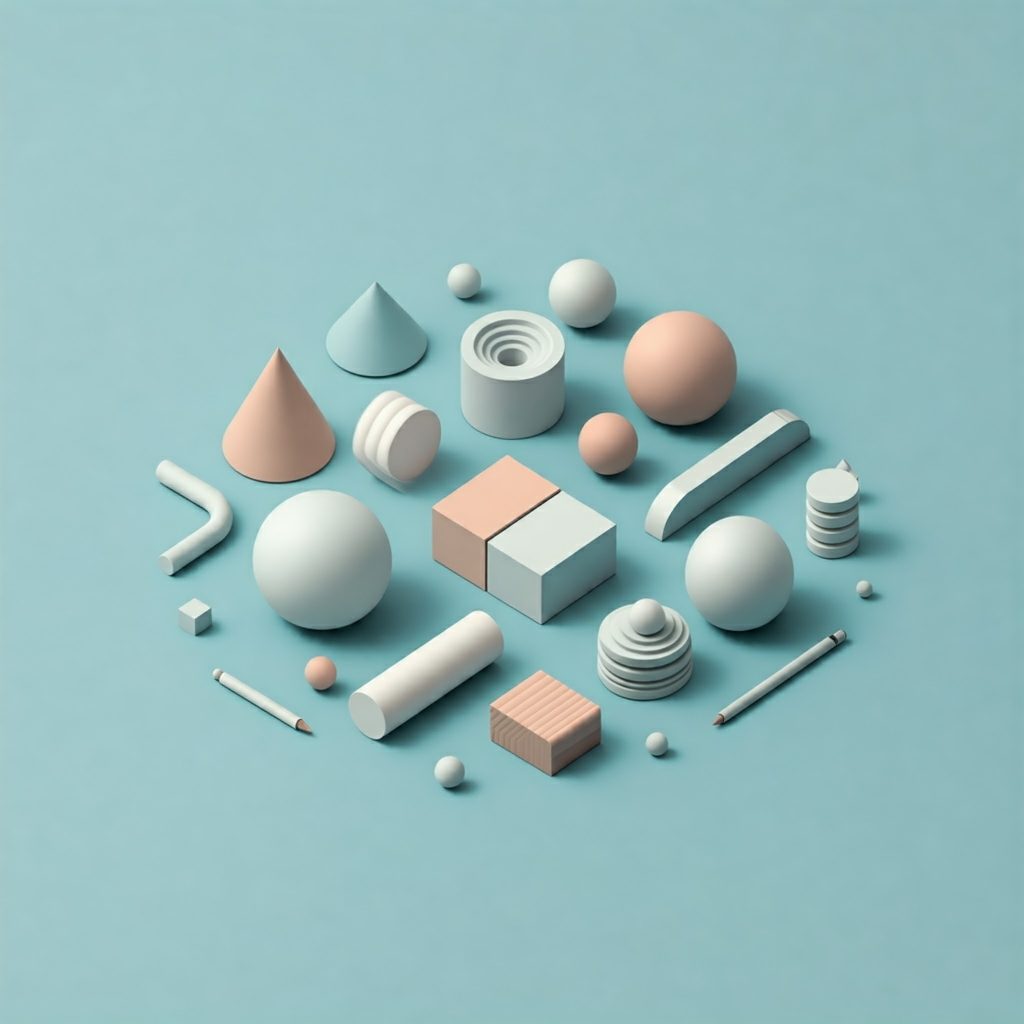
Working with Data Types in SQL – Understanding Different Data Types and Their Usage
As organizations increasingly rely on structured data, understanding SQL (Structured Query Language) and its data types becomes crucial for database management. Data types play a pivotal role in ensuring the integrity, accuracy, and efficiency of databases. Choosing the correct data type for a column can optimize storage space, speed up queries, and improve performance, while incorrect choices can lead to inefficient queries and storage issues. This article provides a comprehensive guide on working with different data types in SQL, explaining their functions and best use cases.
Understanding SQL Data Types
SQL data types define the nature of data that can be stored in a particular column within a table. Different databases, like MySQL, PostgreSQL, SQL Server, and Oracle, have slight variations in their data types, but the core principles remain consistent.
The data types can broadly be classified into five categories:
- Numeric Data Types
- String Data Types
- Date and Time Data Types
- Binary Data Types
- Miscellaneous Data Types
1. Numeric Data Types
Numeric data types are used to store numbers, which may include integers, floating-point numbers, and decimals. The precise selection of a numeric data type depends on the nature of the data being stored and the required precision.
Integer Data Types
Data Type | Description | Range | Size (Bytes) |
---|---|---|---|
TINYINT | Small integer | -128 to 127 | 1 |
SMALLINT | Larger than TINYINT | -32,768 to 32,767 | 2 |
MEDIUMINT | Larger than SMALLINT | -8,388,608 to 8,388,607 | 3 |
INT | Standard integer data type | -2,147,483,648 to 2,147,483,647 | 4 |
BIGINT | Large integer | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | 8 |
Usage Example:
CREATE TABLE employees (
id INT PRIMARY KEY,
age TINYINT,
salary BIGINT
);
Key Points:
- Use
TINYINT
orSMALLINT
for fields that store small numerical values like age or rating. - Use
INT
when the number range is wide but not extreme. BIGINT
should be used for values with a potentially large range, such as financial calculations.
Floating-Point Data Types
Floating-point data types are used when precision and the ability to store fractional numbers are required.
Data Type | Description | Size (Bytes) |
---|---|---|
FLOAT | Single-precision floating-point | 4 |
DOUBLE | Double-precision floating-point | 8 |
DECIMAL | Fixed-point numbers, suitable for currency | Varies by precision |
Usage Example:
CREATE TABLE products (
product_id INT PRIMARY KEY,
price DECIMAL(10, 2),
discount FLOAT
);
Key Points:
FLOAT
is suitable for approximate values where absolute precision is not necessary, such as scientific measurements.DOUBLE
offers higher precision and is suitable for mathematical calculations requiring precision.DECIMAL
is preferable for financial calculations, ensuring accurate results for transactions or currency values.
2. String Data Types
String data types are used to store text data. Depending on the database’s requirements, you can choose from several string types based on length, indexing needs, and performance considerations.
Character String Types
Data Type | Description | Max Length | Usage |
---|---|---|---|
CHAR | Fixed-length string | 255 characters | Suitable for fixed-size fields like country codes |
VARCHAR | Variable-length string | 65,535 characters | Suitable for general text fields like names or addresses |
Usage Example:
CREATE TABLE users (
user_id INT PRIMARY KEY,
username VARCHAR(50),
password CHAR(64)
);
Key Points:
- Use
CHAR
for data that has a fixed length, like codes, where all entries are the same size. VARCHAR
is best suited for text fields of varying length, such as names or descriptions.
Text Data Types
Text data types store longer strings of text.
Data Type | Description | Max Length |
---|---|---|
TEXT | Variable-length long text | 65,535 characters |
MEDIUMTEXT | Medium-length text | 16,777,215 characters |
LONGTEXT | Long text data | 4,294,967,295 characters |
Usage Example:
CREATE TABLE articles (
article_id INT PRIMARY KEY,
title VARCHAR(255),
content LONGTEXT
);
Key Points:
TEXT
is ideal for fields that store large blocks of text, like blog content or product descriptions.MEDIUMTEXT
andLONGTEXT
are reserved for very large text entries, such as documents or extensive descriptions.
3. Date and Time Data Types
Handling dates and times accurately is crucial for time-sensitive data such as transaction records, event logging, and scheduling.
Data Type | Description | Usage |
---|---|---|
DATE | Date only (YYYY-MM-DD) | Birthdates, event dates |
TIME | Time only (HH:MM:SS) | Storing specific times |
DATETIME | Combined date and time (YYYY-MM-DD HH:MM:SS) | Timestamp for events |
TIMESTAMP | Date and time, auto-updated | Automatically stores update times |
YEAR | Stores year values (YYYY) | Specific years |
Usage Example:
CREATE TABLE appointments (
appointment_id INT PRIMARY KEY,
appointment_date DATE,
appointment_time TIME,
last_updated TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
);
Key Points:
- Use
DATE
when only the date is needed, such as for birthdates or anniversaries. DATETIME
is useful for storing event logs or records of transactions.TIMESTAMP
is ideal for tracking changes, as it can automatically update whenever a record is modified.
4. Binary Data Types
Binary data types are used to store data in raw binary format, typically for images, files, or other multimedia data.
Data Type | Description | Max Length |
---|---|---|
BINARY | Fixed-length binary data | 255 bytes |
VARBINARY | Variable-length binary data | 65,535 bytes |
BLOB | Binary large object | 65,535 bytes |
MEDIUMBLOB | Medium binary large object | 16,777,215 bytes |
LONGBLOB | Long binary large object | 4,294,967,295 bytes |
Usage Example:
CREATE TABLE files (
file_id INT PRIMARY KEY,
file_name VARCHAR(255),
file_data LONGBLOB
);
Key Points:
BLOB
types are commonly used for storing multimedia, such as images, videos, and audio.- Use
BINARY
orVARBINARY
for smaller binary data that requires precise storage.
5. Miscellaneous Data Types
SQL databases offer some additional data types to handle specific use cases, such as enumerations or special values.
Enumerated Data Type
ENUM
defines a static set of values that a field can accept, which is useful for limiting options.
Data Type | Description | Usage |
---|---|---|
ENUM | String object with a predefined set of values | Gender, status, categories |
Usage Example:
CREATE TABLE products (
product_id INT PRIMARY KEY,
category ENUM('Electronics', 'Furniture', 'Clothing')
);
Set Data Type
The SET
type is used when a field can store multiple values, but each value must come from a predefined set of values.
Usage Example:
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
skills SET('SQL', 'Python', 'Java')
);
Key Points:
ENUM
is efficient when you need to restrict values to a specific list, ensuring data consistency.- The
SET
type is useful for storing multiple attributes in a field while maintaining constraint to a set of valid options.
Best Practices for Choosing Data Types
Choosing the appropriate data type is essential to ensuring a database’s performance, scalability, and accuracy. Consider the following best practices:
- Optimize Storage: Select the smallest data type that can reliably store the data to save space. For example, instead of
BIGINT
, useTINYINT
orSMALLINT
when appropriate. - Maintain Precision: For financial and mathematical operations, use
DECIMAL
rather than floating-point types likeFLOAT
orDOUBLE
, as rounding errors can occur. - Consider Future Expansion: When choosing string types like
VARCHAR
, account for potential data growth, but avoid over-allocating memory unnecessarily. - Use Constraints and Validations: Wherever possible, combine the right data types with constraints (like
NOT NULL
,UNIQUE
, andCHECK
) to enforce business rules and maintain data integrity.
Conclusion
Understanding and selecting the appropriate data types in SQL is fundamental to designing efficient, scalable, and accurate databases. Each data type has unique properties that make it suitable for specific types of data, whether numeric, text-based, or time-related. By carefully considering the nature of your data and following best practices, you can optimize your database’s performance and ensure data integrity.
By mastering SQL data types, you can improve query performance, reduce storage costs, and enhance the overall reliability of your database applications.
Disclaimer: The information provided in this blog is intended for educational purposes only. Please report any inaccuracies or issues so we can promptly correct them.