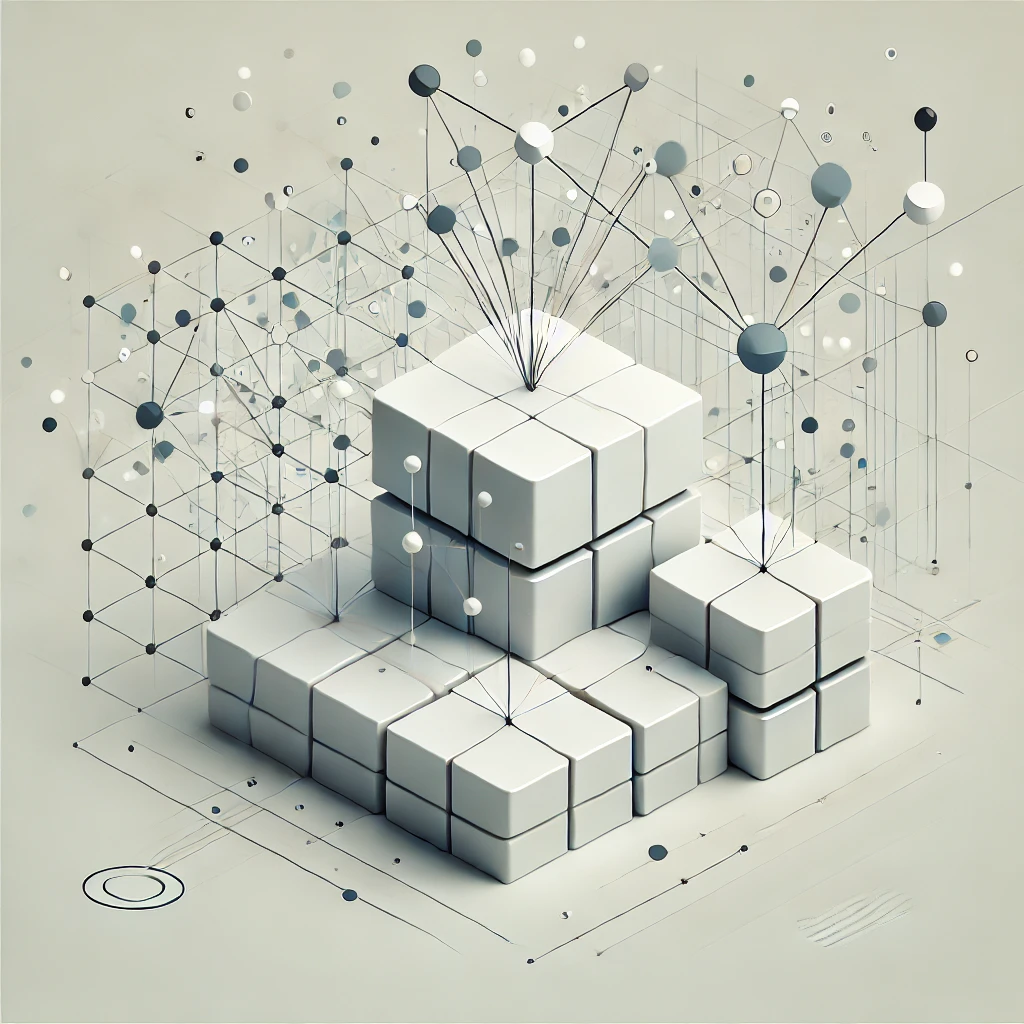
What’s a Data Structure and Why Should I Care?
Have you ever wondered how your favorite apps and websites manage to handle massive amounts of information so quickly and efficiently? Or perhaps you’re a budding programmer looking to level up your skills? Well, my friend, you’re in for a treat! Today, we’re diving into the fascinating world of data structures – the unsung heroes behind the scenes of every successful software application.
The Building Blocks of Efficient Programming
Imagine you’re organizing a massive library. You wouldn’t just toss books onto shelves randomly, right? You’d create a system – maybe alphabetical order, genre categories, or even a complex numbering scheme. That’s exactly what data structures do for information in computer programs. They’re the clever ways we organize and store data to make it easy to access, modify, and manipulate.
But why should you care?
Well, whether you’re a seasoned developer or just starting your coding journey, understanding data structures is like unlocking a superpower. It’s the difference between building a rickety treehouse and constructing a skyscraper. With the right data structures, your programs can:
- Run faster and more efficiently
- Use less memory
- Handle complex problems with elegance
- Scale up to work with massive datasets
So, buckle up! We’re about to embark on a journey through the most important data structures, explore real-world examples, and discover why they’re absolutely crucial for anyone serious about programming.
Arrays: The Simplest Yet Powerful Data Structure
Let’s start with the basics – arrays. Think of an array as a row of boxes, each holding a piece of data. It’s simple, straightforward, and incredibly useful.
When to use arrays:
- When you need to store a fixed number of elements
- For quick access to elements by their position (index)
- To implement other, more complex data structures
Here’s a quick example in Python:
# Creating an array of fruits
fruits = ["apple", "banana", "cherry", "date", "elderberry"]
# Accessing elements
print(fruits[0]) # Output: apple
print(fruits[2]) # Output: cherry
# Modifying elements
fruits[1] = "blueberry"
print(fruits) # Output: ["apple", "blueberry", "cherry", "date", "elderberry"]
# Getting the length
print(len(fruits)) # Output: 5
Arrays are great for situations where you know the size of your data in advance and need quick access to elements. However, they can be inefficient when you need to frequently add or remove elements, especially at the beginning of the array.
Linked Lists: A Chain of Data
Now, let’s move on to linked lists – a data structure that addresses some of the limitations of arrays. Imagine a treasure hunt where each clue points to the location of the next one. That’s essentially how a linked list works!
Key features of linked lists:
- Dynamic size (easy to add or remove elements)
- Efficient insertion and deletion at any position
- Uses more memory than arrays (due to storing pointers)
Here’s a simple implementation of a singly linked list in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def print_list(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
# Usage
my_list = LinkedList()
my_list.append(1)
my_list.append(2)
my_list.append(3)
my_list.print_list() # Output: 1 -> 2 -> 3 -> None
Linked lists shine in scenarios where you need to frequently add or remove elements, especially at the beginning or middle of the list. They’re commonly used in implementing other data structures like stacks and queues.
Stacks: Last In, First Out
Speaking of stacks, let’s dive into this fascinating data structure. Imagine a stack of plates – you always add or remove from the top, right? That’s exactly how a stack data structure works in programming.
Key characteristics of stacks:
- LIFO (Last In, First Out) principle
- Only two main operations: push (add) and pop (remove)
- Great for tracking state or handling recursion
Here’s a simple stack implementation using Python’s built-in list:
class Stack:
def __init__(self):
self.items = []
def is_empty(self):
return len(self.items) == 0
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
else:
return None
def peek(self):
if not self.is_empty():
return self.items[-1]
else:
return None
def size(self):
return len(self.items)
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
Stacks are incredibly useful in many programming scenarios. They’re used in:
- Function call management (call stack)
- Undo mechanisms in software
- Expression evaluation and syntax parsing
- Backtracking algorithms
Understanding stacks can help you tackle complex problems with elegance and efficiency.
Queues: First In, First Out
Now, let’s switch gears and talk about queues. If stacks are like a stack of plates, queues are like a line at a movie theater – first come, first served!
Key features of queues:
- FIFO (First In, First Out) principle
- Main operations: enqueue (add) and dequeue (remove)
- Useful for managing tasks or data that need to be processed in order
Here’s a basic queue implementation in Python:
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def is_empty(self):
return len(self.items) == 0
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
else:
return None
def front(self):
if not self.is_empty():
return self.items[0]
else:
return None
def size(self):
return len(self.items)
# Usage
queue = Queue()
queue.enqueue("Task 1")
queue.enqueue("Task 2")
queue.enqueue("Task 3")
print(queue.dequeue()) # Output: Task 1
print(queue.front()) # Output: Task 2
print(queue.size()) # Output: 2
Queues are essential in many real-world applications:
- Task scheduling in operating systems
- Handling requests in web servers
- Breadth-First Search algorithms in graphs
- Buffering in data streams
By mastering queues, you’ll be well-equipped to handle scenarios that require ordered processing or buffering of data.
Trees: Branching Out
Now that we’ve covered the basics, let’s branch out (pun intended) into more complex territory – trees. In the world of data structures, trees are hierarchical structures that resemble… well, trees!
What makes trees special:
- Hierarchical structure with a root node and child nodes
- Efficient for searching, inserting, and deleting data
- Come in various types (binary trees, AVL trees, B-trees, etc.)
Let’s implement a simple binary tree in Python:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinaryTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = TreeNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert_recursive(node.right, value)
def inorder_traversal(self):
result = []
self._inorder_recursive(self.root, result)
return result
def _inorder_recursive(self, node, result):
if node:
self._inorder_recursive(node.left, result)
result.append(node.value)
self._inorder_recursive(node.right, result)
# Usage
tree = BinaryTree()
tree.insert(5)
tree.insert(3)
tree.insert(7)
tree.insert(1)
tree.insert(9)
print(tree.inorder_traversal()) # Output: [1, 3, 5, 7, 9]
Trees are incredibly versatile and are used in numerous applications:
- File systems in operating systems
- DOM (Document Object Model) in web browsers
- Decision trees in machine learning
- Syntax trees in compilers
Understanding trees opens up a whole new world of possibilities in algorithm design and problem-solving.
Hash Tables: Lightning-Fast Lookups
Have you ever wondered how dictionaries work in programming languages? Or how databases manage to retrieve information so quickly? The secret sauce behind these speedy operations is often the hash table.
What makes hash tables awesome:
- Incredibly fast average-case lookup, insertion, and deletion (O(1))
- Uses a hash function to map keys to array indices
- Handles collisions through various methods (chaining, open addressing)
Let’s implement a simple hash table in Python:
class HashTable:
def __init__(self, size=10):
self.size = size
self.table = [[] for _ in range(self.size)]
def _hash_function(self, key):
return hash(key) % self.size
def insert(self, key, value):
hash_index = self._hash_function(key)
for item in self.table[hash_index]:
if item[0] == key:
item[1] = value
return
self.table[hash_index].append([key, value])
def get(self, key):
hash_index = self._hash_function(key)
for item in self.table[hash_index]:
if item[0] == key:
return item[1]
raise KeyError(key)
def remove(self, key):
hash_index = self._hash_function(key)
for i, item in enumerate(self.table[hash_index]):
if item[0] == key:
del self.table[hash_index][i]
return
raise KeyError(key)
# Usage
ht = HashTable()
ht.insert("apple", 5)
ht.insert("banana", 7)
ht.insert("orange", 3)
print(ht.get("banana")) # Output: 7
ht.remove("apple")
print(ht.get("apple")) # Raises KeyError
Hash tables are the backbone of many high-performance systems:
- Implementing dictionaries and sets in programming languages
- Database indexing for quick data retrieval
- Caching mechanisms in web applications
- Symbol tables in compilers and interpreters
Mastering hash tables can significantly boost your ability to design efficient algorithms and data structures.
Graphs: Connecting the Dots
Last but certainly not least, let’s explore graphs – one of the most versatile and powerful data structures out there. Graphs are all about connections, making them perfect for representing complex relationships.
What makes graphs unique:
- Consist of vertices (nodes) and edges (connections)
- Can be directed or undirected, weighted or unweighted
- Ideal for representing networks, relationships, and paths
Here’s a simple implementation of an undirected graph using an adjacency list:
class Graph:
def __init__(self):
self.graph = {}
def add_vertex(self, vertex):
if vertex not in self.graph:
self.graph[vertex] = []
def add_edge(self, vertex1, vertex2):
if vertex1 not in self.graph:
self.add_vertex(vertex1)
if vertex2 not in self.graph:
self.add_vertex(vertex2)
self.graph[vertex1].append(vertex2)
self.graph[vertex2].append(vertex1)
def remove_edge(self, vertex1, vertex2):
if vertex1 in self.graph and vertex2 in self.graph:
self.graph[vertex1] = [v for v in self.graph[vertex1] if v != vertex2]
self.graph[vertex2] = [v for v in self.graph[vertex2] if v != vertex1]
def get_vertices(self):
return list(self.graph.keys())
def get_edges(self):
edges = []
for vertex in self.graph:
for neighbor in self.graph[vertex]:
if {vertex, neighbor} not in edges:
edges.append({vertex, neighbor})
return edges
# Usage
g = Graph()
g.add_edge('A', 'B')
g.add_edge('B', 'C')
g.add_edge('C', 'D')
g.add_edge('D', 'A')
print(g.get_vertices()) # Output: ['A', 'B', 'C', 'D']
print(g.get_edges()) # Output: [{'A', 'B'}, {'B', 'C'}, {'C', 'D'}, {'A', 'D'}]
Graphs are used in countless real-world applications:
- Social networks (friends connections)
- GPS and mapping systems (finding shortest paths)
- Recommendation systems (suggesting products or content)
- Network topology in computer networks
Understanding graphs and graph algorithms can give you a significant edge in solving complex problems across various domains.
Why Data Structures Matter: The Big Picture
Now that we’ve explored some of the most important data structures, let’s zoom out and look at the big picture. Why should you, as a programmer or aspiring developer, care about all this?
Efficiency and Performance:
Choosing the right data structure can make the difference between an application that runs smoothly and one that crawls along at a snail’s pace. As datasets grow larger and user expectations for speed increase, efficient data structures become more critical than ever.
Problem-Solving Skills:
Understanding data structures sharpens your problem-solving abilities. It gives you a toolkit of approaches to tackle complex challenges. When you encounter a new problem, you’ll be able to analyze it and choose the most appropriate data structure to solve it efficiently.
Foundation for Advanced Concepts:
Many advanced programming concepts and algorithms build upon these fundamental data structures. Whether you’re diving into machine learning, working with big data, or developing high-performance systems, a solid grasp of data structures is essential.
Interviews and Career Advancement:
Let’s face it – knowledge of data structures is often a key component in technical interviews. Companies want to ensure that their developers can write efficient, scalable code. Mastering these concepts can give you a significant edge in your career.
Understanding Existing Systems:
As you work with various programming languages and frameworks, you’ll encounter these data structures under the hood. Understanding how they work allows you to use them more effectively and debug issues more easily.
Conclusion: Your Journey with Data Structures
We’ve covered a lot of ground, from the simple yet powerful arrays to the complex and versatile graphs. Each data structure has its strengths and ideal use cases. As you continue your programming journey, you’ll find yourself reaching for different tools in this toolbox to solve various problems.
Remember, becoming proficient with data structures is a journey, not a destination. The key is to practice implementing them, use them in real projects, and continuously challenge yourself to optimize your code.
So, the next time you’re working on a project, take a moment to consider: “What’s the most appropriate data structure for this task?” Your future self (and your users) will thank you for the efficient, elegant solutions you create.
Disclaimer: While every effort has been made to ensure the accuracy and completeness of the information in this blog post, programming and computer science are rapidly evolving fields. The concepts and implementations discussed here are intended for educational purposes and may not represent the most optimized or up-to-date solutions for all scenarios. Readers are encouraged to further research and adapt these concepts to their specific needs. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly. Happy coding!