User
from repositories.interfaces import IUserRepository
from dependencies import get_user_repository
router = APIRouter()
@router.get("/users/{user_id}")
async def get_user(user_id: int, repo: IUserRepository = Depends(get_user_repository)):
user = repo.get_by_id(user_id)
if not user:
raise HTTPException(status_code=404, detail="User not found")
return user
@router.get("/users")
async def get_all_users(repo: IUserRepository = Depends(get_user_repository)):
return repo.get_all()
@router.post("/users")
async def create_user(user: User, repo: IUserRepository = Depends(get_user_repository)):
return repo.add(user)
And in Java using Spring MVC:
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/users")
public class UserController {
private final IUserRepository userRepository;
public UserController(IUserRepository userRepository) {
this.userRepository = userRepository;
}
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
return userRepository.getById(id)
.map(ResponseEntity::ok)
.orElse(ResponseEntity.notFound().build());
}
@GetMapping
public List<User> getAllUsers() {
return userRepository.getAll();
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userRepository.add(user);
return ResponseEntity.created(URI.create("/api/users/" + createdUser.getId()))
.body(createdUser);
}
}
Best Practices and Design Considerations
When implementing the Repository Pattern, several best practices should be followed to ensure optimal results.
Repository Design Guidelines
Here’s an example of implementing these practices:
from typing import Generic, TypeVar, Type
from sqlalchemy.orm import Session
from core.exceptions import RepositoryException
from core.logging import logger
T = TypeVar('T')
class BaseRepository(Generic[T]):
def __init__(self, session: Session, model: Type[T]):
self._session = session
self._model = model
async def get_by_id(self, entity_id: int) -> Optional[T]:
try:
return await self._session.get(self._model, entity_id)
except Exception as e:
logger.error(f"Error retrieving entity: {str(e)}")
raise RepositoryException("Failed to retrieve entity") from e
async def add(self, entity: T) -> T:
try:
self._session.add(entity)
await self._session.commit()
return entity
except Exception as e:
await self._session.rollback()
logger.error(f"Error adding entity: {str(e)}")
raise RepositoryException("Failed to add entity") from e
Testing Repository Implementations
Testing is a crucial aspect of implementing the Repository Pattern. Here’s an example of unit testing repository implementations:
import pytest
from unittest.mock import Mock
from repositories.user_repository import SQLAlchemyUserRepository
from models.user import User
class TestUserRepository:
@pytest.fixture
def mock_session(self):
return Mock()
@pytest.fixture
def repository(self, mock_session):
return SQLAlchemyUserRepository(mock_session)
def test_get_by_id_returns_user(self, repository, mock_session):
# Arrange
expected_user = User(id=1, name="Test User")
mock_session.query.return_value.filter.return_value.first.return_value = expected_user
# Act
actual_user = repository.get_by_id(1)
# Assert
assert actual_user == expected_user
mock_session.query.assert_called_once_with(User)
Performance Considerations
When implementing the Repository Pattern, it’s important to consider performance implications and optimization strategies.
Consideration | Impact | Optimization Strategy |
---|---|---|
Eager Loading | Reduces N+1 query problems | Use joins and explicit loading |
Caching | Improves read performance | Implement second-level cache |
Batch Operations | Reduces database round trips | Use bulk insert/update operations |
Connection Pooling | Optimizes resource usage | Configure appropriate pool size |
Advanced Repository Pattern Implementations
The Repository Pattern can be extended to support more complex scenarios and requirements.
Specification Pattern Integration
from abc import ABC, abstractmethod
from typing import List, Type, TypeVar
T = TypeVar('T')
class Specification(ABC):
@abstractmethod
def is_satisfied_by(self, candidate: T) -> bool:
pass
class AndSpecification(Specification):
def __init__(self, *specifications: Specification):
self.specifications = specifications
def is_satisfied_by(self, candidate: T) -> bool:
return all(spec.is_satisfied_by(candidate) for spec in self.specifications)
class UserRepository(IUserRepository):
def find_by_specification(self, spec: Specification) -> List[User]:
return [user for user in self.get_all() if spec.is_satisfied_by(user)]
Conclusion
The Repository Pattern is a powerful tool for managing data access in MVC applications. By providing a clean separation between business logic and data access, it promotes maintainable, testable, and scalable code. The examples and best practices presented in this guide demonstrate how to effectively implement the pattern in both Python and Java environments, while considering important aspects such as testing, performance, and advanced implementations.
Disclaimer: The code examples provided in this blog post are intended for educational purposes and may need to be adapted for production use. While we strive for accuracy in all our technical content, software development practices and frameworks evolve rapidly. Please report any inaccuracies or outdated information to our editorial team so we can maintain the quality and relevance of this guide.