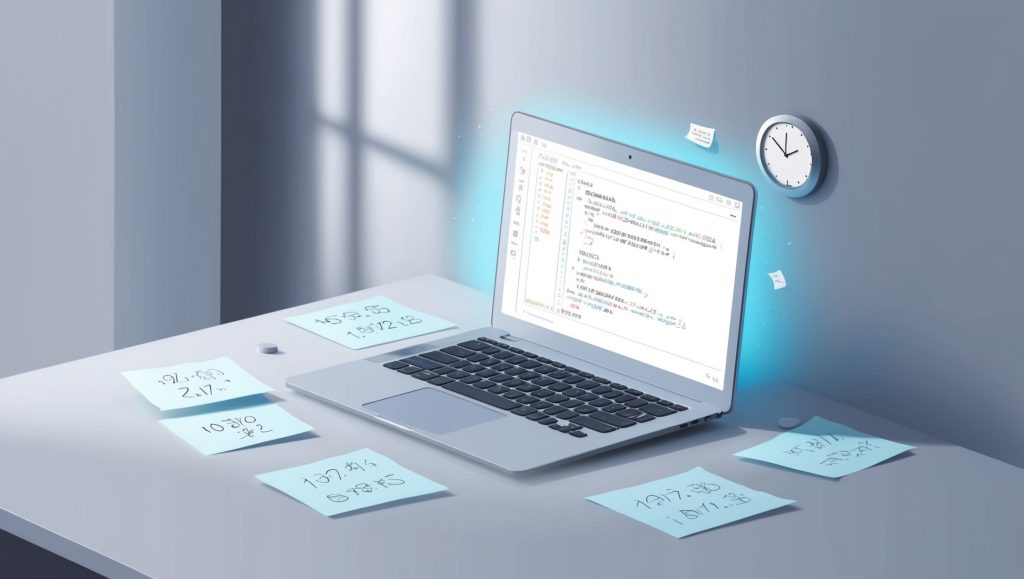
Working with Dates and Times in SQL
Working with dates and times in SQL is a fundamental skill that every database developer and data analyst must master. Temporal data plays a crucial role in various business applications, from tracking transactions and user activities to generating reports and analyzing trends. The complexity of handling dates and times stems from multiple factors, including different date formats, time zones, and the need for precise calculations. This comprehensive guide will explore the various aspects of working with dates and times in SQL, providing practical examples and best practices for manipulating temporal data effectively. Whether you’re a beginner looking to understand the basics or an experienced developer seeking to enhance your skills, this guide will help you navigate the intricacies of temporal data management in SQL.
Understanding Date and Time Data Types
SQL provides several data types for storing temporal information, each serving specific purposes and offering different levels of precision. Understanding these data types is crucial for designing efficient database schemas and performing accurate calculations. The commonly used date and time data types include DATE, TIME, DATETIME, TIMESTAMP, and INTERVAL, though the exact names and behaviors may vary slightly across different database management systems.
Common Date and Time Data Types:
-- Examples of different date and time data types
CREATE TABLE temporal_example (
date_only DATE, -- Stores date without time
time_only TIME, -- Stores time without date
date_time DATETIME, -- Stores both date and time
time_stamp TIMESTAMP, -- Stores date and time with timezone
time_interval INTERVAL -- Stores duration or time period
);
Date and Time Functions
Modern SQL implementations provide a rich set of built-in functions for manipulating and formatting temporal data. These functions enable developers to perform various operations, from simple date extraction to complex calculations involving multiple time zones. Understanding these functions is essential for effective date and time manipulation in SQL applications.
Current Date and Time:
-- Getting current date and time
SELECT
CURRENT_DATE, -- Current date
CURRENT_TIME, -- Current time
CURRENT_TIMESTAMP, -- Current date and time
NOW(); -- Current date and time (alternative)
Extracting Components:
-- Extracting specific parts from a datetime value
SELECT
EXTRACT(YEAR FROM CURRENT_DATE) as year,
EXTRACT(MONTH FROM CURRENT_DATE) as month,
EXTRACT(DAY FROM CURRENT_DATE) as day,
EXTRACT(HOUR FROM CURRENT_TIMESTAMP) as hour,
EXTRACT(MINUTE FROM CURRENT_TIMESTAMP) as minute,
EXTRACT(SECOND FROM CURRENT_TIMESTAMP) as second;
Date Arithmetic and Calculations
One of the most common tasks when working with temporal data is performing date arithmetic. SQL provides various operators and functions for adding or subtracting time intervals, calculating the difference between dates, and handling time periods. These calculations are essential for many business applications, such as determining durations, scheduling events, and analyzing time-based patterns.
Adding and Subtracting Intervals:
-- Adding intervals to dates
SELECT
CURRENT_DATE + INTERVAL '1 day' as tomorrow,
CURRENT_DATE + INTERVAL '1 week' as next_week,
CURRENT_DATE + INTERVAL '1 month' as next_month,
CURRENT_DATE + INTERVAL '1 year' as next_year;
-- Subtracting intervals from dates
SELECT
CURRENT_DATE - INTERVAL '1 day' as yesterday,
CURRENT_DATE - INTERVAL '1 week' as last_week,
CURRENT_DATE - INTERVAL '1 month' as last_month,
CURRENT_DATE - INTERVAL '1 year' as last_year;
Calculating Date Differences:
-- Calculating differences between dates
SELECT
DATE '2024-12-31' - DATE '2024-01-01' as days_difference,
AGE(DATE '2024-12-31', DATE '2024-01-01') as age_interval,
DATEDIFF('month', '2024-01-01', '2024-12-31') as months_between;
Date Formatting and Converting
Different applications and systems may require dates and times to be displayed in specific formats. SQL provides various functions for formatting temporal data and converting between different date representations. Understanding these formatting options is crucial for creating user-friendly outputs and ensuring compatibility between different systems.
Date Formatting Examples:
-- Converting dates to strings with different formats
SELECT
TO_CHAR(CURRENT_DATE, 'MM/DD/YYYY') as us_format,
TO_CHAR(CURRENT_DATE, 'DD/MM/YYYY') as uk_format,
TO_CHAR(CURRENT_DATE, 'YYYY-MM-DD') as iso_format,
TO_CHAR(CURRENT_TIMESTAMP, 'HH24:MI:SS') as time_24hr,
TO_CHAR(CURRENT_TIMESTAMP, 'HH12:MI:SS AM') as time_12hr;
Format Pattern | Description | Example Output |
---|---|---|
YYYY | 4-digit year | 2024 |
MM | 2-digit month | 03 |
DD | 2-digit day | 15 |
HH24 | Hour (24-hour) | 14 |
HH12 | Hour (12-hour) | 02 |
MI | Minutes | 30 |
SS | Seconds | 45 |
TZ | Timezone | UTC |
Working with Time Zones
In today’s globalized world, handling time zones correctly is crucial for many applications. SQL provides functions and data types for working with time zones, enabling developers to store and manipulate temporal data while accounting for different geographical locations and daylight saving time rules.
Time Zone Manipulation:
-- Converting between time zones
SELECT
CURRENT_TIMESTAMP AT TIME ZONE 'UTC' as utc_time,
CURRENT_TIMESTAMP AT TIME ZONE 'America/New_York' as ny_time,
CURRENT_TIMESTAMP AT TIME ZONE 'Europe/London' as london_time,
CURRENT_TIMESTAMP AT TIME ZONE 'Asia/Tokyo' as tokyo_time;
-- Setting session time zone
SET TIME ZONE 'UTC';
Common Date and Time Queries
Certain date and time queries are frequently used in business applications. Understanding how to write these queries efficiently can help developers create more effective database applications. Here are some common scenarios and their SQL solutions.
Finding Records Within Date Ranges:
-- Records from the last 7 days
SELECT *
FROM transactions
WHERE transaction_date >= CURRENT_DATE - INTERVAL '7 days'
AND transaction_date < CURRENT_DATE;
-- Records for the current month
SELECT *
FROM transactions
WHERE EXTRACT(YEAR FROM transaction_date) = EXTRACT(YEAR FROM CURRENT_DATE)
AND EXTRACT(MONTH FROM transaction_date) = EXTRACT(MONTH FROM CURRENT_DATE);
Grouping by Time Periods:
-- Monthly aggregation
SELECT
DATE_TRUNC('month', transaction_date) as month,
COUNT(*) as transaction_count,
SUM(amount) as total_amount
FROM transactions
GROUP BY DATE_TRUNC('month', transaction_date)
ORDER BY month;
-- Weekly aggregation
SELECT
DATE_TRUNC('week', transaction_date) as week,
COUNT(*) as transaction_count,
SUM(amount) as total_amount
FROM transactions
GROUP BY DATE_TRUNC('week', transaction_date)
ORDER BY week;
Performance Considerations
When working with dates and times in SQL, performance optimization is crucial, especially when dealing with large datasets. Several factors can affect query performance, including index usage, function calls, and data type conversions. Understanding these considerations helps in writing more efficient queries.
Indexing Temporal Columns:
-- Creating indexes for date columns
CREATE INDEX idx_transaction_date
ON transactions(transaction_date);
-- Creating indexes for specific date extractions
CREATE INDEX idx_transaction_year_month
ON transactions(EXTRACT(YEAR FROM transaction_date),
EXTRACT(MONTH FROM transaction_date));
Optimizing Date Range Queries:
-- Using BETWEEN for inclusive ranges
SELECT *
FROM transactions
WHERE transaction_date BETWEEN '2024-01-01' AND '2024-12-31';
-- Using half-open intervals for exclusive ranges
SELECT *
FROM transactions
WHERE transaction_date >= '2024-01-01'
AND transaction_date < '2025-01-01';
Best Practices and Common Pitfalls
Working with dates and times requires attention to detail and awareness of common issues that can arise. Following best practices and avoiding common pitfalls can help ensure accurate and maintainable code.
Best Practices:
- Always store dates in UTC when dealing with multiple time zones
- Use appropriate data types for temporal data
- Be consistent with date formats throughout the application
- Consider time zones when comparing dates
- Use indexed columns for date range queries
Common Pitfalls to Avoid:
- Mixing different date formats in comparisons
- Ignoring time zones in temporal calculations
- Using string operations instead of proper date functions
- Not accounting for daylight saving time
- Assuming all months have the same number of days
Advanced Date and Time Techniques
For complex applications, developers often need to implement advanced date and time manipulation techniques. These might include handling fiscal years, calculating business days, or implementing custom calendar logic.
Calculating Business Days:
CREATE FUNCTION business_days_between(start_date DATE, end_date DATE)
RETURNS INTEGER AS $$
DECLARE
days INTEGER;
BEGIN
days := 0;
WHILE start_date <= end_date LOOP
IF EXTRACT(DOW FROM start_date) NOT IN (0, 6) THEN
days := days + 1;
END IF;
start_date := start_date + INTERVAL '1 day';
END LOOP;
RETURN days;
END;
$$ LANGUAGE plpgsql;
Fiscal Year Calculations:
-- Assuming fiscal year starts in April
SELECT
transaction_date,
CASE
WHEN EXTRACT(MONTH FROM transaction_date) >= 4 THEN
EXTRACT(YEAR FROM transaction_date)
ELSE
EXTRACT(YEAR FROM transaction_date) - 1
END as fiscal_year
FROM transactions;
Conclusion
Working with dates and times in SQL requires a solid understanding of various concepts, from basic date manipulation to complex temporal calculations. This guide has covered the essential aspects of handling temporal data, including data types, functions, formatting, time zones, and performance considerations. By following these practices and being aware of common pitfalls, developers can create more robust and efficient applications that handle temporal data correctly.
Disclaimer: This guide is based on standard SQL syntax and common database management systems. Specific implementations may vary across different database platforms. While every effort has been made to ensure accuracy, please consult your database system’s documentation for precise syntax and behavior. If you notice any inaccuracies or have suggestions for improvement, please report them to us for prompt correction.