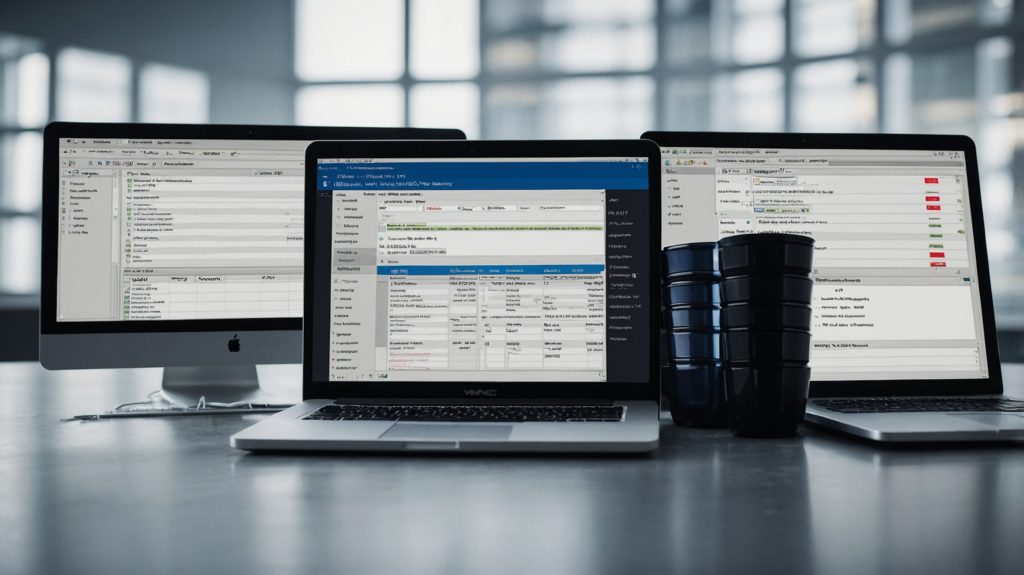
Working with Databases in MVC
Database integration stands as a cornerstone of modern web application development, particularly within the Model-View-Controller (MVC) architectural pattern. Understanding how to effectively work with databases in an MVC context is crucial for developers aiming to build scalable, maintainable, and efficient applications. This comprehensive guide explores various approaches to database integration, from Object-Relational Mapping (ORM) frameworks to direct database connections, providing practical examples in both Python and Java. We’ll delve into best practices, common pitfalls, and implementation strategies that will help you make informed decisions about database integration in your MVC applications.
Understanding Database Integration in MVC
The Model-View-Controller pattern separates application logic into three distinct components, with the Model layer being responsible for data handling and business logic. In database-driven applications, the Model layer interfaces with the database, handling data persistence, retrieval, and manipulation. This separation of concerns ensures that database operations remain isolated from the presentation layer (View) and application flow control (Controller).
ORM vs. Direct Database Connections
ORM Approach
Object-Relational Mapping provides an abstraction layer between your application code and the database, allowing you to work with database records as if they were regular programming objects. This approach offers several advantages, including:
Feature | ORM | Direct Connection |
---|---|---|
Learning Curve | Steeper initial learning curve | Simpler to start with |
Maintenance | Easier to maintain | Requires more manual maintenance |
Performance | May have overhead | Generally better performance |
Productivity | Higher developer productivity | More control but slower development |
Database Agnostic | Yes | No |
Let’s look at practical examples using popular ORMs in both Python and Java.
Python Implementation with SQLAlchemy
SQLAlchemy is one of the most powerful and widely-used ORMs for Python. Here’s a comprehensive example of implementing database operations in an MVC structure:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Database Configuration
engine = create_engine('postgresql://user:password@localhost/dbname')
Base = declarative_base()
Session = sessionmaker(bind=engine)
# Model Definition
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
username = Column(String(50), unique=True)
email = Column(String(120), unique=True)
def __repr__(self):
return f"<User(username='{self.username}', email='{self.email}')>"
# Model Class (Repository Pattern)
class UserModel:
def __init__(self):
self.session = Session()
def create_user(self, username, email):
user = User(username=username, email=email)
try:
self.session.add(user)
self.session.commit()
return user
except Exception as e:
self.session.rollback()
raise e
def get_user_by_id(self, user_id):
return self.session.query(User).filter(User.id == user_id).first()
def update_user(self, user_id, username=None, email=None):
user = self.get_user_by_id(user_id)
if user:
if username:
user.username = username
if email:
user.email = email
try:
self.session.commit()
return user
except Exception as e:
self.session.rollback()
raise e
return None
# Controller
class UserController:
def __init__(self):
self.user_model = UserModel()
def register_user(self, username, email):
try:
user = self.user_model.create_user(username, email)
return {'status': 'success', 'user': str(user)}
except Exception as e:
return {'status': 'error', 'message': str(e)}
def get_user(self, user_id):
user = self.user_model.get_user_by_id(user_id)
if user:
return {'status': 'success', 'user': str(user)}
return {'status': 'error', 'message': 'User not found'}
Java Implementation with Spring Data JPA
Spring Data JPA provides a powerful and flexible ORM solution for Java applications. Here’s an example implementation:
// Entity (Model)
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(unique = true)
private String username;
@Column(unique = true)
private String email;
// Getters and setters
}
// Repository Interface
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
Optional<User> findByEmail(String email);
}
// Service Layer (Model operations)
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User createUser(String username, String email) {
User user = new User();
user.setUsername(username);
user.setEmail(email);
return userRepository.save(user);
}
public Optional<User> getUserById(Long id) {
return userRepository.findById(id);
}
public User updateUser(Long id, String username, String email) {
return userRepository.findById(id)
.map(user -> {
if (username != null) {
user.setUsername(username);
}
if (email != null) {
user.setEmail(email);
}
return userRepository.save(user);
})
.orElseThrow(() -> new RuntimeException("User not found"));
}
}
// Controller
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody UserDTO userDTO) {
User user = userService.createUser(userDTO.getUsername(), userDTO.getEmail());
return ResponseEntity.ok(user);
}
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
return userService.getUserById(id)
.map(ResponseEntity::ok)
.orElse(ResponseEntity.notFound().build());
}
}
Direct Database Connection Approach
While ORMs provide convenience, sometimes direct database connections offer better performance and more control. Here’s how to implement direct database connections in both Python and Java:
Python with psycopg2:
import psycopg2
from psycopg2.extras import DictCursor
class DatabaseConnection:
def __init__(self):
self.conn = psycopg2.connect(
dbname="your_db",
user="your_user",
password="your_password",
host="localhost"
)
def __enter__(self):
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.conn.close()
class UserModel:
@staticmethod
def create_user(username, email):
with DatabaseConnection() as db:
with db.conn.cursor(cursor_factory=DictCursor) as cur:
cur.execute(
"INSERT INTO users (username, email) VALUES (%s, %s) RETURNING id",
(username, email)
)
user_id = cur.fetchone()['id']
db.conn.commit()
return user_id
@staticmethod
def get_user_by_id(user_id):
with DatabaseConnection() as db:
with db.conn.cursor(cursor_factory=DictCursor) as cur:
cur.execute(
"SELECT * FROM users WHERE id = %s",
(user_id,)
)
return cur.fetchone()
Java with JDBC:
public class DatabaseConnection {
private static final String URL = "jdbc:postgresql://localhost:5432/your_db";
private static final String USER = "your_user";
private static final String PASSWORD = "your_password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
}
public class UserDAO {
public User createUser(String username, String email) throws SQLException {
String sql = "INSERT INTO users (username, email) VALUES (?, ?) RETURNING id";
try (Connection conn = DatabaseConnection.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, username);
pstmt.setString(2, email);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
User user = new User();
user.setId(rs.getLong("id"));
user.setUsername(username);
user.setEmail(email);
return user;
}
}
return null;
}
public User getUserById(Long id) throws SQLException {
String sql = "SELECT * FROM users WHERE id = ?";
try (Connection conn = DatabaseConnection.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setLong(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
User user = new User();
user.setId(rs.getLong("id"));
user.setUsername(rs.getString("username"));
user.setEmail(rs.getString("email"));
return user;
}
}
return null;
}
}
Best Practices and Considerations
Connection Pooling
Connection pooling is crucial for maintaining optimal database performance. Here’s how to implement it in both frameworks:
# Python with SQLAlchemy
from sqlalchemy import create_engine
from sqlalchemy.pool import QueuePool
engine = create_engine('postgresql://user:password@localhost/dbname',
poolclass=QueuePool,
pool_size=5,
max_overflow=10,
pool_timeout=30)
// Java with HikariCP
@Configuration
public class DatabaseConfig {
@Bean
public DataSource dataSource() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost:5432/your_db");
config.setUsername("your_user");
config.setPassword("your_password");
config.setMaximumPoolSize(10);
config.setMinimumIdle(5);
config.setIdleTimeout(300000);
return new HikariDataSource(config);
}
}
Security Considerations
When working with databases in MVC applications, security should be a top priority. Here are key considerations:
- **SQL Injection Prevention**: Always use parameterized queries or ORM features to prevent SQL injection attacks.
- **Connection Security**: Use encrypted connections and strong authentication methods.
- **Data Validation**: Implement thorough input validation before performing database operations.
- **Access Control**: Implement proper authorization checks at both the controller and model levels.
Performance Optimization
Query Optimization Tips:
Technique | Description | Implementation |
---|---|---|
Lazy Loading | Load related data only when needed | Use ORM’s lazy loading features |
Eager Loading | Load related data in advance | Implement join queries |
Caching | Cache frequently accessed data | Use caching frameworks |
Indexing | Create appropriate database indexes | Analyze query patterns |
Error Handling and Logging
Proper error handling and logging are essential for maintaining robust database operations:
# Python example with logging
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class UserModel:
def create_user(self, username, email):
try:
user = User(username=username, email=email)
self.session.add(user)
self.session.commit()
logger.info(f"User created successfully: {username}")
return user
except Exception as e:
logger.error(f"Error creating user: {str(e)}")
self.session.rollback()
raise
// Java example with SLF4J
@Slf4j
@Service
public class UserService {
public User createUser(String username, String email) {
try {
User user = new User();
user.setUsername(username);
user.setEmail(email);
User savedUser = userRepository.save(user);
log.info("User created successfully: {}", username);
return savedUser;
} catch (Exception e) {
log.error("Error creating user: {}", e.getMessage(), e);
throw new RuntimeException("Failed to create user", e);
}
}
}
Testing Database Integration
Implementing comprehensive tests for database operations is crucial:
# Python example using pytest
import pytest
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
@pytest.fixture
def test_db():
engine = create_engine('sqlite:///:memory:')
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
return Session()
def test_create_user(test_db):
user_model = UserModel()
user_model.session = test_db
user = user_model.create_user("testuser", "test@example.com")
assert user.username == "testuser"
assert user.email == "test@example.com"
// Java example using JUnit and H2
@SpringBootTest
@AutoConfigureTestDatabase(replace = AutoConfigureTestDatabase.Replace.ANY)
public class UserServiceTest {
@Autowired
private UserService userService;
@Test
public void testCreateUser() {
User user = userService.createUser("testuser", "test@example.com");
assertNotNull(user);
assertEquals("testuser", user.getUsername());
assertEquals("test@example.com", user.getEmail());
}
}
Conclusion
Working with databases in MVC applications requires careful consideration of various factors, including the choice between ORMs and direct connections, security measures, performance optimization, and proper testing. By following the best practices and implementation patterns outlined in this guide, you can create robust, secure, and efficient database integrations in your MVC applications.
Disclaimer: The code examples and best practices provided in this blog post are based on current industry standards and common implementations. While we strive for accuracy, specific requirements and contexts may necessitate different approaches. Please report any inaccuracies or suggestions for improvement to help us maintain the quality of this content.