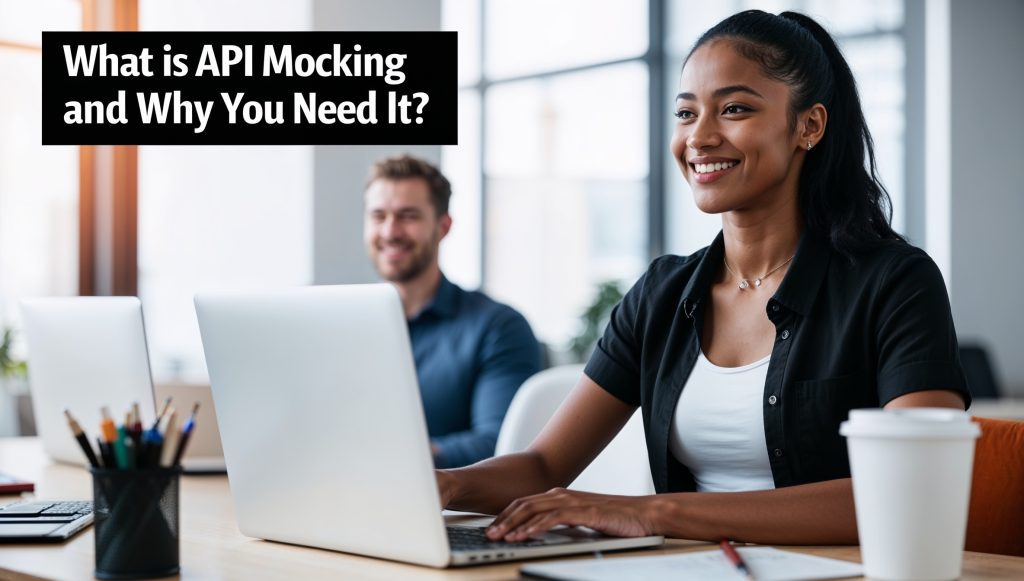
What is API Mocking and Why You Need It?
Ever found yourself scratching your head, wondering what on earth API mocking is all about? Well, you’re in luck because we’re about to embark on a journey through the fascinating world of API mocking. Buckle up, because by the time we’re done, you’ll be mocking APIs like a pro (and no, we’re not talking about making fun of them)! Whether you’re a seasoned developer or just dipping your toes into the vast ocean of coding, this blog post is your friendly guide to understanding why API mocking is the unsung hero of software development. So, grab your favorite beverage, get comfy, and let’s dive into the wonderful world of API mocking!
What in the World is API Mocking?
Before we jump into the nitty-gritty of API mocking, let’s take a step back and make sure we’re all on the same page. API stands for Application Programming Interface, and it’s essentially a set of rules and protocols that allow different software applications to communicate with each other. Think of it as a waiter in a restaurant, taking orders from the customers (your application) and relaying them to the kitchen (another application or service). Now, imagine if that waiter could magically produce the food without ever going to the kitchen. That’s kind of what API mocking does!
API Mocking Defined
API mocking is the practice of simulating the behavior of a real API without actually calling it. It’s like creating a virtual stand-in for the API that behaves just like the real thing, but without all the baggage that comes with it. When you mock an API, you’re essentially creating a fake version of it that you can control and manipulate to suit your needs. This mock API responds to requests just like the real API would, but with data and behavior that you define. It’s like having a stunt double for your API – it looks and acts the part, but it’s not the real deal.
Why Would Anyone Want to Mock an API?
Now, you might be thinking, “Why on earth would I want to create a fake version of something when I have the real thing?” Well, my friend, there are plenty of reasons why API mocking is not just useful, but sometimes absolutely crucial in the software development process. For starters, it allows developers to work independently of the backend team or third-party services. Imagine you’re building a fancy new feature for an app, but the API you need isn’t ready yet. Without mocking, you’d be twiddling your thumbs waiting for the backend team to finish. With mocking, you can forge ahead, building and testing your feature with a simulated API. It’s like being able to practice your dance routine before your dance partner shows up!
The Benefits of API Mocking: More Than Just a Party Trick
Now that we’ve got a handle on what API mocking is, let’s dive into why it’s such a big deal in the world of software development. Trust me, once you see the benefits, you’ll wonder how you ever lived without it!
Speeding Up Development
One of the biggest advantages of API mocking is that it can significantly speed up the development process. When you’re not dependent on the actual API being ready, you can start building and testing your features much earlier in the development cycle. This means less time waiting around and more time actually coding. It’s like being able to start building your house before the foundation is even laid – you can work on the blueprints, design the interior, and even start picking out furniture, all while waiting for the concrete to dry.
Improved Testing
API mocking is a testing powerhouse. It allows you to easily simulate different scenarios and edge cases that might be difficult or impossible to reproduce with a real API. Want to test how your app handles a server timeout? No problem. Need to see what happens when the API returns unexpected data? Easy peasy. With mocking, you’re in control of the API’s behavior, which means you can test to your heart’s content without worrying about external factors. It’s like having a magical testing playground where you make the rules!
Consistent and Reliable Development Environment
When you’re working with real APIs, especially third-party ones, you’re at the mercy of their availability and performance. What if the API is down for maintenance? What if it’s running slowly? These factors can throw a wrench in your development process. With API mocking, you create a consistent and reliable environment for development and testing. Your mock API is always available, always fast, and always behaves exactly as you expect it to. It’s like having a personal chef who’s always ready to whip up whatever dish you’re craving, exactly the way you like it.
Cost-Effective Solution
Some APIs charge based on the number of requests you make. During development and testing, you might end up making a lot of calls to the API, which can rack up quite a bill. API mocking allows you to simulate these calls without actually making them, potentially saving you a significant amount of money. It’s like being able to practice your golf swing without having to pay for rounds at the country club!
How Does API Mocking Work? A Peek Behind the Curtain
Now that we’ve covered the “what” and “why” of API mocking, let’s dive into the “how.” Don’t worry, we won’t get too technical – this is still API Mocking for Dummies, after all!
The Basics of API Mocking
At its core, API mocking involves creating a simulated version of the API that intercepts requests meant for the real API and responds with predefined data. It’s like setting up a cardboard cutout of a person – from a distance, it looks like the real thing, and it serves the purpose you need it to. The mock API mimics the behavior of the real API, including its endpoints, request/response format, and even potential errors or edge cases.
Tools of the Trade
There are various tools and libraries available for API mocking, depending on your programming language and specific needs. Some popular options include Mockito for Java, Nock for Node.js, and WireMock for HTTP-based APIs. These tools provide frameworks for creating mock APIs, defining their behavior, and integrating them into your development and testing processes. It’s like having a toolbox full of different types of hammers – each one is designed for a specific purpose, but they all help you build something amazing.
Creating a Mock API
Let’s walk through a simple example of how you might create a mock API using Java and Mockito. Imagine we have a WeatherService
interface that looks like this:
public interface WeatherService {
String getWeatherForecast(String city);
}
Now, let’s say we want to mock this service for testing. Here’s how we might do it:
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
import static org.junit.jupiter.api.Assertions.*;
public class WeatherAppTest {
@Test
public void testWeatherForecast() {
// Create a mock of the WeatherService
WeatherService mockWeatherService = mock(WeatherService.class);
// Define the behavior of the mock
when(mockWeatherService.getWeatherForecast("New York"))
.thenReturn("Sunny, 75°F");
// Use the mock in our test
String forecast = mockWeatherService.getWeatherForecast("New York");
// Assert that we got the expected result
assertEquals("Sunny, 75°F", forecast);
// Verify that the method was called with the correct argument
verify(mockWeatherService).getWeatherForecast("New York");
}
}
In this example, we’re creating a mock of the WeatherService
, defining its behavior (returning “Sunny, 75°F” for New York), and then using it in our test. This allows us to test our weather app’s logic without actually calling a real weather API.
Common Use Cases for API Mocking: When to Pull Out Your Mocking Tools
Now that we’ve got a handle on what API mocking is and how it works, let’s explore some common scenarios where you might want to reach for your mocking toolbox. Trust me, once you start seeing the potential, you’ll be looking for excuses to mock APIs!
Development Without Dependencies
Imagine you’re working on a new feature for an e-commerce app that needs to integrate with a payment gateway. The catch? The payment gateway API isn’t ready yet. Without API mocking, you’d be stuck twiddling your thumbs, waiting for the API to be completed. But with mocking, you can create a simulated version of the payment gateway API and forge ahead with your development. You can define the expected request and response formats, simulate successful payments, and even test error scenarios. It’s like being able to practice your duet before your singing partner arrives!
Testing Edge Cases
APIs can sometimes behave in unexpected ways. Maybe the server is slow to respond, or perhaps it returns an error code you weren’t expecting. Testing these scenarios with a real API can be challenging and unreliable. With API mocking, you can easily simulate these edge cases and ensure your application handles them gracefully. Want to test what happens when the API times out? No problem. Need to see how your app responds to a 404 error? Easy peasy. It’s like being a movie director with complete control over every aspect of your scene – you can create any scenario you want to test.
Performance Testing
When you’re load testing an application, you don’t want external APIs to become a bottleneck or skew your results. By mocking these APIs, you can isolate your application’s performance and get a clearer picture of how it behaves under load. You can simulate fast responses, slow responses, or even gradual degradation of performance. It’s like being able to test drive a car on a perfectly controlled track, without having to worry about traffic or road conditions.
Offline Development
Sometimes, you need to work on your application when you don’t have an internet connection. Maybe you’re on a long flight, or perhaps you’re stuck in a cabin in the woods (hey, it could happen!). With API mocking, you can continue development and testing even when you’re offline. Your mock APIs will always be available, ready to respond to your requests. It’s like having a genie in a bottle that grants your API wishes, no internet required!
Best Practices for API Mocking: Dos and Don’ts
Alright, now that you’re all fired up about API mocking, let’s talk about how to do it right. Like any powerful tool, API mocking can be a double-edged sword if not used properly. But don’t worry – I’ve got your back with some best practices that’ll help you mock like a pro!
Do: Keep Your Mocks Close to Reality
When creating mock APIs, it’s tempting to just return whatever data makes your tests pass. But resist that urge! Try to make your mock APIs behave as close to the real APIs as possible. Use realistic data structures, respect the same constraints (like rate limits), and simulate real-world scenarios. This will help ensure that your code works correctly when you switch to the real API. It’s like practicing a dance routine with a partner who moves just like your real dance partner – the transition to the real thing will be seamless!
Don’t: Rely Exclusively on Mocks
While API mocking is incredibly useful, it shouldn’t be your only testing strategy. Make sure to also test with the real API when possible. Mocks are great for development and initial testing, but you’ll want to validate everything with the real API before pushing to production. Think of it like rehearsing a play – you practice with stand-ins, but you always have a dress rehearsal with the full cast before opening night.
Do: Version Your Mocks
APIs evolve over time, and your mocks should too. Keep your mock APIs versioned along with your code. This way, if you need to work on an older version of your application, you’ll have the corresponding mock APIs available. It’s like keeping a wardrobe of different costumes – you want to make sure you have the right outfit for every occasion!
Don’t: Hardcode Expected Values in Your Tests
When writing tests that use mock APIs, avoid hardcoding expected values directly in your test cases. Instead, define these values in a configuration file or constants. This makes it easier to update your tests if the API changes and keeps your test code cleaner. It’s like using variables in a recipe instead of specific measurements – it makes it much easier to scale up or down as needed.
Do: Use Mocking to Test Error Handling
Don’t just mock the happy path – use API mocking to test how your application handles errors and edge cases. Simulate timeouts, server errors, malformed responses, and other scenarios that might be difficult to reproduce with a real API. This will help make your application more robust and reliable. It’s like being a stunt coordinator, making sure your star can handle any situation that’s thrown at them!
Tools of the Trade: Popular API Mocking Solutions
Now that we’ve covered the best practices, let’s take a look at some of the tools you can use to implement API mocking in your projects. There’s a whole toolbox of options out there, each with its own strengths and quirks. Let’s explore some of the popular choices!
Mockito (Java)
Mockito is a mocking framework for Java that makes it easy to create mock objects in automated unit tests. It’s known for its clean and simple API, which allows developers to write beautiful tests with a fluent interface. Here’s a quick example of how you might use Mockito:
import static org.mockito.Mockito.*;
// Create a mock
List mockedList = mock(List.class);
// Use mock object
mockedList.add("one");
mockedList.clear();
// Verification
verify(mockedList).add("one");
verify(mockedList).clear();
Mockito is like the Swiss Army knife of mocking tools – it’s versatile, reliable, and gets the job done with style.
WireMock (HTTP)
WireMock is a flexible library for stubbing and mocking web services. It can be used to mock HTTP-based APIs, making it a great choice for testing applications that depend on external web services. WireMock can be run as a standalone process or embedded into your Java code. Here’s a simple example:
import static com.github.tomakehurst.wiremock.client.WireMock.*;
stubFor(get(urlEqualTo("/some/thing"))
.willReturn(aResponse()
.withHeader("Content-Type", "text/plain")
.withBody("Hello world!")));
WireMock is like having a miniature web server at your disposal, ready to respond however you want it to.
Nock (Node.js)
Nock is an HTTP mocking and expectations library for Node.js. It’s great for testing modules that perform HTTP requests. With Nock, you can easily mock HTTP responses and verify that your code makes the expected requests. Here’s a quick example:
const nock = require('nock');
// Define a mock response
nock('https://api.example.com')
.get('/users')
.reply(200, { users: [{ id: 1, name: 'John' }] });
// Your code that makes a request to 'https://api.example.com/users'
// will now receive the mocked response
Nock is like having a traffic controller for your HTTP requests – it intercepts outgoing requests and returns whatever responses you’ve defined.
Postman
While primarily known as an API testing tool, Postman also offers powerful mocking capabilities. You can create mock servers directly within Postman, making it easy to simulate API responses without setting up a real backend. Here’s how you might set up a mock server in Postman:
- Create a new collection
- Add a request to the collection
- Click on the “Mocks” tab
- Create a new mock server for the collection
- Define the response you want for each request
Postman is like having a fully equipped API testing laboratory at your fingertips – you can test, mock, and document your APIs all in one place.
Real-World Examples: API Mocking in Action
Theory is great, but sometimes it helps to see how API mocking is used in real-world scenarios. Let’s look at a couple of examples that demonstrate the power and versatility of API mocking.
E-commerce Application
Imagine you’re building an e-commerce application that needs to integrate with multiple external services: a product inventory API, a payment gateway, and a shipping service. Each of these services is being developed by different teams, and they won’t all be ready at the same time. This is where API mocking comes to the rescue!
You can create mock versions of each of these APIs:
- Product Inventory API: You can mock this to return a list of products, their prices, and availability. This allows you to develop and test your product listing and detail pages without waiting for the real inventory system to be ready.
- Payment Gateway: You can mock different payment scenarios – successful payments, declined cards, network errors. This lets you build and test your checkout process thoroughly.
- Shipping Service: You can mock responses for shipping cost calculations and tracking information. This enables you to develop the order confirmation and tracking features of your application.
By mocking these APIs, you can develop and test your entire e-commerce flow independently of the external services. It’s like being able to rehearse a complex dance routine with virtual partners who never get tired or make mistakes!
Weather App
Let’s say you’re developing a weather app that relies on data from a third-party weather API. Here’s how API mocking can be useful:
- Offline Development: You can create mock responses for different weather conditions, allowing you to develop and test your app’s UI for sunny, rainy, or snowy weather, even when you’re coding on a plane or in a remote cabin with no internet.
- Error Handling: You can mock API errors or slow responses to ensure your app gracefully handles these scenarios. This helps you create a robust app that doesn’t crash when the network is spotty or the API is down.
- Location Testing: You can mock responses for different cities or countries without having to physically travel there or manipulate your device’s GPS. Want to see how your app displays weather for Tokyo or Timbuktu? No problem!
By using API mocking, you can ensure your weather app looks great and functions perfectly in all conditions, without having to wait for the perfect storm (or sunshine) in real life.
Overcoming Challenges in API Mocking
While API mocking is incredibly useful, it’s not without its challenges. But don’t worry – we’ve got solutions for you! Let’s look at some common hurdles you might face and how to leap over them gracefully.
Challenge: Keeping Mocks Up-to-Date
As the real API evolves, your mocks need to keep pace. Otherwise, you might find yourself testing against an outdated version of the API, leading to nasty surprises when you switch to the real thing.
Solution: Implement a regular review process for your mock APIs. Whenever the real API is updated, make it a priority to update your mocks accordingly. You can also use tools that generate mocks from API specifications (like OpenAPI/Swagger) to help keep things in sync.
Challenge: Mocks Becoming a Crutch
It’s easy to become overly reliant on mocks, especially when they’re working well. But remember, mocks are a simulation, not the real deal.
Solution: Make sure to include integration tests with the real API in your testing strategy. Use mocks for development and initial testing, but always validate against the real API before pushing to production. It’s like practicing in a flight simulator – incredibly useful, but you need to log some real flight hours too!
Challenge: Managing Complex Mock Scenarios
As your application grows, you might find yourself needing to mock increasingly complex scenarios involving multiple APIs and intricate data relationships.
Solution: Use a dedicated API mocking tool that supports scenario-based mocking. These tools allow you to define complex sequences of requests and responses, simulating real-world usage patterns. It’s like being a movie director – you can create and manage elaborate scenes with multiple actors (APIs) interacting in precise ways.
The Future of API Mocking: What’s on the Horizon?
As we wrap up our journey through the land of API mocking, let’s gaze into our crystal ball and see what the future might hold for this essential development technique.
AI-Powered Mocking
Imagine AI that can analyze your application and automatically generate appropriate mock responses. As artificial intelligence continues to advance, we might see tools that can create increasingly sophisticated and context-aware mocks, making the process even more seamless for developers.
Increased Integration with CI/CD Pipelines
We’re likely to see even tighter integration of API mocking into continuous integration and continuous deployment (CI/CD) pipelines. This could mean automated updates to mocks based on changes to the real API, ensuring that your tests are always running against the most up-to-date mocks.
Virtual API Environments
The future might bring us complete virtual API environments, where entire ecosystems of interconnected APIs can be simulated. This would allow developers to test complex, microservices-based applications in a completely controlled environment.
Wrapping Up
And there you have it, folks – your whirlwind tour of the wonderful world of API mocking! We’ve covered what it is, why it’s crucial, how to do it right, and even peeked into its future. By now, you should be feeling ready to don your mocking cape and start creating some API magic of your own.
Remember, API mocking is more than just a development technique – it’s a superpower that allows you to bend the rules of reality (well, digital reality at least). It gives you the freedom to develop and test your applications in ways that would be impossible or impractical with real APIs. So go forth and mock! Your future self (and your team, and your users) will thank you for it.
As you embark on your API mocking journey, keep in mind that like any powerful tool, it requires practice and wisdom to use effectively. Start small, experiment, and gradually incorporate mocking into your development workflow. Before you know it, you’ll be orchestrating complex mock scenarios with the finesse of a seasoned conductor.
So, whether you’re building the next big e-commerce platform, a weather app that would make meteorologists jealous, or anything in between, remember that with API mocking in your toolkit, the sky’s the limit. Happy mocking, and may all your API calls be swift and successful!
Disclaimer: While we strive for accuracy in all our content, the world of technology moves fast. Some information in this article may become outdated over time. Always refer to the latest documentation and best practices when implementing API mocking in your projects. If you spot any inaccuracies, please let us know so we can update our information promptly. Remember, in the world of API mocking, even our mistakes can be valuable learning opportunities!