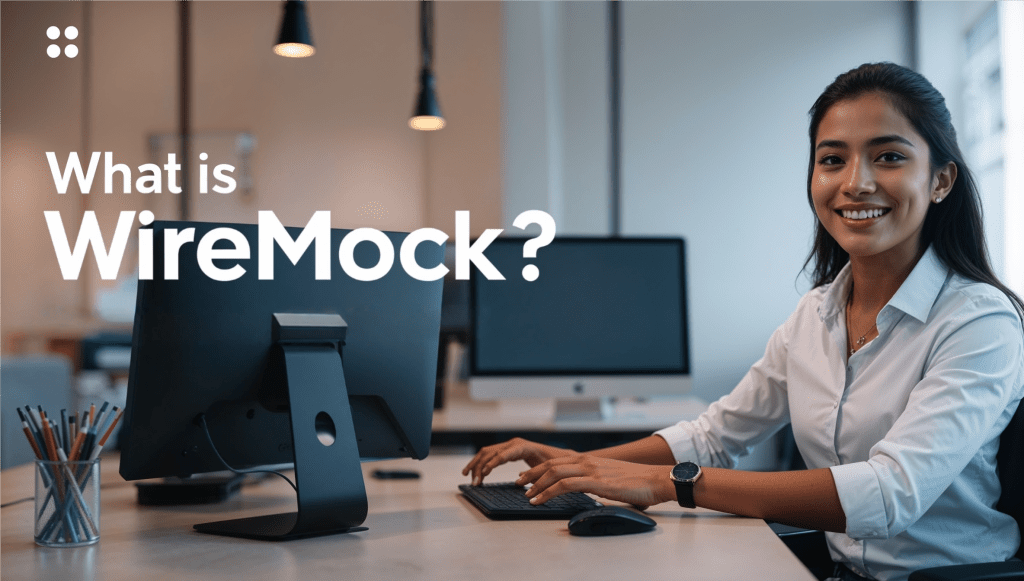
What is WireMock and How to Use It?
Before we jump into the nitty-gritty details, let’s take a moment to understand what WireMock is and why it’s such a game-changer in the world of software testing. WireMock is an HTTP mock server that allows you to simulate HTTP-based APIs for testing purposes. It’s like having a magical genie that can impersonate any web service you want, without the need for an actual internet connection or live server. Pretty cool, right?
But why should you care about WireMock? Well, imagine you’re developing an application that relies on external APIs. These APIs might be slow, unreliable, or have usage limits. With WireMock, you can create mock responses that mimic these APIs, giving you complete control over the testing environment. This means faster, more reliable tests, and the ability to simulate edge cases that might be difficult or impossible to reproduce with real services. Plus, you can develop and test your application offline, which is perfect for those coding sessions on long flights or in remote cabins (we’ve all been there, right?).
Getting Started with WireMock
Now that we’ve piqued your interest, let’s roll up our sleeves and get WireMock up and running on your machine. Don’t worry; it’s easier than assembling IKEA furniture, and we promise you won’t have any leftover screws at the end!
Step 1: Adding WireMock to Your Project
The first step in our WireMock journey is to add it to your project. If you’re using Maven (and let’s face it, who isn’t these days?), you can simply add the following dependency to your pom.xml
file:
<dependency>
<groupId>com.github.tomakehurst</groupId>
<artifactId>wiremock-jre8</artifactId>
<version>2.35.0</version>
<scope>test</scope>
</dependency>
If you’re more of a Gradle person (we don’t judge), you can add this to your build.gradle
file:
testImplementation 'com.github.tomakehurst:wiremock-jre8:2.35.0'
Make sure to check for the latest version on the WireMock GitHub repository, as the tech world moves faster than a caffeinated squirrel!
Step 2: Setting Up a WireMock Server
Now that we have WireMock in our project, it’s time to create our mock server. There are two main ways to do this: using JUnit rules or programmatically. Let’s look at both approaches, shall we?
Using JUnit Rule:
import com.github.tomakehurst.wiremock.junit.WireMockRule;
import org.junit.Rule;
import org.junit.Test;
import static com.github.tomakehurst.wiremock.core.WireMockConfiguration.options;
public class MyAwesomeTest {
@Rule
public WireMockRule wireMockRule = new WireMockRule(options().port(8089));
@Test
public void testSomethingCool() {
// Your test code here
}
}
Programmatically:
import com.github.tomakehurst.wiremock.WireMockServer;
import static com.github.tomakehurst.wiremock.core.WireMockConfiguration.options;
public class MyEvenMoreAwesomeTest {
private WireMockServer wireMockServer;
@Before
public void setup() {
wireMockServer = new WireMockServer(options().port(8089));
wireMockServer.start();
}
@After
public void teardown() {
wireMockServer.stop();
}
@Test
public void testSomethingEvenCooler() {
// Your test code here
}
}
Both approaches accomplish the same thing: starting a WireMock server on port 8089. Choose the one that fits your testing style best. It’s like choosing between coffee and tea – there’s no wrong answer, just personal preference!
Stubbing HTTP Requests
Now that our WireMock server is up and running, it’s time to teach it some tricks. In WireMock lingo, we call this “stubbing” – it’s like training a digital puppy to respond to specific commands, except instead of “sit” and “stay,” we’re using HTTP methods and URLs.
Basic Stubbing
Let’s start with a simple example. Say we want to mock a GET request to “/hello” that returns a friendly greeting:
stubFor(get(urlEqualTo("/hello"))
.willReturn(aResponse()
.withStatus(200)
.withHeader("Content-Type", "text/plain")
.withBody("Hello, WireMock!")));
This stub tells WireMock to respond with a 200 status code, a plain text content type, and a cheerful message whenever it receives a GET request to “/hello”. It’s like setting up an automated greeter for your API – always polite, never takes a coffee break!
Stubbing with Request Matching
But wait, there’s more! WireMock isn’t just a one-trick pony. You can get really specific with your request matching. Let’s say you want to mock a POST request that only responds when the request body contains specific JSON:
stubFor(post(urlEqualTo("/api/users"))
.withHeader("Content-Type", equalTo("application/json"))
.withRequestBody(equalToJson("{ \"name\": \"John Doe\", \"email\": \"john@example.com\" }"))
.willReturn(aResponse()
.withStatus(201)
.withHeader("Content-Type", "application/json")
.withBody("{ \"id\": 1234, \"status\": \"created\" }")));
This stub is like a bouncer at an exclusive club – it only lets in requests that meet very specific criteria. In this case, it’s looking for a POST request to “/api/users” with a JSON body containing a specific name and email. If everything checks out, it responds with a 201 status and some JSON indicating the user was created.
Stubbing with Priorities
Sometimes, you might have multiple stubs that could match a single request. In these cases, you can use priorities to determine which stub should win:
stubFor(get(urlMatching("/api/.*")).atPriority(5)
.willReturn(aResponse().withStatus(404)));
stubFor(get(urlEqualTo("/api/specific-endpoint")).atPriority(1)
.willReturn(aResponse().withStatus(200).withBody("Specific response")));
In this example, we have a catch-all stub for any GET request starting with “/api/”, which returns a 404 status. However, we also have a more specific stub for “/api/specific-endpoint” with a higher priority (lower number). This means that requests to “/api/specific-endpoint” will get the 200 response, while any other “/api/” requests will get the 404. It’s like having a default “Sorry, I don’t understand” response, but with specific answers for questions you do know!
Advanced WireMock Features
Now that we’ve covered the basics, let’s explore some of WireMock’s more advanced features. These are like the secret levels in a video game – not necessary to finish, but they make the experience so much more enjoyable!
Response Templating
WireMock allows you to use Handlebars templates in your responses, which means you can create dynamic responses based on the incoming request. It’s like having a chef who can customize each dish based on the customer’s order!
To enable response templating, you need to use the WireMockConfiguration
:
WireMockConfiguration config = WireMockConfiguration.options()
.extensions(new ResponseTemplateTransformer(false));
WireMockServer wireMockServer = new WireMockServer(config);
Now you can use Handlebars syntax in your responses:
stubFor(get(urlPathEqualTo("/template"))
.willReturn(aResponse()
.withBody("{{request.path.[0]}} {{request.query.foo}}")
.withTransformers("response-template")));
If you send a GET request to “/template?foo=bar”, the response will be “template bar”. It’s like magic, but better because it’s actually just clever programming!
Stateful Behavior
Sometimes you want your mock to remember things and change its behavior based on previous requests. WireMock has got you covered with its stateful behavior feature. It’s like giving your mock server a short-term memory!
Here’s an example of how you might use this to simulate a simple “login” system:
Scenario scenario = new Scenario("Login flow");
stubFor(post(urlEqualTo("/login"))
.inScenario("Login flow")
.whenScenarioStateIs(Scenario.STARTED)
.willReturn(aResponse().withStatus(200).withBody("Logged in"))
.willSetStateTo("Logged in"));
stubFor(get(urlEqualTo("/protected"))
.inScenario("Login flow")
.whenScenarioStateIs("Logged in")
.willReturn(aResponse().withStatus(200).withBody("Protected content")));
stubFor(get(urlEqualTo("/protected"))
.inScenario("Login flow")
.whenScenarioStateIs(Scenario.STARTED)
.willReturn(aResponse().withStatus(401).withBody("Unauthorized")));
In this example, accessing “/protected” will return a 401 status until a POST request is made to “/login”. After that, “/protected” will return a 200 status with the protected content. It’s like having a virtual bouncer who remembers faces!
Fault Simulation
Testing how your application handles errors is crucial, and WireMock makes it easy to simulate various network faults. It’s like being able to control the weather in your test environment!
stubFor(get(urlEqualTo("/flaky"))
.willReturn(aResponse().withFault(Fault.CONNECTION_RESET_BY_PEER)));
stubFor(get(urlEqualTo("/slow"))
.willReturn(aResponse().withFixedDelay(5000))); // 5 second delay
The first stub simulates a connection reset, while the second introduces a 5-second delay. These are great for testing how your application handles timeouts and network issues. It’s like purposely sabotaging your own API, but in a controlled, productive way!
Best Practices for Using WireMock
Now that you’re armed with all this WireMock knowledge, you might be tempted to go wild and mock everything in sight. But hold your horses! Like with any powerful tool, it’s important to use WireMock responsibly. Here are some best practices to keep in mind:
Keep Your Stubs Organized
As your test suite grows, you might find yourself with a large number of stubs. Keeping these organized is crucial for maintaining your tests. Consider grouping related stubs into separate files or classes. You can use WireMock’s StubMapping
class to define stubs programmatically:
public class UserServiceStubs {
public static StubMapping getUserStub() {
return stubFor(get(urlEqualTo("/api/users/1"))
.willReturn(aResponse()
.withStatus(200)
.withHeader("Content-Type", "application/json")
.withBody("{ \"id\": 1, \"name\": \"John Doe\" }")));
}
public static StubMapping createUserStub() {
return stubFor(post(urlEqualTo("/api/users"))
.willReturn(aResponse()
.withStatus(201)
.withHeader("Content-Type", "application/json")
.withBody("{ \"id\": 2, \"status\": \"created\" }")));
}
}
You can then use these stubs in your tests:
@Test
public void testGetUser() {
UserServiceStubs.getUserStub();
// Test code here
}
This approach keeps your test code clean and your stubs reusable. It’s like having a well-organized toolbox – you’ll always know where to find what you need!
Use Verification
WireMock isn’t just about returning mock responses; it can also verify that certain requests were made. This is incredibly useful for ensuring that your code is making the expected calls:
stubFor(post(urlEqualTo("/api/data"))
.willReturn(aResponse().withStatus(200)));
// Your code that should make a POST request to "/api/data"
yourCode.doSomething();
verify(postRequestedFor(urlEqualTo("/api/data"))
.withHeader("Content-Type", equalTo("application/json")));
This verifies that a POST request was made to “/api/data” with a specific Content-Type header. It’s like having a security camera for your API calls!
Don’t Overuse Mocking
While WireMock is a powerful tool, it’s important not to rely on it too heavily. Mocks are great for unit tests and simulating edge cases, but they shouldn’t completely replace integration tests with real services. A balanced approach using both mocked and real services will give you the most comprehensive test coverage. It’s like a healthy diet – variety is key!
Troubleshooting Common WireMock Issues
Even with the best laid plans, sometimes things don’t work quite as expected. Don’t worry, though – we’ve got your back! Here are some common issues you might encounter when using WireMock, and how to solve them:
Stub Not Matching
If your stub isn’t matching as expected, the first step is to enable verbose logging. You can do this by adding the following to your WireMock configuration:
WireMockConfiguration.options().verbose(true)
This will give you detailed information about incoming requests and which stubs (if any) they matched. It’s like turning on the lights in a dark room – suddenly, you can see what’s going on!
Another common cause of mismatches is forgetting to reset the WireMock server between tests. Make sure you’re calling WireMock.reset()
in your test teardown, or use the @After
annotation in JUnit:
@After
public void teardown() {
WireMock.reset();
}
Port Conflicts
If you’re running multiple tests in parallel, you might encounter port conflicts. To avoid this, you can let WireMock choose a random available port:
WireMockRule wireMockRule = new WireMockRule(options().dynamicPort());
You can then get the port number using wireMockRule.port()
. It’s like letting WireMock choose its own seat at the table – it’ll find a spot where it won’t bump elbows with anyone else!
Performance Issues
If you notice your tests running slowly with WireMock, there are a few things you can try:
- Use the standalone JAR: Running WireMock as a standalone process can be faster than in-process for large numbers of stubs.
- Minimize use of complex request matchers: Matchers like
equalToJson
can be slower than simple URL or header matching. - Use response templating judiciously: While powerful, excessive use of templating can slow down response generation.
Remember, a well-optimized WireMock setup should be nearly as fast as the real service it’s mocking. It’s like tuning a race car – small adjustments can lead to big performance gains!
Conclusion
And there you have it, folks – a comprehensive guide to setting up and using WireMock for HTTP mocking. We’ve covered everything from basic setup to advanced features, best practices, and even some troubleshooting tips. By now, you should be well-equipped to harness the power of WireMock in your testing arsenal.
Remember, WireMock is more than just a tool – it’s a game-changer in the world of software testing. With WireMock at your side, you can:
- Create reliable, repeatable tests that aren’t at the mercy of external services
- Simulate edge cases and error scenarios that might be difficult or impossible to trigger with real services
- Develop and test your applications offline, freeing you from the shackles of network connectivity
- Improve the speed and efficiency of your test suite by eliminating network calls and controlling response times
As you continue your journey with WireMock, don’t be afraid to experiment and push the boundaries of what’s possible. Try combining different features, like using response templating with stateful behavior to create complex, dynamic mocks. Or use WireMock’s record and playback functionality to capture real API responses and use them in your tests.
Remember, the goal isn’t to replace your entire API with mocks, but to use mocking strategically to enhance your testing capabilities. Like a master chef choosing the right ingredients, you’ll learn when to use real services and when to reach for WireMock.
As you become more proficient with WireMock, you might even find yourself thinking differently about API design and testing. You might start considering how to make your APIs more mockable, or how to structure your applications to be more easily testable with tools like WireMock.
So go forth and mock with confidence! Your tests will be more robust, your development process more efficient, and your applications more reliable. And who knows? You might even start to enjoy writing tests. (Okay, let’s not get carried away – but you’ll definitely dread them less!)
Thank you for joining me on this deep dive into the world of WireMock. Happy mocking, and may all your tests be green!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of this guide, software and its ecosystem are constantly evolving. The information provided here is based on the latest available knowledge at the time of writing. Please always refer to the official WireMock documentation for the most up-to-date information. If you notice any inaccuracies or have suggestions for improvements, please report them so we can correct them promptly. Remember, in the world of software development, learning is a continuous journey – stay curious and keep exploring!