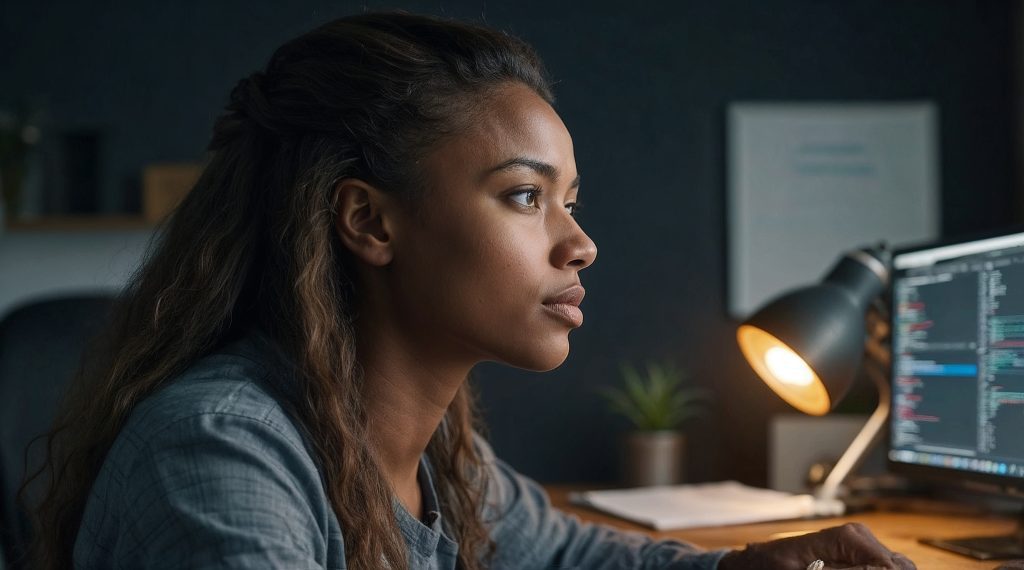
What is Hibernate and Why Should You Care?
In the vast ecosystem of Java development, Hibernate stands out as a game-changer. But what exactly is Hibernate, and why should you, as a developer, sit up and take notice? At its core, Hibernate is an open-source Object-Relational Mapping (ORM) framework that simplifies the way Java applications interact with relational databases. It acts as a bridge between the object-oriented world of Java and the relational world of databases, allowing developers to work with persistent objects rather than dealing directly with database tables and SQL queries.
Think of Hibernate as a talented translator, fluent in both Java and SQL. When you work with Hibernate, you write your code in Java, and Hibernate takes care of translating that into the appropriate SQL statements for your database. This abstraction layer not only saves you time but also reduces the likelihood of errors that can creep in when manually writing complex SQL queries.
But the benefits of Hibernate don’t stop there. By using Hibernate, you gain database independence. Want to switch from MySQL to PostgreSQL? With Hibernate, it’s a breeze. The framework handles the database-specific SQL, allowing your application to remain largely agnostic to the underlying database system. This flexibility can be a lifesaver when scaling your application or adapting to different deployment environments.
Moreover, Hibernate brings the power of caching to your application. It implements multiple levels of caching, which can significantly boost your application’s performance by reducing the number of database hits. This means faster response times and happier users – a win-win situation for any developer.
Key Benefits of Hibernate:
- Productivity Boost: Say goodbye to writing boilerplate JDBC code and SQL queries. Hibernate handles the heavy lifting, allowing you to focus on your application logic.
- Maintainability: With less code to manage and a clear separation of concerns, your applications become easier to maintain and evolve over time.
- Performance Optimization: Hibernate’s intelligent fetching strategies and caching mechanisms can dramatically improve your application’s performance.
- Database Portability: Write your application once and deploy it with different database systems without major code changes.
As we delve deeper into this tutorial, you’ll discover how these benefits translate into real-world advantages for your Java projects. But first, let’s roll up our sleeves and get our hands dirty with some code!
Setting Up Your Hibernate Environment
Before we can start reaping the benefits of Hibernate, we need to set up our development environment. Don’t worry – it’s not as daunting as it might sound. We’ll walk through the process step by step, ensuring you have everything you need to start your Hibernate journey.
Step 1: Choose Your Tools
First things first, you’ll need a Java Development Kit (JDK) installed on your machine. Hibernate works with Java 8 and above, so make sure you have a compatible version. Next, you’ll want an Integrated Development Environment (IDE). While you can use any text editor, an IDE like IntelliJ IDEA, Eclipse, or NetBeans can significantly enhance your productivity when working with Hibernate.
Step 2: Add Hibernate Dependencies
The easiest way to incorporate Hibernate into your project is by using a build tool like Maven or Gradle. If you’re using Maven, add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.6.5.Final</version>
</dependency>
If you prefer Gradle, add this to your build.gradle
file:
implementation 'org.hibernate:hibernate-core:5.6.5.Final'
Remember to also include the JDBC driver for your specific database. For example, if you’re using MySQL, you’d add:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.27</version>
</dependency>
Step 3: Configure Hibernate
Hibernate needs to know how to connect to your database and how to behave in certain situations. This configuration can be done either through an XML file or programmatically. Let’s look at both approaches:
XML Configuration (hibernate.cfg.xml
):
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydb</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<!-- JDBC connection pool settings -->
<property name="hibernate.c3p0.min_size">5</property>
<property name="hibernate.c3p0.max_size">20</property>
<!-- Specify dialect -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- Echo all executed SQL to stdout -->
<property name="hibernate.show_sql">true</property>
<!-- Drop and re-create the database schema on startup -->
<property name="hibernate.hbm2ddl.auto">update</property>
</session-factory>
</hibernate-configuration>
Programmatic Configuration:
Configuration configuration = new Configuration();
configuration.setProperty("hibernate.connection.driver_class", "com.mysql.cj.jdbc.Driver");
configuration.setProperty("hibernate.connection.url", "jdbc:mysql://localhost:3306/mydb");
configuration.setProperty("hibernate.connection.username", "root");
configuration.setProperty("hibernate.connection.password", "password");
configuration.setProperty("hibernate.dialect", "org.hibernate.dialect.MySQLDialect");
configuration.setProperty("hibernate.show_sql", "true");
configuration.setProperty("hibernate.hbm2ddl.auto", "update");
SessionFactory sessionFactory = configuration.buildSessionFactory();
With these steps completed, you’re now ready to start using Hibernate in your Java application. In the next section, we’ll dive into the heart of Hibernate: mapping Java objects to database tables.
The Heart of Hibernate: Mapping Java Objects to Database Tables
At the core of Hibernate’s magic lies its ability to map Java objects to database tables. This mapping is what allows you to work with your data using familiar object-oriented concepts, while Hibernate takes care of the behind-the-scenes work of translating these operations into database actions.
Understanding Entity Classes
In Hibernate, a Java class that represents a database table is called an entity. Let’s create a simple User
entity to illustrate how this works:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "first_name")
private String firstName;
@Column(name = "last_name")
private String lastName;
@Column(unique = true)
private String email;
// Getters and setters
// ...
}
Let’s break down what’s happening in this class:
- The
@Entity
annotation tells Hibernate that this class is an entity that should be persisted to the database. @Table(name = "users")
specifies the name of the database table this entity maps to.@Id
marks theid
field as the primary key of the table.@GeneratedValue
indicates that theid
should be automatically generated by the database.@Column
annotations provide additional mapping information, such as the column name in the database or constraints likeunique = true
.
Mapping Relationships
Of course, in real-world applications, entities often have relationships with other entities. Hibernate provides annotations to map these relationships as well. Let’s add an Address
entity and establish a one-to-many relationship with User
:
@Entity
@Table(name = "addresses")
public class Address {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String street;
private String city;
private String country;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
// Getters and setters
// ...
}
And update our User
class:
@Entity
@Table(name = "users")
public class User {
// ... previous fields ...
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL)
private List<Address> addresses = new ArrayList<>();
// ... getters and setters ...
}
Here, we’ve used @ManyToOne
and @OneToMany
annotations to establish a bidirectional relationship between User
and Address
. The cascade = CascadeType.ALL
means that operations performed on a User
entity will cascade to its associated Address
entities.
Inheritance Mapping
Hibernate also supports mapping inheritance relationships. Let’s say we have different types of users in our system:
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name = "user_type")
public abstract class User {
// ... common fields and methods ...
}
@Entity
@DiscriminatorValue("CUSTOMER")
public class Customer extends User {
private String loyaltyNumber;
// ... customer-specific fields and methods ...
}
@Entity
@DiscriminatorValue("EMPLOYEE")
public class Employee extends User {
private String department;
// ... employee-specific fields and methods ...
}
In this example, we’re using the single table inheritance strategy, where all subclasses are stored in the same table, distinguished by a discriminator column.
By mastering these mapping techniques, you’ll be able to represent even the most complex domain models in your Hibernate-powered applications. In the next section, we’ll explore how to perform CRUD operations using these mapped entities.
That concludes the first three major sections of the blog post. Would you like me to continue with the next sections, or would you like to review or make any changes to what I’ve written so far?
Certainly, I’ll complete the remaining sections of the blog post. Here’s the continuation:
CRUD Operations with Hibernate: A Developer’s Dream
Now that we’ve set up our environment and mapped our entities, it’s time to dive into the bread and butter of database operations: Create, Read, Update, and Delete (CRUD). Hibernate simplifies these operations, allowing you to work with Java objects rather than SQL statements.
Creating and Saving Objects
Let’s start by creating and saving a new User
object:
Session session = sessionFactory.openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
User user = new User();
user.setFirstName("John");
user.setLastName("Doe");
user.setEmail("john.doe@example.com");
Address address = new Address();
address.setStreet("123 Main St");
address.setCity("Anytown");
address.setCountry("USA");
address.setUser(user);
user.getAddresses().add(address);
session.save(user);
transaction.commit();
} catch (Exception e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
In this example, we’re creating a new User
with an associated Address
. Notice how we don’t need to explicitly save the Address
object – it’s saved automatically thanks to the cascade setting we defined earlier.
Reading Objects
Retrieving objects from the database is equally straightforward:
Session session = sessionFactory.openSession();
try {
User user = session.get(User.class, 1L); // 1L is the ID of the user we want to retrieve
System.out.println("User: " + user.getFirstName() + " " + user.getLastName());
for (Address address : user.getAddresses()) {
System.out.println("Address: " + address.getStreet() + ", " + address.getCity());
}
} finally {
session.close();
}
Updating Objects
Updating objects is as simple as modifying the Java object and saving it back to the database:
Session session = sessionFactory.openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
User user = session.get(User.class, 1L);
user.setLastName("Smith");
Address newAddress = new Address();
newAddress.setStreet("456 Elm St");
newAddress.setCity("Other Town");
newAddress.setCountry("USA");
newAddress.setUser(user);
user.getAddresses().add(newAddress);
session.update(user);
transaction.commit();
} catch (Exception e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
Deleting Objects
Finally, deleting objects is equally straightforward:
Session session = sessionFactory.openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
User user = session.get(User.class, 1L);
session.delete(user);
transaction.commit();
} catch (Exception e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
Thanks to our cascade settings, deleting a User
will also delete all associated Address
entities.
Querying with Hibernate: HQL and Criteria API
While basic CRUD operations are essential, real-world applications often require more complex querying capabilities. Hibernate provides two powerful mechanisms for this: Hibernate Query Language (HQL) and the Criteria API.
Hibernate Query Language (HQL)
HQL is an object-oriented query language similar to SQL, but instead of operating on tables and columns, it works with persistent objects and their properties. Here’s an example:
Session session = sessionFactory.openSession();
try {
String hql = "FROM User u WHERE u.lastName = :lastName";
Query<User> query = session.createQuery(hql, User.class);
query.setParameter("lastName", "Doe");
List<User> users = query.list();
for (User user : users) {
System.out.println("User: " + user.getFirstName() + " " + user.getLastName());
}
} finally {
session.close();
}
This query retrieves all User
entities with the last name “Doe”. HQL supports a wide range of operations, including joins, aggregations, and subqueries.
Criteria API
The Criteria API provides a type-safe, programmatic way of constructing queries. It’s particularly useful for building dynamic queries:
Session session = sessionFactory.openSession();
try {
CriteriaBuilder builder = session.getCriteriaBuilder();
CriteriaQuery<User> criteriaQuery = builder.createQuery(User.class);
Root<User> root = criteriaQuery.from(User.class);
criteriaQuery.select(root)
.where(builder.equal(root.get("lastName"), "Doe"));
List<User> users = session.createQuery(criteriaQuery).getResultList();
for (User user : users) {
System.out.println("User: " + user.getFirstName() + " " + user.getLastName());
}
} finally {
session.close();
}
This example achieves the same result as the HQL query above, but using the Criteria API.
Hibernate Relationships: Navigating the Web of Connections
We’ve already touched on relationships when we set up our User
and Address
entities, but let’s dive deeper into how Hibernate handles various types of relationships.
One-to-Many and Many-to-One
We’ve already seen an example of this with our User
and Address
entities. Let’s revisit it:
@Entity
public class User {
// ...
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL)
private List<Address> addresses = new ArrayList<>();
// ...
}
@Entity
public class Address {
// ...
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
// ...
}
This setup allows a User
to have multiple Address
entities, and each Address
is associated with one User
.
Many-to-Many
Let’s introduce a new entity, Project
, and create a many-to-many relationship with User
:
@Entity
public class User {
// ...
@ManyToMany(cascade = {CascadeType.PERSIST, CascadeType.MERGE})
@JoinTable(
name = "user_project",
joinColumns = @JoinColumn(name = "user_id"),
inverseJoinColumns = @JoinColumn(name = "project_id")
)
private Set<Project> projects = new HashSet<>();
// ...
}
@Entity
public class Project {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToMany(mappedBy = "projects")
private Set<User> users = new HashSet<>();
// ...
}
This setup creates a join table user_project
to manage the many-to-many relationship between User
and Project
.
One-to-One
Finally, let’s add a one-to-one relationship. We’ll create a UserProfile
entity that has a one-to-one relationship with User
:
@Entity
public class User {
// ...
@OneToOne(mappedBy = "user", cascade = CascadeType.ALL)
private UserProfile profile;
// ...
}
@Entity
public class UserProfile {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String bio;
@OneToOne
@JoinColumn(name = "user_id")
private User user;
// ...
}
This setup ensures that each User
can have one UserProfile
, and each UserProfile
is associated with one User
.
Performance Optimization: Turbocharging Your Hibernate Applications
While Hibernate can significantly simplify database operations, it’s important to use it wisely to ensure optimal performance. Here are some key strategies:
1. Use Appropriate Fetch Types
Hibernate provides two fetch types: EAGER and LAZY. EAGER fetching loads related entities immediately, while LAZY fetching loads them only when accessed.
@Entity
public class User {
// ...
@OneToMany(mappedBy = "user", fetch = FetchType.LAZY)
private List<Address> addresses;
// ...
}
In general, it’s recommended to use LAZY fetching for collections to avoid loading unnecessary data.
2. Leverage Caching
Hibernate provides a powerful caching mechanism that can significantly improve performance:
@Entity
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class User {
// ...
}
This enables second-level caching for the User
entity, reducing database hits for frequently accessed data.
3. Use Batch Processing
For bulk operations, use Hibernate’s batch processing capabilities:
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
for (int i = 0; i < 100000; i++) {
User user = new User();
user.setFirstName("User" + i);
session.save(user);
if (i % 50 == 0) {
session.flush();
session.clear();
}
}
tx.commit();
session.close();
This approach can significantly speed up bulk inserts or updates.
Best Practices and Common Pitfalls to Avoid
As we wrap up our Hibernate journey, let’s review some best practices and common pitfalls:
Best Practices:
- Use meaningful and consistent naming conventions for entities and their properties.
- Always use transactions for data modifications.
- Close Session and SessionFactory objects when they’re no longer needed.
- Use query parameters to prevent SQL injection attacks.
- Optimize your entity mappings based on your specific use cases.
Common Pitfalls:
- N+1 Select Problem: This occurs when you fetch a list of entities and then access a lazy-loaded collection for each one. Use join fetching or batch fetching to mitigate this.
- Detached Entity Exceptions: Be careful when working with entities across different sessions.
- Overuse of Eager Fetching: This can lead to performance issues due to excessive data loading.
- Ignoring Caching: Proper use of caching can dramatically improve performance.
Embracing the Hibernate Revolution
As we’ve seen throughout this tutorial, Hibernate offers a powerful and flexible approach to database interactions in Java applications. By abstracting away much of the complexity of JDBC and SQL, it allows developers to focus on their domain logic rather than the intricacies of database operations.
From basic CRUD operations to complex querying and performance optimization, Hibernate provides a comprehensive toolkit for building robust, efficient, and maintainable database-driven applications. By mastering Hibernate, you’re not just learning a framework – you’re adopting a paradigm that can revolutionize the way you think about and interact with databases in your Java projects.
Remember, like any powerful tool, Hibernate requires understanding and careful use to reap its full benefits. But with the knowledge you’ve gained from this tutorial, you’re well-equipped to start your Hibernate journey and take your Java database interactions to the next level.
Happy coding, and may your Hibernate adventures be bug-free and performant!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this tutorial, it is provided for educational purposes only. Technologies and best practices may change over time, and readers are encouraged to consult official documentation and stay updated with the latest developments in Hibernate and related technologies. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.